Field type | Field name | Field code | Remarks |
Creator | Creator | Creator |
|
Creation time | Creation Time | Creation time |
|
##Single-line text box
Company name |
company |
| Set as required
The value is unique
|
Single-line text box
Company representative | representative |
|
|
Single line text box
Region |
area |
|
|
Single line text box
Location |
address |
|
|
Single line text box
Company phone number |
tel |
|
|
After the application is successfully created, enter three pieces of data

WeChat public account settings
1. Visit WeChat public platform and click "Enter the WeChat public account test account application system" and apply for the WeChat public account test account
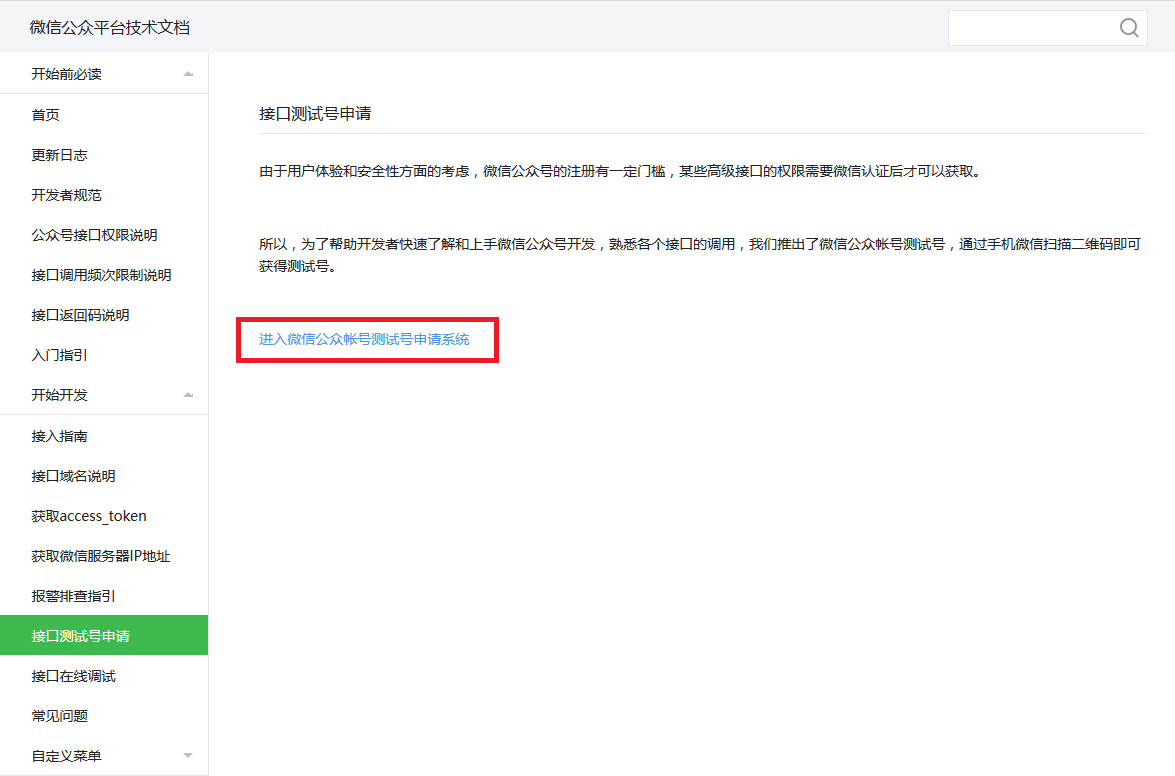
2. Enter the WeChat public test account
for testing In the account management page, we can see the appID and appsecret. Write down these two pieces of information, it will be useful later.
