This course uses PHP and Web front-end technology to implement a website registration and login entry page, learn and practice PHP programming, etc. Interested students can refer to it.
This article introduces the realization of user registration and login function based on PHP. This project is divided into four parts: 1. Front-end page production, 2. Verification code production, 3. Implementation of registration and login, 4. Functional improvement. Details can be found below.
Verification code production
1. Experiment introduction
This experiment will lead you to use object-oriented thinking to encapsulate a verification code kind. And displayed on the registration and login interface. Through the study of this experiment, you will understand the OOP ideas of PHP, as well as the use of GD library and verification code generation.
1.1 Knowledge points involved
PHP
GD library
OOP Programming
1.2 Development Tools
sublime, a convenient and fast text editor. Click on the lower left corner of the desktop: Application menu/Development/sublime
2. Encapsulation verification code class
2.1 Create a directory and prepare fonts
Create an admin directory in the web directory as our backend directory to store backend code files. Create a fonts directory under admin to store the fonts required for making verification codes.
Create a new Captcha.php file under admin. This is the verification code file we need to edit.
Current directory hierarchy:
Edit Captcha.php file:
<?php /** * Captcha class */ class Captcha { function __construct() { # code... } }
Add the private properties and construction methods of this class:
<?php /** * Captcha class */ class Captcha { private $codeNum; //验证码位数 private $width; //验证码图片宽度 private $height; //验证码图片高度 private $img; //图像资源句柄 private $lineFlag; //是否生成干扰线条 private $piexFlag; //是否生成干扰点 private $fontSize; //字体大小 private $code; //验证码字符 private $string; //生成验证码的字符集 private $font; //字体 function __construct($codeNum = 4,$height = 50,$width = 150,$fontSize = 20,$lineFlag = true,$piexFlag = true) { $this->string = 'qwertyupmkjnhbgvfcdsxa123456789'; //去除一些相近的字符 $this->codeNum = $codeNum; $this->height = $height; $this->width = $width; $this->lineFlag = $lineFlag; $this->piexFlag = $piexFlag; $this->font = dirname(__FILE__).'/fonts/consola.ttf'; $this->fontSize = $fontSize; } }
Font files can be downloaded to the fonts directory through the following command:
$ wget http://labfile.oss.aliyuncs.com/courses/587/consola.ttf
Next, start writing the specific method:
Create image resources Handle
//创建图像资源 public function createImage(){ $this->img = imagecreate($this->width, $this->height); //创建图像资源 imagecolorallocate($this->img,mt_rand(0,100),mt_rand(0,100),mt_rand(0,100)); //填充图像背景(使用浅色) }
Related functions used
imagecreate: Create a new palette-based image
imagecolorallocate: Assign a color to an image
mt_rand: Generate better random numbers
Create the verification code string and output it to the image
//创建验证码 public function createCode(){ $strlen = strlen($this->string)-1; for ($i=0; $i < $this->codeNum; $i++) { $this->code .= $this->string[mt_rand(0,$strlen)]; //从字符集中随机取出四个字符拼接 } $_SESSION['code'] = $this->code; //加入 session 中 //计算每个字符间距 $diff = $this->width/$this->codeNum; for ($i=0; $i < $this->codeNum; $i++) { //为每个字符生成颜色(使用深色) $txtColor = imagecolorallocate($this->img,mt_rand(100,255),mt_rand(100,255),mt_rand(100,255)); //写入图像 imagettftext($this->img, $this->fontSize, mt_rand(-30,30), $diff*$i+mt_rand(3,8), mt_rand(20,$this->height-10), $txtColor, $this->font, $this->code[$i]); } }
Related functions used
imagecreate: Create a new palette-based image
imagecolorallocate: Assign a color to an image
mt_rand: Generate better random numbers
Create a verification code string and output it to the image
//创建验证码 public function createCode(){ $strlen = strlen($this->string)-1; for ($i=0; $i < $this->codeNum; $i++) { $this->code .= $this->string[mt_rand(0,$strlen)]; //从字符集中随机取出四个字符拼接 } $_SESSION['code'] = $this->code; //加入 session 中 //计算每个字符间距 $diff = $this->width/$this->codeNum; for ($i=0; $i < $this->codeNum; $i++) { //为每个字符生成颜色(使用深色) $txtColor = imagecolorallocate($this->img,mt_rand(100,255),mt_rand(100,255),mt_rand(100,255)); //写入图像 imagettftext($this->img, $this->fontSize, mt_rand(-30,30), $diff*$i+mt_rand(3,8), mt_rand(20,$this->height-10), $txtColor, $this->font, $this->code[$i]); } }
Related functions used:
imagettftext: Write text to an image using TrueType fonts
Create interference lines
//创建干扰线条(默认四条) public function createLines(){ for ($i=0; $i < 4; $i++) { $color = imagecolorallocate($this->img,mt_rand(0,155),mt_rand(0,155),mt_rand(0,155)); //使用浅色 imageline($this->img,mt_rand(0,$this->width),mt_rand(0,$this->height),mt_rand(0,$this->width),mt_rand(0,$this->height),$color); } }
Related functions used:
imageline: draw a line segment
Create interference points
//创建干扰点 (默认一百个点) public function createPiex(){ for ($i=0; $i < 100; $i++) { $color = imagecolorallocate($this->img,mt_rand(0,255),mt_rand(0,255),mt_rand(0,255)); imagesetpixel($this->img,mt_rand(0,$this->width),mt_rand(0,$this->height),$color); } }
Related functions used:
imagesetpixel: draw a single pixel
External output image:
public function show() { $this->createImage(); $this->createCode(); if ($this->lineFlag) { //是否创建干扰线条 $this->createLines(); } if ($this->piexFlag) { //是否创建干扰点 $this->createPiex(); } header('Content-type:image/png'); //请求页面的内容是png格式的图像 imagepng($this->img); //以png格式输出图像 imagedestroy($this->img); //清除图像资源,释放内存 }
Related functions used:
imagepng: Output the image to the browser or file in PNG format
imagedestroy: Destroy an image
Provide verification code to the outside world:
public function getCode(){ return $this->code; } 完整代码如下: <?php /** * Captcha class */ class Captcha { private $codeNum; private $width; private $height; private $img; private $lineFlag; private $piexFlag; private $fontSize; private $code; private $string; private $font; function __construct($codeNum = 4,$height = 50,$width = 150,$fontSize = 20,$lineFlag = true,$piexFlag = true) { $this->string = 'qwertyupmkjnhbgvfcdsxa123456789'; $this->codeNum = $codeNum; $this->height = $height; $this->width = $width; $this->lineFlag = $lineFlag; $this->piexFlag = $piexFlag; $this->font = dirname(__FILE__).'/fonts/consola.ttf'; $this->fontSize = $fontSize; } public function createImage(){ $this->img = imagecreate($this->width, $this->height); imagecolorallocate($this->img,mt_rand(0,100),mt_rand(0,100),mt_rand(0,100)); } public function createCode(){ $strlen = strlen($this->string)-1; for ($i=0; $i < $this->codeNum; $i++) { $this->code .= $this->string[mt_rand(0,$strlen)]; } $_SESSION['code'] = $this->code; $diff = $this->width/$this->codeNum; for ($i=0; $i < $this->codeNum; $i++) { $txtColor = imagecolorallocate($this->img,mt_rand(100,255),mt_rand(100,255),mt_rand(100,255)); imagettftext($this->img, $this->fontSize, mt_rand(-30,30), $diff*$i+mt_rand(3,8), mt_rand(20,$this->height-10), $txtColor, $this->font, $this->code[$i]); } } public function createLines(){ for ($i=0; $i < 4; $i++) { $color = imagecolorallocate($this->img,mt_rand(0,155),mt_rand(0,155),mt_rand(0,155)); imageline($this->img,mt_rand(0,$this->width),mt_rand(0,$this->height),mt_rand(0,$this->width),mt_rand(0,$this->height),$color); } } public function createPiexs(){ for ($i=0; $i < 100; $i++) { $color = imagecolorallocate($this->img,mt_rand(0,255),mt_rand(0,255),mt_rand(0,255)); imagesetpixel($this->img,mt_rand(0,$this->width),mt_rand(0,$this->height),$color); } } public function show() { $this->createImage(); $this->createCode(); if ($this->lineFlag) { $this->createLines(); } if ($this->piexFlag) { $this->createPiexs(); } header('Content-type:image/png'); imagepng($this->img); imagedestroy($this->img); } public function getCode(){ return $this->code; } }
The above is all the code for the verification code class. It does seem quite simple, but it uses a lot of image processing functions. I have also made necessary links and usage instructions for the above related functions. There is no need to memorize these functions by rote. If you encounter something unclear, please refer to the official PHP documentation at any time. The most important thing is that there are Chinese documentation.
