The content of this article is about how JavaScript determines palindrome strings (example analysis). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
Paindromes(Paindromes), in Chinese, means that the backward and forward readings are the same, and symmetrical, such as "Shanghai tap water comes from the sea"; in English, It refers to words that are the same when viewed frontally and backwards, such as "madam"; and for numbers, also called palindromes, they refer to a symmetrical number like "16461", that is, the digits of this number are in reverse order. The number obtained after rearranging the order is the same as the original number.
Problem description
Judge the given string. If the string is a Palindromes, then return true
, otherwise return false
.
Implementation method
1. reverse()
function Palindromes(str) { let reg = /[\W_]/g; // \w 匹配所有字母和数字以及下划线; \W与之相反; [\W_] 表示匹配下划线或者所有非字母非数字中的任意一个;/g全局匹配 let newStr = str.replace(reg, '').toLowerCase(); let reverseStr = newStr.split('').reverse().join('') return reverseStr === newStr; // 与 newStr 对比 }
Actually, many steps are done here to operate the array, converting characters to arrays, flipping arrays and then converting to strings, so here Performance isn't great either. It is thought that the array is a reference type. To change the array, a new heap address space needs to be opened.
2. for loop
function Palindromes(str) { let reg = /[\W_]/g; let newStr = str.replace(reg, '').toLowerCase(); for(let i = 0, len = Math.floor(newStr.length / 2); i
Writing method 2
function Palindromes(str) { let reg = /[\W_]/g; let newStr = str.replace(reg, '').toLowerCase(); let len = newStr.length; for(let i = 0, j = len - 1; i <h4 id="Recursion">3. Recursion</h4><pre class="brush:php;toolbar:false">function palin(str) { let reg = /[\W_]/g; let newStr = str.replace(reg, '').toLowerCase(); let len = newStr.length; while(len >= 1) { console.log('--') if(newStr[0] != newStr[len - 1]) { // len = 0; // 为了终止 while 循环 否则会陷入死循环 return false; } else { return palin(newStr.slice(1, len - 1)); // 截掉收尾字符 再次比较收尾字符是否相等 // 直到字符串剩下一个字符(奇数项)或者 0 个字符(偶数项) } } return true; }
