This time I will bring you a detailed explanation of how to use pack and unpack in PHP, and a brief discussion of the precautions for using pack and unpack in PHP. The following is a practical case, let's take a look.
This function is used to pack the corresponding parameters ($args) into a binary string.
The first parameter, $format, has the following options (there are many optional parameters, and I will select a few common ones to explain later):
Code |
Description |
a |
Padding string gaps with NUL bytes |
A |
Fill the string with SPACE(space) |
h |
Hexadecimal string, low digit first |
H |
Hexadecimal string, high-order bit first |
c |
Signed characters |
C |
Unsigned character |
s |
Signed short (16 bits, host byte order) |
S |
Unsigned short (16 bits, host byte order) |
n |
None Signed short (16 bits, big endian) |
v |
Unsigned short (16 bits, little endian) |
i |
Signed integer (machine dependent size endian) |
I |
Unsigned Integer type (machine dependent size byte order) |
l |
Signed long integer type (32 bits, host byte order) |
L |
Unsigned long (32-bit, host byte order) |
##N | Unsigned long (32 bits, big endian) |
V | Unsigned long integer (32 bits, little endian) |
q | Signed long integer (64 bits, host byte order) |
Q | Unsigned long Long integer type (64 bits, host byte order) |
J | Unsigned long integer type (64 bits, big endian byte order) |
P | Unsigned long integer (64 bits, little endian) |
f | Single PrecisionFloating point type(machine dependent size)
|
d | Double precision floating point type(machine dependent size) |
x | NUL byte |
X | Roll back one byte |
Z | Padding string blanks with NUL bytes (new in PHP 5.5) |
@ | NUL padding to absolute position |
这么多参数看下来,我第一次是真心懵逼了,大部分说明都很好理解,但是其中的主机、大端、小端等字节序是什么鬼呢?接下里的内容比较枯燥,但必须理解才行,坚持吧。
字节序是什么?
就是字节的顺序,说白了就是多字节数据的存放顺序(一个字节显然不需要顺序)。
比如A和B分别对应的二进制表示为0100 0001、0100 0010。对于储存字符串AB,我们可以0100 0001 0100 0010也可以0100 0010 0100 0001,这个顺序就是所谓的字节序。
高/低位字节
比如字符串AB,左高右低(我们正常的阅读顺序),A为高字节,B为低字节
高/低地址
假设0x123456是按从高位到底位的顺序储存,内存中是这样存放的:
高地址 -> 低地址
12 -> 34 -> 56
大端字节序(网络字节序)
大端就是将高位字节放到内存的低地址端,低位字节放到高地址端。网络传输中(比如TCP/IP)低地址端(高位字节)放在流的开始,对于2个字节的字符串(AB),传输顺序为:A(0-7bit)、B(8-15bit)。
那么小端字节序自然和大端相反。
主机字节序
表示当年机器的字节序(也就是网络字节序是确定的,而主机字节序是依机器确定的),一般为小端字节序。
a和A(打包字符串,用NUL或者空格填充)
$string = pack('a6', 'china');
var_dump($string); //输出结果: string(6) "china",最后一个字节是不可见的NUL
echo ord($string[5]); //输出结果: 0(ASCII码中0对应的就是nul)
//A同理
$string = pack('A6', 'china');
var_dump($string); //输出结果: string(6) "china ",最后一个字节是空格
echo ord($string[5]); //输出结果: 32(ASCII码中32对应的就是空格)
