I have never used Javascript's setter and getter methods before when writing projects, so it is a concept that needs to be understood. Today I saw this knowledge point in a book, but it was still vague, so I planned to Research and study;
The properties of a Javascript object are composed of a name, a value and a set of properties. So first, let’s take a look at the two properties of the object:
Data properties, which we often use, should be familiar with
Accessor properties, also called accessor properties
What are accessor properties? It is a set of functions that get and set values. In ECMAScript5, property values can be set using one or two methods, the two methods are getters and setters; therefore, properties defined by getters and setters are called accessor properties.
var o = { get val(){ /*函数体*/ return ; }, set val(n){ /*函数体*/ } }
The above is the simplest way to define an accessor property. It can be seen that the getter and setter methods actually replace A function of function.
var o = { a:3, get val(){ return Math.pow(this.a,2); } } console.log(o.val);//9 o.val = 100; console.log(o.val);//9
The getter method has no parameters and returns a value; when the getter method is set separately, only the attribute value can be obtained. The defined attribute values cannot be changed, ensuring data security;
var o = { a:3, set val(n){ this.a = n; } } console.log(o.val);//undefined
The setter method has parameters and no return value ; When setting the setter method alone, the attribute value cannot be read;
var o ={ a:3, get val(){ return Math.pow(this.a,n); }, set val(n){ this.a = Math.max(this.a,n); } } console.log(o.a);//3 console.log(o.val);//9 o.val = 10; console.log(o.a);//10 console.log(o.val);//100
As can be seen from the above code, Where this refers to its object (i.e. "o" in the code);
var o ={ a:3, get val(){ return Math.pow(this.a,n); }, set val(n){ this.a = Math.max(this.a,n); } } o.val = 10; var foo = Object.create(o); console.log(foo.val);//10 foo.val = 9; console.log(foo.val);//10
In addition, the accessor attribute can also be inherited.
Related recommendations:
Basic introduction to getters and setters in Javascript
##Sample code sharing of getter/setter implementation in JavaScript
Implemented the code of a PHP5 getter/setter base class_php skills
The above is the detailed content of JavaScript setter and getter methods. For more information, please follow other related articles on the PHP Chinese website!
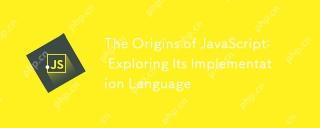
JavaScript originated in 1995 and was created by Brandon Ike, and realized the language into C. 1.C language provides high performance and system-level programming capabilities for JavaScript. 2. JavaScript's memory management and performance optimization rely on C language. 3. The cross-platform feature of C language helps JavaScript run efficiently on different operating systems.
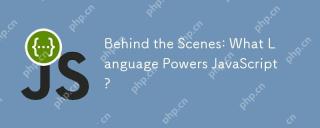
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
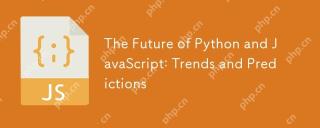
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
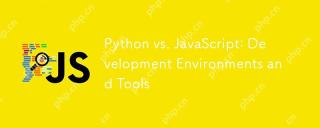
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
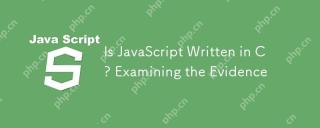
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
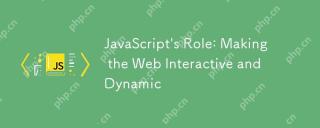
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
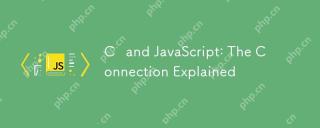
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
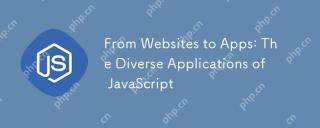
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
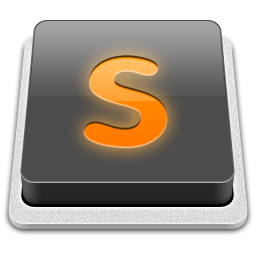
SublimeText3 Mac version
God-level code editing software (SublimeText3)
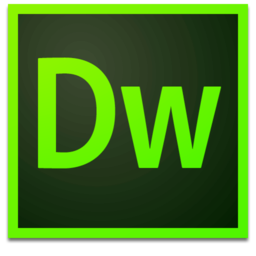
Dreamweaver Mac version
Visual web development tools
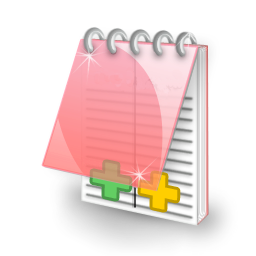
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