2.2 Use the verification code
Now that it has been encapsulated, you can start using it. For convenience here, call this class directly below the Captcha class:
session_start(); //开启session $captcha = new Captcha(); //实例化验证码类(可自定义参数) $captcha->show(); //调用输出
3. After the front-end display
The verification code has been prepared on the end, and the front-end interface can be displayed. Modify the verification code part of the registration and login form in index.php:
<p class="form-group"> <p class="col-sm-12"> <img src="/static/imghwm/default1.png" data-src="admin/Captcha.php" class="lazy" alt="" id="codeimg" onclick="javascript:this.src = 'admin/Captcha.php?'+Math.random();"> <span>Click to Switch</span> </p> </p># The js code of the click event is added to the ##img tag, so that the function of clicking to change the verification code can be realized!
Summary: The above is the entire content of this article, I hope it will be helpful to everyone's study.
Related recommendations:
PHP uses Curl to implement simulated login and data capture function examples
php Use cookies to implement the function of remembering user names and passwords on web pages
phpSolution to the problem of garbled characters after testing with Apache
The above is the detailed content of How to implement user registration and login function in PHP. For more information, please follow other related articles on the PHP Chinese website!
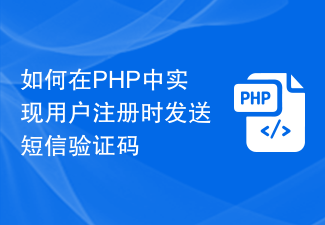
如何在PHP中实现用户注册时发送短信验证码随着移动互联网的普及,手机号码已经成为用户注册和登录的重要凭证之一。为了保证用户账号的安全性,很多网站和应用都会在用户注册时发送短信验证码进行验证。本文将介绍如何在PHP中实现用户注册时发送短信验证码的功能,并附上具体的代码示例。一、创建短信验证码发送接口首先,我们需要创建一个短信验证码发送接口,用于向用户的手机号码
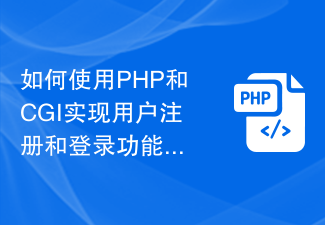
