1.
Array
Array method
##Mutator
method———— — "Mutation method" will change the value of the array itself;
Accessor
method --- "Access method" will not change the value of the array itself;
Iteration
Method————"Traversal method";
2.Mutator
Method
- ①
[ ].push
—
Function: Add one or more elements to the end of the array,
Pass parameters: (single or multiple array elements);
Return value: The length of the new array;
//标准用法 arr.push(el1, el2 ……elN); //合并两个数组 [].push.apply(arr1, arr2)
- ②
[ ].pop()
,
Function: Delete the last element,
Pass parameters: None;
Return value: The deleted element.
//标准用法 let a = [1 ,2 ,3 ]; a.pop();//3
- ③
[ ].unshift
—
Function: Add one or more elements to the beginning of the array,
Pass parameters: (Single or multiple array elements);
Return value: The length of the new array;
//标准用法 arr.unshift(el1, el2 ……elN);
- ④
[].shift()
,
Function: delete the first element,
Pass parameter: None;
Return value: deleted element.
//标准用法 let a = [1 ,2 ,3 ]; a.shift();//1
- ⑤
[].reverse()
,
Function: Reverse the position of array elements,
Pass parameters: None ;
Return value: reversed array.
//标准用法 arr.reverse()
- ⑥
[].splice()
,
Function: Reverse the position of array elements,
Pass parameters: ( Index, number to delete [select], element to be added [select]);
Return value: An array composed of deleted elements.
//标准用法 array.splice(start) array.splice(start, deleteCount) array.splice(start, deleteCount, item1, item2, ...)
- ⑦
[].fill()
,
Function: Fill an array with a fixed value from the starting index to the ending index All elements within,
Pass parameters: (value used to fill array elements, starting index [select], ending index [select]);
Return value: modified array .
//标准用法 arr.fill(value) arr.fill(value, start) arr.fill(value, start, end) //例子 [1, 2, 3].fill(4) // [4, 4, 4] [1, 2, 3].fill(4, 1) // [1, 4, 4] [1, 2, 3].fill(4, 1, 2) // [1, 4, 3]
- ⑧
[].sort()
,
Function: Sort the elements of the array and return the array,
pass Parameter: (function to specify the sorting order [optional]);
Return value: Arranged array.
//标准用法 arr.sort() arr.sort(compareFunction) //例子 var numbers = [4, 2, 5, 1, 3]; numbers.sort(function(a, b) { return a - b; });// [1, 2, 3, 4, 5]
3.Accessor
Method
- ##①
- [ ].join
—
Function
: Join all elements of an array (or an array-like object) into a string. ,Pass parameters
: (Specify a string to separate each element of the array [select]);Return value
: A string concatenating all array elements;<pre class='brush:php;toolbar:false;'>//标准用法 var a = [&#39;Wind&#39;, &#39;Rain&#39;, &#39;Fire&#39;]; var myVar1 = a.join(); // myVar1的值变为"Wind,Rain,Fire" var myVar2 = a.join(&#39;, &#39;); // myVar2的值变为"Wind, Rain, Fire"</pre>
② - [ ].concat
—
Function
: Concatenate two or more arrays. ,Passing parameters
: (Connect arrays and/or values into a new array [select]);Return value
: Merged array;<pre class='brush:php;toolbar:false;'>//标准用法 var alpha = [&#39;a&#39;, &#39;b&#39;, &#39;c&#39;]; var numeric = [1, 2, 3]; alpha.concat(numeric); //[&#39;a&#39;, &#39;b&#39;, &#39;c&#39;, 1, 2, 3]</pre>
③ - [ ].slice
—
