This article mainly shares with you several ways to create objects in JS, hoping to help you.
1. Use native constructors to create objects of specific types
var person =new Object(); person.name="wangwu"; person.age="20"; person.sayName=function(){ alert(this.name); }
2. Use object literals
var person = { name:"wangwu", age:"20", sayName: function(){ alert(this.name); } }
Summary : Both methods can be used to create a single object, but they have obvious disadvantages. Using the same interface to create many objects will produce a lot of duplicate code.
3. Use the factory pattern
function createPerson(name,age){ var o=new Object(); o.name=name; o.age=age; o.sayName=function(){ alert(this.name); }; return o; } var person1=createPerson("wangwu",20);
Abstracts the process of creating specific objects, invents a function, and uses the function to encapsulate the creation of objects with a specific interface detail.
4. Constructor pattern
function Person(name,age){ this.name=name; this.age=age; this.sayName=function(){ alert(this.name); }; } var person1=new Person("wangwu",20);
Create a custom constructor to define the properties and methods of the custom object type.
5. Prototype pattern
function Person(){ } Person.prototype.name="wangwu"; Person.prototype.age=20; Person.prototype.sayName=function(){ alert(this.name); } var person1=new Person(); person1.sayName(); //wangwu
Every function we create has a prototype (prototype) attribute, which is a pointer pointing to an object, and this The purpose of an object is to contain properties and methods that can be shared by all instances of a specific type. The disadvantage of the prototype pattern is that it omits the step of passing initialization parameters to the constructor. As a result, all instances obtain the same attribute values by default; all attributes in the prototype are shared by many instances. For attributes containing reference type values, The problem is more prominent.
6. Combined use of constructor pattern and prototype pattern
function Person(name,age){ this.name=name; this.age=age; this.friends=["wangwu","zhangsan"]; } Person.prototype={ constructor:Person, sayName:function(){ alert(this.name); } } var person1=new Person("wangwu",20); var person2=new Person("zhangsan",23); person1.friends.push("lisi"); alert(person1.friends); //"wangwu,zhangsan,lisi" alert(person2.friends); //"wangwu,zhangsan"
