


Detailed explanation of the method of staying on the current page after adding, deleting, modifying and checking in Yii2
ImplementationAdd, Delete, Modify and CheckIt will remain on the current page after the operation is successful, which can give the user a good experience. However, the Yii2 framework itself does not have the effect of remaining on the current page after the add, delete, modify, operation is successful. To achieve such an effect, you have to write it yourself. My principle is not to leave the core code alone and always stick to my own principles. Now that I have achieved it, I will share it. Different paths lead to the same goal. If there is a better way to implement Add, Delete, Modify and Check, please feel free to communicate.
Encapsulation code
There are two files ActionColumn.php and Helper.php
1 , ActionColumn.php file
<?php use Closure; use kartik\icons\Icon; use Yii; use yii\grid\Column; use yii\helpers\ArrayHelper; use yii\helpers\Html; use yii\helpers\Url; use common\components\Helper; /* *重写ActionColumn */ class ActionColumn extends Column { public $buttons; private $defaultButtons = []; private $callbackButtons; public $controller; public $urlCreator; public $url_append = ''; public $appendReturnUrl = true; //默认为true,返回当前链接 public function init() { parent::init(); $this->defaultButtons = [ [ 'url' => 'view', 'icon' => 'eye', 'class' => 'btn btn-success btn-xs', 'label' => Yii::t('yii', 'View'), 'appendReturnUrl' => false, 'url_append' => '', 'keyParam' => 'id',//是否传id,不传设置null ], [ 'url' => 'update', 'icon' => 'pencil', 'class' => 'btn btn-primary btn-xs', 'label' => Yii::t('yii', 'Update'), ], [ 'url' => 'delete', 'icon' => 'trash-o', 'class' => 'btn btn-danger btn-xs', 'label' => Yii::t('yii', 'Delete'), 'options' => [ 'data-action' => 'delete', ], ] ]; if (null === $this->buttons) { $this->buttons = $this->defaultButtons; } elseif ($this->buttons instanceof Closure) { $this->callbackButtons = $this->buttons; } } public function createUrl( $action, $model, $key, $index, $appendReturnUrl = null, $url_append = null, $keyParam = 'id', $attrs = [] ) { if ($this->urlCreator instanceof Closure) { return call_user_func($this->urlCreator, $action, $model, $key, $index); } else { $params = []; if (is_array($key)) { $params = $key; } else { if (is_null($keyParam) === false) { $params = [$keyParam => (string)$key]; } } $params[0] = $this->controller ? $this->controller . '/' . $action : $action; foreach ($attrs as $attrName) { if ($attrName === 'model') { $params['model'] = $model; } elseif ($attrName === 'mainCategory.category_group_id' && $model->getMainCategory()) { $params['category_group_id'] = $model->getMainCategory()->category_group_id; } else { $params[$attrName] = $model->getAttribute($attrName); } } if (is_null($appendReturnUrl) === true) { $appendReturnUrl = $this->appendReturnUrl; } if (is_null($url_append) === true) { $url_append = $this->url_append; } if ($appendReturnUrl) { $params['returnUrl'] = Helper::getReturnUrl(); } return Url::toRoute($params) . $url_append; } } protected function renderDataCellContent($model, $key, $index) { if ($this->callbackButtons instanceof Closure) { $btns = call_user_func($this->callbackButtons, $model, $key, $index, $this); if (null === $btns) { $this->buttons = $this->defaultButtons; } else { $this->buttons = $btns; } } $min_width = count($this->buttons) * 34; //34 is button-width $data = Html::beginTag('div', ['class' => 'btn-group', 'style' => 'min-width: ' . $min_width . 'px']); foreach ($this->buttons as $button) { $appendReturnUrl = ArrayHelper::getValue($button, 'appendReturnUrl', $this->appendReturnUrl); $url_append = ArrayHelper::getValue($button, 'url_append', $this->url_append); $keyParam = ArrayHelper::getValue($button, 'keyParam', 'id'); $attrs = ArrayHelper::getValue($button, 'attrs', []); Html::addCssClass($button, 'btn'); Html::addCssClass($button, 'btn-sm'); $buttonText = isset($button['text']) ? ' ' . $button['text'] : ''; $data .