PHP Filter is used to verify and filter data from non-secure sources, such as user input. This article will learn more about filters in detail.
What is a PHP filter?
PHP filters are used to validate and filter data from non-secure sources.
Validating and filtering user input or custom data is an important part of any web application.
The PHP filter extension is designed to make data filtering easier and faster.
Why use filters?
Nearly all web applications rely on external input. This data usually comes from users or other applications (such as web services). By using filters, you can ensure that your application gets the correct input type.
You should always filter external data!
Input filtering is one of the most important application security topics.
What is external data?
Input data from forms
Cookies
Server variables
Database query results
Functions and filters
To filter variables, use one of the following filter functions:
filter_var() - Filter a single variable by a specified filter
filter_var_array() - Filter by the same or different filters to filter multiple variables
filter_input - Get an input variable , and filter it
filter_input_array - Get multiple input variables, and filter them by Filter them with the same or different filters
In the following example, we validate an integer using the filter_var() function:
<?php $int = 123; if(!filter_var($int, FILTER_VALIDATE_INT)) { echo("Integer is not valid"); } else { echo("Integer is valid"); } ?>
The above code uses the "FILTER_VALIDATE_INT" filter filter to filter variables. Since this integer is legal, the output of the code is: "Integer is valid".
If we try to use a non-integer variable, the output is: "Integer is not valid".
For a complete list of functions and filters, please visit our PHP Filter Reference Manual.
Validating and Sanitizing
There are two types of filters:
Validating filter:
Used to validate user input
strict Format rules (such as URL or E-Mail validation)
Returns the expected type if successful, or FALSE if failed
Sanitizing filter:
Used to allow or Disallows the specified characters in the string
No data format rules
Always returns the string
Options and flags
Options and flags are used to specify Filters add additional filtering options.
Different filters have different options and flags.
In the example below, we validate an integer using filter_var() with the "min_range" and "max_range" options:
<?php $var=300;$int_options = array( "options"=>array ( "min_range"=>0, "max_range"=>256 ) );if(!filter_var($var, FILTER_VALIDATE_INT, $int_options)) { echo("Integer is not valid"); } else { echo("Integer is valid"); } ?>
Like the code above, the options must be put in a in a related array called "options". If using flags, they don't need to be in an array.
Since the integer is "300", which is not within the specified range, the output of the above code will be "Integer is not valid".
For a complete list of functions and filters, please visit the PHP Filter reference manual provided by W3School. You can see the available options and flags for each filter.
Validate Input
Let's try to validate the input from the form.
The first thing we need to do is confirm whether the input data we are looking for exists.
Then we use the filter_input() function to filter the input data.
In the following example, the input variable "email" is passed to the PHP page:
<?php if(!filter_has_var(INPUT_GET, "email")) { echo("Input type does not exist"); } else { if (!filter_input(INPUT_GET, "email", FILTER_VALIDATE_EMAIL)) { echo "E-Mail is not valid"; } else { echo "E-Mail is valid"; } } ?>
Explanation of the example:
The above example has one passed through the "GET" method Input variable (email):
Detect whether there is "GET" type "email" input variable
If there is an input variable, check whether it is a valid email address
Purify input
Let's try to clean up the URL passed from the form.
First, we need to confirm that the input data we are looking for exists.
Then, we use the filter_input() function to purify the input data.
In the following example, the input variable "url" is passed to the PHP page:
<?php if(!filter_has_var(INPUT_POST, "url")) { echo("Input type does not exist"); } else { $url = filter_input(INPUT_POST, "url", FILTER_SANITIZE_URL); } ?>
Explanation of the example:
The above example has a transmission via the "POST" method Input variable (url):
Detect whether there is a "url" input variable of "POST" type
If this input variable exists, purify it (remove illegal characters), and It is stored in the $url variable
If the input variable is similar to this: "http://www.W3 illegal ol.com.c character n/", then the purified $url variable should be like this of:
http://www.W3School.com.cn/
Filter multiple inputs
Forms usually consist of multiple input fields. To avoid repeated calls to filter_var or filter_input, we can use filter_var_array or the filter_input_array function.