Function
: The method returns a shallow copy of a part of the selected array from the beginning to the end (excluding the end) to a new array. ,Pass parameters
: (Start index [select], End index [select]);Return value
: Truncated array;<pre class='brush:php;toolbar:false;'>//标准用法 var fruits = [&#39;Banana&#39;, &#39;Orange&#39;, &#39;Lemon&#39;, &#39;Apple&#39;, &#39;Mango&#39;]; var citrus = fruits.slice(1, 3); //[&#39;Orange&#39;,&#39;Lemon&#39;] //类数组转数组 function list() { return [].slice.call(arguments)} var list1 = list(1, 2, 3); // [1, 2, 3]</pre>
④ - [ ].toString
—
Function
: Return a string representing the specified array and its elements,Pass parameters
: (none);Return value
: Converted string; (=[].join()
)<pre class='brush:php;toolbar:false;'>//标准用法 var monthNames = [&#39;Jan&#39;, &#39;Feb&#39;, &#39;Mar&#39;, &#39;Apr&#39;]; var myVar = monthNames.toString(); // assigns "Jan,Feb,Mar,Apr" to myVar.</pre>
⑤ - [ ].includes
—
Function
: Determine whether an array contains a specified value,Pass parameters
: (the element to be found);Return value
: true or false;<pre class='brush:php;toolbar:false;'>//标准用法 let a = [1, 2, 3]; a.includes(2); // true a.includes(4); // false</pre>
⑥ - [ ].indexOf
—
Function
: The first index of a given element can be found in the array,Pass parameters
: (element to be found);Return value
: Not found -1, index found;<pre class='brush:php;toolbar:false;'>var array = [2, 5, 9]; array.indexOf(2); // 0 array.indexOf(7); // -1</pre>
4.IterationMethod
- —
Function
: Each element executes the provided function once,
Pass parameters: (callback(current element, index, the array));
Return value: None;
//标准用法 array.forEach(callback(currentValue, index, array){ //do something }, this)
②
[ ].find - —
Function
: Return the array that satisfies the provided test The value of the first element of the function,
Pass parameters: (callback(current element, index, the array));
Return value: The element; (
[]. findIndex()Return index)
//标准用法 array. find(callback(currentValue, index, array){ //do something }, this)
③
[ ].filter - —
Function
: Create a new array containing All elements of the test implemented by the provided function,
Pass parameters: (callback(current element, index, the array));
Return value: Array of the collection of elements that pass the test;
//标准用法 let arr = array. filter(callback(currentValue, index, array){ //do something }, this)
-
④
[ ].map
—作用
:创建一个新数组,其结果是该数组中的每个元素都调用一个提供的函数后返回的结果。,传参
:(callback(当前元素,索引,该数组));返回值
:一个新数组,每个元素都是回调函数的结果;//标准用法 var numbers = [1, 4, 9]; var roots = numbers.map(Math.sqrt); // roots的值为[1, 2, 3], numbers的值仍为[1, 4, 9]
-
⑤
[ ].every
—作用
:测试数组的所有
元素是否都通过了指定函数的测试;传参
:(callback(当前元素,索引,该数组));返回值
:true
或false
;//标准用法 function isBigEnough(element, index, array) { return (element >= 10);} var passed = [12, 5, 8, 130, 44].every(isBigEnough);// passed is false passed = [12, 54, 18, 130, 44].every(isBigEnough);// passed is true
-
⑥
[ ].some
—作用
:测试数组的某些
元素是否都通过了指定函数的测试;传参
:(callback(当前元素,索引,该数组));返回值
:true
或false
