This article mainly introduces in detail the two ways to implement carousel charts in js, one is the constructor, and the other is the object-oriented way. It has certain reference value. Interested friends can refer to it. Hope it helps everyone.
1. Constructor
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title></title> <style type='text/css'> *{ margin:0; padding:0;} #wrap{ width:500px; height:360px; margin:100px auto; position:relative; } #pic{ width:500px; height:360px; position:relative; } #pic img{ width: 100%; height: 100%; position:absolute; top:0; left:0; display:none; } #tab{ width:105px; height:10px; position:absolute; bottom:10px; left:50%; margin-left:-50px; } #tab ul li{ width:10px; height:10px; margin:0 5px; background:#bbb; border-radius:100%; cursor:pointer; list-style:none; float:left; } #tab ul li.on{ background:#f60;} #btn p{ width:40px; height:40px; position:absolute; top:50%; margin-top:-20px; color:#fff; background:#999; background:rgba(0,0,0,.5); font-size:20px; font-weight:bold; font-family:'Microsoft yahei'; line-height:40px; text-align:center; cursor:pointer; } #btn p#left{ left:0;} #btn p#right{ right:0;} </style> </head> <body> <p id="wrap"> <p id="pic"> <img src="/static/imghwm/default1.png" data-src="img/1.jpg" class="lazy" alt="" /> <img src="/static/imghwm/default1.png" data-src="img/2.jpg" class="lazy" alt="" /> <img src="/static/imghwm/default1.png" data-src="img/3.jpg" class="lazy" alt="" /> <img src="/static/imghwm/default1.png" data-src="img/4.jpg" class="lazy" alt="" /> </p> <p id="tab"> <ul> <li></li> <li></li> <li></li> <li></li> </ul> </p> <p id="btn"> <p id='left'><</p> <p id='right'>></p> </p> </p> <script> var oWrap=document.getElementById('wrap') var picImg=document.getElementById('pic').getElementsByTagName('img'); var tabLi=document.getElementById('tab').getElementsByTagName('li'); var btnp=document.getElementById('btn').getElementsByTagName('p'); var index=0; var timer=null;//设置一个timer变量,让他的值为空 //初始化 picImg[0].style.display='block'; tabLi[0].className='on'; for(var i=0;i<tabLi.length;i++){ tabLi[i].index=i; tabLi[i].onclick=function(){ //不然要for循环清空 /* for(var i=0;i<tabLi.length;i++){ picImg[i].style.display='none'; tabLi[i].className=''; }*/ picImg[index].style.display='none'; //每个li都有index自定义属性 tabLi[index].className=''; index=this.index; picImg[index].style.display='block'; tabLi[index].className='on'; } }; for(var i=0;i<btnp.length;i++){ btnp[i].index=i; btnp[i].onselectstart=function(){ //禁止选择 return false; } btnp[i].onclick=function(){ picImg[index].style.display='none'; //每个li都有index自定义属性 tabLi[index].className=''; //index=this.index; if(this.index){ index++; //进来就加1,index就相当1%4 2%4 3%4 4%4 //if(index>tabLi.length){index=0} //index=index%arrUrl.length; 自己取模自己等于0 alert(3%3) == 0 index%=tabLi.length;//相当于当大于tabLi.length就等于0 }else{ index--; if(index<0)index=tabLi.length-1; } picImg[index].style.display='block'; tabLi[index].className='on'; } }; auto(); oWrap.onmouseover=function(){ clearInterval(timer) } oWrap.onmouseleave=function(){ auto(); } function auto(){ timer=setInterval(function(){ //一般都是向左轮播,index++ picImg[index].style.display='none'; tabLi[index].className=''; index++; index%=tabLi.length; picImg[index].style.display='block'; tabLi[index].className='on'; },2000) }; </script> </body> </html>
2. Object-oriented
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title></title> <style type='text/css'> *{ margin:0; padding:0;} #wrap{ width:500px; height:360px; margin:100px auto; position:relative; } #pic{ width:500px; height:360px; position:relative; } #pic img{ width: 100%; height: 100%; position:absolute; top:0; left:0; display:none; } #tab{ width:105px; height:10px; position:absolute; bottom:10px; left:50%; margin-left:-50px; } #tab ul li{ width:10px; height:10px; margin:0 5px; background:#bbb; border-radius:100%; cursor:pointer; list-style:none; float:left; } #tab ul li.on{ background:#f60;} #btn p{ width:40px; height:40px; position:absolute; top:50%; margin-top:-20px; color:#fff; background:#999; background:rgba(0,0,0,.5); font-size:20px; font-weight:bold; font-family:'Microsoft yahei'; line-height:40px; text-align:center; cursor:pointer; } #btn p#left{ left:0;} #btn p#right{ right:0;} </style> </head> <body> <p id="wrap"> <p id="pic"> <img src="/static/imghwm/default1.png" data-src="img/1.jpg" class="lazy" alt="" /> <img src="/static/imghwm/default1.png" data-src="img/2.jpg" class="lazy" alt="" /> <img