


This article mainly introduces the detailed explanation of how to use traits in PHP. Interested friends can refer to it. I hope it will be helpful to everyone. To put it simply, the trait keyword is used in PHP to solve the problem that a class wants to integrate the attributes and methods of a base class, but also wants to have methods of other base classes, and traits are generally used in conjunction with use.
<?php trait Drive { public $carName = 'trait'; public function driving() { echo "driving {$this->carName}\n"; } } class Person { public function eat() { echo "eat\n"; } } class Student extends Person { use Drive; public function study() { echo "study\n"; } } $student = new Student(); $student->study(); $student->eat(); $student->driving(); ?>
The output results are as follows:
study eat driving trait
In the above example, the Student class has the eat method by inheriting Person, and the driving method and attribute carName by combining Drive.
If there is a property or method with the same name in Trait, base class and this class, which one will be retained in the end?
<?php trait Drive { public function hello() { echo "hello drive\n"; } public function driving() { echo "driving from drive\n"; } } class Person { public function hello() { echo "hello person\n"; } public function driving() { echo "driving from person\n"; } } class Student extends Person { use Drive; public function hello() { echo "hello student\n"; } } $student = new Student(); $student->hello(); $student->driving(); ?>
The output results are as follows:
hello student driving from drive
So we come to the conclusion: when a method or attribute has the same name, the method in the current class will override the trait's method, and the trait's method will override the method in the base class.
If you want to combine multiple Traits, separate the Trait names by commas:
use Trait1, Trait2;
If multiple Traits contain What will happen if the method or property has the same name? The answer is that when multiple combined Traits contain properties or methods with the same name, they need to be explicitly declared to resolve conflicts, otherwise a fatal error will occur.
<?php trait Trait1 { public function hello() { echo "Trait1::hello\n"; } public function hi() { echo "Trait1::hi\n"; } } trait Trait2 { public function hello() { echo "Trait2::hello\n"; } public function hi() { echo "Trait2::hi\n"; } } class Class1 { use Trait1, Trait2; } ?>
The output results are as follows:
Copy code The code is as follows:
PHP Fatal error: Trait method hello has not been applied, because there are collisions with other trait methods on Class1 in ~/php54/trait_3.php on line 20
Use insteadof and as operators to resolve conflicts. Insteadof uses a method to replace another, while as gives an alias to the method. Please see the code for specific usage:
<?php trait Trait1 { public function hello() { echo "Trait1::hello\n"; } public function hi() { echo "Trait1::hi\n"; } } trait Trait2 { public function hello() { echo "Trait2::hello\n"; } public function hi() { echo "Trait2::hi\n"; } } class Class1 { use Trait1, Trait2 { Trait2::hello insteadof Trait1; Trait1::hi insteadof Trait2; } } class Class2 { use Trait1, Trait2 { Trait2::hello insteadof Trait1; Trait1::hi insteadof Trait2; Trait2::hi as hei; Trait1::hello as hehe; } } $Obj1 = new Class1(); $Obj1->hello(); $Obj1->hi(); echo "\n"; $Obj2 = new Class2(); $Obj2->hello(); $Obj2->hi(); $Obj2->hei(); $Obj2->hehe(); ?>
The output results are as follows:
Trait2::hello Trait1::hi Trait2::hello Trait1::hi Trait2::hi Trait1::hello
## The
<?php trait Hello { public function sayHello() { echo "Hello\n"; } } trait World { use Hello; public function sayWorld() { echo "World\n"; } abstract public function getWorld(); public function inc() { static $c = 0; $c = $c + 1; echo "$c\n"; } public static function doSomething() { echo "Doing something\n"; } } class HelloWorld { use World; public function getWorld() { return 'get World'; } } $Obj = new HelloWorld(); $Obj->sayHello(); $Obj->sayWorld(); echo $Obj->getWorld() . "\n"; HelloWorld::doSomething(); $Obj->inc(); $Obj->inc(); ?>
Hello World get World Doing something 1 2
[php classes and objects]trait
php Traits attributes and basic usage
Code reuse Trait usage steps detailed explanation
The above is the detailed content of Detailed graphic and text explanation of how to use traits in PHP. For more information, please follow other related articles on the PHP Chinese website!
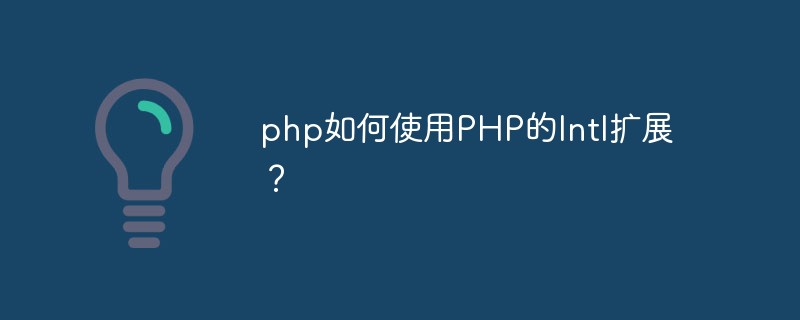
PHP的Intl扩展是一个非常实用的工具,它提供了一系列国际化和本地化的功能。本文将介绍如何使用PHP的Intl扩展。一、安装Intl扩展在开始使用Intl扩展之前,需要安装该扩展。在Windows下,可以在php.ini文件中打开该扩展。在Linux下,可以通过命令行安装:Ubuntu/Debian:sudoapt-getinstallphp7.4-

随着网络技术的发展,PHP已经成为了Web开发的重要工具之一。而其中一款流行的PHP框架——CodeIgniter(以下简称CI)也得到了越来越多的关注和使用。今天,我们就来看看如何使用CI框架。一、安装CI框架首先,我们需要下载CI框架并安装。在CI的官网(https://codeigniter.com/)上下载最新版本的CI框架压缩包。下载完成后,解压缩
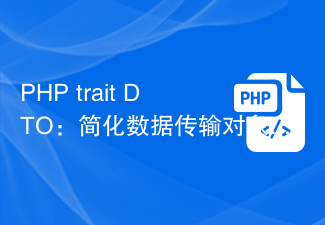
PHPtraitDTO:简化数据传输对象的开发引言:在现代的软件开发中,数据传输对象(DataTransferObject,简称DTO)起到了重要的作用。DTO是一种纯粹的数据容器,用于在层与层之间传递数据。然而,在开发过程中,开发人员需要编写大量的相似的代码来定义和操作DTO。为了简化这一过程,PHP中引入了trait特性,我们可以利用trait特
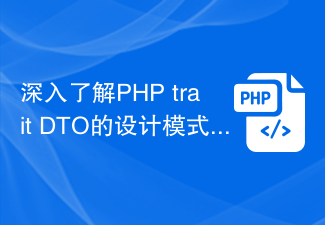
深入了解PHPtraitDTO的设计模式与实践Introduction:在PHP开发中,设计模式是必不可少的一部分。其中,DTO(DataTransferObject)是一种常用的设计模式,用于封装数据传输的对象。而在实现DTO的过程中,使用trait(特征)可以有效地提高代码的复用性和灵活性。本文将深入探讨PHP中traitDTO的设计模式与实践

PHP是一种流行的服务器端脚本语言,它可以处理网页上的动态内容。PHP的geoip扩展可以让你在PHP中获取有关用户位置的信息。在本文中,我们将介绍如何使用PHP的geoip扩展。什么是PHP的GeoIP扩展?PHP的geoip扩展是一个免费的、开源的扩展,它允许你获取有关IP地址和位置信息的数据。该扩展可以与GeoIP数据库一起使用,这是一个由MaxMin
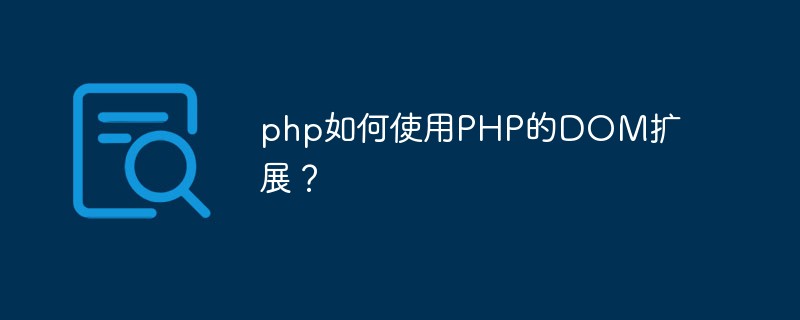
PHP的DOM扩展是一种基于文档对象模型(DOM)的PHP库,可以对XML文档进行创建、修改和查询操作。该扩展可以使PHP语言更加方便地处理XML文件,让开发者可以快速地实现对XML文件的数据分析和处理。本文将介绍如何使用PHP的DOM扩展。安装DOM扩展首先需要确保PHP已经安装了DOM扩展,如果没有安装需要先安装。在Linux系统中,可以使用以下命令来安
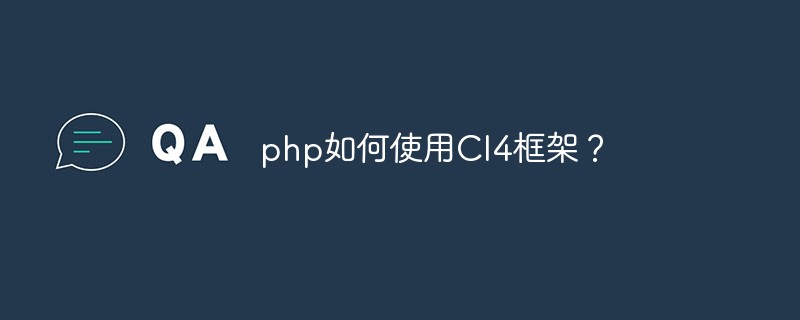
PHP是一种广泛使用的服务器端脚本语言,而CodeIgniter4(CI4)是一个流行的PHP框架,它提供了一种快速而优秀的方法来构建Web应用程序。在这篇文章中,我们将通过引导您了解如何使用CI4框架,来使您开始使用此框架来开发出众的Web应用程序。1.下载并安装CI4首先,您需要从官方网站(https://codeigniter.com/downloa
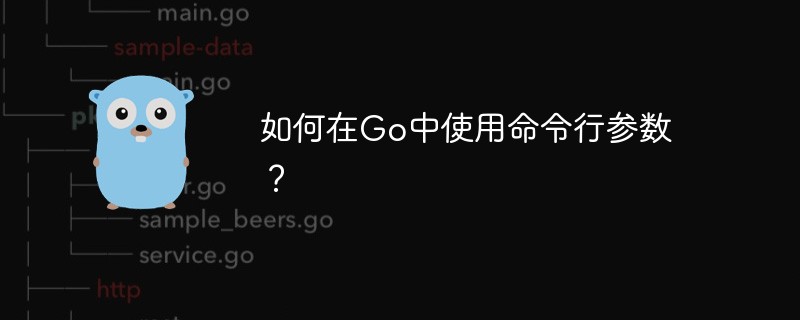
在Go语言中,命令行参数是非常重要的一种方式,用于向程序传递输入并指定运行时的行为。Go提供了一个标准库flag来解析命令行参数,本文将介绍如何在Go中使用命令行参数。什么是命令行参数命令行参数是在程序运行时通过命令行传递给程序的参数,用于指定程序运行时的行为和输入。举个例子,Linux中的ls命令可以接受多个命令行参数,如-l用于列出详细信息,-a用于显示


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
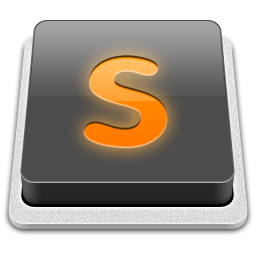
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
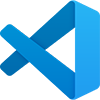
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
