


This article mainly introduces you to the relevant information about the dataclass decorator, a new feature of Python 3.7. The article introduces it in great detail through example code. It has certain reference learning value for everyone's study or work. Friends who need it Let’s learn together.
Preface
Python 3.7 will be released this summer, and there will be many new things in Python 3.7:
Various character set improvements
Delayed evaluation of comments
and support for dataclass
One of the most exciting new features is the dataclass decorator.
What is Data Class
Most Python developers have written many classes like the following:
class MyClass: def __init__(self, var_a, var_b): self.var_a = var_a self.var_b = var_b
dataclass can automatically generate methods for simple cases, for example, an __init__ that accepts these parameters and assigns them to itself, the previous small example can be rewritten as:
@dataclass class MyClass: var_a: str var_b: str
Let’s take an example to see how to use it
Star Wars API
You can use requests to obtain resources from the Star Wars API:
response = requests.get('https://swapi.co/api/films/1/') dictionary = response.json()
Let's take a look at the dictionary (simplified) results:
{ 'characters': ['https://swapi.co/api/people/1/',… ], 'created': '2014-12-10T14:23:31.880000Z', 'director': 'George Lucas', 'edited': '2015-04-11T09:46:52.774897Z', 'episode_id': 4, 'opening_crawl': 'It is a period of civil war.\r\n … ', 'planets': ['https://swapi.co/api/planets/2/', … ], 'producer': 'Gary Kurtz, Rick McCallum', 'release_date': '1977-05-25', 'species': ['https://swapi.co/api/species/5/',…], 'starships': ['https://swapi.co/api/starships/2/',…], 'title': 'A New Hope', 'url': 'https://swapi.co/api/films/1/', 'vehicles': ['https://swapi.co/api/vehicles/4/',…]
Encapsulating API
In order to properly encapsulate an API, we should create a user that can Object used in its application, therefore, in Python 3.6 an object is defined to contain requests responses to /films/endpoint:
class StarWarsMovie: def __init__(self, title: str, episode_id: int, opening_crawl: str, director: str, producer: str, release_date: datetime, characters: List[str], planets: List[str], starships: List[str], vehicles: List[str], species: List[str], created: datetime, edited: datetime, url: str ): self.title = title self.episode_id = episode_id self.opening_crawl= opening_crawl self.director = director self.producer = producer self.release_date = release_date self.characters = characters self.planets = planets self.starships = starships self.vehicles = vehicles self.species = species self.created = created self.edited = edited self.url = url if type(self.release_date) is str: self.release_date = dateutil.parser.parse(self.release_date) if type(self.created) is str: self.created = dateutil.parser.parse(self.created) if type(self.edited) is str: self.edited = dateutil.parser.parse(self.edited)
Careful Reader You may have noticed some duplicate code here.
This is a classic case of using the dataclass decorator, we need to create a class that is primarily used to hold data, with just a little validation, so let's see what we need to modify.
First, the data class automatically generates some dunder methods. If we do not specify any options for the data class decorator, the generated methods are: __init__, __eq__ and __repr__, if you have defined __repr__ But __str__ is not defined, and by default Python (not just data classes) will implement the output __str__ method that returns __repr__. Therefore, the four dunder methods can be implemented by simply changing the code to the following code:
@dataclass class StarWarsMovie: title: str episode_id: int opening_crawl: str director: str producer: str release_date: datetime characters: List[str] planets: List[str] starships: List[str] vehicles: List[str] species: List[str] created: datetime edited: datetime url: str
We removed the __init__ method to ensure that the data class decoration The converter can add the corresponding methods it generates. However, we lost some functionality in the process, our Python 3.6 constructor not only defines all the values, but also attempts to parse the date, how can we do this with a data class?
