

JS interception and splitting string methods: the difference between substring, substr, and slice
These three methods are all methods for extracting substrings from strings. Although they are commonly used, you have to read the documentation every time to prevent parameter transfer errors. The following is a detailed distinction between these three methods.
substring
String.substring(x,y):
x: required. A nonnegative integer that specifies the position in stringObject of the first character of the substring to be extracted.
y: Optional. A non-negative integer that is + 1 from the position in stringObject of the last character of the substring to be extracted.
/2个参数的情况"abcdefg".substring(1,4); //"bcd"//1个参数的情况:返回开始位置到字符串的结尾"abcdefg".substring(1); //"bcdefg"//如果x=y:返回一个空串(即长度为 0 的字符串)"abcdefg".substring(1,1); //""//如果x>y:先交换这两个参数然后同第一种情况"abcdefg".substring(4,1); //"bcd"//如果x<0或y<0;会把小于0的参数当作0处理(理论上不接受负数);"abcdefg".substring(1,-4); =》"abcdefg".substring(1,0); =》"abcdefg".substring(0,1); //"a"
The examples in this article describe the common methods of intercepting and splitting strings with JS. Share it with everyone for your reference, the details are as follows:
JS can use substring() or slice() to intercept strings
Function: | substring() |
Definition: | substring(start,end) represents the string from start to end, including the character at the start position But the characters at the end position are not included. |
Function: | String interception, for example, if you want to get "Minidx" from "MinidxSearchEngine", you need to use substring(0,6) |
例子:
var src="images/off_1.png"; alert(src.substring(7,10)); //弹出值为:off
函数:substr()
定义:substr(start,length)表示从start位置开始,截取length长度的字符串。
功能:字符串截取
例子:
var src="images/off_1.png"; alert(src.substr(7,3)); //弹出值为:off
函数:split()
功能:使用一个指定的分隔符把一个字符串分割存储到数组
例子:
str="jpg|bmp|gif|ico|png"; arr=theString.split("|"); //arr是一个包含字符值"jpg"、"bmp"、"gif"、"ico"和"png"的数组
函数:John()
功能:使用您选择的分隔符将一个数组合并为一个字符串
例子:
var delimitedString=myArray.join(delimiter); var myList=new Array("jpg","bmp","gif","ico","png"); var portableList=myList.join("|"); //结果是jpg|bmp|gif|ico|png
函数:indexOf()
功能:返回字符串中匹配子串的第一个字符的下标
var myString="JavaScript"; var w=myString.indexOf("v");w will be 2 var x=myString.indexOf("S");x will be 4 var y=myString.indexOf("Script");y will also be 4 var z=myString.indexOf("key");z will be -1
函数:lastIndexOf()
定义:lastIndexOf()方法返回从右向左出现某个字符或字符串的首个字符索引值(与indexOf相反)
功能:返回字符串索引值
var src="images/off_1.png"; alert(src.lastIndexOf('/')); alert(src.lastIndexOf('g')); //弹出值依次为:6,15
补充:substr 和 substring方法的区别
substr 方法
返回一个从指定位置开始的指定长度的子字符串。
stringvar.substr(start [, length ])
参数
stringvar 必选项。要提取子字符串的字符串文字或 String 对象。
start 必选项。所需的子字符串的起始位置。字符串中的第一个字符的索引为 0。
length可选项。在返回的子字符串中应包括的字符个数。
说明 如果 length 为 0 或负数,将返回一个空字符串。如果没有指定该参数,则子字符串将延续到 stringvar 的最后。
示例 下面的示例演示了substr 方法的用法。
function SubstrDemo(){ var s, ss; // 声明变量。 var s = "The rain in Spain falls mainly in the plain."; ss = s.substr(12, 5); // 获取子字符串。 return(ss); // 返回 "Spain"。 }
substring 方法
返回位于 String 对象中指定位置的子字符串。
strVariable.substring(start, end)
"String Literal".substring(start, end)
参数
start 指明子字符串的起始位置,该索引从 0 开始起算。
end 指明子字符串的结束位置,该索引从 0 开始起算。
说明 substring 方法将返回一个包含从 start 到最后(不包含 end )的子字符串的字符串。
substring 方法使用 start 和 end 两者中的较小值作为子字符串的起始点。例如, strvar.substring(0, 3) 和 strvar.substring(3, 0) 将返回相同的子字符串。
如果 start 或 end 为 NaN 或者负数,那么将其替换为0。
子字符串的长度等于 start 和 end 之差的绝对值。例如,在 strvar.substring(0, 3) 和 strvar.substring(3, 0) 返回的子字符串的的长度是 3。
示例
下面的示例演示了 substring 方法的用法。
function SubstringDemo(){ var ss; // 声明变量。 var s = "The rain in Spain falls mainly in the plain.."; ss = s.substring(12, 17); // 取子字符串。 return(ss); // 返回子字符串。 }
slice
String.slice(x,y):
x:必需。要抽取的片断的起始下标。如果是负数,则该参数规定的是从字符串的尾部开始算起的位置。也就是说,-1 指字符串的最后一个字符,-2 指倒数第二个字符,以此类推。
y:可选。要抽取的片段的结尾的下标+1。若未指定此参数,则要提取的子串包括 start 到原字符串结尾的字符串。如果该参数是负数,那么它规定的是从字符串的尾部开始算起的位置。
//2个参数的情况"abcdefg".slice(1,4); //"bcd"//1个参数的情况:返回开始位置到字符串的结尾"abcdefg".slice(1); //"bcdefg"//如果x=y:返回一个空串(即长度为 0 的字符串)"abcdefg".slice(1,1); //""//如果x>y:返回一个空串(即长度为 0 的字符串)"abcdefg".slice(4,1); //""//如果x*因为slice不能像substring一样当x
substr
String.substr(x,y):
x:必需。必需。要抽取的子串的起始下标。必须是数值。如果是负数,那么该参数声明从字符串的尾部开始算起的位置。也就是说,-1 指字符串中最后一个字符,-2 指倒数第二个字符,以此类推。
y:可选。子串中的字符长度。必须是数值。如果省略了该参数,那么返回从 stringObject 的开始位置到结尾的字串。
//In the case of 2 parameters "abcdefg".substr(1,4); //"bcde"//In the case of 1 parameter: return the starting position to the end of the string "abcdefg".substr(1) ; //"bcdefg"//If xImportant: ECMAscript does not standardize this method and therefore discourages its use.
Important: In IE 4, the value of parameter start is invalid. In this BUG, start specifies the position of the 0th character. This BUG has been corrected in later versions. 123
Summary
The first parameter of the three methods is the starting position, and the second parameter of substring and slice is the ending position (excluding this position), slice and substr can accept negative values but the second parameter of substr is the length.
Then the question is, how to get the last character of a string?
"abcdefg".substring(length-1);//g "abcdefg".slice(-1);//g "abcdefg".substr(-1);//g"abcdefg". charAt(length-1);//g 1234

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment
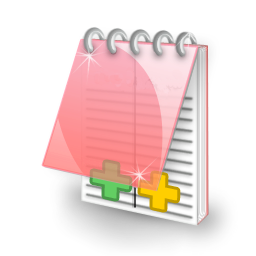
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.