;//标准用法 function isBigEnough(element, index, array) { return (element >= 10);} var passed = [1, 5, 8, 3, 4].some(isBigEnough);// passed is false passed = [2, 4, 18, 13, 4].some(isBigEnough);// passed is true
-
⑦
[ ].reduce
—作用
:对累加器和数组中的每个元素(从左到右)应用一个函数,将其减少为单个值;传参
:(callback(累加器accumulator,当前元素,索引,该数组));返回值
:函数累计处理的结果;//标准用法 var total = [0, 1, 2, 3].reduce(function(sum, value) { return sum + value; }, 0);// total is 6 var flattened = [[0, 1], [2, 3], [4, 5]].reduce(function(a, b) { return a.concat(b);}, []); // flattened is [0, 1, 2, 3, 4, 5]
-
⑧
[ ].entries
—作用
:返回一个新的Array Iterator对象,该对象包含数组中每个索引的键/值对;传参
:(无));返回值
:一个新的 Array 迭代器对象;//标准用法 var arr = ["a", "b", "c"]; var iterator = arr.entries();// undefined console.log(iterator);// Array Iterator {} console.log(iterator.next().value); // [0, "a"] console.log(iterator.next().value); // [1, "b"] console.log(iterator.next().value); // [2, "c"]
-
⑨
[ ].values
—作用
:数组转对象;传参
:(无));返回值
:一个新的 Array 迭代器对象;//标准用法 let arr = ['w', 'y', 'k', 'o', 'p']; let eArr = arr.values();// 您的浏览器必须支持 for..of 循环 // 以及 let —— 将变量作用域限定在 for 循环中 for (let letter of eArr) { console.log(letter);}
Mutator
方法————"突变方法"会改变数组自身的值;Accessor
方法————"访问方法"不会改变数组自身的值;Iteration
方法————"遍历的方法" ;-
①
[ ].push
—作用
:将一个或多个元素添加到数组的末尾,传参
:(单个或多个数组元素);返回值
:新数组的长度;//标准用法 arr.push(el1, el2 ……elN); //合并两个数组 [].push.apply(arr1, arr2)
-
②
[].pop()
,作用
:删除最后一个元素,传参
:无;返回值
:删除的元素。//标准用法 let a = [1 ,2 ,3 ]; a.pop();//3
-
③
[ ].unshift
—作用
:将一个或多个元素添加到数组的开头,传参
:(单个或多个数组元素);返回值
:新数组的长度;//标准用法 arr.unshift(el1, el2 ……elN);
-
④
[].shift()
,作用
:删除第一个元素,传参
:无;返回值
:删除的元素。//标准用法 let a = [1 ,2 ,3 ]; a.shift();//1
-
⑤
[].reverse()
,作用
:数组元素颠倒位置,传参
:无;返回值
:颠倒后的数组。//标准用法 arr.reverse()
-
⑥
[].splice()
,作用
:数组元素颠倒位置,传参
:(索引,删除个数【选】,要添加的元素【选】);返回值
:被删除的元素组成的一个数组。//标准用法 array.splice(start) array.splice(start, deleteCount) array.splice(start, deleteCount, item1, item2, ...)
-
⑦
[].fill()
,作用
:用一个固定值填充一个数组中从起始索引到终止索引内的全部元素,传参
:(用来填充数组元素的值,起始索引【选】,终止索引【选】);返回值
:修改后的数组。//标准用法 arr.fill(value) arr.fill(value, start) arr.fill(value, start, end) //例子 [1, 2, 3].fill(4) // [4, 4, 4] [1, 2, 3].fill(4, 1) // [1, 4, 4] [1, 2, 3].fill(4, 1, 2) // [1, 4, 3]
-
⑧
[].sort()
,作用
:对数组的元素进行排序,并返回数组,传参
:(指定排列顺序的函数【选】);返回值
:排列后的数组。//标准用法 arr.sort() arr.sort(compareFunction) //例子 var numbers = [4, 2, 5, 1, 3]; numbers.sort(function(a, b) { return a - b; });// [1, 2, 3, 4, 5]
-
①
<pre class='brush:php;toolbar:false;'>//标准用法 var a = [&#39;Wind&#39;, &#39;Rain&#39;, &#39;Fire&#39;]; var myVar1 = a.join(); // myVar1的值变为"Wind,Rain,Fire" var myVar2 = a.join(&#39;, &#39;); // myVar2的值变为"Wind, Rain, Fire"</pre>[ ].join
—作用
:将数组(或一个类数组对象)的所有元素连接到一个字符串中。,传参
:(指定一个字符串来分隔数组的每个元素【选】);返回值
:一个所有数组元素连接的字符串; -
②
[ ].concat
—作用
:并两个或多个数组。,传参
:(将数组和/或值连接成新数组【选】);返回值
:合并后的数组;//标准用法 var alpha = ['a', 'b', 'c']; var numeric = [1, 2, 3]; alpha.concat(numeric); //['a', 'b', 'c', 1, 2, 3]
-
③
<pre class='brush:php;toolbar:false;'>//标准用法 var fruits = [&#39;Banana&#39;, &#39;Orange&#39;, &#39;Lemon&#39;, &#39;Apple&#39;, &#39;Mango&#39;]; var citrus = fruits.slice(1, 3); //[&#39;Orange&#39;,&#39;Lemon&#39;] //类数组转数组 function list() { return [].slice.call(arguments)} var list1 = list(1, 2, 3); // [1, 2, 3]</pre>[ ].slice
