Detailed introduction to the usage of data objects in js (with code)
This article brings you a detailed introduction to the usage of data objects in js (with code). It has certain reference value. Friends in need can refer to it. I hope it will be helpful to you.
Introduction
Handling dates in JavaScript can be complex and often a pain for developers regardless of their skills.
JavaScript provides us with date processing functions through a powerful Date
object.
DATE Object
Date
object instance represents a single point in time.
Although named Date
, it is also used Processing time.
Initialize Date object
We initialize a Date object through the following code:
new Date()
The above code creates a date object that represents the current moment.
Internally, the date represents the number of milliseconds since January 1, 1970 (UTC). This time is important because as far as the computer is concerned, this is when it started.
You may be familiar with UNIX timestamps: this represents the number of seconds that have elapsed since that famous date.
Note that UNIX timestamps are in seconds and JavaScript dates are in milliseconds
If we have a UNIX timestamp, we can initialize a JavaScript date object through the following method:
const timestamp = 1530826365 new Date(timestamp * 1000)
If we pass in 0, we will get the date representing Jan 1st 1970 (UTC).
new Date(0)
If we pass in a string instead of a value, the Date object will use the parse
method to determine which date you pass in, such as:
new Date('2018-07-22') new Date('2018-07') //July 1st 2018, 00:00:00 new Date('2018') //Jan 1st 2018, 00:00:00 new Date('07/22/2018') new Date('2018/07/22') new Date('2018/7/22') new Date('July 22, 2018') new Date('July 22, 2018 07:22:13') new Date('2018-07-22 07:22:13') new Date('2018-07-22T07:22:13') new Date('25 March 2018') new Date('25 Mar 2018') new Date('25 March, 2018') new Date('March 25, 2018') new Date('March 25 2018') new Date('March 2018') //Mar 1st 2018, 00:00:00 new Date('2018 March') //Mar 1st 2018, 00:00:00 new Date('2018 MARCH') //Mar 1st 2018, 00:00:00 new Date('2018 march') //Mar 1st 2018, 00:00:00
It’s very flexible here. You can add or omit leading zeros within months or days.
You need to pay attention to the position of month/day, otherwise the month may be parsed as a date.
Use Date.parse
You can also process strings:
Date.parse('2018-07-22') Date.parse('2018-07') //July 1st 2018, 00:00:00 Date.parse('2018') //Jan 1st 2018, 00:00:00 Date.parse('07/22/2018') Date.parse('2018/07/22') Date.parse('2018/7/22') Date.parse('July 22, 2018') Date.parse('July 22, 2018 07:22:13') Date.parse('2018-07-22 07:22:13') Date.parse('2018-07-22T07:22:13')
Date.parse
will return a timestamp expressed in milliseconds instead of a Date object
You can also pass in values in order to represent each part of the date. The parameter order is as follows: year, month (starting from 0), date, hour, minute, second, millisecond
new Date(2018, 6, 22, 7, 22, 13, 0) new Date(2018, 6, 22)
At least three parameters need to be passed in, but most JavaScript engines can also parse less than three parameters
new Date(2018, 6) //Sun Jul 01 2018 00:00:00 GMT+0200 (Central European Summer Time) new Date(2018) //Thu Jan 01 1970 01:00:02 GMT+0100 (Central European Standard Time)
The final result of the above code is a relative value that depends on your computer's time zone. This means that passing the same parameters may produce different results on different computers.
JavaScript, without any information about the time zone, treats the date as UTC and the result is automatically converted for the current computer time zone.
To summarize, there are four methods that allow you to create a new Date object:
-
If no parameters are passed, a Date object will be created based on the current time;
Pass in a value representing the number of milliseconds in the past since 1 Jan 1970 00:00 GMT;
-
Pass in a string representing the date;
Pass in a series of parameters that represent each item;
Time zone
When initializing the date, you can also pass in the time zone, At this point the date is not assumed to be UTC and is then converted to the local time zone.