src="/static/imghwm/default1.png" data-src="img/3.jpg" class="lazy" alt="" /> <img src="/static/imghwm/default1.png" data-src="img/4.jpg" class="lazy" alt="" /> </p> <p id="tab"> <ul> <li></li> <li></li> <li></li> <li></li> </ul> </p> <p id="btn"> <p id='left'><</p> <p id='right'>></p> </p> </p> <script> var oWrap=document.getElementById('wrap') var picImg=document.getElementById('pic').getElementsByTagName('img'); var tabLi=document.getElementById('tab').getElementsByTagName('li'); var btnp=document.getElementById('btn').getElementsByTagName('p'); function Banner(oWrap,picImg,tabLi,btnp){ this.wrap=oWrap this.list=picImg this.tab=tabLi this.btn=btnp this.index=0; //这些都必须是私有的,不然两个banner会一样 this.timer=null; this.length=this.tab.length; // this.init();//下面创建好,要在这里执行 } //初始化分类 Banner.prototype.init=function(){ //先把下面的分类 var This=this; //var 一个This变量把this存起来 this.list[0].style.display='block'; this.tab[0].className='on'; for(var i=0;i<this.length;i++){ this.tab[i].index=i; this.tab[i].onclick=function(){ //this.list[index].style.display='none'; 这里的this指向tab的this This.list[This.index].style.display='none'; This.tab[This.index].className=''; //index=this.index; This.index=this.index; This.list[This.index].style.display='block'; //This.tab[This.index].className='on'; this.className='on'; } }; for(var i=0;i<this.btn.length;i++){ this.btn[i].index=i; this.btn[i].onselectstart=function(){ return false; } this.btn[i].onclick=function(){ This.list[This.index].style.display='none'; This.tab[This.index].className=''; if(this.index){ This.index++; This.index%=This.length; }else{ This.index--; if(index<0)This.index=This.length-1; } This.list[This.index].style.display='block'; This.tab[This.index].className='on'; } } this.auto(); this.clear(); }; Banner.prototype.auto=function(){ var This=this; This.timer=setInterval(function(){ //一般都是向左轮播,index++ This.list[This.index].style.display='none'; This.tab[This.index].className=''; This.index++; This.index%=This.length; This.list[This.index].style.display='block'; This.tab[This.index].className='on'; },2000) }; Banner.prototype.clear=function(){ var This=this; this.wrap.onmouseover=function(){ clearInterval(This.timer) } this.wrap.onmouseleave=function(){ This.auto(); } }; var banner1=new Banner(oWrap,picImg,tabLi,btnp); banner1.init(); /* * init() * function init(){ for(var i=0;i<tabLi.length;i++){ tabLi[i].index=i; tabLi[i].onclick=function(){ picImg[index].style.display='none'; tabLi[index].className=''; index=this.index; picImg[index].style.display='block'; tabLi[index].className='on'; } }; } for(var i=0;i<btnp.length;i++){ btnp[i].index=i; btnp[i].onselectstart=function(){ return false; } btnp[i].onclick=function(){ picImg[index].style.display='none'; tabLi[index].className=''; if(this.index){ index++; index%=tabLi.length; }else{ index--; if(index<0)index=tabLi.length-1; } picImg[index].style.display='block'; tabLi[index].className='on'; } }; auto(); oWrap.onmouseover=function(){ clearInterval(timer) } oWrap.onmouseleave=function(){ auto(); } function auto(){ timer=setInterval(function(){ //一般都是向左轮播,index++ picImg[index].style.display='none'; tabLi[index].className=''; index++; index%=tabLi.length; picImg[index].style.display='block'; tabLi[index].className='on'; },2000) }; */ </script> </body> </html>
Related Recommended:
Explanation of the actual sliding focus carousel chart of encapsulated motion framework
js realizes the effect of finger sliding carousel chart on the mobile terminal
jquery version carousel effect and extend extension example sharing
The above is the detailed content of Two js ways to implement carousel images. For more information, please follow other related articles on the PHP Chinese website!
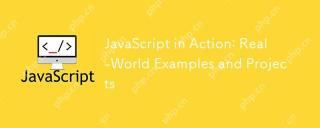
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
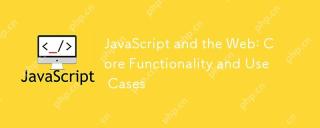
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
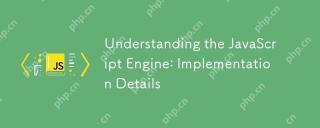
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
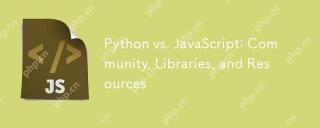
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
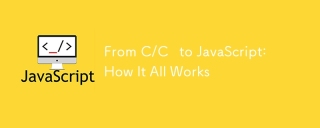
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
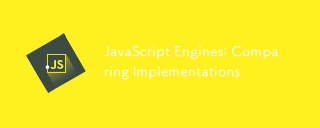
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
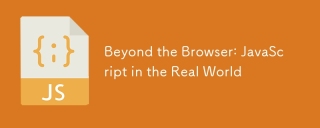
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
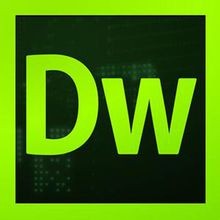
Dreamweaver CS6
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 Chinese version
Chinese version, very easy to use