—作用
:方法返回一个从开始到结束(不包括结束)选择的数组的一部分浅拷贝到一个新数组。,传参
:(开始索引【选】,结束索引【选】);返回值
:截去后的数组; -
④
<pre class='brush:php;toolbar:false;'>//标准用法 var monthNames = [&#39;Jan&#39;, &#39;Feb&#39;, &#39;Mar&#39;, &#39;Apr&#39;]; var myVar = monthNames.toString(); // assigns "Jan,Feb,Mar,Apr" to myVar.</pre>[ ].toString
—作用
:返回一个字符串,表示指定的数组及其元素,传参
:(无);返回值
:转化成的字符串;(=[].join()
) -
⑤
<pre class='brush:php;toolbar:false;'>//标准用法 let a = [1, 2, 3]; a.includes(2); // true a.includes(4); // false</pre>[ ].includes
—作用
:判断一个数组是否包含一个指定的值,传参
:(要查找的元素);返回值
:true或 false; -
⑥
<pre class='brush:php;toolbar:false;'>var array = [2, 5, 9]; array.indexOf(2); // 0 array.indexOf(7); // -1</pre>[ ].indexOf
—作用
:在数组中可以找到一个给定元素的第一个索引,传参
:(要查找的元素);返回值
:找不到-1,找得到索引; -
①
[ ].forEach
—作用
:每个元素执行一次提供的函数,传参
:(callback(当前元素,索引,该数组));返回值
:无;//标准用法 array.forEach(callback(currentValue, index, array){ //do something }, this)
-
②
[ ].find
—作用
:返回数组中满足提供的测试函数的第一个元素的值,传参
:(callback(当前元素,索引,该数组));返回值
:该元素;([].findIndex()
返回索引)//标准用法 array. find(callback(currentValue, index, array){ //do something }, this)
-
③
[ ].filter
—作用
:创建一个新数组, 其包含通过所提供函数实现的测试的所有元素,传参
:(callback(当前元素,索引,该数组));返回值
:通过测试的元素的集合的数组;//标准用法 let arr = array. filter(callback(currentValue, index, array){ //do something }, this)
-
④
[ ].map
—作用
:创建一个新数组,其结果是该数组中的每个元素都调用一个提供的函数后返回的结果。,传参
:(callback(当前元素,索引,该数组));返回值
:一个新数组,每个元素都是回调函数的结果;//标准用法 var numbers = [1, 4, 9]; var roots = numbers.map(Math.sqrt); // roots的值为[1, 2, 3], numbers的值仍为[1, 4, 9]
-
⑤
[ ].every
—作用
:测试数组的所有
元素是否都通过了指定函数的测试;传参
:(callback(当前元素,索引,该数组));返回值
:true
或false
;//标准用法 function isBigEnough(element, index, array) { return (element >= 10);} var passed = [12, 5, 8, 130, 44].every(isBigEnough);// passed is false passed = [12, 54, 18, 130, 44].every(isBigEnough);// passed is true
-
⑥
[ ].some
—作用
:测试数组的某些
元素是否都通过了指定函数的测试;传参
:(callback(当前元素,索引,该数组));返回值
:true
或false
;//标准用法 function isBigEnough(element, index, array) { return (element >= 10);} var passed = [1, 5, 8, 3, 4].some(isBigEnough);// passed is false passed = [2, 4, 18, 13, 4].some(isBigEnough);// passed is true
-
⑦
[ ].reduce
—作用
:对累加器和数组中的每个元素(从左到右)应用一个函数,将其减少为单个值;传参
:(callback(累加器accumulator,当前元素,索引,该数组));返回值
:函数累计处理的结果;//标准用法 var total = [0, 1, 2, 3].reduce(function(sum, value) { return sum + value; }, 0);// total is 6 var flattened = [[0, 1], [2, 3], [4, 5]].reduce(function(a, b) { return a.concat(b);}, []); // flattened is [0, 1, 2, 3, 4, 5]
-
⑧
[ ].entries
—作用
:返回一个新的Array Iterator对象,该对象包含数组中每个索引的键/值对;传参
:(无));返回值
:一个新的 Array 迭代器对象;//标准用法 var arr = ["a", "b", "c"]; var iterator = arr.entries();// undefined console.log(iterator);// Array Iterator {} console.log(iterator.next().value); // [0, "a"] console.log(iterator.next().value); // [1, "b"] console.log(iterator.next().value); // [2, "c"]
-
⑨
[ ].values
—作用
:数组转对象;传参
:(无));返回值
:一个新的 Array 迭代器对象;//标准用法 let arr = ['w', 'y', 'k', 'o', 'p']; let eArr = arr.values();// 您的浏览器必须支持 for..of 循环 // 以及 let —— 将变量作用域限定在 for 循环中 for (let letter of eArr) { console.log(letter);}
- [ ].forEach
参考资料:https://developer.mozilla.org...
