This article mainly introduces the two-way binding principle (data binding mechanism) of AngularJs. The editor thinks it is quite good, so I will share it with you now and give it as a reference. Let’s follow the editor to take a look.
So what is two-way binding? Let’s briefly explain it below.
First we need to understand data binding. The website pages we see are composed of two parts: data and design. Converting the design into a language that the browser can understand is what HTML and CSS mainly do. Displaying data on the page and having certain interactive effects (such as clicks and other user operations and corresponding page reactions) is the main job of js. Many times we cannot refresh the page (get request) every time we update data. Instead, we request relevant data from the backend and update the page (post request) by loading without refreshing. Then after the data is updated, the corresponding location on the page can automatically make corresponding modifications, which is data binding.
In the previous development model, this step usually uses jq to operate the DOM structure to update the page. But this brings a lot of code and a lot of operations. If we can determine the operations that need to be performed on the page from the data obtained from the backend at the beginning, when the data changes, the relevant content of the page will also automatically change, which will greatly facilitate the development of front-end engineers. In the new framework (angualr, react, vue, etc.), by monitoring the data, if changes are found, the page will be modified according to the rules that have been written, thus realizing data binding. It can be seen that data binding is a modification of M (model, data) to V (view) through VM (model-view, the transformation rule between data and pages).
The two-way binding adds a reverse path. When the user operates the page (such as entering a value in Input), the data can change in time, and according to the change in the data, corresponding modifications are made in another part of the page. A common example is the shopping cart in Taobao. When the quantity of goods changes, the price of the goods can also change in time. This achieves a two-way binding of V-M-VM-V.
AngularJs sets a listening queue on the scope model to listen for data changes and update the view. Every time you bind something to view (html), AngularJs will insert a $watch into the $watch queue to detect whether there are changes in the model it monitors. The $digest loop fires when the browser receives an event that can be handled by the angular context. $digest will iterate through all $watches. Thus updating the DOM.
$watch
This is somewhat similar to our observer pattern. Under the current scope $scope, we create a monitor $watchers and a listener$ watch, $watchers are responsible for managing all $watches. Every time we bind it to the UI, we will automatically create a $watch and put it in $watchers.
controller.js
app.controller('MainCtrl', function($scope) { $scope.Hello = "Hello"; $scope.world = "World"; });
index.html
<p>{{Hello}}</p>
Here, even if we add two variables to $scope, but there is only one It is bound to the UI, so only one $watch
$digest
can be processed by the angular context when the browser receives it. event, the $digest loop will trigger. $digest will traverse our $watches. If $watch has no changes, the loop detection will stop. If at least one has been updated, the loop will be triggered again until all $watches have no changes. This ensures that each model will not change again. This is the Dirty Checking mechanism
controller.js
app.controller('MainCtrl', function() { $scope.name = "Foo"; $scope.changeFoo = function() { $scope.name = "Bar"; } });
index.js
<p>{{ name }}</p> <button ng-click="changeFoo()">Change the name</button>
When we press the button
The browser receives an event and enters the angular context.
The $digest loop begins to execute, querying whether each $watch changes.
Because the $watch monitoring $scope.name reports a change, it will force another $digest cycle.
New $digest loop no changes detected.
Update the DOM corresponding to the new value of $scope.name.
$apply
$apply We can directly understand it as refreshing the UI. If you call $apply when the event is triggered, it will enter the angular context. If it is not called, it will not enter, and the subsequent $digest detection mechanism will not trigger
app.directive('clickable', function() { return { restrict: "E", scope: { foo: '=' }, template: '<ul style="background-color: lightblue"><li>{{foo}}</li></ul>', link: function(scope, element, attrs) { element.bind('click', function() { scope.foo++; console.log(scope.foo); }); } } });
When we call the clickable instruction, we can see that the value of foo has increased, but the content displayed on the interface has not changed. The $digest dirty detection mechanism is not triggered, and the $watch to detect foo is not executed.
Two forms of $apply() method
1) No parameters
$scope.$apply();
element.bind('click', function() { scope.foo++; //if error scope.$apply(); });
When we use this form, if the program occurs before scope.$apply Exception, then scope.$apply is not executed and the interface will not be updated
2) If there are parameters
$scope.$apply(function(){ ... })
element.bind('click', function() { scope.$apply(function() { scope.foo++; }); })
If you use this form, even if an exception occurs later, the data will still be updated.
Using $watch in AngularJS
Common usage:
$scope.name = 'Hello'; $scope.$watch('name', function(newValue, oldValue) { if (newValue === oldValue) { return; } $scope.updated++; });
传入到$watch()中的第二个参数是一个回调函数,该函数在name的值发生变化的时候会被调用。
如果要监听的是一个对象,那还需要第三个参数:
$scope.data.name = 'Hello'; $scope.$watch('data', function(newValue, oldValue) { if (newValue === oldValue) { return; } $scope.updated++; }, true);
表示比较的是对象的值而不是引用,如果不加第三个参数true,在 data.name 变化时,不会触发相应操作,因为引用的是同一引用。
总结
1) 只有在$scope变量绑定到页面上,才会创建 $watch
2) $apply决定事件是否可以进入angular context
3) $digest 循环检查model时最少两次,最多10次(多于10次抛出异常,防止无限检查)
4) AngularJs自带的指令已经实现了$apply,所以不需要我们额外的编写
5) 在自定义指令时,建议使用带function参数的$apply
上面是我整理给大家的,希望今后会对大家有帮助。
相关文章:
在javaScript中如何使用手机号码校验工具类PhoneUtils
The above is the detailed content of How to use two-way binding in AngularJs. For more information, please follow other related articles on the PHP Chinese website!
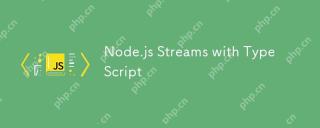
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
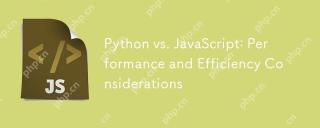
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
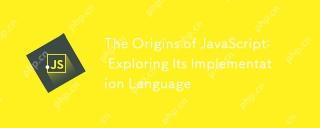
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
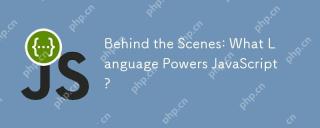
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
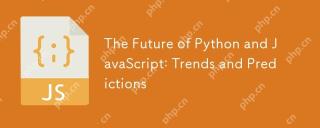
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
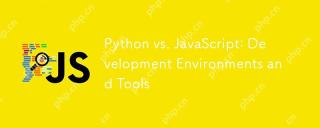
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
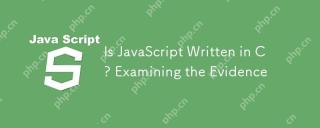
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
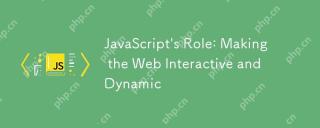
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
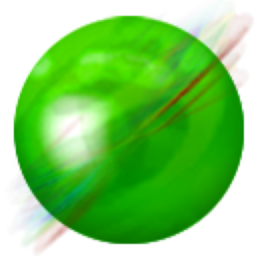
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