7. Dynamic prototype pattern
function Person(name,age,job){ //属性 this.name=name; this.age=age; this.job=job; //方法 if(typeof this.sayName!="function"){ person.prototype.sayName=function(){ alert(this.name); }; } } var friend=new Person("wangwu",20); friends.sayName();
8. Parasitic constructor pattern
function Person(name,age){ var 0=new Object(); o.name="wangwu"; o.age=20; o.sayName=function(){ alert(this.name); }; return o; } var friend=new Person("wangwu",20); friend.sayName(); //"wangwu"
9. Safe constructor pattern
function Person(name,age,job){ //创建要返回的对象 var o=new Object(); //可以在这里定义私有变量和函数 //添加方法 o.sayName=function(){ alert(name); }; //返回对象 return o; } var friend=Person("wangwu",20); friend.sayName(); //"wangwu"
Related recommendations:
JS Create Object Summary of methods (sample code)
Several ways to create objects in JS
Summary of several common ways to create objects in JS (recommended) _js object-oriented
The above is the detailed content of Share several ways to create objects in JS. For more information, please follow other related articles on the PHP Chinese website!
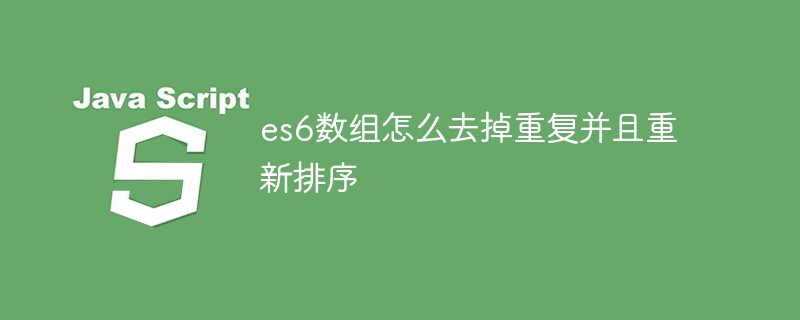
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
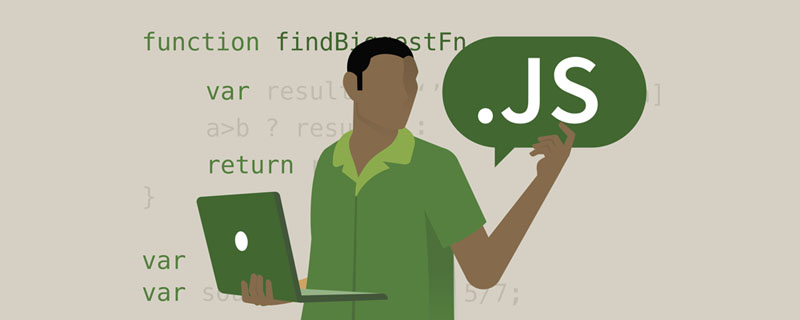
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
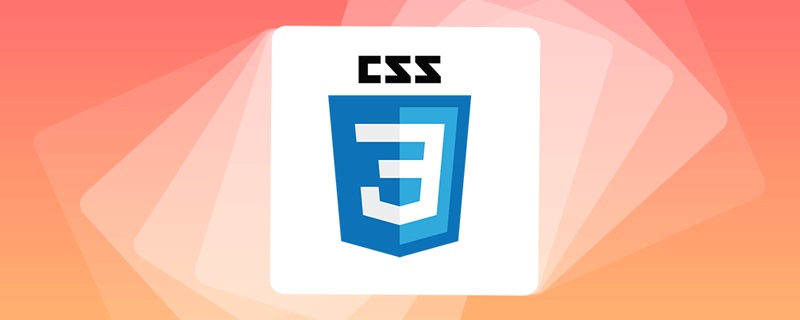
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
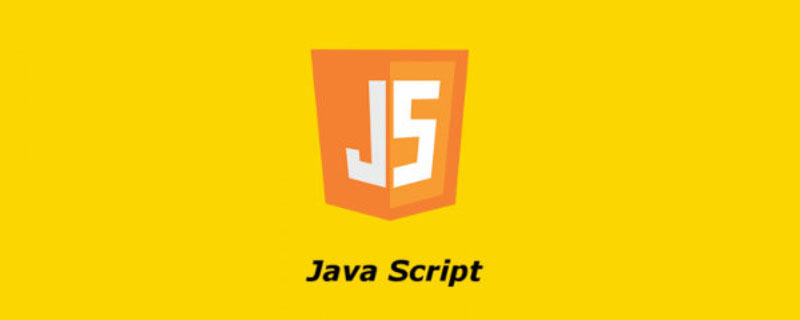
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
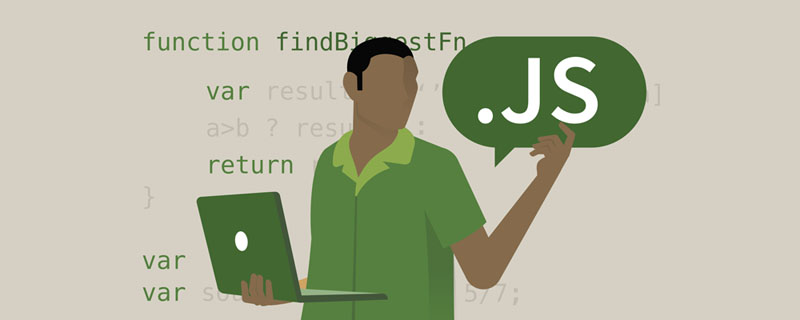
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
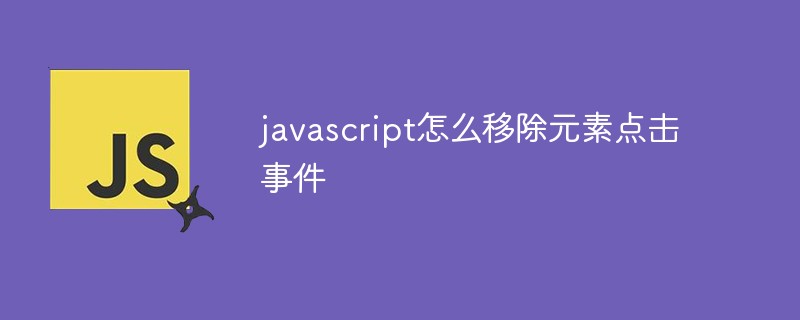
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
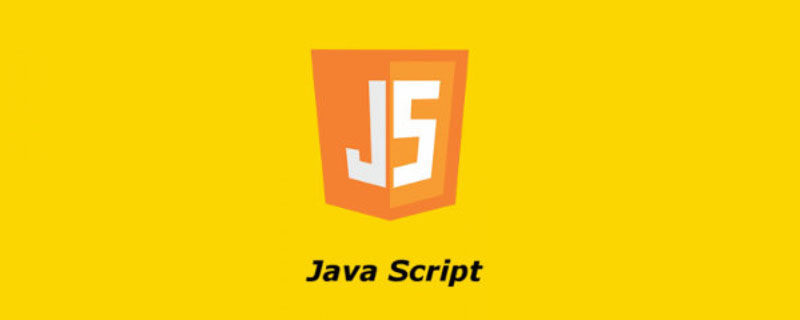
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
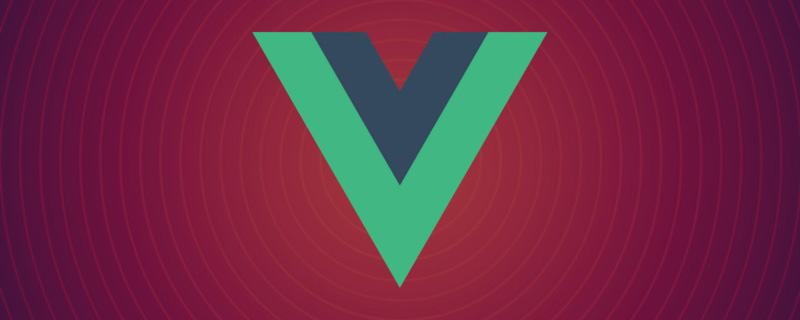
本篇文章整理了20+Vue面试题分享给大家,同时附上答案解析。有一定的参考价值,有需要的朋友可以参考一下,希望对大家有所帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
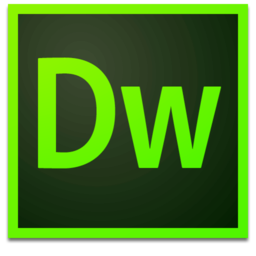
Dreamweaver Mac version
Visual web development tools
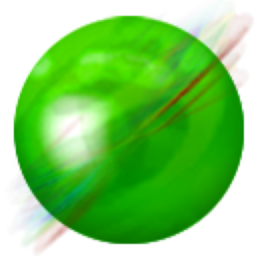
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