Give the question Add another requirement
For a given string, you can delete at most one character to determine whether it is still a palindrome.
Set a variable flag. When the pair of characters on both sides are found to be different for the first time, the comparison can be continued; if differences are found in subsequent comparisons, the result will be returned immediately.
function palin(str) { let flag = false; // 第一次不同可允许 let len = str.length; for(let [i, j] = [0, len - 1]; i <p>Related recommendations: <br></p><p><a href="http://www.php.cn/php-notebook-178476.html" target="_self">PHP determines whether a string is a palindrome string</a><br></p><p><a href="http://www.php.cn/js-tutorial-8061.html" target="_self">JavaScript search String and return search results to a string method_javascript skills</a></p>
The above is the detailed content of How to determine palindrome string in javascript (example analysis). For more information, please follow other related articles on the PHP Chinese website!
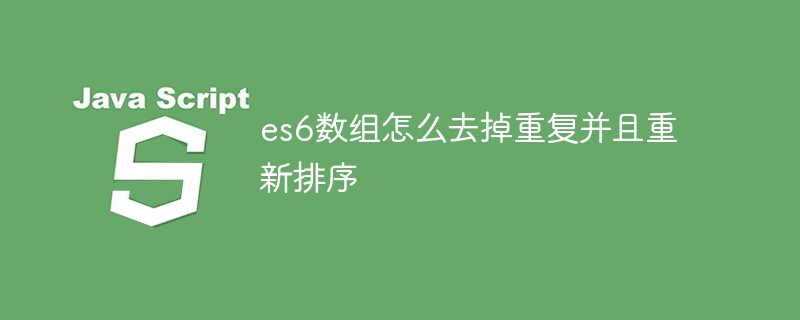
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
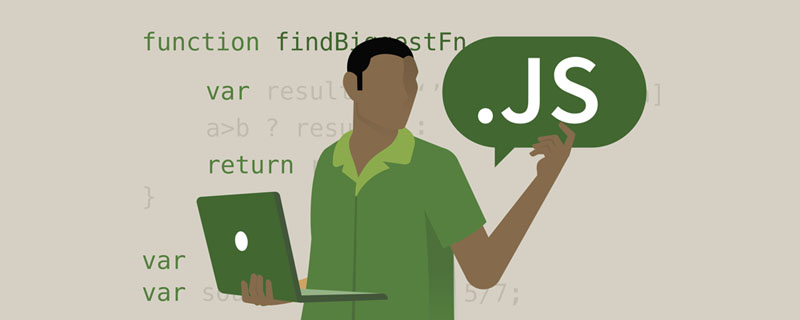
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
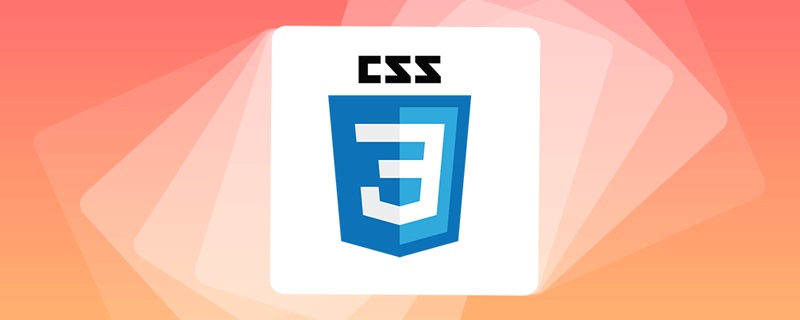
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
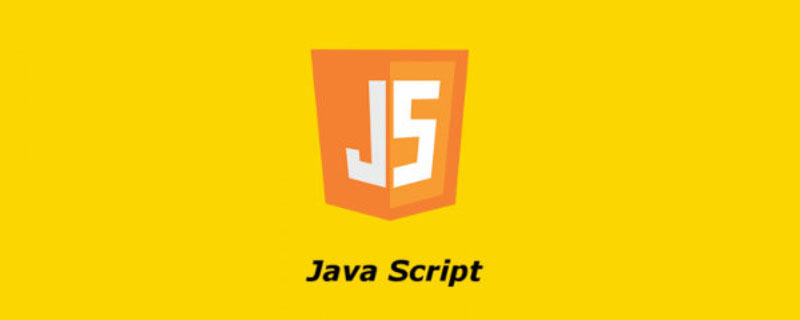
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
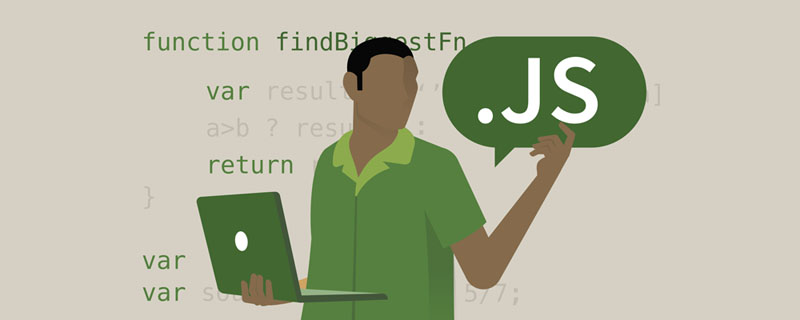
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
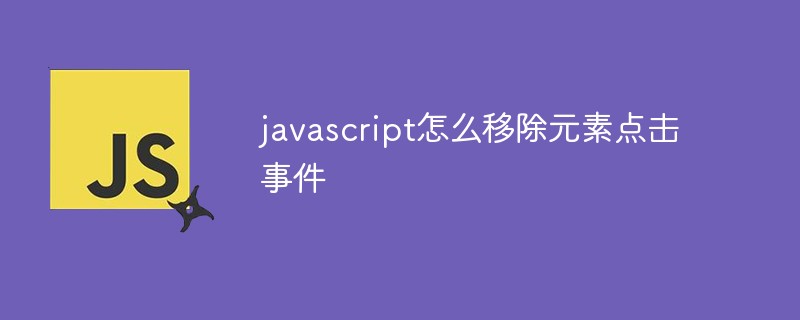
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
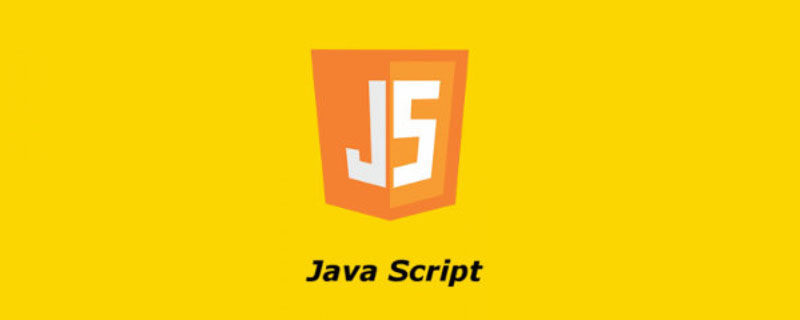
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
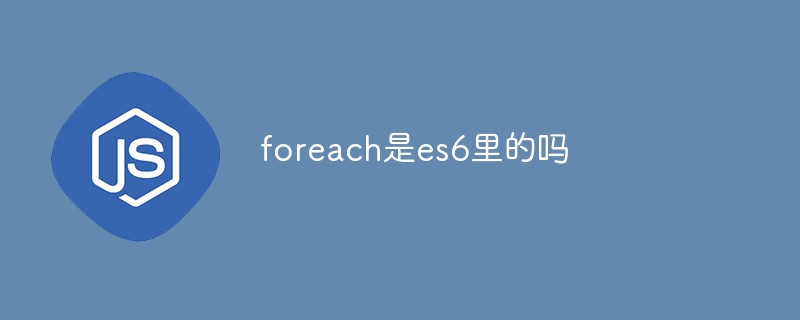
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
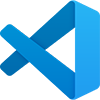
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
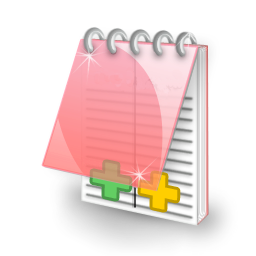
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
