How to create a mysql table through php. This article will explain in detail how to create a mysql table through php.
Creating a MySQL table using MySQLi and PDO
The CREATE TABLE statement is used to create a MySQL table.
Before creating the table, we need to use use myDB to select the database to operate:
use myDB;
We will create a table named "MyGuests" Table, with 5 columns: "id", "firstname", "lastname", "email" and "reg_date":
CREATE TABLE MyGuests ( id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, firstname VARCHAR(30) NOT NULL, lastname VARCHAR(30) NOT NULL, email VARCHAR(50), reg_date TIMESTAMP )
Data typeSpecifies what type of data the column can store. For complete data types please refer to our Data Types Reference Manual.
After setting the data type, you can specify the properties of other options for each column:
NOT NULL - Each row must contain a value (cannot be empty), the null value is not Allowed.
DEFAULT value - Set the default value
UNSIGNED - Use unsigned numeric type, 0 and positive numbers
AUTO INCREMENT - Set the value of the MySQL field every time when adding a record Automatically increase 1 times
PRIMARY KEY - Set the unique identification of each record in the data table. Typically the column's PRIMARY KEY is set to the ID value, used with AUTO_INCREMENT.
Each table should have a primary key (this column is the "id" column), and the primary key must contain a unique value.
The following example shows how to create a table in PHP:
Example (MySQLi - Object-oriented)
<?php$servername = "localhost";$username = "username";$password = "password";$dbname = "myDB";// 创建连接$conn = new mysqli($servername, $username, $password, $dbname);// 检测连接if ($conn->connect_error) { die("连接失败: " . $conn->connect_error);} // 使用 sql 创建数据表$sql = "CREATE TABLE MyGuests (id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, firstname VARCHAR(30) NOT NULL,lastname VARCHAR(30) NOT NULL,email VARCHAR(50),reg_date TIMESTAMP)";if ($conn->query($sql) === TRUE) { echo "Table MyGuests created successfully";} else { echo "创建数据表错误: " . $conn->error;}$conn->close();?> 实例 (MySQLi - 面向过程) <?php$servername = "localhost";$username = "username";$password = "password";$dbname = "myDB";// 创建连接$conn = mysqli_connect($servername, $username, $password, $dbname);// 检测连接if (!$conn) { die("连接失败: " . mysqli_connect_error());}// 使用 sql 创建数据表$sql = "CREATE TABLE MyGuests (id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, firstname VARCHAR(30) NOT NULL,lastname VARCHAR(30) NOT NULL,email VARCHAR(50),reg_date TIMESTAMP)";if (mysqli_query($conn, $sql)) { echo "数据表 MyGuests 创建成功";} else { echo "创建数据表错误: " . mysqli_error($conn);}mysqli_close($conn);?> 实例 (PDO) <?php$servername = "localhost";$username = "username";$password = "password";$dbname = "myDBPDO";try { $conn = new PDO("mysql:host=$servername;dbname=$dbname", $username, $password); // 设置 PDO 错误模式,用于抛出异常 $conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // 使用 sql 创建数据表 $sql = "CREATE TABLE MyGuests ( id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY, firstname VARCHAR(30) NOT NULL, lastname VARCHAR(30) NOT NULL, email VARCHAR(50), reg_date TIMESTAMP )"; // 使用 exec() ,没有结果返回 $conn->exec($sql); echo "数据表 MyGuests 创建成功";}catch(PDOException $e){ echo $sql . "<br>" . $e->getMessage();}$conn = null;?>
This article explains PHP in detail For the operation of creating a data table, please pay attention to the php Chinese website for more learning materials.
Related recommendations:
How to create a database through PHP MySQL
PHP connection to MySQL related knowledge and operations
Introduction to PHP MySQL (database related knowledge)
The above is the detailed content of How to create a MySQL table through PHP. For more information, please follow other related articles on the PHP Chinese website!