如何使用PHP和CGI实现用户注册和登录功能用户注册和登录是许多网站必备的功能之一。在本文中,我们将介绍如何使用PHP和CGI来实现这两个功能。我们将通过代码示例来演示整个过程。一、用户注册功能的实现用户注册功能允许新用户创建一个账户,并将其信息保存到数据库中。以下是实现用户注册功能的代码示例:创建数据库表首先,我们需要创建一个数据库表,用于存储用户信息。可
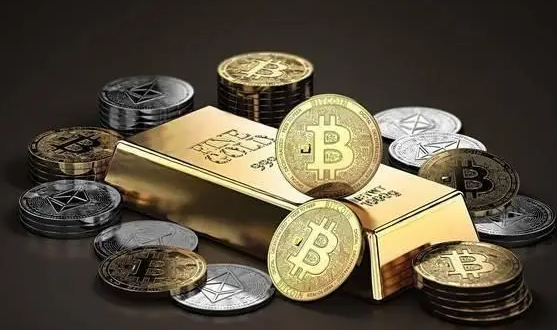
芝麻交易所是我国领先的在线交易平台之一,为各类商品提供安全、高效的交易服务。它的交易流程包括用户注册、商品展示、订单生成、交易撮合、物流配送以及售后服务等多个环节。本文将详细介绍芝麻交易所的交易流程,帮助读者更好地了解和使用该平台。用户注册与登录在使用芝麻交易所进行交易之前,用户必须注册并登录。用户可以选择通过手机验证码、第三方账号或手动注册的方式登录。在注册过程中,用户需要提供真实有效的个人信息,并同意遵守芝麻交易所的交易规则和用户协议。商品展示与搜索注册成功后,用户可进入芝麻交易所主页,通过
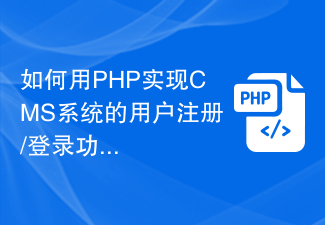
如何用PHP实现CMS系统的用户注册/登录功能?随着互联网的发展,CMS(ContentManagementSystem,内容管理系统)系统成为了网站开发中非常重要的一环。而其中的用户注册/登录功能更是不可或缺的一部分。本文将介绍如何使用PHP语言实现CMS系统的用户注册/登录功能,并附上相应的代码示例。以下是实现步骤:创建用户数据库首先,我们需要建立一
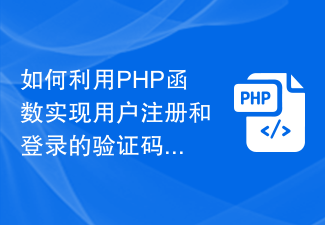
如何利用PHP函数实现用户注册和登录的验证码生成和验证?在网站的用户注册和登录页面中,为了防止机器人批量注册和攻击,通常需要添加验证码功能。本文将介绍如何利用PHP函数实现用户注册和登录的验证码生成和验证。验证码生成首先,我们需要生成随机的验证码图片供用户填写。PHP提供了GD库和图像处理函数,可以方便地生成验证码图片。<?php//创建一个画布
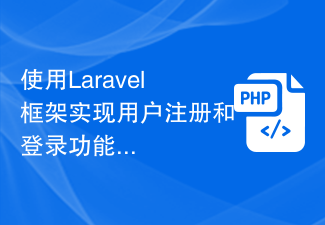
使用Laravel框架实现用户注册和登录功能的步骤Laravel是一个流行的PHP开发框架,提供了许多强大的功能和工具,使得开发者可以轻松构建各种Web应用程序。用户注册和登录是任何应用程序的基本功能之一,下面我们将使用Laravel框架来实现这两个功能。步骤1:创建新的Laravel项目首先,我们需要在本地计算机上创建一个新的Laravel项目。打开终端或
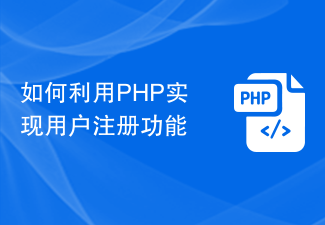
如何利用PHP实现用户注册功能在现代的网络应用程序中,用户注册功能是一个非常常见的需求。通过注册功能,用户可以创建自己的账户并使用相应的功能。本文将通过PHP编程语言来实现用户注册功能,并提供详细的代码示例。首先,我们需要创建一个HTML表单,用于接收用户的注册信息。在表单中,我们需要包含一些输入字段,如用户名、密码、邮箱等。可以根据实际需求自定义表单字段。
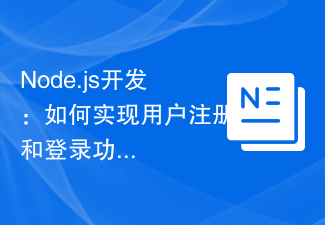
Node.js开发:如何实现用户注册和登录功能,需要具体代码示例引言:在Web应用程序开发过程中,用户注册和登录功能是必不可少的一部分。本文将详细介绍如何使用Node.js实现用户注册和登录功能,提供具体的代码示例。一、用户注册功能的实现创建数据库首先,我们需要创建一个数据库来存储用户的注册信息。可以使用MongoDB、MySQL等数据库来存储用户信息。创建


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
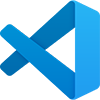
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
