This article mainly introduces the basic algorithm collection of PHP, which has certain reference value. Now I share it with everyone. Friends in need can refer to it
Binary search (searching for an element in an array)
function bin_sch($array, $low, $high, $k){ if ( $low <= $high){ $mid = intval(($low+$high)/2 ); if ($array[$mid] == $k){ return $mid; }elseif ( $k < $array[$mid]){ return bin_sch($array, $low, $mid-1, $k); }else{ return bin_sch($array, $mid+ 1, $high, $k); } } return -1; }
This method is for index arrays and the value is sorted from small to large
Associative arrays are not applicable, the array sorting method is inconsistent or there is no sorting, please modify the if condition accordingly to increase the sorting Wait
Sequential search (search for an element in the array)
function seq_sch($array, $n, $k){ $array[$n] = $k; for($i=0; $i<$n; $i++){ if( $array[$i]==$k){ break; } } if ($i<$n){ return $i; }else{ return -1; } } 此方法适用于索引数组并且$n = count($array);
Simplified enhanced version
function seq_sch($array, $k){ $y = $m = 'no'; foreach($array as $i => $v){ if($v == $k){ if($i == 'no'){$m = 'yes'}//防止key = no $y = $i; break; } } if ($y != 'no' || $m == 'yes'){ return $y; }else{ return -1; } } 此方法适用于所有一维数组
Deletion of linear tables (implemented in the array)
function delete_array_element($array , $i){ $len = count($array); for ($j=$i; $j<$len; $j++){ $array[$j] = $array [$j+1]; } array_pop ($array); return $array ; }
$i specifies the deletion parameter position
Bubble sort (array sort)
function bubble_sort($array){ $count = count( $array); if ($count <= 0 ) return false; for($i=0 ; $i<$count; $i ++){ for($j=$count-1 ; $j>$i; $j--){ if ($array[$j] < $array [$j-1]){ //引用第三变量进项数组交换 $tmp = $array[$j]; $array[$j] = $array[ $j-1]; $array [$j-1] = $tmp; } } } return $array; }
Quick sort (array sort)
function quick_sort($array ) { if (count($array) <= 1) return $array; $key = $array [0]; $left_arr = array(); $right_arr = array(); for ($i= 1; $i<count($array ); $i++){ if ($array[ $i] <= $key) $left_arr [] = $array[$i]; else $right_arr[] = $array[$i]; } $left_arr = quick_sort($left_arr); $right_arr = quick_sort($right_arr); return array_merge($left_arr , array($key), $right_arr); }
String length
function strlen ($str){ if ($str == '' ) return 0; $count = 0; while (1){ if ($str[$count] != NULL){ $count++; continue; }else{ break; } } return $count; }
while (1) 1 represents a constant expression, which will never equal 0. Therefore, the loop will continue to execute. Unless you set a break or similar statement to jump out of the loop, the loop will terminate.
-
$str[count] PHP is a weakly typed language. You can use subscripts to read the corresponding parameters at the corresponding position
Intercept substring
function substr($str, $start, $length=NULL){ if ($str== '' || $start>strlen($str)) return; if (($length!=NULL) && ($start>0) && ($length>strlen($str)-$start)) return; if (($length!=NULL) && ($start<0) && ($length>strlen($str )+$start)) return; if ($length == NULL) $length = (strlen($str) - $start); if ($start < 0){ for ($i=(strlen($str)+$start); $i<(strlen ($str)+$start+$length ); $i++) { $substr .= $str[$i]; } } if ($length > 0){ for ($i= $start; $i<($start+$length); $i++) { $substr .= $str[$i]; } } if ($length < 0){ for ($i =$start; $i<(strlen($str)+$length); $i++) { $substr .= $str[$i ]; } } return $substr;
}
String flip
function strrev($str){ if ($str == '') return 0 ; for ($i=(strlen($str)- 1); $i>=0; $i --){ $rev_str .= $str[$i ]; } return $rev_str; }
String comparison
function strcmp($s1, $s2){ if (strlen($s1) < strlen($s2)) return -1 ; if (strlen($s1) > strlen( $s2)) return 1; for ($i=0; $i<strlen($s1); $i++){ if ($s1[$i] == $s2[$i]){ continue; }else{ return false; } } return 0; }
Find string
function strstr($str, $substr){ $m = strlen($str); $n = strlen($substr); if ($m < $n) return false ; for($i=0; $i<=($m-$n+1); $i++){ $sub = substr($str, $i, $n); if (strcmp($sub, $substr) == 0) return $i; } return false ; }
strcmp(
substr) String comparison method If you don’t want to use the comparison method, please add a for loop
String replacement
function str_replace($substr, $newsubstr, $str){ $m = strlen($str); $n = strlen($substr); $x = strlen($newsubstr); if (strchr($str, $substr) == false) return false; $str_new = $str for ($i=0; $i<=($m-$n+1); $i++){ $i = strchr($str, $substr); $str = str_delete($str_new, $i, $n); $str = str_insert($str_new, $i, $newstr); } return $str_new; }
strchr() function searches for the first occurrence of a string in another string.