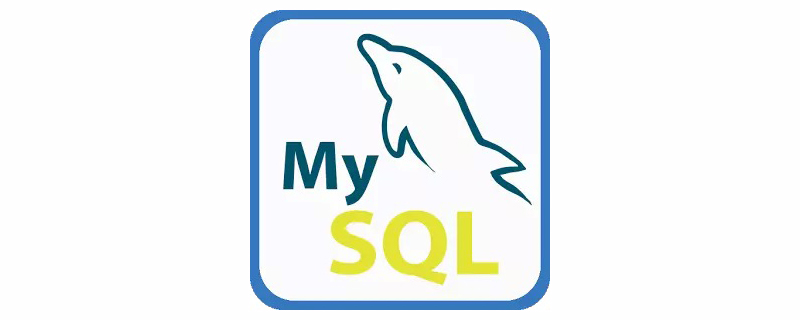
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于架构原理的相关内容,MySQL Server架构自顶向下大致可以分网络连接层、服务层、存储引擎层和系统文件层,下面一起来看一下,希望对大家有帮助。
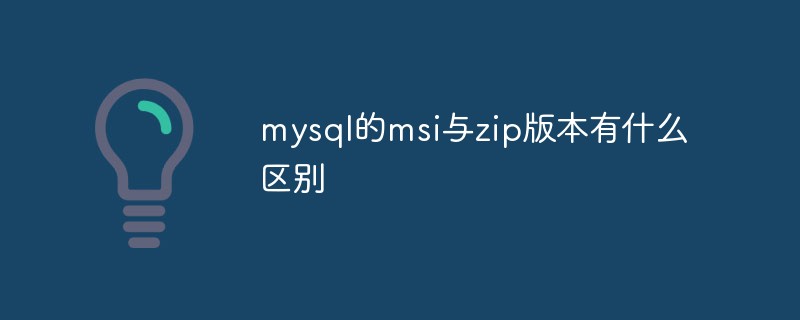
mysql的msi与zip版本的区别:1、zip包含的安装程序是一种主动安装,而msi包含的是被installer所用的安装文件以提交请求的方式安装;2、zip是一种数据压缩和文档存储的文件格式,msi是微软格式的安装包。
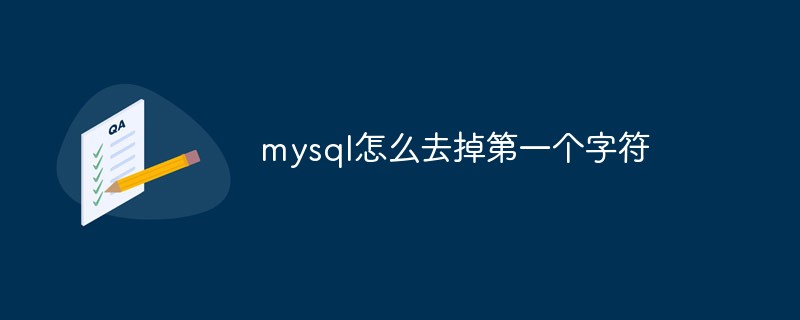
方法:1、利用right函数,语法为“update 表名 set 指定字段 = right(指定字段, length(指定字段)-1)...”;2、利用substring函数,语法为“select substring(指定字段,2)..”。
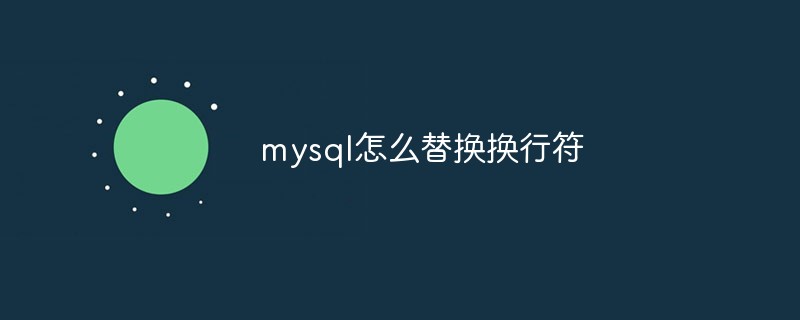
在mysql中,可以利用char()和REPLACE()函数来替换换行符;REPLACE()函数可以用新字符串替换列中的换行符,而换行符可使用“char(13)”来表示,语法为“replace(字段名,char(13),'新字符串') ”。
