How to implement multipart form file upload using Node layer
Below I will share with you an example of file upload using Node layer simulation to implement multipart forms. It has a good reference value and I hope it will be helpful to everyone.
Sometimes there is such a demand. Nodejs is used as a webserver to upload files from the browser to the back-end server. The Node layer only does a data transfer. If in this process, the Node webserver needs to properly process the data. Processing, and then Posting to the backend, then you have to simulate file upload at the Node layer.
First, upload the file through the browser. The PostData format looks like this:
Screenshot 2014 -11-22 PM 9.18.45.png
As shown in the picture, each set of data is actually separated by "-----WebkitFormBoundary....", and finally used This delimiter ends, and this delimiter is completely customizable.
Each piece of submitted data is described by Content-Disposition. If Content-Type is not specified, the default is text/plain. If it is an uploaded binary file, just specify its mime-type.
Simply encapsulate a method to implement file upload at the Node layer:
/** * 上传文件 * @param files 经过formidable处理过的文件 * @param req httpRequest对象 * @param postData 额外提交的数据 */ function uploadFile(files, req, postData) { var boundaryKey = Math.random().toString(16); var endData = '\r\n----' + boundaryKey + '--'; var filesLength = 0, content; // 初始数据,把post过来的数据都携带上去 content = (function (obj) { var rslt = []; Object.keys(obj).forEach(function (key) { arr = ['\r\n----' + boundaryKey + '\r\n']; arr.push('Content-Disposition: form-data; name="' + key + '"\r\n\r\n'); arr.push(obj[key]); rslt.push(arr.join('')); }); return rslt.join(''); })(postData); // 组装数据 Object.keys(files).forEach(function (key) { if (!files.hasOwnProperty(key)) { delete files.key; return; } content += '\r\n----' + boundaryKey + '\r\n' + 'Content-Type: application/octet-stream\r\n' + 'Content-Disposition: form-data; name="' + key + '"; ' + 'filename="' + files[key].name + '"; \r\n' + 'Content-Transfer-Encoding: binary\r\n\r\n'; files[key].contentBinary = new Buffer(content, 'utf-8'); filesLength += files[key].contentBinary.length + fs.statSync(files[key].path).size; }); req.setHeader('Content-Type', 'multipart/form-data; boundary=--' + boundaryKey); req.setHeader('Content-Length', filesLength + Buffer.byteLength(endData)); // 执行上传 var allFiles = Object.keys(files); var fileNum = allFiles.length; var uploadedCount = 0; allFiles.forEach(function (key) { req.write(files[key].contentBinary); var fileStream = fs.createReadStream(files[key].path, {bufferSize: 4 * 1024}); fileStream.on('end', function () { // 上传成功一个文件之后,把临时文件删了 fs.unlink(files[key].path); uploadedCount++; if (uploadedCount == fileNum) { // 如果已经是最后一个文件,那就正常结束 req.end(endData); } }); fileStream.pipe(req, {end: false}); }); }
The idea is like this, the code is not complicated, and you may need to pay extra attention The thing is, in the response processing of http.request, the response.headers may be gzip. At this time, the buffer cannot be directly toString. It needs to be decoded by zlib and then converted to string. The general idea is:
var result = []; response.on('data', function (chunk) { result.push(chunk); }); // 处理response var _dealResponse = function (data) { var buffer = data; try { data = data.toString('utf8'); data = data ? (JSON.parse(data) || data) : false; } catch (err) { // 接口返回数据格式异常,解析失败 console.log(err); } self.res.writeHead(response.statusCode, 'OK', { 'content-type': 'text/plain; charset=utf-8', 'content-length': buffer.length }); self.res.write(buffer); self.res.end(); }; response.on('end', function () { result = Buffer.concat(result); // gzip 的数据,需要zlib解码 if (response.headers['content-encoding'] == 'gzip') { zlib.gunzip(result, function (err, dezipped) { var data = err ? new Buffer('{}') : dezipped; _dealResponse(data); }); } else { _dealResponse(result); } });
Mark it, maybe You just need it when you pass by~~~
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
Gzip compression issues in HTTP
##How to write a Snake game using JS code (detailed tutorial)
How to convert path to base64 encoding in Javascript
The above is the detailed content of How to implement multipart form file upload using Node layer. For more information, please follow other related articles on the PHP Chinese website!
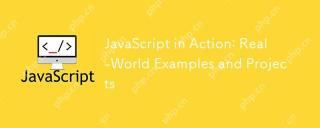
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
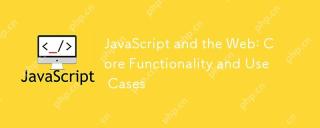
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
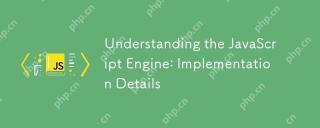
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
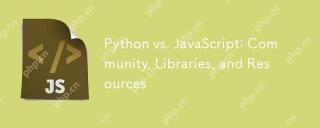
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
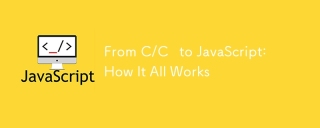
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
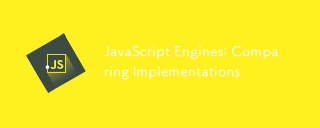
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
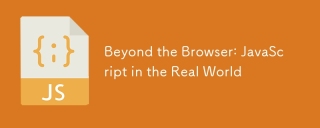
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor
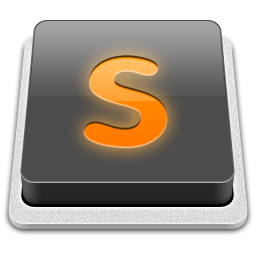
SublimeText3 Mac version
God-level code editing software (SublimeText3)
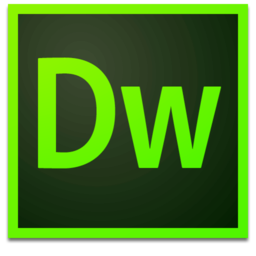
Dreamweaver Mac version
Visual web development tools
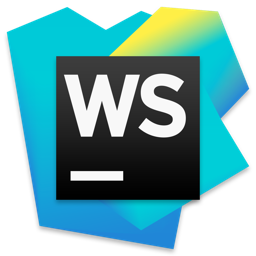
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment