This article mainly introduces Laravel's database migration method. The editor thinks it is quite good, so I will share it with you now and give it as a reference. Let’s follow the editor to take a look, I hope it can help everyone.
Generate migration
The --table and --create options can be used to specify the name of the data table, or a new data table that will be created when the migration is executed. These options need to be filled in the specified data table when pre-generating the migration file:
php artisan make:migration create_users_table php artisan make:migration create_users_table --create=users php artisan make:migration add_votes_to_users_table --table=users
Add fields
\database\migrations \2017_07_30_133748_create_users_table.php
<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateUsersTable extends Migration { /** * 运行数据库迁移 * * @return void */ public function up() { // Schema::create('users',function (Blueprint $table){ $table->increments('id')->comment('递增ID'); $table->string('email',60)->comment('会员Email'); $table->string('phone',20)->comment('会员手机号'); $table->string('username',60)->comment('用户名'); $table->string('password',32)->comment('用户密码'); $table->char('rank',10)->comment('会员等级'); $table->unsignedSmallInteger('sex')->comment('性别;0保密;1男;2女'); $table->unsignedSmallInteger('status')->comment('用户状态'); $table->ipAddress('last_ip')->default('0.0.0.0')->comment('最后一次登录IP'); $table->timeTz('last_login')->comment('最后一次登录时间'); $table->timestamps(); }); } /** * 回滚数据库迁移 * * @return void */ public function down() { // Schema::drop('users'); } }
To create a new data table, you can use the create method of the Schema facade. The create method receives two parameters: the first parameter is the name of the data table, and the second parameter is a closure. This closure will receive a Blueprint object used to define a new data table.
You can conveniently Use the hasTable and hasColumn methods to check whether the data table or field exists:
if (Schema::hasTable('users')) { // } if (Schema::hasColumn('users', 'email')) { // }
If you want to perform database structure operations in a non-default database connection, you can use connection method:
Schema::connection('foo')->create('users', function (Blueprint $table) { $table->increments('id'); });
You can set the engine attribute on the database structure constructor to set the storage engine of the data table:
Schema::create('users', function (Blueprint $table) { $table->engine = 'InnoDB'; $table->increments('id'); });
Renaming and deleting data tables
Schema::rename($from, $to);//重命名 //删除已存在的数据表 Schema::drop('users'); Schema::dropIfExists('users');
Creating fields
Schema::table('users', function (Blueprint $table) { $table->string('email'); });
命令 | 描述 |
---|---|
$table->bigIncrements('id'); | 递增 ID(主键),相当于「UNSIGNED BIG INTEGER」型态。 |
$table->bigInteger('votes'); | 相当于 BIGINT 型态。 |
$table->binary('data'); | 相当于 BLOB 型态。 |
$table->boolean('confirmed'); | 相当于 BOOLEAN 型态。 |
$table->char('name', 4); | 相当于 CHAR 型态,并带有长度。 |
$table->date('created_at'); | 相当于 DATE 型态 |
$table->dateTime('created_at'); | 相当于 DATETIME 型态。 |
$table->dateTimeTz('created_at'); | DATETIME (带时区) 形态 |
$table->decimal('amount', 5, 2); | 相当于 DECIMAL 型态,并带有精度与基数。 |
$table->double('column', 15, 8); | 相当于 DOUBLE 型态,总共有 15 位数,在小数点后面有 8 位数。 |
$table->enum('choices', ['foo', 'bar']); | 相当于 ENUM 型态。 |
$table->float('amount', 8, 2); | 相当于 FLOAT 型态,总共有 8 位数,在小数点后面有 2 位数。 |
$table->increments('id'); | 递增的 ID (主键),使用相当于「UNSIGNED INTEGER」的型态。 |
$table->integer('votes'); | 相当于 INTEGER 型态。 |
$table->ipAddress('visitor'); | 相当于 IP 地址形态。 |
$table->json('options'); | 相当于 JSON 型态。 |
$table->jsonb('options'); | 相当于 JSONB 型态。 |
$table->longText('description'); | 相当于 LONGTEXT 型态。 |
$table->macAddress('device'); | 相当于 MAC 地址形态。 |
$table->mediumIncrements('id'); | 递增 ID (主键) ,相当于「UNSIGNED MEDIUM INTEGER」型态。 |
$table->mediumInteger('numbers'); | 相当于 MEDIUMINT 型态。 |
$table->mediumText('description'); | 相当于 MEDIUMTEXT 型态。 |
$table->morphs('taggable'); | 加入整数 taggable_id 与字符串 taggable_type。 |
$table->nullableMorphs('taggable'); | 与 morphs() 字段相同,但允许为NULL。 |
$table->nullableTimestamps(); | 与 timestamps() 相同,但允许为 NULL。 |
$table->rememberToken(); | 加入 remember_token 并使用 VARCHAR(100) NULL。 |
$table->smallIncrements('id'); | 递增 ID (主键) ,相当于「UNSIGNED SMALL INTEGER」型态。 |
$table->smallInteger('votes'); | 相当于 SMALLINT 型态。 |
$table->softDeletes(); | 加入 deleted_at 字段用于软删除操作。 |
$table->string('email'); | 相当于 VARCHAR 型态。 |
$table->string('name', 100); | 相当于 VARCHAR 型态,并带有长度。 |
$table->text('description'); | 相当于 TEXT 型态。 |
$table->time('sunrise'); | 相当于 TIME 型态。 |
$table->timeTz('sunrise'); | 相当于 TIME (带时区) 形态。 |
$table->tinyInteger('numbers'); | 相当于 TINYINT 型态。 |
$table->timestamp('added_on'); | 相当于 TIMESTAMP 型态。 |
$table->timestampTz('added_on'); | 相当于 TIMESTAMP (带时区) 形态。 |
$table->timestamps(); | 加入 created_at 和 updated_at 字段。 |
$table->timestampsTz(); | 加入 created_at and updated_at (带时区) 字段,并允许为NULL。 |
$table->unsignedBigInteger('votes'); | 相当于 Unsigned BIGINT 型态。 |
$table->unsignedInteger('votes'); | 相当于 Unsigned INT 型态。 |
$table->unsignedMediumInteger('votes'); | 相当于 Unsigned MEDIUMINT 型态。 |
$table->unsignedSmallInteger('votes'); | 相当于 Unsigned SMALLINT 型态。 |
$table->unsignedTinyInteger('votes'); | 相当于 Unsigned TINYINT 型态。 |
$table->uuid('id'); | 相当于 UUID 型态。 |
字段修饰
Schema::table('users', function (Blueprint $table) { $table->string('email')->nullable(); });
Modifier | Description |
---|---|
->after('column') | 将此字段放置在其它字段「之后」(仅限 MySQL) |
->comment('my comment') | 增加注释 |
->default($value) | 为此字段指定「默认」值 |
->first() | 将此字段放置在数据表的「首位」(仅限 MySQL) |
->nullable() | 此字段允许写入 NULL 值 |
->storedAs($expression) | 创建一个存储的生成字段 (仅限 MySQL) |
->unsigned() | 设置 integer 字段为 UNSIGNED |
->virtualAs($expression) | 创建一个虚拟的生成字段 (仅限 MySQL) |
字段更新
Schema::table('users', function (Blueprint $table) { $table->string('phone',20)->change(); $table->string('username',60)->->nullable()->change(); });
重命名字段
Schema::table('users', function (Blueprint $table) { $table->renameColumn('from', 'to'); });
字段移除
Schema::table('users', function (Blueprint $table) { $table->dropColumn(['last_ip', 'last_login']); });
在使用字段更新,重命名字段,字段移除之前,请务必在你的 composer.json文件require键名中添加值。然后composer update进行更新或
composer require doctrine/dbal
创建索引
$table->string('email')->unique();
Command | Description |
---|---|
$table->primary('id'); | 加入主键。 |
$table->primary(['first', 'last']); | 加入复合键。 |
$table->unique('email'); | 加入唯一索引。 |
$table->unique('state', 'my_index_name'); | 自定义索引名称。 |
$table->unique(['first', 'last']); | 加入复合唯一键。 |
$table->index('state'); | 加入基本索引。 |
开启和关闭外键约束
Schema::enableForeignKeyConstraints(); Schema::disableForeignKeyConstraints();
运行迁移
php artisan migrate
在线上环境强制执行迁移
php artisan migrate --force
回滚迁移
若要回滚最后一次迁移,则可以使用 rollback 命令。此命令是对上一次执行的「批量」迁移回滚,其中可能包括多个迁移文件:
php artisan migrate:rollback
在 rollback 命令后加上 step 参数,你可以限制回滚迁移的个数。例如,下面的命令将会回滚最后的 5 个迁移。
php artisan migrate:rollback --step=5
migrate:reset 命令可以回滚应用程序中的所有迁移:
php artisan migrate:reset
使用单个命令来执行回滚或迁移
migrate:refresh 命令不仅会回滚数据库的所有迁移还会接着运行 migrate 命令。所以此命令可以有效的重新创建整个数据库:
php artisan migrate:refresh // 刷新数据库结构并执行数据填充 php artisan migrate:refresh --seed
使用 refresh 命令并加上 step 参数,你也可以限制执行回滚和再迁移的个数。比如,下面的命令会回滚并再迁移最后的 5 个迁移:
php artisan migrate:refresh --step=5
无法生成迁移文件
在 Laravel 项目中,由于测试,有时候用 PHP artisan make:migration create_xxx_table 创建数据库迁移。如果把创建的迁移文件 database/migrations/2017_07_30_133748_create_xxx_table.php 文件给删除了,再次执行 php artisan make:migration create_xxx_table 会报错:
复制代码 代码如下:
[ErrorException]
include(E:\laraver\vendor\composer/../../database/migrations/2017_07_30_133748_create_users_table.php): failed to open stream: No such file or directory
重新运行 composer update 又可以执行上面的命令了。
相关推荐:
The above is the detailed content of Detailed example of Laravel database migration method. For more information, please follow other related articles on the PHP Chinese website!
