


Detailed explanation of the case of simple pop-up window function implemented by Vue component
This time I will bring you a detailed explanation of the case of Vue component implementing simple pop-up window function. What are the precautions for Vue component to implement simple pop-up window function. The following is a practical case, let's take a look.
I have been using the element-ui framework recently and used the Dialog dialog component. The effect achieved is roughly the same as a pop-up component I made in my previous mobile project. Then I wanted to share with you the implementation method of this pop-up window component. The following article will guide you through the implementation of a pop-up window component.
The main content of this article will involve the implementation of pop-up window masks, the use of slot slots, props and $emit parameters, and the specific component codes are also uploaded. If you like it, you can like/follow and support it. I hope everyone can gain something from reading this article.
The final effect of the component
- First build the html and css styles, mask layer and content layer of the component.
- Customize the pop-up window content: The pop-up window component accepts the pop-up window content passed from the parent component through the slot slot.
- Customized pop-up window style: The pop-up window component receives the pop-up window width, up, down, left and right positions passed from the parent component through props.
- Component switch: The props passed in through the parent component control the display and hiding of the component. When the child component is closed, the event $emit triggers the parent component to change the value.
<template> <p> <!--外层的遮罩 点击事件用来关闭弹窗,isShow控制弹窗显示 隐藏的props--> </p> <p></p> <!-- transition 这里可以加一些简单的动画效果 --> <transition> <!--style 通过props 控制内容的样式 --> <p> </p> <p> <!--弹窗头部 title--> <slot>提示信息</slot> </p> <p> <!--弹窗的内容--> <slot>弹窗内容</slot> </p> <!--弹窗关闭按钮--> <p> </p> <p></p> </transition> </template>The following is the main css in the component The style is fully annotated, and the masking effect is mainly achieved through z-index and
background. The css of the specific content can be set according to your own needs.
<style> // 最外层 设置position定位 .dialog { position: relative; color: #2e2c2d; font-size: 16px; } // 遮罩 设置背景层,z-index值要足够大确保能覆盖,高度 宽度设置满 做到全屏遮罩 .dialog-cover { background: rgba(0,0,0, 0.8); position: fixed; z-index: 200; top: 0; left: 0; width: 100%; height: 100%; } // 内容层 z-index要比遮罩大,否则会被遮盖, .dialog-content{ position: fixed; top: 35%; // 移动端使用felx布局 display: flex; flex-direction: column; justify-content: center; align-items: center; z-index: 300; } </style>2. Customize the pop-up content through slotIn this step, as long as you understand the role and usage of slot, there will be no problem. Single slot:
The above is a single slot It is called the default slot. The correct way to use the slot in the parent component is:
<my-component> <!--在my-component里面的所有内容片段都将插入到slot所在的DOM位置,并且会替换掉slot标签--> <!--这两个p标签,将替换整个slot标签里面的内容--> <p>这是一些初始内容</p> <p>这是更多的初始内容</p> </my-component>ps: If the child component contains a slot, the content of the p tag above will be discarded. Named slot: The so-called named slot is to assign a name attribute to the slot tag. Named slots can place different content fragments in the parent component in different places in the child component. Named slots can still have a default slot. Below you can take a look at how the pop-up component slot is used:
<p> <!--弹窗头部 title--> <slot>提示信息</slot> </p> <p> <!--弹窗的内容--> <slot>弹窗内容</slot> </p>How to use it in the parent component: Introduce the pop-up component into the component to be used, and register it through components components.
The method of using the pop-up component slot in the parent component is as follows.
<dialogcomponent> <p>插入到name为header的slot标签里面</p> <p> 这里是内容插入到子组件的slot的name为main里面,可以在父组件中添加class定义样式,事件类型等各种操作 </p> </dialogcomponent>That’s it for the introduction of slots used in components. The application of slots in pop-up components is a typical example. You can see that the slots are quite powerful, and the slots themselves It is not difficult to use. Have students fallen in love with slots? 3. Use props to control pop-up window visibility && customize pop-up window stylepsops is a way for parent components in Vue to transfer data to sub-components. Friends who are not familiar with it can check it out Check out the props document. Because pop-up components are introduced into other components, in order to be suitable for pop-ups in different component scenarios, pop-up components must have a certain degree of customizability, otherwise such components will not be able to be used at all. Meaningless, let's introduce how to use props, taking the pop-up component as an example: First, you need to define some characteristics of props in the passed-in component, such as verification.