The time zone can be passed in HPURS format or by adding a time zone name.
new Date('July 22, 2018 07:22:13 +0700') new Date('July 22, 2018 07:22:13 (CET)')
If an incorrect time zone name is passed in during parsing, JavaScript will use UTC by default and will not report an error.
If a value in the wrong format is passed in, JavaScript will report an Invaild Date
error.
Date conversion and formatting
For a given date object, there are many methods to produce a string based on the date
const date = new Date('July 22, 2018 07:22:13') date.toString() // "Sun Jul 22 2018 07:22:13 GMT+0200 (Central European Summer Time)" date.toTimeString() //"07:22:13 GMT+0200 (Central European Summer Time)" date.toUTCString() //"Sun, 22 Jul 2018 05:22:13 GMT" date.toDateString() //"Sun Jul 22 2018" date.toISOString() //"2018-07-22T05:22:13.000Z" (ISO 8601 format) date.toLocaleString() //"22/07/2018, 07:22:13" date.toLocaleTimeString() //"07:22:13" date.getTime() //1532236933000 date.getTime() //1532236933000
GETTER method of Date object
The Date object provides several methods for checking its value. The results of these methods all depend on the current time zone of the computer
const date = new Date('July 22, 2018 07:22:13') date.getDate() //22 date.getDay() //0 (0 means sunday, 1 means monday..) date.getFullYear() //2018 date.getMonth() //6 (starts from 0) date.getHours() //7 date.getMinutes() //22 date.getSeconds() //13 date.getMilliseconds() //0 (not specified) date.getTime() //1532236933000 date.getTimezoneOffset() //-120 (will vary depending on where you are and when you check - this is CET during the summer). Returns the timezone difference expressed in minutes
The above methods have corresponding versions for obtaining UTC time:
date.getUTCDate() //22 date.getUTCDay() //0 (0 means sunday, 1 means monday..) date.getUTCFullYear() //2018 date.getUTCMonth() //6 (starts from 0) date.getUTCHours() //5 (not 7 like above) date.getUTCMinutes() //22 date.getUTCSeconds() //13 date.getUTCMilliseconds() //0 (not specified)
Editing the Date object
The Date object provides Several editing date value methods
const date = new Date('July 22, 2018 07:22:13') date.setDate(newValue) date.setDay(newValue) date.setFullYear(newValue) //note: avoid setYear(), it's deprecated date.setMonth(newValue) date.setHours(newValue) date.setMinutes(newValue) date.setSeconds(newValue) date.setMilliseconds(newValue) date.setTime(newValue) date.setTimezoneOffset(newValue)
setDay
andsetMonth
all start processing from the value 0, for example, March should be the value 2
Here is one Trivia: These methods "overlap", so for example if you use date.setHours (48)
, the result will affect the day.
There is another piece of trivia: you can pass in multiple parameters to the setHours()
method to set minutes, seconds, and milliseconds, such as setHours(0, 0, 0, 0) Similar situations exist for
, setMinutes
and setSeconds
.
Similar to many methods of getting dates, there are also methods of setting dates for the UTC version:
const date = new Date('July 22, 2018 07:22:13') date.setUTCDate(newalue) date.setUTCDay(newValue) date.setUTCFullYear(newValue) date.setUTCMonth(newValue) date.setUTCHours(newValue) date.setUTCMinutes(newValue) date.setUTCSeconds(newValue) date.setUTCMilliseconds(newValue)
Get the current timestamp
If you want to get the milliseconds The current timestamp in units, it is recommended to use the following method:
Date.now()
instead of
new Date().getTime()
JavaScript always try to get the most accurate results
As mentioned above, you The number of days passed in will affect the total date. This will not report an error and will directly update the month.