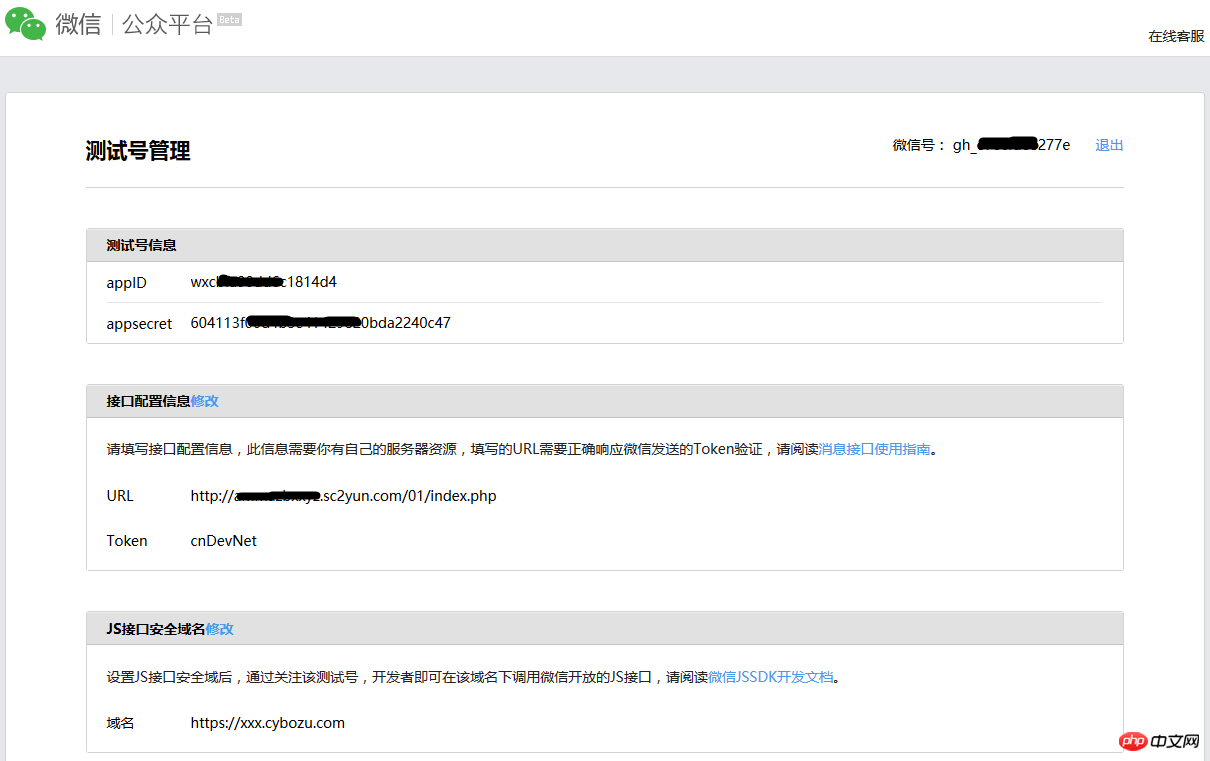
3. Fill in the interface configuration information
This information requires its own server resources. There are many cloud server resources online, and everyone can choose freely.
If you have a server with a public IP, you can also use it. Below we mainly use the PHP environment (the specific server configuration method is omitted)
Next, write the server verification code to make it Can correctly respond to the Token verification sent by WeChat. For details, please refer to Access Guide.