Then bind the props data in the parent component.
<script> export default { props: { isShow: { //弹窗组件是否显示 默认不显示 type: Boolean, default: false, required:true, //必须 }, //下面这些属性会绑定到p上面 详情参照上面的html结构 // 如: :style="{top:topDistance+'%',width:widNum+'%'}" widNum:{ //内容宽度 type: Number, default:86.5 }, leftSite:{ // 左定位 type: Number, default:6.5 }, topDistance: { //top上边距 type: Number, default:35 }, pdt:{ //上padding type: Number, default:22 }, pdb:{ //下padding type: Number, default:47 } }, } </script>Used in parent component:
<dialogcomponent> </dialogcomponent>
ps:props传递数据不是双向绑定的,而是 单向数据流 ,父组件的数据变化时,也会传递到子组件中,这就意外着我们不应该在子组件中修改props。所以我们在关闭弹窗的时候就 需要通过 $emit 来修改父组件的数据 ,然后数据会自动传到子组件中。
现在基本上弹窗组件都已实现的差不多了,还差一个弹窗的关闭事件,这里就涉及到子组件往父组件传参了。
4. $emit 触发父组件事件修改数据,关闭弹窗
Vue中在子组件往父组件传参,很多都是通过 $emit 来触发父组件的事件来修改数据。
在子组件中,在点击关闭,或者遮罩层的时候触发下面这个方法:
methods: { closeMyself() { this.$emit("on-close"); //如果需要传参的话,可以在"on-close"后面再加参数,然后在父组件的函数里接收就可以了。 } }
父组件中的写法:
<dialogcomponent> </dialogcomponent> //"on-close是监听子组件的时间有没有触发,触发的时候执行closeDialog函数 methods:{ closeDialog(){ // this.status.isShowPublish=false; //把绑定的弹窗数组 设为false即可关闭弹窗 }, }
可以用弹窗组件实现下列这种信息展示,或者事件交互:
弹窗组件代码
相信看了本文案例你已经掌握了方法,更多精彩请关注php中文网其它相关文章!
推荐阅读:
The above is the detailed content of Detailed explanation of the case of simple pop-up window function implemented by Vue component. For more information, please follow other related articles on the PHP Chinese website!
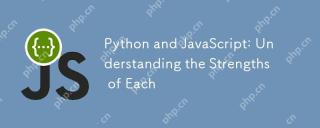
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
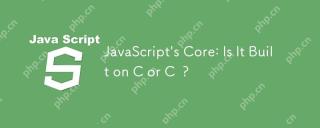
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
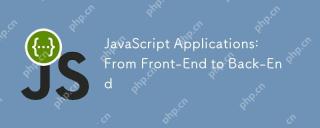
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
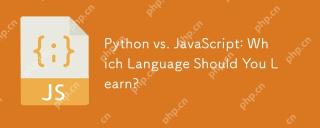
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
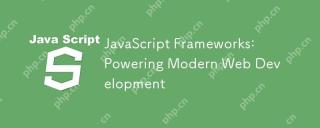
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
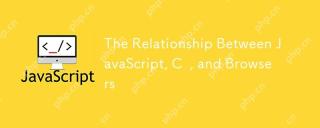
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
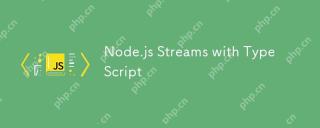
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
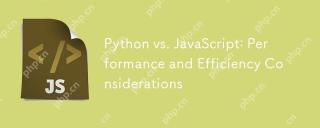
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Notepad++7.3.1
Easy-to-use and free code editor
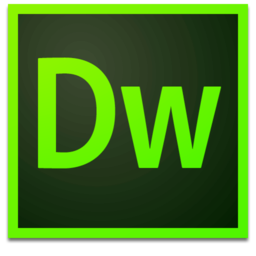
Dreamweaver Mac version
Visual web development tools
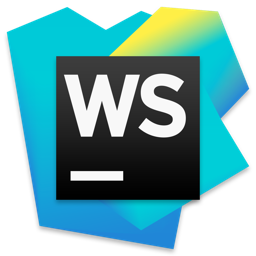
WebStorm Mac version
Useful JavaScript development tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
