This article mainly introduces the Python code to implement the Django framework. The editor thinks it is quite good. Now I will share it with you and give it a reference. Let’s follow the editor and take a look.
1. URLconf
When the user accesses the URL through the browser to request the website, he finds the corresponding function through the URL for execution, and Django The url configuration in is configured in settings.py in the folder with the same name as the project
1, configuration
(1), test1/settings.py configuration file
settings.py has specified the url configuration through ROOT_URLCONF by default, pointing to the urls.py file under test1:
url(r'^',include('应用名称.urls')), # 此处的urls指的是各自应用(booktest)中urls.py文件的名称,如果是urls.py,即为urls(2), Syntax 2: Definition, specify the corresponding relationship between URL and view function Create urls.py inside the respective applications and specify the corresponding relationship between the request address and the view. The format is as follows:
url(正则,'视图函数名称')Example: Create the url of the homepage in booktest/urls.py:
from django.conf.urls import url from booktest import views urlpatterns=[ url(r'^$',views.index), ]Note: It is recommended to use r in the regular part, which means that the string is not escaped, so you only need to write one \ in the regular expression. You cannot add a backslash at the beginning. It is recommended to add a backslash at the end, because the browser's URL is the same with or without adding \ at the end. 3. Obtain the parameter value carried in the urlThe requested url is regarded as an ordinary python string and matches request parameters that do not include domain name, get or post, such as: The request address is as follows: http://localhost:8080/detail/1?a=10 Among the above request addresses, the only strings that match the regular expression in the url() function are:
detail/
If you want to carry RESTFUL style parameters in the requested url, you need to use grouping in the regular expression of the url() function, that is, use the () sign, which is divided into: Positional parameters, keyword parameters. Note: Do not mix the two parameter methods. Only one parameter method can be used in a regular expression. (1) Positional parametersUse the () sign directly and pass it to the view through the positional parametersThe regular expression in the url() function is written as:# The corresponding function writing in ##url(r'^detail(\d+)/$',views.show_books),
views.py is:
def show_books(request, id): # 此处获取的id为1 return HttpResponse('show_books')
(2), keyword parameters
If keyword parameters are used, the regular expression in the url() function The expression grouping is to name each group
. For example, the accessed url is: http://localhost:8000/delete/1?a=10
The regular expression of the url() function The expression is written as:
url(r'^delete(?P<id1>\d+)/$',views.show_book),
The function in views.py is written as:
def show_arg(request,id1): return HttpResponse('show %s'%id1)
Note: If keyword parameters are used, Then the parameter name of the corresponding function in views.py must be consistent with the name of the group in the regular expression, otherwise an error will be reported. 2. View
1. What is a view
A view is a function in python. Views are generally defined in the "application/views.py" file, which is this file. Example "booktest/views.py".
The view must return an HttpResponse object or sub-object as a response. The response can be the HTML content of a web page, a redirect function, or a 404 error, etc.
The first parameter of the view must be an HttpRequest object. Other parameters may also include: keyword parameters or positional parameters (either one of the two)
Built-in error view
(1), built-in error view
Django has built-in views for handling HTTP errors. The main errors and views include:
404: page not found View
500: server error View
If you want to see the error view instead of debugging information, you need to modify the DEBUG item configuration information in the "test/settings.py" file
(2)、404错误及视图
将请求地址进行url匹配之后,没有找到匹配的正则表达式,则调用404视图,这个视图会调用404.html模版进行渲染,视图传递变量request_path给模版,表示导致错误的URL。
在templates中创建404.html
<html> <head> <title></title> </head> <body> 找不到了 <hr/> {{request_path}} </body> </html>
在浏览器中输入如下网址:
http://localhost:8000/test/
运行效果如下:
(3)、500错误及视图
在视图中代码运行报错将会发生500错误,调用内置错误视图,使用templates/500.html模版进行渲染。
三、HttpRequest对象
1、HttpRequest对象
服务器接收到HTTP请求后,会根据报文创建HttpRequest对象,这个对象不需要我们手动创建,直接使用服务器构建好的对象即可。视图函数中的第一个参数必须是HttpRequest对象,该对象类定义在django.http模块中。
2、属性
path:一个字符串,表示请求的页面的完整路径,不包含域名和参数部分。
method:一个字符串,表示请求使用的HTTP方法,常用值包括:'GET'、'POST'。
在浏览器中给出地址发出请求采用get方式,如超链接。
在浏览器中点击表单的提交按钮发起请求,如果表单的method设置为post则为post请求。
encoding:一个字符串,表示提交的数据的编码方式。
如果为None则表示使用浏览器的默认设置,一般为utf-8。
这个属性是可写的,可以通过修改它来修改访问表单数据使用的编码,接下来对属性的任何访问将使用新的encoding值。
GET:QueryDict类型对象,类似于字典,包含get请求方式的所有参数。
POST:QueryDict类型对象,类似于字典,包含post请求方式的所有参数。
FILES:一个类似于字典的对象,包含所有的上传文件。
COOKIES:一个标准的Python字典,包含所有的cookie,键和值都为字符串。
session:一个既可读又可写的类似于字典的对象,表示当前的会话,只有当Django 启用会话的支持时才可用,详细内容见"状态保持"。
The above is the detailed content of In-depth understanding of Python's Django framework. For more information, please follow other related articles on the PHP Chinese website!
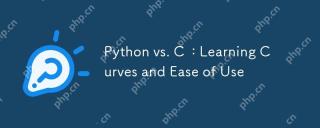
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
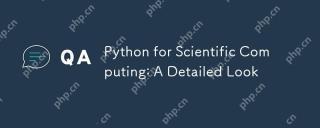
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
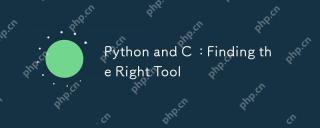
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
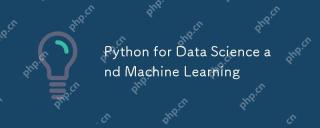
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
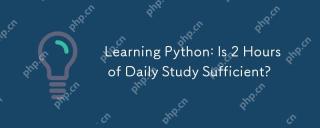
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
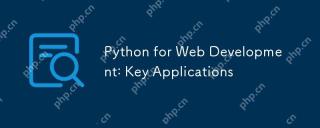
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
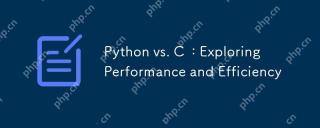
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
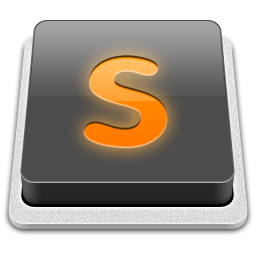
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.