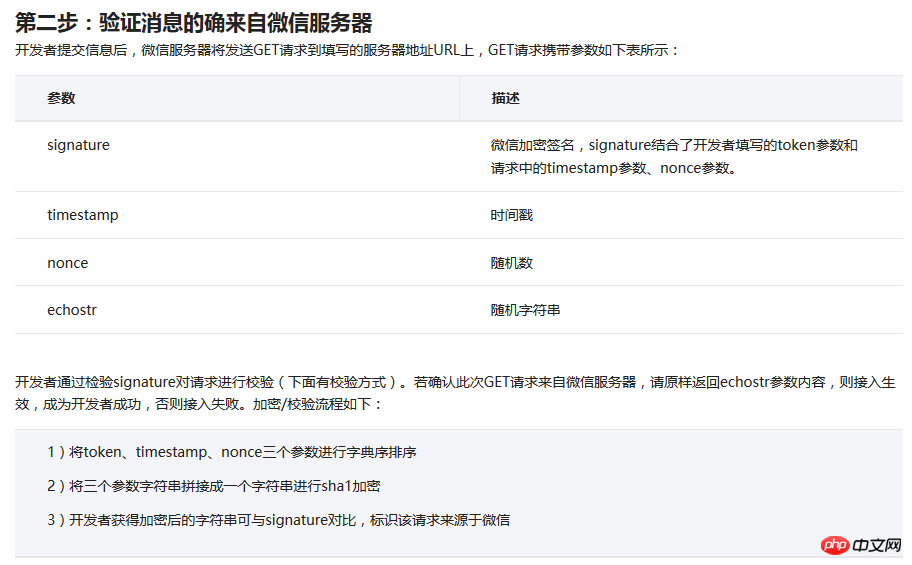
##Code
<?php
define("APPID", "wxcbfaxxxxxx1814d4"); //appID
define("APPSECRET", "604113xxxxxxxxxxxxxxx0bda2240c47"); //appsecret
define("TOKEN", "cnDevNet"); //Token
require "./wechat.inc.php";
$wechat = new WeChat(APPID, APPSECRET, TOKEN);
$wechat->valid(); //Token验证
?>
class WeChat
{
private $_appid;
private $_appsecret;
private $_token;
public function __construct($appid, $appsecret, $token)
{
$this->_appid = $appid;
$this->_appsecret = $appsecret;
$this->_token = $token;
}
public function valid()
{
$echoStr = $_GET["echostr"];
//valid signature , option
if($this->checkSignature())
{
echo $echoStr;
exit;
}
}
private function checkSignature()
{
$signature = $_GET["signature"];
$timestamp = $_GET["timestamp"];
$nonce = $_GET["nonce"];
$token = $this->_token;
$tmpArr = array($token, $timestamp, $nonce);
sort($tmpArr);
$tmpStr = implode( $tmpArr );
$tmpStr = sha1( $tmpStr );
if( $tmpStr == $signature )
{
return true;
}
else
{
return false;
}
}
}
Click "Modify" of the interface configuration information, After filling in the URL and Token, click the "Submit" button. If you see the following information, the configuration is successful.

Associated with kintoneThe following is the main schematic diagram. WeChat forwards the message to the server, and after the server interacts with kintone, the result is returned to the official account.

To interact with kintone, we mainly use the curl tool and kintone's API to retrieve records. For details, please refer to php documentation and kintone API: Get records in batches (specify conditions in the query) .
// 请求头部
$header = array(
"Host: " . $this->_subDomain . ".cybozu.com:443",
"X-Cybozu-API-Token: " . $this->_apiToken
);
$queryStr = 'company like "'. $keyword. '"';
$params = "?app=$this->_appId&query=".urlencode($queryStr)
. "&fields[0]=". urlencode("company")
. "&fields[1]=". urlencode("representative")
. "&fields[2]=". urlencode("area")
. "&fields[3]=". urlencode("address")
. "&fields[4]=". urlencode("tel");
$url = "https://" . $this->_subDomain . ".cybozu.com/k/v1/records.json". $params;
$response = $this->_request($url, true, "get", null, $header); //curl提交
$result = json_decode($response, true);
if (count($result["records"]) > 0) {
foreach($result["records"] as $value) {
if ($contentStr != '') {
$contentStr .= "\n\n";
}
$contentStr .= "公司名:". $value["company"]["value"]."\n"
. "公司代表:". $value["representative"]["value"]."\n"
. "地域:". $value["area"]["value"]."\n"
. "所在地:". $value["address"]["value"]."\n"
. "电话:". $value["tel"]["value"];
}
}
else {
$contentStr = "未找到该企业信息";
}
Detailed code
All codes can be viewed here
Reference
WeChat public platform technical documentation