This article mainly introduces the constructor pattern of JavaScript programming design pattern, briefly describes the concept and principle of the constructor pattern, and analyzes the definition and usage of the constructor pattern in the form of examples. Friends in need can refer to it. I hope Can help everyone.
The examples in this article describe the constructor pattern of JavaScript programming design patterns. Share it with everyone for your reference, the details are as follows:
In the classic OOP language, the constructor (also called the constructor) is a special method used to initialize objects. In JS, because everything is an object, object constructors are often mentioned.
The object constructor is used to create an object of a specified type (Class) and can accept parameters to initialize the properties and methods of the object.
Object creation
In JS, there are three commonly used methods for creating objects:
//1, 推荐使用 var newObject = {}; //2, var newObject = Object.create( null ); //3, 不推荐 var newObject = new Object();
However, this only creates three empty objects without any properties and methods. We can set properties and methods for objects through the following four methods.
// ECMAScript 3 兼容的方式 // 1. 常规对象定义方式 //设置属性 newObject.someKey = "Hello World"; //获取属性 var key = newObject.someKey; // 2. 方括号方式 // 设置属性 newObject["someKey"] = "Hello World"; //获取属性 var key = newObject["someKey"]; // 仅仅用于ECMAScript 5 // 3. Object.defineProperty // 设置属性 Object.defineProperty( newObject, "someKey", { value: "for more control of the property's behavior", writable: true, enumerable: true, configurable: true }); //可以通过下面的函数简化属性设置 var defineProp = function ( obj, key, value ){ config.value = value; Object.defineProperty( obj, key, config ); }; // 使用方法 var person = Object.create( null );defineProp( person, "car", "Delorean" ); defineProp( person, "dateOfBirth", "1981" ); defineProp( person, "hasBeard", false ); // 4. Object.defineProperties //设置属性 Object.defineProperties( newObject, { "someKey": { value: "Hello World", writable: true }, "anotherKey": { value: "Foo bar", writable: false } }); // 3和4的获取属性方法同1,2.
Basic constructor
We know that there is no concept of Class in JS, but it It also supports creating objects using constructors.
By using the [new] keyword, we can make a function behave like a constructor to create its own object instance.
A basic constructor form is as follows:
function Car( model, year, miles ) { //这里,this指向新建立的对象自己 this.model = model; this.year = year; this.miles = miles; this.toString = function () { return this.model + " has done " + this.miles + " miles"; }; } //用法 // 建立两个car实例 var civic = new Car( "Honda Civic", 2009, 20000 ); var mondeo = new Car( "Ford Mondeo", 2010, 5000 ); // 输出结果 console.log( civic.toString() ); console.log( mondeo.toString() );
This is the simple constructor pattern. It has two main problems,
First, it is difficult to inherit; second, toString() is defined once for each object instance. As a function, it should be shared by every instance of the Car type.
Using prototype constructors
There is a very good feature in JS: prototype [Prototype],
Use It, when creating an object, all properties in the constructor prototype can be obtained by the object instance.
This way multiple object instances can share the same prototype.
We improve the previous Car example as follows:
function Car( model, year, miles ) { this.model = model; this.year = year; this.miles = miles; } Car.prototype.toString = function () { return this.model + " has done " + this.miles + " miles"; }; // 用法 var civic = new Car( "Honda Civic", 2009, 20000 ); var mondeo = new Car( "Ford Mondeo", 2010, 5000 ); //输出 console.log( civic.toString() ); console.log( mondeo.toString() );
In the above example, the toString() method is shared by multiple Car object instances.
Related recommendations:
JavaScript Design Patterns Factory Pattern and Constructor Pattern_javascript skills
Node.js design The pattern uses streams for encoding
A brief introduction to the structural flyweight pattern of js design patterns
The above is the detailed content of JavaScript Constructor Pattern Example Analysis. For more information, please follow other related articles on the PHP Chinese website!
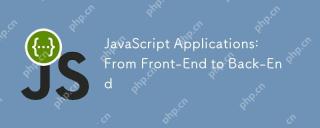
JavaScript can be used for front-end and back-end development. The front-end enhances the user experience through DOM operations, and the back-end handles server tasks through Node.js. 1. Front-end example: Change the content of the web page text. 2. Backend example: Create a Node.js server.
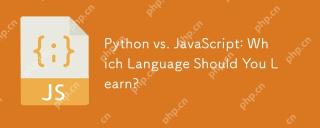
Choosing Python or JavaScript should be based on career development, learning curve and ecosystem: 1) Career development: Python is suitable for data science and back-end development, while JavaScript is suitable for front-end and full-stack development. 2) Learning curve: Python syntax is concise and suitable for beginners; JavaScript syntax is flexible. 3) Ecosystem: Python has rich scientific computing libraries, and JavaScript has a powerful front-end framework.
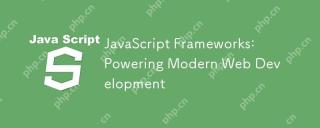
The power of the JavaScript framework lies in simplifying development, improving user experience and application performance. When choosing a framework, consider: 1. Project size and complexity, 2. Team experience, 3. Ecosystem and community support.
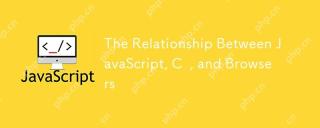
Introduction I know you may find it strange, what exactly does JavaScript, C and browser have to do? They seem to be unrelated, but in fact, they play a very important role in modern web development. Today we will discuss the close connection between these three. Through this article, you will learn how JavaScript runs in the browser, the role of C in the browser engine, and how they work together to drive rendering and interaction of web pages. We all know the relationship between JavaScript and browser. JavaScript is the core language of front-end development. It runs directly in the browser, making web pages vivid and interesting. Have you ever wondered why JavaScr
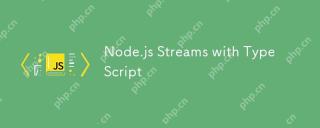
Node.js excels at efficient I/O, largely thanks to streams. Streams process data incrementally, avoiding memory overload—ideal for large files, network tasks, and real-time applications. Combining streams with TypeScript's type safety creates a powe
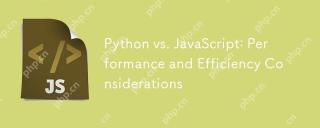
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
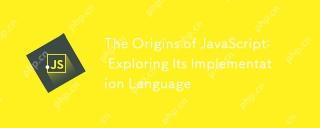
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
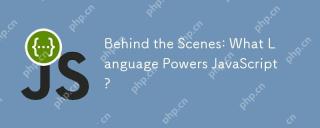
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment
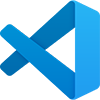
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 English version
Recommended: Win version, supports code prompts!

SublimeText3 Linux new version
SublimeText3 Linux latest version

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