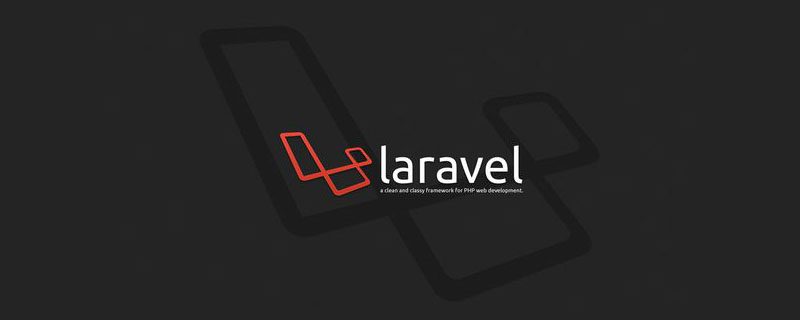
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于单点登录的相关问题,单点登录是指在多个应用系统中,用户只需要登录一次就可以访问所有相互信任的应用系统,下面一起来看一下,希望对大家有帮助。
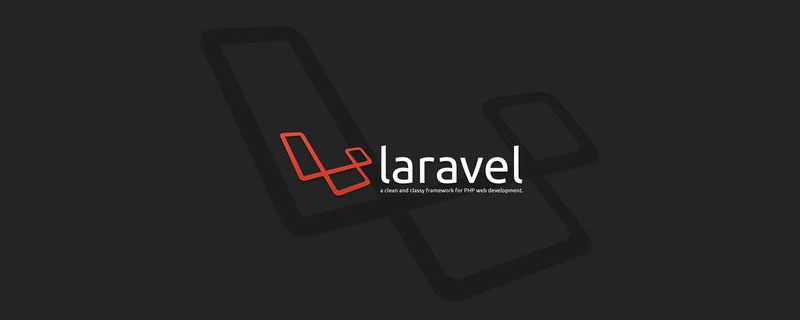
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于Laravel的生命周期相关问题,Laravel 的生命周期从public\index.php开始,从public\index.php结束,希望对大家有帮助。
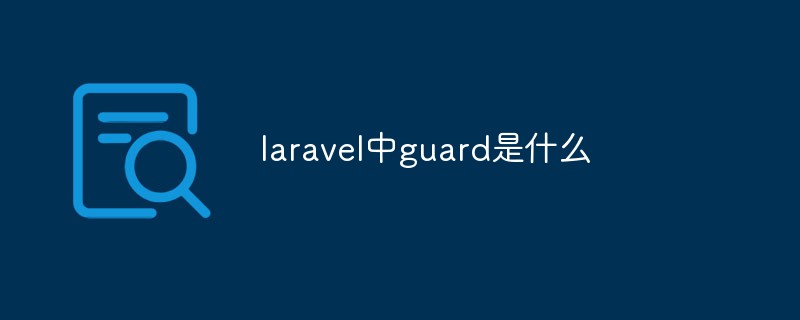
在laravel中,guard是一个用于用户认证的插件;guard的作用就是处理认证判断每一个请求,从数据库中读取数据和用户输入的对比,调用是否登录过或者允许通过的,并且Guard能非常灵活的构建一套自己的认证体系。
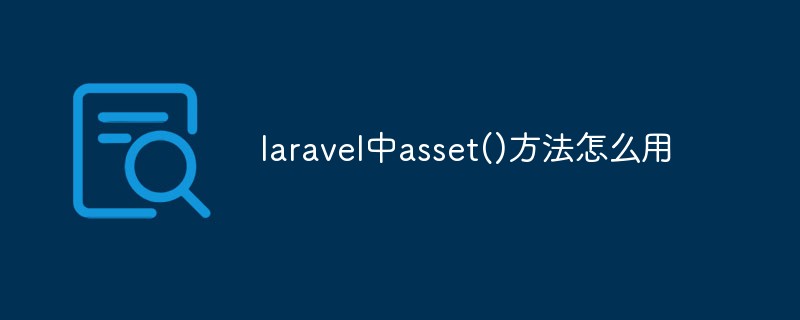
laravel中asset()方法的用法:1、用于引入静态文件,语法为“src="{{asset(‘需要引入的文件路径’)}}"”;2、用于给当前请求的scheme前端资源生成一个url,语法为“$url = asset('前端资源')”。
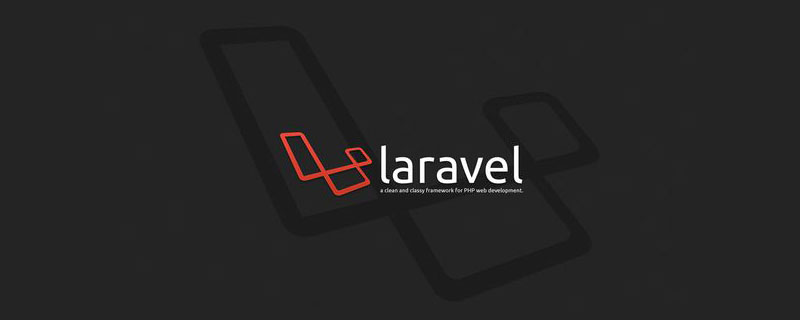
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于使用中间件记录用户请求日志的相关问题,包括了创建中间件、注册中间件、记录用户访问等等内容,下面一起来看一下,希望对大家有帮助。
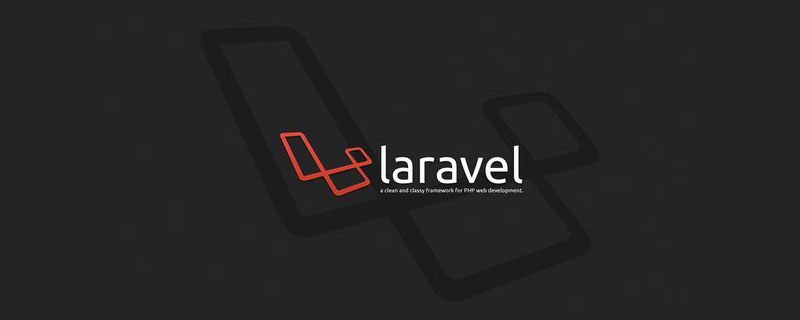
本篇文章给大家带来了关于laravel的相关知识,其中主要介绍了关于中间件的相关问题,包括了什么是中间件、自定义中间件等等,中间件为过滤进入应用的 HTTP 请求提供了一套便利的机制,下面一起来看一下,希望对大家有帮助。
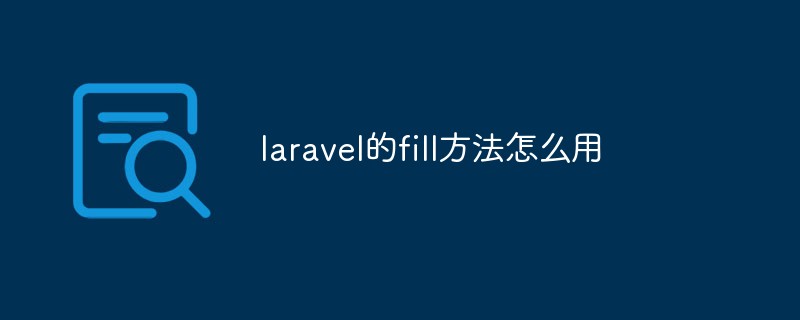
在laravel中,fill方法是一个给Eloquent实例赋值属性的方法,该方法可以理解为用于过滤前端传输过来的与模型中对应的多余字段;当调用该方法时,会先去检测当前Model的状态,根据fillable数组的设置,Model会处于不同的状态。
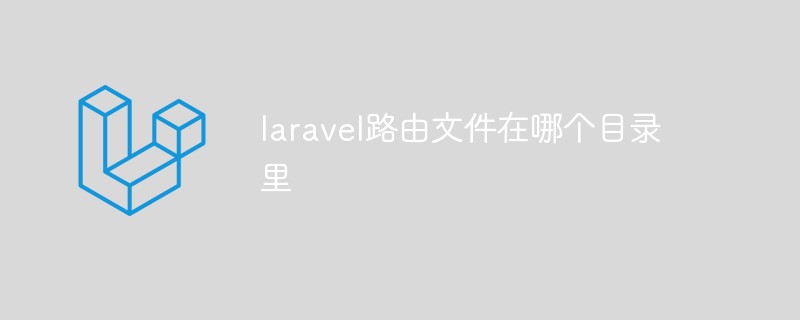
laravel路由文件在“routes”目录里。Laravel中所有的路由文件定义在routes目录下,它里面的内容会自动被框架加载;该目录下默认有四个路由文件用于给不同的入口使用:web.php、api.php、console.php等。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
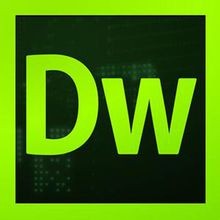
Dreamweaver CS6
Visual web development tools
