This article mainly introduces the introduction to React high-order components. In this article, we introduce in detail what high-order components are and how to use high-order components. Now we share them with you and give them a reference.
Definition of higher-order components
HoC does not belong to React’s API. It is an implementation pattern, which is essentially a function that accepts one or more React components. As a parameter, return a brand new React component instead of modifying the existing component. Such components are called higher-order components. During the development process, when some functions need to be reused in multiple component classes, a Hoc can be created.
Basic usage
Wrapping method
const HoC = (WrappendComponent) => { const WrappingComponent = (props) => ( <p className="container"> <WrappendComponent {...props} /> </p> ); return WrappingComponent; };
In the above code, WrappendComponent is accepted as a parameter. This parameter is what will be HoC For ordinary wrapped components, wrap a p in render and give it the className attribute. The resulting WrappingComponent and the passed-in WrappendComponent are two completely different components.
In WrappingComponent, you can read, add, edit, and delete the props passed to WrappendComponent, and you can also wrap WrappendComponent with other elements to implement encapsulation styles, add layout, or other operations.
Composition method
const HoC = (WrappedComponent, LoginView) => { const WrappingComponent = () => { const {user} = this.props; if (user) { return <WrappedComponent {...this.props} /> } else { return <LoginView {...this.props} /> } }; return WrappingComponent; };
There are two components in the above code, WrappedComponent and LoginView. If user exists in the incoming props, the WrappedComponent component will be displayed normally, otherwise Display the LoginView component to allow the user to log in. The parameters passed by HoC can be multiple. Pass multiple components to customize the behavior of the new component. For example, the main page is displayed when the user is logged in, and the login interface is displayed when the user is not logged in. When rendering the list, pass in the List and Loading components to add the new component. Loading behavior.
Inheritance method
const HoC = (WrappendComponent) => { class WrappingComponent extends WrappendComponent { render() ( const {user, ...otherProps} = this.props; this.props = otherProps; return super.render(); } } return WrappingComponent; };
WrappingComponent is a new component that inherits from WrappendComponent and shares the functions and properties of the parent. You can use super.render() or super.componentWillUpdate() to call the parent's life cycle function, but this will couple the two components together and reduce the reusability of the component.
The packaging of components in React is based on the idea of the smallest available unit. Ideally, a component only does one thing, which is in line with the single responsibility principle in OOP. If you need to enhance the functionality of a component, enhance the component by combining it or adding code instead of modifying the original code.
Note
Do not use higher-order components in the render function
render() { // 每一次render函数调用都会创建一个新的EnhancedComponent实例 // EnhancedComponent1 !== EnhancedComponent2 const EnhancedComponent = enhance(MyComponent); // 每一次都会使子对象树完全被卸载或移除 return <EnhancedComponent />; }
The diff algorithm in React will compare the old and new sub-object trees to determine whether Update the existing sub-object tree or drop the existing sub-tree and remount.
Static methods must be copied
// 定义静态方法 WrappedComponent.staticMethod = function() {/*...*/} // 使用高阶组件 const EnhancedComponent = enhance(WrappedComponent); // 增强型组件没有静态方法 typeof EnhancedComponent.staticMethod === 'undefined' // true
The Refs attribute cannot be passed
The ref specified in HoC will not be passed to the sub-component. It is required Passed using props through the callback function.
The above is what I compiled for everyone. I hope it will be helpful to everyone in the future.
Related articles:
How to implement a circular Nodelist Dom list using JS
How to implement text color change after clicking in Vue
How to set the background color for a separate page in Vue-cli
Refresh and tab switching in vue
The above is the detailed content of Detailed introduction to higher-order components in React. For more information, please follow other related articles on the PHP Chinese website!
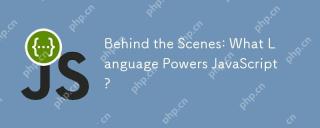
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
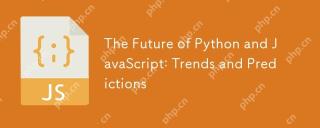
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
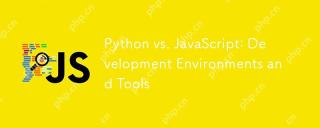
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
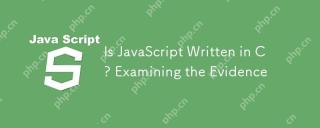
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
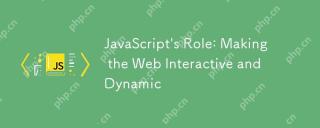
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
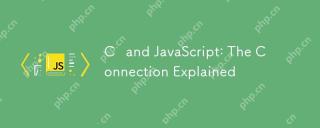
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
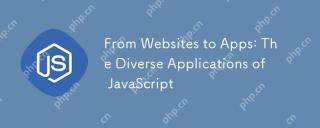
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
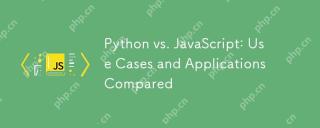
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Atom editor mac version download
The most popular open source editor
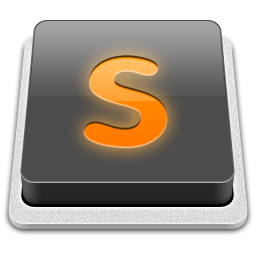
SublimeText3 Mac version
God-level code editing software (SublimeText3)