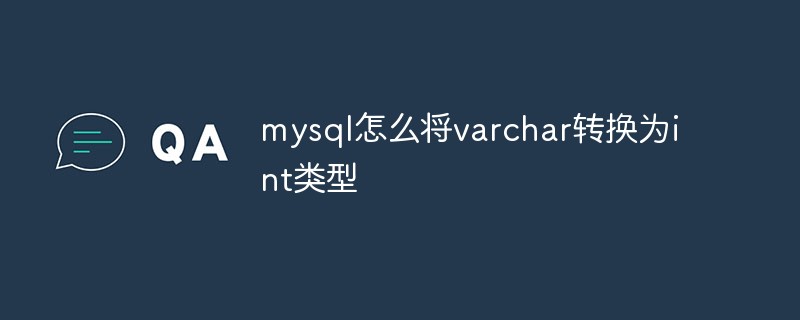
转换方法:1、利用cast函数,语法“select * from 表名 order by cast(字段名 as SIGNED)”;2、利用“select * from 表名 order by CONVERT(字段名,SIGNED)”语句。
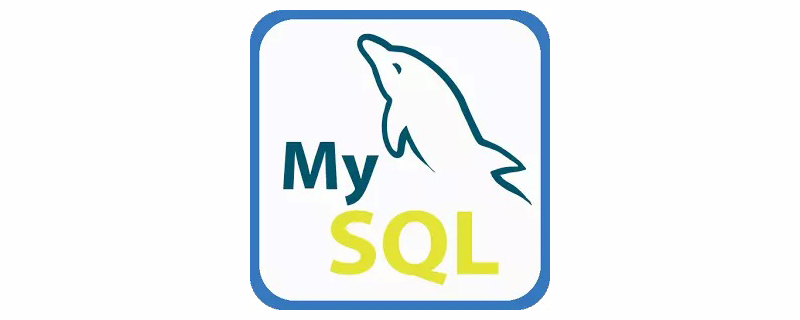
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了关于MySQL复制技术的相关问题,包括了异步复制、半同步复制等等内容,下面一起来看一下,希望对大家有帮助。
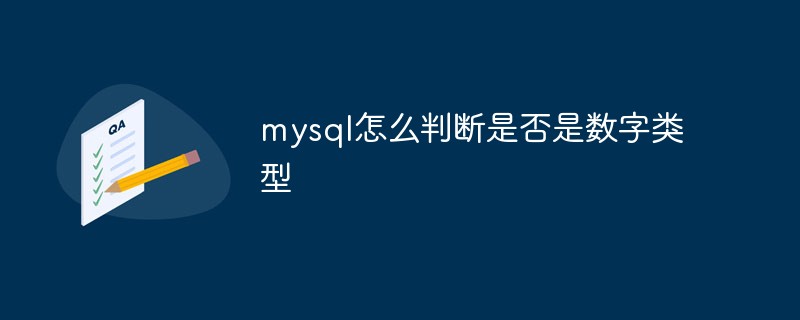
在mysql中,可以利用REGEXP运算符判断数据是否是数字类型,语法为“String REGEXP '[^0-9.]'”;该运算符是正则表达式的缩写,若数据字符中含有数字时,返回的结果是true,反之返回的结果是false。
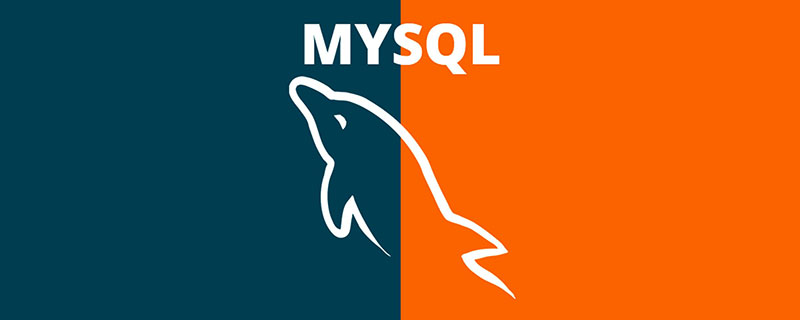
本篇文章给大家带来了关于mysql的相关知识,其中主要介绍了mysql高级篇的一些问题,包括了索引是什么、索引底层实现等等问题,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