= Html::a( $button['label'] . $buttonText, $url = $this->createUrl( $button['url'], $model, $key, $index, $appendReturnUrl, $url_append, $keyParam, $attrs ), ArrayHelper::merge( isset($button['options']) ? $button['options'] : [], [ //'data-pjax' => 0, // 'data-action' => $button['url'], 'class' => $button['class'], 'title' => $button['label'], ] ) ) . ' '; } $data .= '</div>'; return $data; } }
2, Helper.php file
<?php use Yii; class Helper { private static $returnUrl; public static $returnUrlWithoutHistory = false; /** * @param int $depth * @return string */ public static function getReturnUrl() { if (is_null(self::$returnUrl)) { $url = parse_url(Yii::$app->request->url); $returnUrlParams = []; if (isset($url['query'])) { $parts = explode('&', $url['query']); foreach ($parts as $part) { $pieces = explode('=', $part); if (static::$returnUrlWithoutHistory && count($pieces) == 2 && $pieces[0] === 'returnUrl') { continue; } if (count($pieces) == 2 && strlen($pieces[1]) > 0) { $returnUrlParams[] = $part; } } } if (count($returnUrlParams) > 0) { self::$returnUrl = $url['path'] . '?' . implode('&', $returnUrlParams); } else { self::$returnUrl = $url['path']; } } return self::$returnUrl; } }
View call
1. Call it directly and replace the ['class' => 'yiigridActionColumn'] that comes with Yii2 with the new one we wrote ['class' => 'common\components\ActionColumn'].
2. If the direct call cannot meet your requirements, you can customize the link. The custom link is written as follows:
[ 'class' => 'common\components\ActionColumn', 'urlCreator' => function($action, $model, $key, $index) use ($id) { //自定义链接传的参数 $params = [ $action, 'option_id' => $model->option_id, 'id' => $id, ]; $params['returnUrl'] = common\components\Helper::getReturnUrl(); return yii\helpers\Url::toRoute($params); }, 'buttons' => [ [ 'url' =>'view', 'class' => 'btn btn-success btn-xs', 'label' => Yii::t('yii', 'View'), 'appendReturnUrl' => false,//是否保留当前URL,默认为true 'url_append' => '', 'keyParam' => 'id', //是否传id,不传设置null ], [ 'url' => 'update', 'class' => 'btn btn-primary btn-xs btn-sm', 'label' => Yii::t('yii', 'Update'), 'appendReturnUrl' => true,//是否保留当前URL,默认为true 'url_append' => '', 'keyParam' => 'id', //是否传id,不传设置null ], [ 'url' => 'delete', 'class' => 'btn btn-danger btn-xs btn-sm', 'label' => Yii::t('yii', 'Delete'), 'options' => [ 'data-action' => 'delete', ], 'appendReturnUrl' => true,//是否保留当前URL,默认为true 'url_append' => '', 'keyParam' => 'id', //是否传id,不传设置null ], ], ],
3. If you add a new one, quote it like this:
<?= Html::a(Yii::t('yii', 'Create'), ['create','returnUrl' => Helper::getReturnUrl()], ['class' => 'btn btn-success']) ?> 。
ControllerLogic
1. Use get to get returnUrl, code: $returnUrl = Yii::$ app->request->get('returnUrl'); .
2. The URL to jump to: return $this->redirect($returnUrl);.
Analysis Summary
1. The advantage of this method is that it does not leave the core code, and the calling method retains the Yii2 built-in method.
2. The disadvantage is that when customizing the link, you need to write out each operation update, view, and delete. You cannot use this kind of 'template' => '{view}{ update}{delete}' is simple and comfortable to look at. You can write it according to your needs.
Okay, that’s the entire content of this article. I hope the content of this article can be of some help to everyone’s study or work. If you have any questions, you can leave a message to communicate.
The above is a detailed explanation of the method of leaving the current page after adding, deleting, modifying and checking in Yii2. Welcome to discuss and exchange issues in PHP Chinese Community!