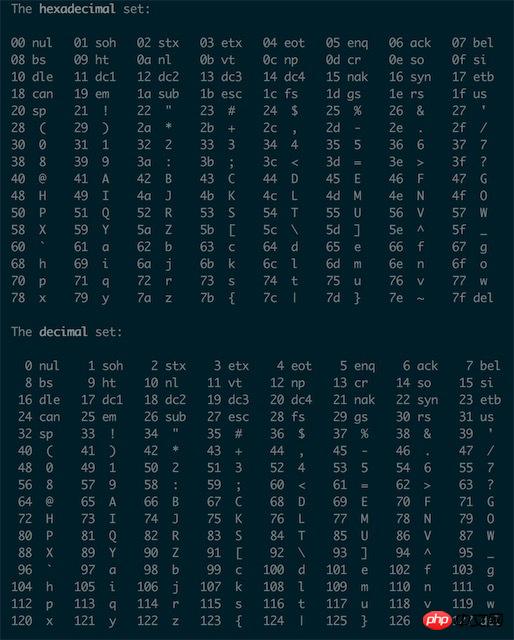
附赠ASCII表一张(linux/unix下可以使用man ascii查看)
h和H
$string = pack('H3', 281);
var_dump($string); //输出结果: string(2) "("
for($i=0;$i<strlen($string);$i++) {
echo ord($string[$i]) . PHP_EOL;
}
//输出结果: 40 16
h和H需要特殊说明一下,它们是将对应的参数看做十六进制字符然后打包。什么意思呢?比如上面的281,打包前会将281转换为0x281,因为十六进制的一位对应二进制的四位,上面的0x281只有1.5个字节,后面会默认补0变成0x2810,0x28对应的十进制为40((),0x10对应的十进制为16(dle不可见字符),懂了吧?不懂可以给我留言。。
c和C
$string = pack('c3', 67, 68, -1);
var_dump($string); //输出:string(3) "CD�"
for($i=0;$i<strlen($string);$i++) {
echo ord($string[$i]) . PHP_EOL;
}
//输出: 67 68 225
最后输出本能应该觉得是67 68 -1
ord获取的是字符的ASCII码(范围0-255),这时-1(0000 0001)对应的字符将以补码的形式输出也就是255(1111 1110 + 0000 0001 = 1111 1111)
整型相关
所有的整型类型使用方法完全一样,主要注意它们的位和字节序就可以了,下面以L作为例子展示
$string = pack('L', 123456789);
var_dump($string); //输出:string(4) "�["
for($i=0;$i<strlen($string);$i++) {
echo ord($string[$i]) . PHP_EOL;
}
//输出: 21 205 91 7
f和d
$string = pack('f', 12345.123);
var_dump($string);
//输出:string(4) "~�@F"
var_dump(unpack('f', $string)); //这里提前用到了unpack,后面会讲解
//输出:float(12345.123046875)
f和d是针对浮点数打包,至于为什么打包前是12345.123解包后是12345.123046875,这个和浮点数的储存有关系,后面可以单开一个文章讲解一下IEEE标准
x、X、Z、@
$string = pack('x'); //打包一个nul字符串
echo ord($string); //输出: 0
关于X(大写X),试了N次,没搞明白怎么用,有清楚的童鞋可以给我留言,多谢。
$string = pack('Z2', 'abc5'); //其实就是将从Z后面的数字位置开始,全部设置为nul
var_dump($string); //输出:string(2) "a"
for($i=0;$i<strlen($string);$i++) {
echo ord($string[$i]) . PHP_EOL;
}
//输出: 97 0
$string = pack('@4'); //我理解为填充N个nul
var_dump($string); //输出: string(4) ""
for($i=0;$i<strlen($string);$i++) {
echo ord($string[$i]) . PHP_EOL;
}
//输出: 0 0 0 0
unpack
array unpack ( string $format , string $data )
unpack的使用相当简单,就是讲pack打包的数据解包,打包的时候用的什么参数,就用什么参数解包,具体使用懒得说了,列几个小例子
$string = pack('L4', 1, 2, 3, 4);
var_dump(unpack('L4', $string));
//输出:
array(4) {
[1]=>
int(1)
[2]=>
int(2)
[3]=>
int(3)
[4]=>
int(4)
}
$string = pack('L4', 1, 2, 3, 4);
var_dump(unpack('Ll1/Ll2/Ll3/Ll4', $string)); //可以指定key,用/分割
//输出:
array(4) {
["l1"]=>
int(1)
["l2"]=>
int(2)
["l3"]=>
int(3)
["l4"]=>
int(4)
}
这两个函数到底有啥用途
数据通信(通过二进制格式与其它语言通信)
数据加密(如果不告诉第三方你的打包方式,对方解包的难度就相对很大)
节省空间(比如比较大的数字按字符串储存会浪费很多空间,打包成二进制格式才需要4位<32位数字>)
相信看了本文案例你已经掌握了方法,更多精彩请关注php中文网其它相关文章!
推荐阅读:
php summary of methods for deleting duplicate values in a two-dimensional array
php operates a string and splits it into an array
The above is the detailed content of A brief discussion on how to use pack and unpack in PHP. For more information, please follow other related articles on the PHP Chinese website!