new Date(2018, 6, 40) //Thu Aug 09 2018 00:00:00 GMT+0200 (Central European Summer Time)
The above phenomenon also takes effect on dates, hours, minutes, seconds and milliseconds
依据本地情况格式化日期
Internationalization API 在现代浏览器中有很好的支持(除了 UC浏览器),允许你转换日期。
本地化方法通过,通过 Int1
对象暴露,这个对象还可以用来帮助本地化数值,字符串以及货币。
这里我们用到的是 Intl.DateTimeFormat()
我们可以通过下述方法来依据电脑的本地情况格式化一个日期:
const date = new Date('July 22, 2018 07:22:13') new Intl.DateTimeFormat().format(date) //"22/07/2018" in my locale
也可以依据不同的时区格式化日期:
new Intl.DateTimeFormat('en-US').format(date) //"7/22/2018"
Intl.DateTimeFormat
方法还接收一个可选的参数用以自定义输出格式,可以用来展示 小时,分钟和秒
const options = { year: 'numeric', month: 'numeric', day: 'numeric', hour: 'numeric', minute: 'numeric', second: 'numeric' } new Intl.DateTimeFormat('en-US', options).format(date) //"7/22/2018, 7:22:13 AM" new Intl.DateTimeFormat('it-IT', options2).format(date) //"22/7/2018, 07:22:13"
点击这个链接可以查看所有可以用到的属性
两个日期的对比
可以通过 Date.getTime()
获取两个日期之间的差别
const date1 = new Date('July 10, 2018 07:22:13') const date2 = new Date('July 22, 2018 07:22:13') const diff = date2.getTime() - date1.getTime() //difference in milliseconds
同样也可以通过这个方法检测两个日期是否相同:
const date2 = new Date('July 10, 2018 07:22:13') if (date2.getTime() === date1.getTime()) { //dates are equal }
需要注意的是,getTime()
方法比较的是毫秒,所以 July 10, 2018 07:22:13
和 July 10, 2018
并不相等。不过你可以通过 setHours(0, 0, 0, 0)
来重置时间。
相关推荐:
js data日期初始化的5种方法_javascript技巧
javascript-问:php使用post方式提交data,进行js加密,然后显示出来
The above is the detailed content of Detailed introduction to the usage of data objects in js (with code). For more information, please follow other related articles on the PHP Chinese website!
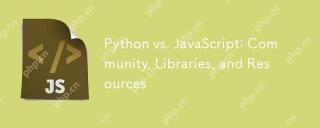
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
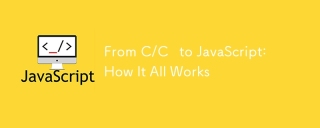
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
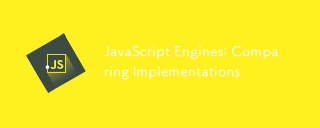
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
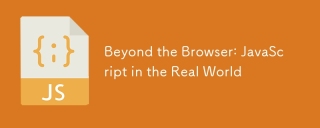
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
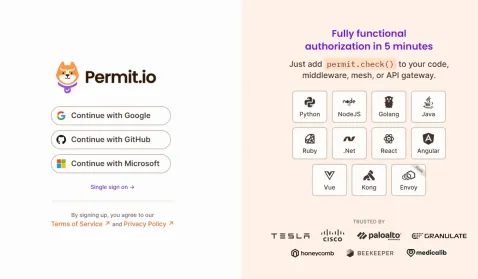
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
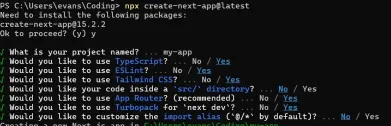
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
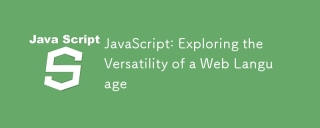
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
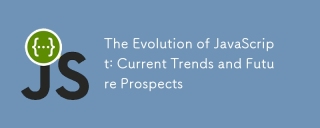
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
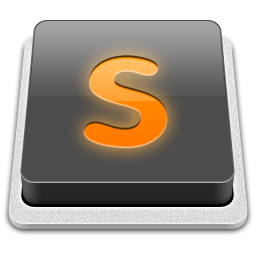
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
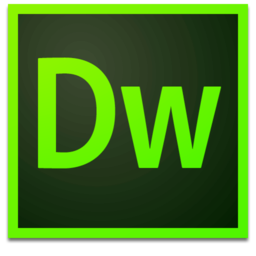
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool