This article mainly introduces a summary of the experience of using axios in node.js, and analyzes the errors encountered in the process. Please refer to it.
Axios is a Promise-based HTTP library that can be used in browsers and node.js. Because of Youda’s recommendation, axios has become more and more popular. I have encountered some problems using axios in recent projects, so I will take this opportunity to summarize them. If there are any mistakes, please feel free to correct them.
Function
The browser initiates an XMLHttpRequests request
The node layer initiates an http request
Supports Promise API
Intercept requests and responses
Convert request and response data
Cancel request
Automatically convert JSON data
The client supports defense against XSRF (cross-site request forgery) )
Compatible
Use
npm
npm install axios
bower
bower install axios
cdn
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
Initiate a request
GET
axios.get('/user?ID=123') .then( res => { console.info(res) }).catch( e => { if(e.response){ //请求已发出,服务器返回状态码不是2xx。 console.info(e.response.data) console.info(e.response.status) console.info(e.response.headers) }else if(e.request){ // 请求已发出,但没有收到响应 // e.request 在浏览器里是一个XMLHttpRequest实例, // 在node中是一个http.ClientRequest实例 console.info(e.request) }else{ //发送请求时异常,捕捉到错误 console.info('error',e.message) } console.info(e.config) }) // 等同以下写法 axios({ url: '/user', method: 'GET', params: { ID: 123 } }).then( res => { console.info(res) }).catch( e=> { console.info(e) })
POST
axios.post('/user', { firstName: 'Mike', lastName: 'Allen' }).then( res => { console.info(res) }).catch( e => { console.info(e) }) // 等同以下写法 axios({ url: '/user', method: 'POST', data: { firstName: 'Mike', lastName: 'Allen' } }).then( res => { console.info(res) }).catch( e => { console.info(e) })
Notes
Params are used when passing parameters using the GET method, and the official documentation introduces: params are the URL parameters to be sent with the request. Must be a plain object or a URLSearchParams object. Translated as: params is sent as a parameter in the URL link in the request, and it must be a plain object or a URLSearchParams object. Plain object refers to an ordinary object defined in JSON form or a simple object created by new Object(); while the URLSearchParams object refers to an object that can be used to process the query string of the URL using some practical methods defined by the URLSearchParams interface. , that is to say, the params parameters are passed in the form of /user?ID=1&name=mike&sex=male.
When using POST, the corresponding parameter transfer uses data, and data is sent as the request body. This form is also used for PUT, PATCH and other request methods. One thing to note is that the default request body type of POST in axios is Content-Type: application/json (JSON specification is popular), which is also the most common request body type, which means that the serialized json format is used String to pass parameters, such as: { "name" : "mike", "sex" : "male" }; at the same time, the background must receive parameters in the form of supporting @RequestBody, otherwise the front-end parameters will be passed correctly and the background will receive situation.
If you want to set the type to Content-Type:application/x-www-form-urlencoded (browser native support), axios provides two methods, as follows:
Browser side
const params = new URLSearchParams(); params.append('param1', 'value1'); params.append('param2', 'value2'); axios.post('/user', params);
However, not all browsers support URLSearchParams. Check caniuse.com for compatibility, but there is a Polyfill (polyfill: used to implement native APIs that the browser does not support) The code can be vaguely understood as a patch, and at the same time, ensure that the polyfill is in the global environment).
Alternatively, you can also use the qs library to format the data. By default, the qs library can be used after installing axios.
const qs = require('qs'); axios.post('/user', qs.stringify({'name':'mike'}));
node layer
querystring can be used in the node environment. Similarly, you can also use qs to format data.
const querystring = require('querystring'); axios.post('http://something.com/', querystring.stringify({'name':'mike'}));
Supplement
There is another common request body type, namely multipart/form-data (native browser support), which is commonly used to submit form data a format. Contrast this with x-www-form-urlencoded, where data is encoded into key-value pairs separated by '&', while keys and values are separated by '='. Characters that are not alphabetic or numeric are percent-encoded (URL encoding), which is why this type does not support binary data (multipart/form-data should be used instead).
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
How to implement the China map in vue vuex axios echarts
Solve the problem of the input box being blocked by the input method
How to solve the problem that the soft keyboard blocks the input box in js
How to implement component interaction in Angular2
The above is the detailed content of How to use axios in node.js. For more information, please follow other related articles on the PHP Chinese website!
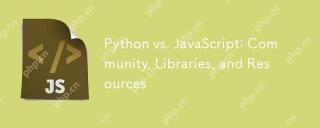
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
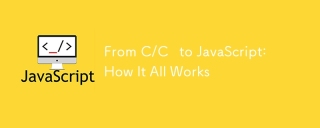
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
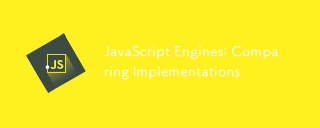
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
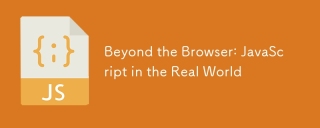
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
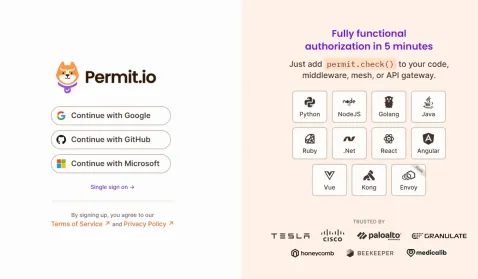
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
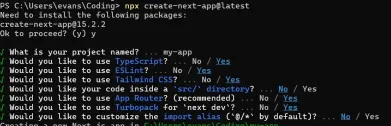
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
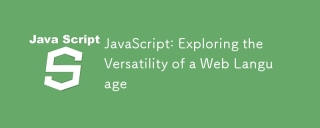
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
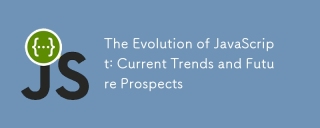
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
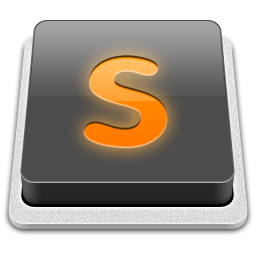
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
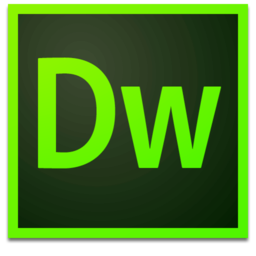
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool