This article makes a relevant summary of the use of jquery.
jQuery is a small, fast, and powerful JavaScript library. It simplifies many things through some easy-to-use APIs, such as: DOM operations, event listening, animation, AJAX, etc.
What jQuery can do, native JS can do, but native JS will be more complicated, and turning complexity into simplicity is the purpose of jQuery.
Write less, do more.
What is the difference between jQuery objects and DOM native objects? How to convert?
Suppose such an HTML fragment
We can use jQuery method or native DOM method Obtain this element node
$("#btn1"); //jQuesy方法;document.querySelector("#btn1"); //原生DOM方法;
The element objects obtained through these two methods are two completely different objects.
What is obtained by the jQuesy method is called a jQuesy object. It is an array-like object. It has its own methods and cannot use native DOM methods.
What is obtained by the native DOM method is called a DOM native object, which also has its own The method of jQuery cannot be used;
The two objects can be converted to each other
$("#btn1")[0]; //jQuery object is converted into a native DOM object. Use the index to get the corresponding element object; $(document.querySelector("#btn1")); //Wrap the DOM element object with $ to get the jQuery object;
How to bind in jQuery event? What are the functions of bind, unbind, delegate, live, on, and off? Which one is recommended? How to use on to bind events and use event proxy?
In jQuery, we can use the on() method to bind events
$("#btn1").on("click",function() { //最简单的事件绑定; console.log("hello world"); })
Other event binding methods
bind(), this method has been abandoned in versions after 3.0 Use, use on() instead;
1.4.3 version, it accepts the following parameters: eventType (event type, "click", etc.), eventData (data passed to the event processing function), handler (event processing function) ), preventBubble (a Boolean to prevent default events and prevent events from bubbling)
Since it can only be bound to existing elements, newly added elements will not be bound to events, which is not flexible enough and has been deprecated;
unbind(), this method can remove the events bound by the bind() method. If no parameters are passed, all events will be deleted. Passing parameters can delete the specified events and event processing functions
delegate() method is a commonly used event proxy method before version 1.7, and has been replaced by on(). Accepts several parameters:
selector: selector string, used to filter elements that trigger events
evenType: event type, multiple separated by spaces
eventData: data passed to the event handler
handler: event handler
live() is also a method of event proxy, attaching an event handler to all elements matched by the selector, but it binds the event directly to the document, through related Parameters to determine whether to trigger an event
events: event type
data: data passed to the event handler
handler: event handler
Because live() binds the event to the document, This caused the bubbling chain to be too long and was deprecated.
on() is a common method for binding events now, accepting several parameters
1.events: one or more space-separated event types and optional namespaces, or just naming Space, such as "click", "keydown.myPlugin", or ".myPlugin";
2.selector: A selector string used to filter out descendant elements that can trigger events among the selected elements. If the selector is null or the selector is ignored, the selected element can always trigger the event;
3.data: When an event is triggered, the event.data to be passed to the event handler function;
4.handler(eventObject): Function executed when the event is triggered. If the function only needs to execute returnfalse, then the parameter position can be directly abbreviated as false
off() method can remove the event bound by the on() method. If no parameters are passed, all events will be deleted. Events, passing parameters can delete the specified event and event handling function
jQuery event proxy writing method
Assume HTML fragment
<ul id="container"> <li>content1</li> <li>content2</li> <li>content3</li></ul>
We can use the on() method and provide relevant parameters, You can complete the event proxy
//Bind the event to the parent container. Only child elements that meet the filter selector can trigger the event $("#container").on("click","li ",function() { //do something..})
jQuery How to show/hide elements?
In jQuery, elements are hidden through the hide() method, which accepts three parameters:
[duration]: How long the animation lasts
[easing]: Indicates which easing function is used for transition, jQuery itself Provide "linear" and "swing"
[complete]: a function executed when the animation is completed
When no parameters are added, the method is equivalent to directly setting the display of the element to none
By adding parameters, this method You can achieve the effect of a gradient hidden element
$(element).hide() -------- $(element).hide(3000,function() { alert("hello world") })
Similarly, jQuery uses the show() method to display hidden elements. The usage method is the same as hide()
How to use jQuery animation?
jQuery中通过hide()方法隐藏元素,其接受三个参数
[duration]:动画持续多久
[easing]:表示过渡使用哪种缓动函数,jQuery自身提供"linear" 和 "swing"
[complete]:在动画完成时执行的函数
不添加参数时,其方法等同于直接设置元素的display为none
通过添加参数,该方法可以实现一个渐变的隐藏元素的效果
$(element).hide() -------- $(element).hide(3000,function() { alert("hello world") })
同样,jQuery中使用show()方法来展示隐藏的元素,使用方法与hide()相同
hide()方法会把元素的display变为none,show()方法会还原元素的display
toggle()方法用于切换元素的隐藏/显示,参数与hide()``show()相同,它的机制就是元素如果是隐藏的,就显示该元素,如果元素是显示的,就隐藏该元素,来回切换
fadeIn()/fadeOut用调整元素透明度的方法来显示/隐藏元素,一旦透明度变为0,display将设置为none,接受参数与hide()、show()相同
不设置参数,fadeIn()/fadeOut默认会有渐进显示/隐藏的效果
$(element).fadeIn()
$(element).fadeOut()
fadeTo以动画的形式调整元素到指定的透明度,接受这几个参数:
duration, opacity [, easing ] [, complete ]
opacity为指定变化的透明度
当opacity为0时,fadeTo方法不会使元素display为none
$(element).fadeTo(1000,0.5) //在1s内透明度变化到0.5
fadeToggle会通过改变透明度的方式显示和隐藏元素,如果元素是隐藏的,则显示,显示的,则隐藏,参数与fadeIn()``fadeOut()相同
fadeToggle在元素透明度为0时,会display为none
fadeIn()/fadeOut和show()/hide()的区别:
前者通过调整元素透明度实现元素隐藏和显示,透明度为0时设置display为none。后者通过改变同时元素的width/height/opacity来显示隐藏元素
slideUp()/slideDown()通过上下滑动来实现元素的隐藏/显示,接受参数与show()/hide()相同
slideToggle()通过上下滑动的方式切换元素的隐藏/显示
animate()是自定义动画方法,接受这几个参数
properties:一个CSS属性和值的对象,动画将根据这组对象进行变化
[duration]:动画时间
[easing]:缓动函数
[complete]:完成动画后的回调函数
animate()的本质是通过动画的方式把元素的样式变为指定的样式
animate()可以通过链式调用实现多个动画
$(element).animate({//something}) .animate({//something}) .animate({//something}) .animate({//something})
多个动画可以整合到一个数组中,对数组进行遍历,执行所有动画
var action = [{//action1}, {//action2}, {//action3}, {//action4}]action.forEach(function(action,index) { ${element}.animate(action) })
stop()方法可以停止当前动画,它接受2个参数:
[clearQueue]:一个布尔值,当为true时,当前动画停止,未执行的动画全部删除
[jumpToEnd]:为true时,当前动画将停止,但该元素上的 css属性会被立刻修改成动画的目标值
stop()不添加任何参数时,会立即结束掉元素当前动画(不完成),马上进入下一个动画(如果有的话)
如何设置和获取元素内部 HTML 内容?如何设置和获取元素内部文本?
在原生DOM中,我们可以使用innerText操作元素文本,innerHTML操作元素内的HTML
在jQuery中提供了相同功能的方法:html()和text()
当没有传递参数时,获取元素内的innerHTML和innerText;当传递了一个string参数的时候,修改元素的innerHTM和innerText为参数值
<ul id="container"> <li>content1</li></ul> $("#container").html() //"<li>content1</li>"$("#container").text() //"content1"
如何设置和获取表单用户输入或者选择的内容?如何设置和获取元素属性?
val()方法可以用来获取和设置input的value
当没有传递参数时,获取value的值
传递一个字符串参数时,将value的值改为参数值
attr()可以获取指定属性,也可以设置属性
$(element).attr("id") //获取元素id属性值$(element).attr("id","container") //设置元素id值为container
本篇对jquery的作用进行了相关的讲解,更多相关内容请关注php中文网。
相关推荐:
The above is the detailed content of What can jQuery do?. For more information, please follow other related articles on the PHP Chinese website!
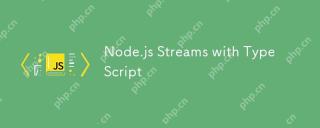
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
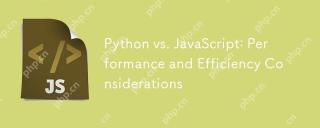
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
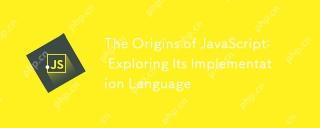
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
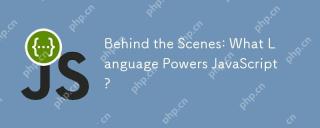
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
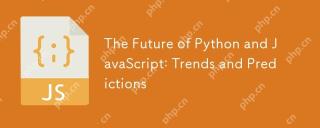
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
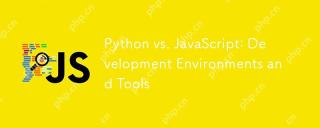
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
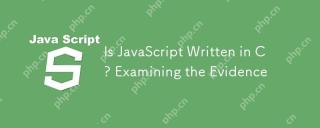
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
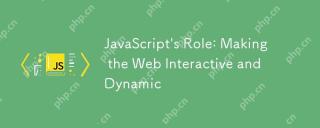
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
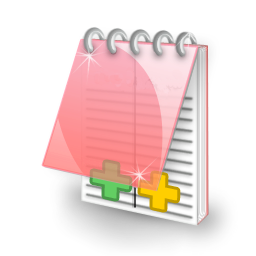
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
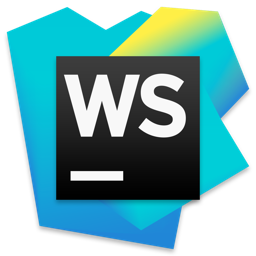
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