In this example, we use the filter_input_array() function to filter three GET variables. The GET variables received are a name, an age, and an email address:
<?php$filters = array ( "name" => array ( "filter"=>FILTER_SANITIZE_STRING ), "age" => array ( "filter"=>FILTER_VALIDATE_INT, "options"=>array ( "min_range"=>1, "max_range"=>120 ) ), "email"=> FILTER_VALIDATE_EMAIL, );$result = filter_input_array(INPUT_GET, $filters); if (!$result["age"]) { echo("Age must be a number between 1 and 120.<br />"); } elseif(!$result["email"]) { echo("E-Mail is not valid.<br />"); } else { echo("User input is valid"); } ?>
例子解释:
上面的例子有三个通过 "GET" 方法传送的输入变量 (name, age and email)
设置一个数组,其中包含了输入变量的名称,以及用于指定的输入变量的过滤器
调用 filter_input_array 函数,参数包括 GET 输入变量及刚才设置的数组
检测 $result 变量中的 "age" 和 "email" 变量是否有非法的输入。(如果存在非法输入,)
filter_input_array() 函数的第二个参数可以是数组或单一过滤器的 ID。
如果该参数是单一过滤器的 ID,那么这个指定的过滤器会过滤输入数组中所有的值。
如果该参数是一个数组,那么此数组必须遵循下面的规则:
必须是一个关联数组,其中包含的输入变量是数组的键(比如 "age" 输入变量)
此数组的值必须是过滤器的 ID ,或者是规定了过滤器、标志以及选项的数组
使用 Filter Callback
通过使用 FILTER_CALLBACK 过滤器,可以调用自定义的函数,把它作为一个过滤器来使用。这样,我们就拥有了数据过滤的完全控制权。
您可以创建自己的自定义函数,也可以使用已有的 PHP 函数。
规定您准备用到过滤器函数的方法,与规定选项的方法相同。
在下面的例子中,我们使用了一个自定义的函数把所有 "_" 转换为空格:
<?phpfunction convertSpace($string) { return str_replace("_", " ", $string); }$string = "Peter_is_a_great_guy!"; echo filter_var($string, FILTER_CALLBACK, array("options"=>"convertSpace")); ?>
以上代码的结果是这样的:
Peter is a great guy!
例子解释:
上面的例子把所有 "_" 转换成空格:
创建一个把 "_" 替换为空格的函数
调用 filter_var() 函数,它的参数是 FILTER_CALLBACK 过滤器以及包含我们的函数的数组。
本篇对过滤器进行了讲解,更多的学习资料清关注php中文网即可观看。
相关推荐:
The above is the detailed content of Related knowledge about PHP filter (Filter). For more information, please follow other related articles on the PHP Chinese website!
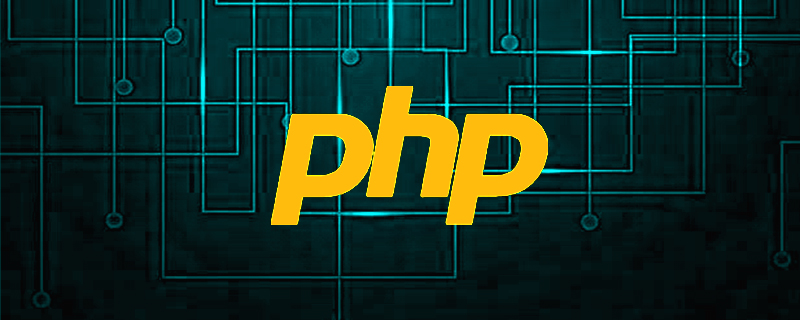
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
