JavaScript Object
Javascript objects include: JS Array (array object), JS Boolean (Boolean object), JS Date (date object), JS Math (Math object), JS Number (numeric object), JS String (string object), JS RegExp (regular expression), JS Function (function object), Window and Screen in Browser, etc.
JS Array object
The Array object is used to store multiple values in a single variable.
Method | Description |
---|---|
Concatenates two or more arrays and returns the result. | |
Put all elements of the array into a string. Elements are separated by the specified delimiter. | |
Deletes and returns the last element of the array | |
Adds one or more elements to the end of an array and returns the new length. | |
Reverse the order of elements in the array. | |
Delete and return the first element of the array | |
Return selected elements from an existing array | |
Sort the elements of the array | |
Returns the source code of the object. | |
Convert the array to a string and return the result. | |
Convert the array to a local array and return the result. | |
Adds one or more elements to the beginning of the array and returns the new length. | |
Return the original value of the array object |
<script type="text/javascript">var a = [1,2,3]; document.write(a.concat(4,5));</script>Output:
1,2,3,4,5Join() creates an array and puts all its elements into a string:
<script type="text/javascript">var arr = new Array(3) arr[0] = "George"arr[1] = "John"arr[2] = "Thomas"document.write(arr.join())</script>Output:
George,John,Thomassort() will create an array to sort
<script>var x=[4,5,7,1,6,9,3,10,132,12]; console.log(x.sort()); console.log(x.sort(function(a,b){ return a-b; })); console.log(x.sort(function(a,b){ return b-a; })) ; console.log(x);</script>Output:
[1, 10, 12, 132, 3, 4, 5, 6, 7, 9] [1, 3, 4, 5, 6, 7, 9, 10, 12, 132] [132, 12, 10, 9, 7, 6, 5, 4, 3, 1] [132, 12, 10, 9, 7, 6, 5, 4, 3, 1]pop() creates an array and then deletes the array the last element of . Note that this will also change the length of the array:
<script type="text/javascript">var arr = new Array(3) arr[0] = "George"arr[1] = "John"arr[2] = "Thomas"document.write(arr) document.write("<br />") document.write(arr.pop()) document.write("<br />") document.write(arr)</script>Output:
George,John,Thomas Thomas George,Johnpush() method adds one or more elements to the end of the array and returns the new length.
<script type="text/javascript">var arr = new Array(3) arr[0] = "George"arr[1] = "John"arr[2] = "Thomas"document.write(arr + "<br />") document.write(arr.push("James") + "<br />") document.write(arr)</script>Output:
George,John,Thomas 4 George,John,Thomas,JamesThis article explains the local objects of JavaScript. For more related content, please pay attention to the php Chinese website. Related recommendations:
Introducing a small case for getting started with JS
Achieving dynamic display of processes through js
particlesJS usage introduction related content
The above is the detailed content of JavaScript local objects. For more information, please follow other related articles on the PHP Chinese website!
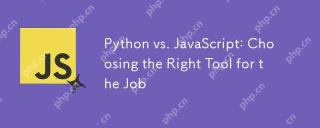
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
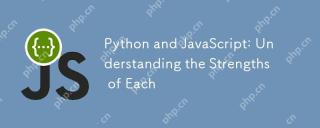
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
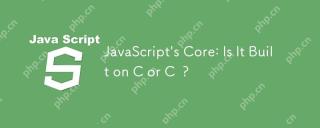
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
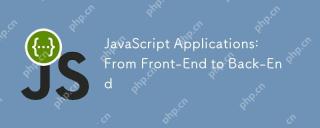
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
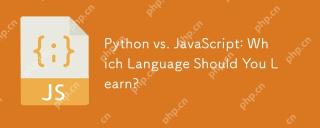
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
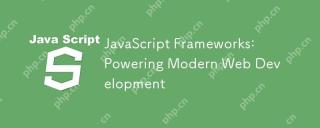
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
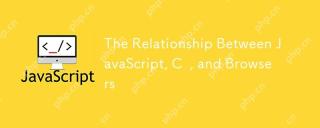
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
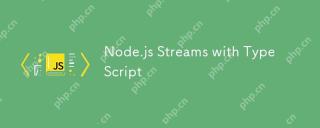
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
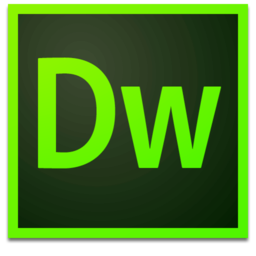
Dreamweaver Mac version
Visual web development tools
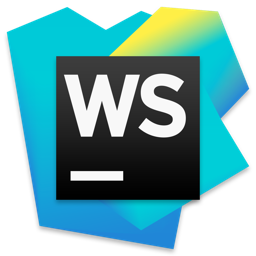
WebStorm Mac version
Useful JavaScript development tools
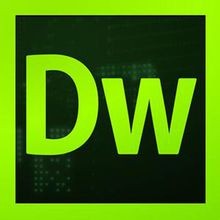
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
