This time I will show you how to make a d.ts file and what are the precautions for making a d.ts file. The following is a practical case, let's take a look.
Preface
This article mainly talks about how to write a typescript description file (a file name ending with d.ts, such as xxx.d. ts).
I recently started switching from js to ts. However, some description files (d.ts) are required. Commonly used ones such as jquery can be downloaded through npm to npm install @types/jquery that has been written by others. However, there are still some niche or internal public libraries within the company or public js codes that have been written before that require manual writing of description files.
I also found some information from the Internet before, but it was still unclear. After a period of exploration, I recorded the results of the exploration, and I hope it can give others a reference.
If you only write js, d.ts is also useful for you. Most editors can recognize d.ts files and give you smart prompts when you write js code. The effect looks like this:
For details, you can read some articles I have written before: http://www.jb51.net/article/138211.htm
Normally, when we write js, there are two ways to introduce js:
1. Globally introduce global variables in the html file through the <script> tag. </script>
2, require other js files through the module loader: for example, var j=require('jquery').
Global type
First give an example of the first way.
Variable
For example, if there is a global variable now, then the corresponding d.ts file writes like this.
declare var aaa:number
The keyword declare means declaration. The global variable is aaa, and the type is numeric type (number). Of course, it can also be a string type or something else:
declare var aaa:number|string //注意这里用的是一个竖线表示"或"的意思
If it is a constant, use the keyword const to express it:
declare const max:200
Function
From the above From the way of writing global variables, we can naturally infer that the way of writing a global function is as follows:
/** id是用户的id,可以是number或者string */ decalre function getName(id:number|string):string
The last string represents the type of the return value of the function. If the function does not return a value, it can be expressed as void.
When called in js, it will prompt:
The comments we wrote above can also prompt when writing js.
Sometimes the same function has several ways of writing:
get(1234) get("zhangsan",18)
Then the corresponding way of writing d.ts:
declare function get(id: string | number): string declare function get(name:string,age:number): string
If there are some parameters It is optional. You can add a ? to indicate that it is not necessary.
declare function render(callback?:()=>void): string
When calling in js, the callback can be passed or not:
render() render(function () { alert('finish.') })
class
Of course, in addition to variables and functions, we also have class.
declare class Person { static maxAge: number //静态变量 static getMaxAge(): number //静态方法 constructor(name: string, age: number) //构造函数 getName(id: number): string }
constructor represents the construction method:
where static means static and is used to represent static variables And static methods:
Object
declare namespace OOO{ }
Of course, this object may have variables, functions, or kind.
declare namespace OOO{ var aaa: number | string function getName(id: number | string): string class Person { static maxAge: number //静态变量 static getMaxAge(): number //静态方法 constructor(name: string, age: number) //构造函数 getName(id: number): string //实例方法 } }
In fact, put the above writing methods into the curly brackets enclosed by this namespace. Note that the declare keyword is not needed inside the brackets.
Effect:
对象里面套对象也是可以的:
declare namespace OOO{ var aaa: number | string // ... namespace O2{ let b:number } }
效果:
混合类型
有时候有些值既是函数又是class又是对象的复杂对象。比如我们常用的jquery有各种用法:
new $() $.ajax() $()
既是函数又是对象
declare function $2(s:string): void declare namespace $2{ let aaa:number }
效果:
作为函数用:
作为对象用:
也就是ts会自动把同名的namespace和function合并到一起。
既是函数,又是类(可以new出来)
// 实例方法 interface People{ name: string age: number getName(): string getAge():number } interface People_Static{ new (name: string, age: number): People /** 静态方法 */ staticA():number (w:number):number } declare var People:People_Static
效果:
作为函数使用:
类的静态方法:
类的构造函数:
类的实例方法:
模块化
除了上面的全局的方式,我们有时候还是通过require的方式引入模块化的代码。
比如这样的效果:
对应的写法是这样的:
declare module "abcde" { export let a: number export function b(): number export namespace c{ let cd: string } }
其实就是外面套了一层 module "xxx",里面的写法和之前其实差不多,把declare换成了export。
此外,有时候我们导出去的是一个函数本身,比如这样的:
对应的写法很简单,长这个样子:
declare module "app" { function aaa(some:number):number export=aaa }
以此类推,导出一个变量或常量的话这么写:
declare module "ccc" { const c:400 export=c }
效果:
UMD
有一种代码,既可以通过全局变量访问到,也可以通过require的方式访问到。比如我们最常见的jquery:
其实就是按照全局的方式写d.ts,写完后在最后加上declare namespace "xxx"的描述:
declare namespace UUU{ let a:number } declare module "UUU" { export =UUU }
效果这样:
作为全局变量使用:
作为模块加载使用:
其他
有时候我们扩展了一些内置对象。比如我们给Date增加了一个format的实例方法:
对应的d.ts描述文件这样写:
interface Date { format(f: string): string }
相信看了本文案例你已经掌握了方法,更多精彩请关注php中文网其它相关文章!