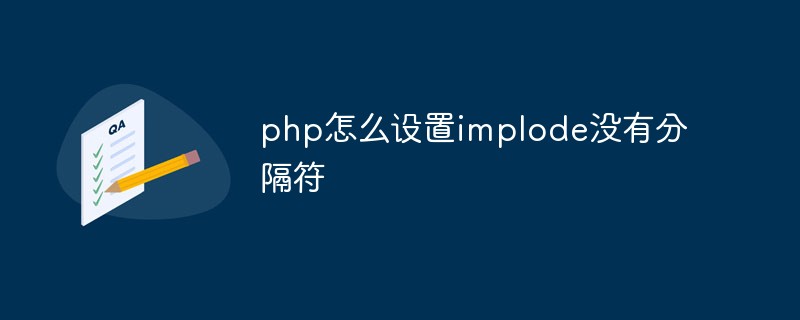
在PHP中,可以利用implode()函数的第一个参数来设置没有分隔符,该函数的第一个参数用于规定数组元素之间放置的内容,默认是空字符串,也可将第一个参数设置为空,语法为“implode(数组)”或者“implode("",数组)”。
![解决“[Vue warn]: Failed to resolve filter”错误的方法](https://img.php.cn/upload/article/000/887/227/169243040583797.jpg)
解决“[Vuewarn]:Failedtoresolvefilter”错误的方法在使用Vue进行开发的过程中,我们有时候会遇到一个错误提示:“[Vuewarn]:Failedtoresolvefilter”。这个错误提示通常出现在我们在模板中使用了一个未定义的过滤器的情况下。本文将介绍如何解决这个错误并给出相应的代码示例。当我们在Vue的
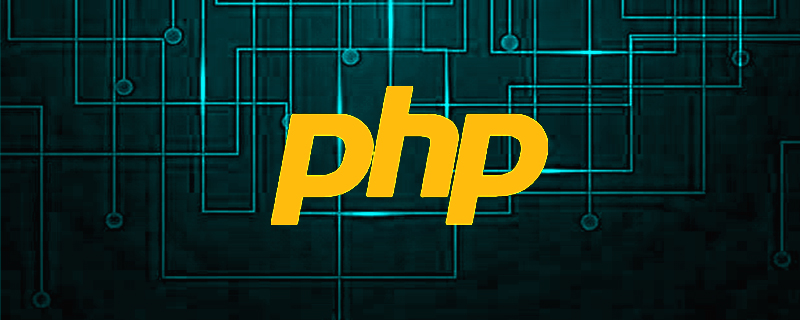
去除方法:1、使用substr_replace()函数将首位数字替换为空字符串即可,语法“substr_replace($num,"",0,1)”;2、用substr截取从第二位数字开始的全部字符即可,语法“substr($num,1)”。
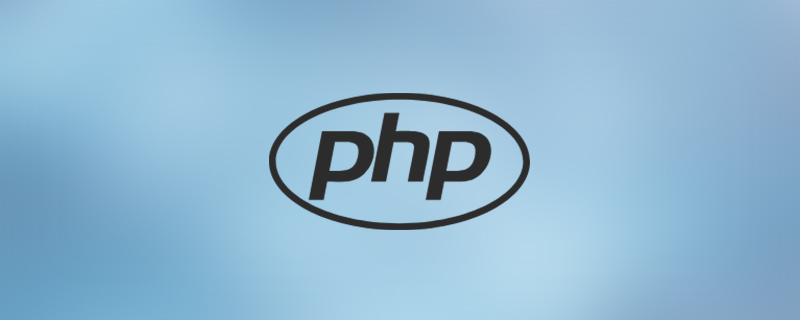
php有操作时间的方法。php中提供了丰富的日期时间处理方法:1、date(),格式化本地日期和时间;2、mktime(),返回日期的时间戳;3、idate(),格式化本地时间为整数;4、strtotime(),将时间字符串转为时间戳等等。
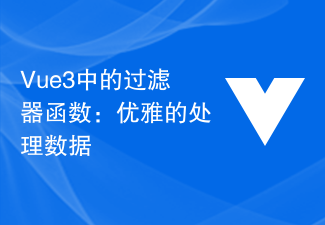
Vue3中的过滤器函数:优雅的处理数据Vue是一个流行的JavaScript框架,拥有庞大的社区和强大的插件系统。在Vue中,过滤器函数是一种非常实用的工具,允许我们在模板中对数据进行处理和格式化。Vue3中的过滤器函数有了一些改变,在这篇文章中,我们将深入探讨Vue3中的过滤器函数,学习如何使用它们优雅地处理数据。什么是过滤器函数?在Vue中,过滤器函数是
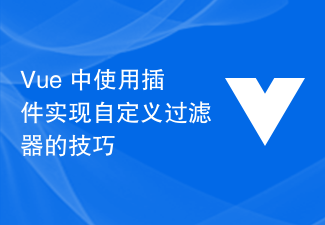
Vue中使用插件实现自定义过滤器的技巧Vue.js提供了一种方便的方式来处理视图数据过滤的需求,即过滤器(Filter)。过滤器主要负责将视图中的数据进行格式化和处理,使数据更加直观和易于理解。Vue内置了一些常用的过滤器,例如日期格式化、货币格式化等,同时也支持自定义过滤器。本文将介绍如何使用Vue插件实现自定义过滤器的技巧,并提供一些实用的过滤
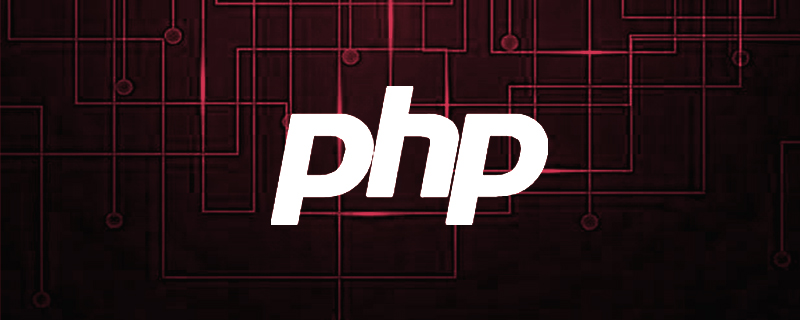
增加元素的方法:1、使用“array_unshift(数组,数组元素)”语句,在数组的开头添加元素;2、使用“array_push(数组,数组元素)”语句,在数组的末尾添加元素;3、用“array_pad(数组,数组长度+1,元素)”语句。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

SublimeText3 Linux new version
SublimeText3 Linux latest version
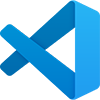
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Atom editor mac version download
The most popular open source editor
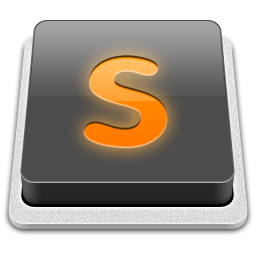
SublimeText3 Mac version
God-level code editing software (SublimeText3)
