Learn JavaScript design patterns (agent mode)_javascript skills
The proxy pattern is to provide a surrogate or placeholder for an object in order to control access to it
The use of the proxy pattern (personal understanding): In order to ensure the single responsibility (relative independence) of the current object, another object needs to be created to handle some logic before calling the current object to improve code efficiency, status judgment, etc.
The most commonly used proxy modes are virtual proxy and caching proxy
1. Virtual Agent
Virtual agents delay the creation and execution of some expensive objects until they are actually needed
Example: Virtual agent implements image preloading
// 图片加载函数 var myImage = (function(){ var imgNode = document.createElement("img"); document.body.appendChild(imgNode); return { setSrc: function(src) { imgNode.src = src; } } })(); // 引入代理对象 var proxyImage = (function(){ var img = new Image; img.onload = function(){ // 图片加载完成,正式加载图片 myImage.setSrc( this.src ); }; return { setSrc: function(src){ // 图片未被载入时,加载一张提示图片 myImage.setSrc("file://c:/loading.png"); img.src = src; } } })(); // 调用代理对象加载图片 proxyImage.setSrc( "http://images/qq.jpg");
Example: Virtual proxy merges HTTP requests
Suppose a function requires frequent network requests, which will cause considerable overhead. The solution is to use a proxy function to collect requests within a period of time and send them to the server at once.
For example: a file synchronization function, when we select a file, it will be synchronized to another backup server
// 文件同步函数 var synchronousFile = function( id ){ console.log( "开始同步文件,id为:" + id ); } // 使用代理合并请求 var proxySynchronousFile = (function(){ var cache = [], // 保存一段时间内需要同步的ID timer; // 定时器指针 return function( id ){ cache[cache.length] = id; if( timer ){ return; } timer = setTimeout( function(){ proxySynchronousFile( cache.join( "," ) ); // 2s 后向本体发送需要同步的ID集合 clearTimeout( timer ); // 清空定时器 timer = null; cache = []; // 晴空定时器 },2000 ); } })(); // 绑定点击事件 var checkbox = document.getElementsByTagName( "input" ); for(var i= 0, c; c = checkbox[i++]; ){ c.onclick = function(){ if( this.checked === true ){ // 使用代理进行文件同步 proxySynchronousFile( this.id ); } } }
2. Caching proxy
The caching proxy can provide temporary storage for some expensive operation results. In the next operation, if the parameters passed in are consistent with the previous operation, the previous operation results can be returned.
Example: Create caching proxies for multiplication, addition, etc.
// 计算乘积 var mult = function(){ var a = 1; for( var i = 0, l = arguments.length; i < l; i++){ a = a * arguments[i]; } return a; }; // 计算加和 var plus = function () { var a = 0; for( var i = 0, l = arguments.length; i < l; i++ ){ a += arguments[i]; } return a; }; // 创建缓存代理的工厂 var createProxyFactory = function( fn ){ var cache = {}; // 缓存 - 存放参数和计算后的值 return function(){ var args = Array.prototype.join.call(arguments, "-"); if( args in cache ){ // 判断出入的参数是否被计算过 console.log( "使用缓存代理" ); return cache[args]; } return cache[args] = fn.apply( this, arguments ); } }; // 创建代理 var proxyMult = createProxyFactory( mult ), proxyPlus = createProxyFactory( plus ); console.log( proxyMult( 1, 2, 3, 4 ) ); // 输出: 24 console.log( proxyMult( 1, 2, 3, 4 ) ); // 输出: 缓存代理 24 console.log( proxyPlus( 1, 2, 3, 4 ) ); // 输出: 10 console.log( proxyPlus( 1, 2, 3, 4 ) ); // 输出: 缓存代理 10
The above three examples introduce the JavaScript proxy mode in detail, and I hope it will be helpful to your learning.
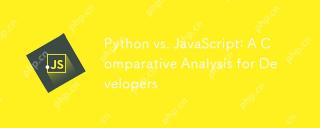
The main difference between Python and JavaScript is the type system and application scenarios. 1. Python uses dynamic types, suitable for scientific computing and data analysis. 2. JavaScript adopts weak types and is widely used in front-end and full-stack development. The two have their own advantages in asynchronous programming and performance optimization, and should be decided according to project requirements when choosing.
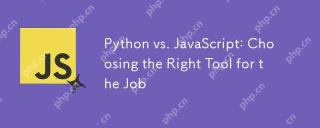
Whether to choose Python or JavaScript depends on the project type: 1) Choose Python for data science and automation tasks; 2) Choose JavaScript for front-end and full-stack development. Python is favored for its powerful library in data processing and automation, while JavaScript is indispensable for its advantages in web interaction and full-stack development.
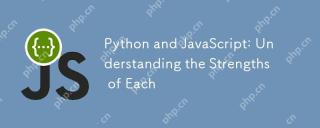
Python and JavaScript each have their own advantages, and the choice depends on project needs and personal preferences. 1. Python is easy to learn, with concise syntax, suitable for data science and back-end development, but has a slow execution speed. 2. JavaScript is everywhere in front-end development and has strong asynchronous programming capabilities. Node.js makes it suitable for full-stack development, but the syntax may be complex and error-prone.
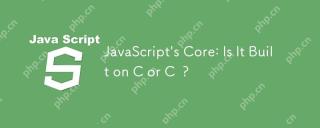
JavaScriptisnotbuiltonCorC ;it'saninterpretedlanguagethatrunsonenginesoftenwritteninC .1)JavaScriptwasdesignedasalightweight,interpretedlanguageforwebbrowsers.2)EnginesevolvedfromsimpleinterpreterstoJITcompilers,typicallyinC ,improvingperformance.
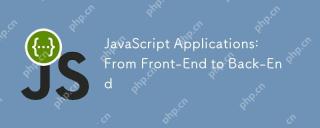
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
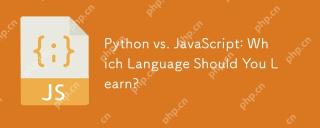
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
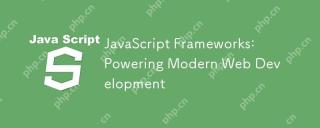
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
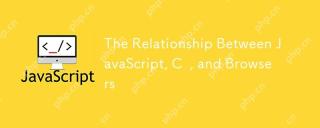
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
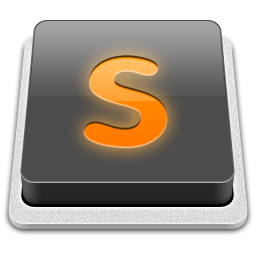
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
