Difficulties in JavaScript array operations (detailed tutorial)
This article explains the difficulties of JavaScript array operations and what needs to be paid attention to by giving examples of code analysis. Let's study and refer to it together.
The following content is the experience summarized when learning JavaScript arrays and the points that need to be paid attention to.
Don’t use for_in to traverse arrays
This is a common misunderstanding among JavaScript beginners. for_in is used to traverse all enumerable (enumerable) keys in the object including the prototype chain. It does not originally exist for traversing arrays.
There are three problems with using for_in to traverse arrays:
1. The traversal order is not fixed
The JavaScript engine does not guarantee the traversal order of objects. When traversing an array as a normal object, the index order of the traversal is also not guaranteed.
2. The values on the object prototype chain will be traversed.
If you change the prototype object of the array (such as polyfill) without setting it to enumerable: false
, for_in will iterate over these things.
3. Low operating efficiency.
Although theoretically JavaScript uses the form of objects to store arrays, the JavaScript engine is particularly optimized for arrays, a very commonly used built-in object. https://jsperf.com/for-in-vs-...
You can see that using for_in to traverse an array is more than 50 times slower than using subscripts to traverse an array
PS: You may want to Find for_of
Don’t use JSON.parse(JSON.stringify()) to deep copy arrays
Some people use JSON to deep copy objects or arrays. Although this is a simple and convenient method in most cases, it may also cause unknown bugs because: some specific values will be converted to null
NaN, undefined, Infinity for JSON that is not These supported values will be converted to null when serializing JSON. After deserialization, they will naturally be null
Key-value pairs with undefined values will be lost
When serializing JSON Keys with undefined values will be ignored and will naturally be lost after deserialization.
Will convert the Date object into a string
JSON does not support object types. For Date objects in JS The processing method is to convert it into a string in ISO8601 format. However, deserialization does not convert the time format string into a Date object
The operation efficiency is low.
As native functions, JSON.stringify
and JSON.parse
operate on JSON strings very quickly. However, it is completely unnecessary to serialize the object to JSON and deserialize it back in order to deep copy the array.
I spent some time writing a simple function for deep copying arrays or objects. The test found that the running speed is almost 6 times that of using JSON transfer. By the way, it also supports the copying of TypedArray and RegExp objects
https://jsperf.com/deep-clone...
Don’t use arr.find instead of arr.some
Array.prototype.find
is a new array search function in ES2015, which is similar to Array.prototype.some
, but cannot replace the latter.
Array.prototype.find
Returns the first qualified value, directly use this value to do if
to determine whether it exists. If this qualified value happens to be 0 What to do?
arr.find
is to find the value in the array and then further process it. It is generally used in the case of object array; arr.some
is to check the existence; The two cannot be mixed.
Don’t use arr.map instead of arr.forEach
is also a mistake that JavaScript beginners often make. They often don’t distinguish between Array.prototype.map
and ## The actual meaning of #Array.prototype.forEach.
map is called
MAP in Chinese. It derives another new sequence by executing a certain function on a certain sequence in sequence. This function usually has no side effects and does not modify the original array (so-called pure function).
forEach There are not so many explanations. It simply processes all items in the array with a certain function. Since
forEach has no return value (returns undefined), its callback function usually contains side effects, otherwise this
forEach is meaningless.
map is more powerful than
forEach, but
map will create a new array and occupy memory. If you don't use the return value of
map, then you should use
forEach
Supplement: experience supplement
ES6 previous , there are two main methods for traversing an array: handwritten loop iteration using subscripts, and usingArray.prototype.forEach. The former is versatile and the most efficient, but it is more cumbersome to write - it cannot directly obtain the values in the array.
forEach accepts a callback function, you can return
in advance, which is equivalent to continue
in a handwritten loop. But you can't break
- because there is no loop in the callback function for you to break
:
[1, 2, 3, 4, 5].forEach(x => { console.log(x); if (x === 3) { break; // SyntaxError: Illegal break statement } });
There are still solutions. Other functional programming languages such as scala
have encountered similar problems. They provide a function
break, which throws an exception.
We can follow this approach to achieve the break
of arr.forEach
:
try { [1, 2, 3, 4, 5].forEach(x => { console.log(x); if (x === 3) { throw 'break'; } }); } catch (e) { if (e !== 'break') throw e; // 不要勿吞异常。。。 }
still There are other ways, such as using Array.prototype.some
instead of Array.prototype.forEach
.
Consider the characteristics of Array.prototype.some. When some
finds a value that meets the conditions (the callback function returns true
), the loop will be terminated immediately, using this Features can simulate break
: The return value of
[1, 2, 3, 4, 5].some(x => { console.log(x); if (x === 3) { return true; // break } // return undefined; 相当于 false });
some
is ignored, and it has been separated from the judgment of whether there are elements in the array that meet the given conditions. original meaning.
Before ES6, I mainly used this method (in fact, due to the expansion of Babel code, I also use it occasionally now). ES6 is different. We have for...of. for...of
is a real loop and can break
:
for (const x of [1, 2, 3, 4, 5]) { console.log(x); if (x === 3) { break; } }
But there is a problem, for...of
seems to take Less than the subscript of the loop. In fact, the JavaScript language developers thought of this problem and can solve it as follows:
for (const [index, value] of [1, 2, 3, 4, 5].entries()) { console.log(`arr[${index}] = ${value}`); }
Array.prototype.entries
##for...of and
forEach Performance test: https://jsperf.com/array-fore...
for...of is faster in Chrome
The above is the detailed content of Difficulties in JavaScript array operations (detailed tutorial). For more information, please follow other related articles on the PHP Chinese website!
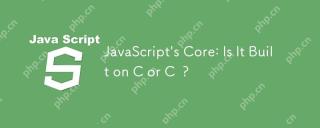
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
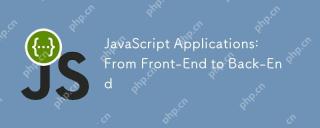
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
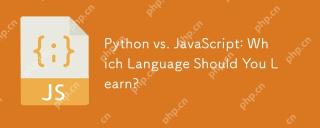
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
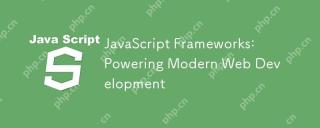
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
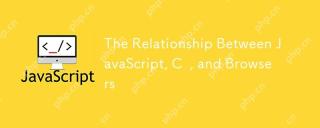
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
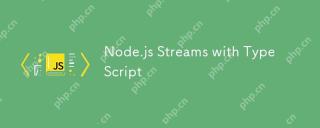
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
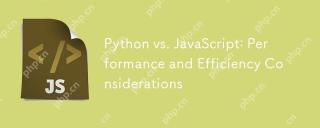
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
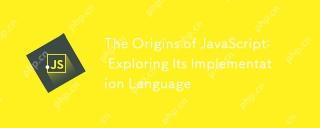
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
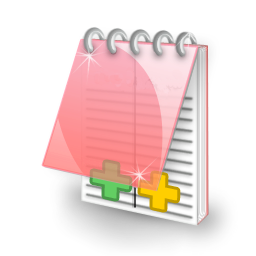
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