This function is an alias of the strstr() function.
Insert a string
function str_insert($str, $i , $substr) { for($j=0 ; $j<$i; $j++){ $startstr .= $str[$j]; } for ($j=$i; $j <strlen($str); $j++){ $laststr .= $str[$j ]; } $str = $startstr.$substr.$laststr; return $str ; }
Delete a string
function str_delete($str, $i, $j){ for ( $c=0; $c<$i; $c++){ $startstr .= $str [$c]; } for ($c=( $i+$j); $c<strlen ($str); $c++){ $laststr .= $str[$c]; } $str = $startstr.$laststr; return $str; }
Copy string
function strcpy($s1, $s2){ if (strlen($s1)==NULL || !isset($s2)) return; for ($i=0; $i<strlen($s1); $i++){ $s2[] = $s1[$i]; } return $s2; }
Connect string
function strcat($s1 ,$s2){ if (!isset($s1) || !isset( $s2)) return; $newstr = $s1 ; for($i=0; $i<strlen($s2); $i++){ $newstr .= $s2[$i]; } return $newstr; }
The above is the detailed content of A collection of basic algorithms for PHP. For more information, please follow other related articles on the PHP Chinese website!
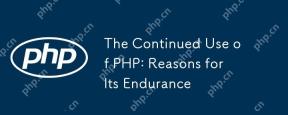
What’s still popular is the ease of use, flexibility and a strong ecosystem. 1) Ease of use and simple syntax make it the first choice for beginners. 2) Closely integrated with web development, excellent interaction with HTTP requests and database. 3) The huge ecosystem provides a wealth of tools and libraries. 4) Active community and open source nature adapts them to new needs and technology trends.
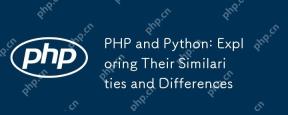
PHP and Python are both high-level programming languages that are widely used in web development, data processing and automation tasks. 1.PHP is often used to build dynamic websites and content management systems, while Python is often used to build web frameworks and data science. 2.PHP uses echo to output content, Python uses print. 3. Both support object-oriented programming, but the syntax and keywords are different. 4. PHP supports weak type conversion, while Python is more stringent. 5. PHP performance optimization includes using OPcache and asynchronous programming, while Python uses cProfile and asynchronous programming.
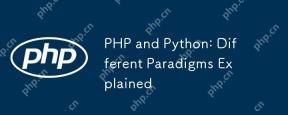
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
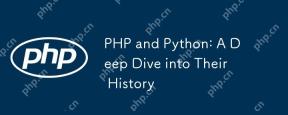
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
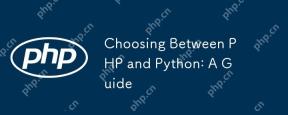
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
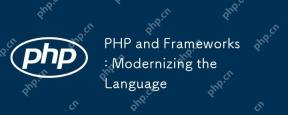
PHP remains important in the modernization process because it supports a large number of websites and applications and adapts to development needs through frameworks. 1.PHP7 improves performance and introduces new features. 2. Modern frameworks such as Laravel, Symfony and CodeIgniter simplify development and improve code quality. 3. Performance optimization and best practices further improve application efficiency.
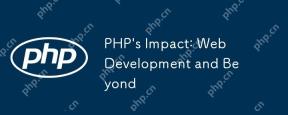
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
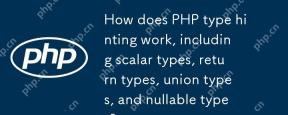
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.