If we want to override __init__, we will lose the advantage of data class, so if we want to handle any additional functionality, we can use the new dunder method: __post_init__, let us see the __post_init__ method for our wrapper class What does it look like:
def __post_init__(self): if type(self.release_date) is str: self.release_date = dateutil.parser.parse(self.release_date) if type(self.created) is str: self.created = dateutil.parser.parse(self.created) if type(self.edited) is str: self.edited = dateutil.parser.parse(self.edited)
That’s it! We can use the data class decorator to implement our class in two-thirds of the code.
More good stuff
The data class can be further customized for the use case by using the decorator's options. The default options are:
@dataclass(init=True, repr=True, eq=True, order=False, unsafe_hash=False, frozen=False)
init determines whether to generate the __init__ dunder method
repr determines whether to generate the __repr__ dunder method
eq does the same for the __eq__ dunder method, which determines the behavior of the equality check (your_class_instance == another_instance)
order actually creates There are four dunder methods that determine the behavior of all checks for less than, and/or, and greater than, and if set to true, the list of objects can be sorted.
The last two options determine whether the object can be hashed, which is necessary if you want to use objects of your class as dictionary keys.
For more information, please refer to: PEP 557 -- Data Classes
##
The above is the detailed content of Detailed explanation of the dataclass decorator, a new feature of Python 3.7. For more information, please follow other related articles on the PHP Chinese website!
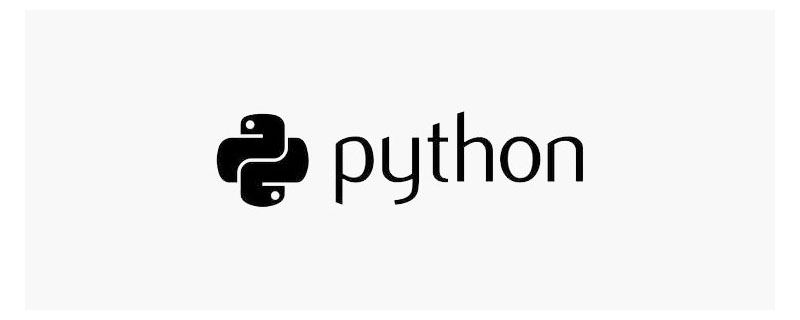
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
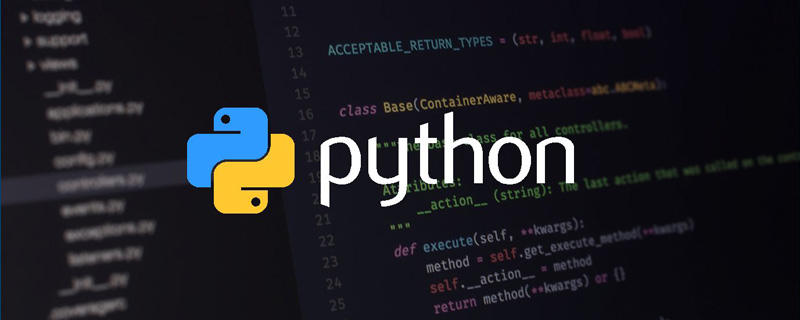
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
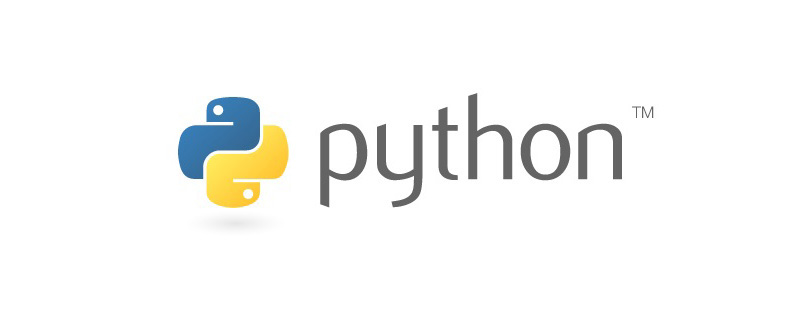
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
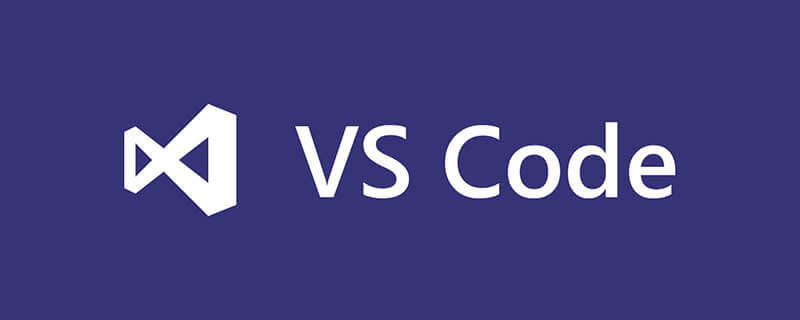
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
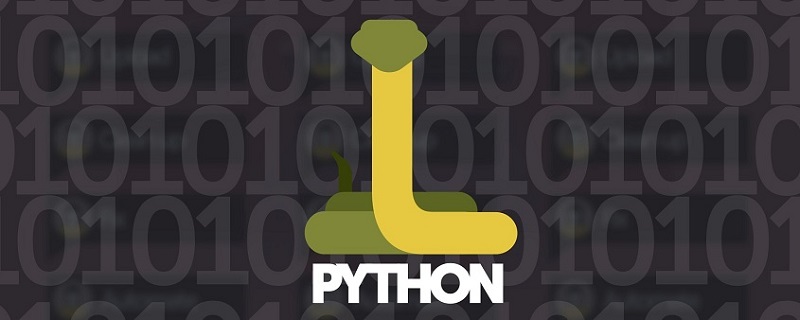
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
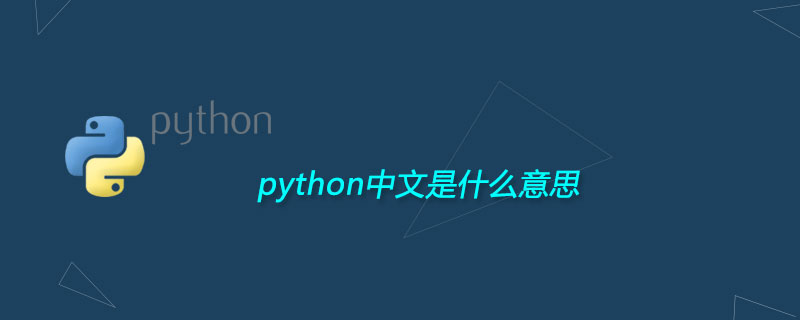
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。
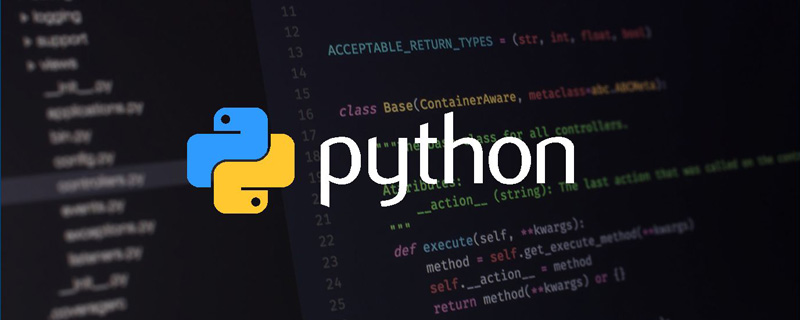
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
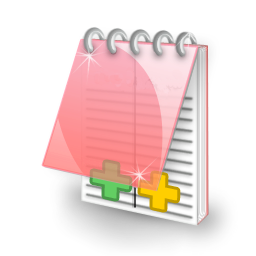
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
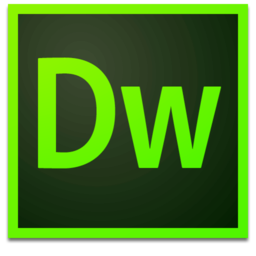
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
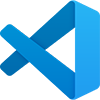
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