Related recommendations:
Detailed explanation of the registration and creation methods of components in Yii2
How Yii2 uses camel case naming to access controller instances
Implementation code for the QR code generation function of Yii2.0 framework
How to implement the automatic login and login and exit functions of Yii2 framework
The above is the detailed content of Detailed explanation of the method of staying on the current page after adding, deleting, modifying and checking in Yii2. For more information, please follow other related articles on the PHP Chinese website!
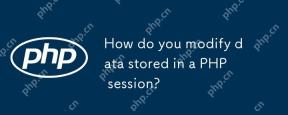
TomodifydatainaPHPsession,startthesessionwithsession_start(),thenuse$_SESSIONtoset,modify,orremovevariables.1)Startthesession.2)Setormodifysessionvariablesusing$_SESSION.3)Removevariableswithunset().4)Clearallvariableswithsession_unset().5)Destroythe
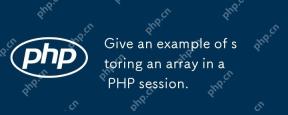
Arrays can be stored in PHP sessions. 1. Start the session and use session_start(). 2. Create an array and store it in $_SESSION. 3. Retrieve the array through $_SESSION. 4. Optimize session data to improve performance.
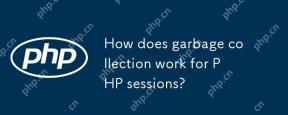
PHP session garbage collection is triggered through a probability mechanism to clean up expired session data. 1) Set the trigger probability and session life cycle in the configuration file; 2) You can use cron tasks to optimize high-load applications; 3) You need to balance the garbage collection frequency and performance to avoid data loss.
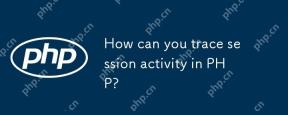
Tracking user session activities in PHP is implemented through session management. 1) Use session_start() to start the session. 2) Store and access data through the $_SESSION array. 3) Call session_destroy() to end the session. Session tracking is used for user behavior analysis, security monitoring, and performance optimization.
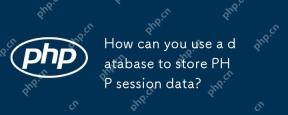
Using databases to store PHP session data can improve performance and scalability. 1) Configure MySQL to store session data: Set up the session processor in php.ini or PHP code. 2) Implement custom session processor: define open, close, read, write and other functions to interact with the database. 3) Optimization and best practices: Use indexing, caching, data compression and distributed storage to improve performance.
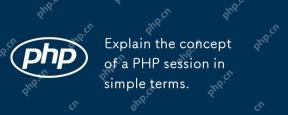
PHPsessionstrackuserdataacrossmultiplepagerequestsusingauniqueIDstoredinacookie.Here'showtomanagethemeffectively:1)Startasessionwithsession_start()andstoredatain$_SESSION.2)RegeneratethesessionIDafterloginwithsession_regenerate_id(true)topreventsessi
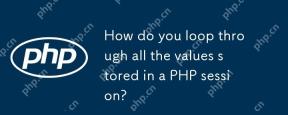
In PHP, iterating through session data can be achieved through the following steps: 1. Start the session using session_start(). 2. Iterate through foreach loop through all key-value pairs in the $_SESSION array. 3. When processing complex data structures, use is_array() or is_object() functions and use print_r() to output detailed information. 4. When optimizing traversal, paging can be used to avoid processing large amounts of data at one time. This will help you manage and use PHP session data more efficiently in your actual project.
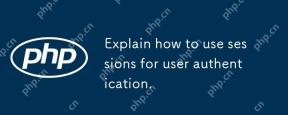
The session realizes user authentication through the server-side state management mechanism. 1) Session creation and generation of unique IDs, 2) IDs are passed through cookies, 3) Server stores and accesses session data through IDs, 4) User authentication and status management are realized, improving application security and user experience.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
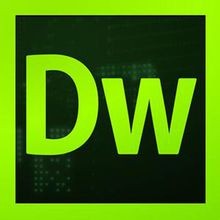
Dreamweaver CS6
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
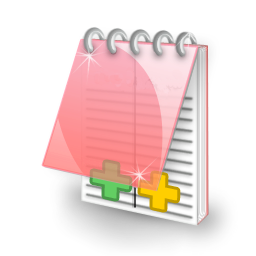
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