推荐阅读:
The above is the detailed content of How to make d.ts file. For more information, please follow other related articles on the PHP Chinese website!
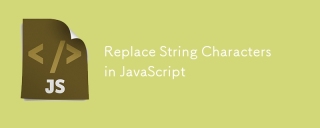
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
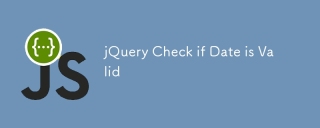
Simple JavaScript functions are used to check if a date is valid. function isValidDate(s) { var bits = s.split('/'); var d = new Date(bits[2] '/' bits[1] '/' bits[0]); return !!(d && (d.getMonth() 1) == bits[1] && d.getDate() == Number(bits[0])); } //test var
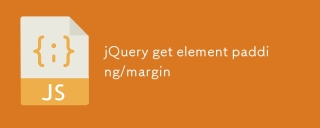
This article discusses how to use jQuery to obtain and set the inner margin and margin values of DOM elements, especially the specific locations of the outer margin and inner margins of the element. While it is possible to set the inner and outer margins of an element using CSS, getting accurate values can be tricky. // set up $("div.header").css("margin","10px"); $("div.header").css("padding","10px"); You might think this code is
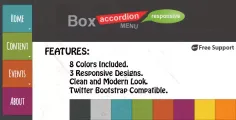
This article explores ten exceptional jQuery tabs and accordions. The key difference between tabs and accordions lies in how their content panels are displayed and hidden. Let's delve into these ten examples. Related articles: 10 jQuery Tab Plugins
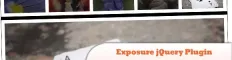
Discover ten exceptional jQuery plugins to elevate your website's dynamism and visual appeal! This curated collection offers diverse functionalities, from image animation to interactive galleries. Let's explore these powerful tools: Related Posts: 1
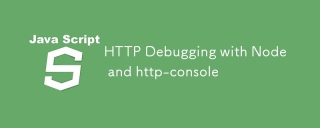
http-console is a Node module that gives you a command-line interface for executing HTTP commands. It’s great for debugging and seeing exactly what is going on with your HTTP requests, regardless of whether they’re made against a web server, web serv
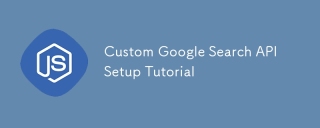
This tutorial shows you how to integrate a custom Google Search API into your blog or website, offering a more refined search experience than standard WordPress theme search functions. It's surprisingly easy! You'll be able to restrict searches to y
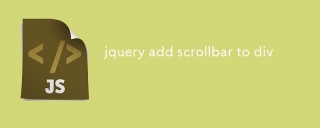
The following jQuery code snippet can be used to add scrollbars when the div content exceeds the container element area. (No demonstration, please copy it directly to Firebug) //D = document //W = window //$ = jQuery var contentArea = $(this), wintop = contentArea.scrollTop(), docheight = $(D).height(), winheight = $(W).height(), divheight = $('#c


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
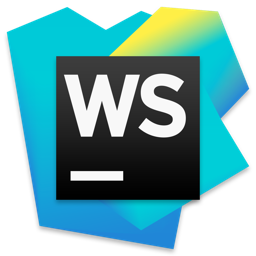
WebStorm Mac version
Useful JavaScript development tools
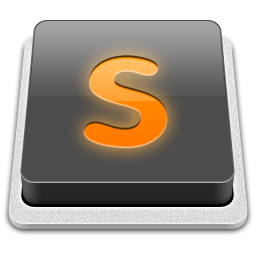
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
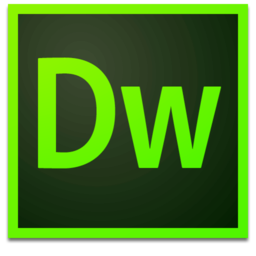
Dreamweaver Mac version
Visual web development tools