1.
Array
数组的方法
2.
Mutator
方法
3.
Accessor
方法
4.
Iteration
方法
The above is the detailed content of Summary of JS array Array methods. For more information, please follow other related articles on the PHP Chinese website!
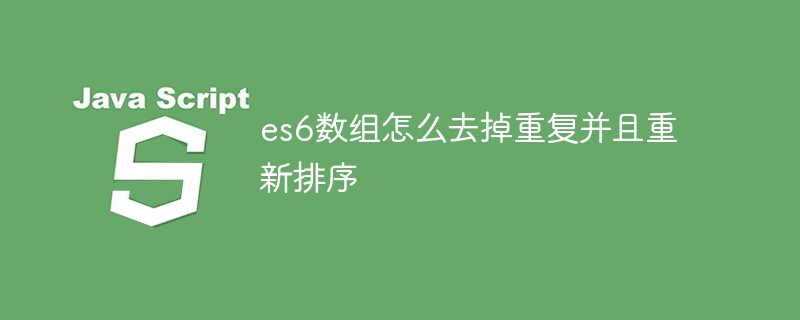
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
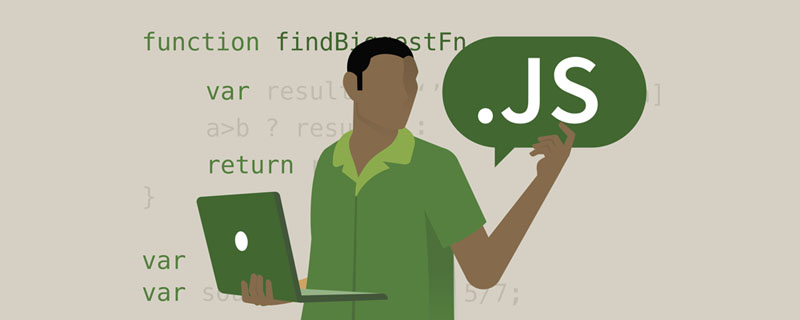
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
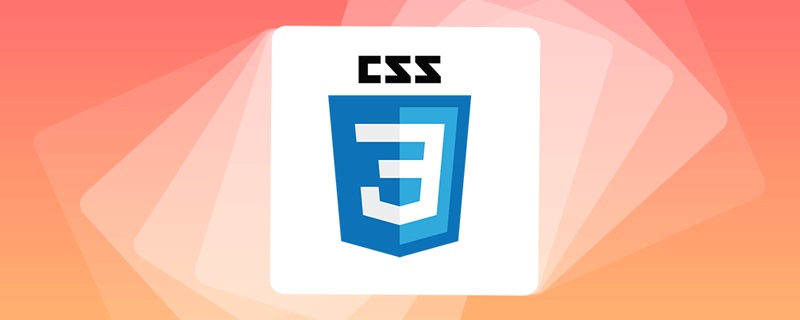
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
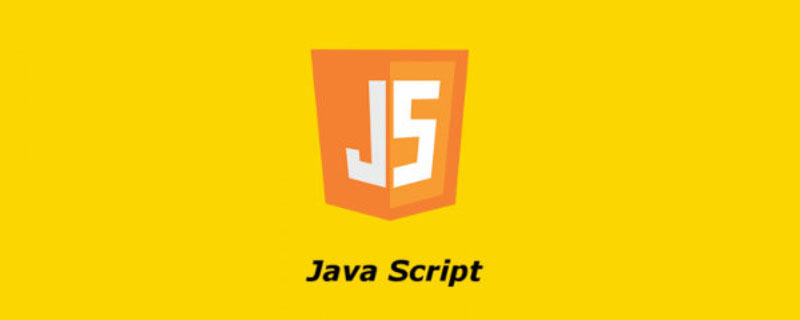
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
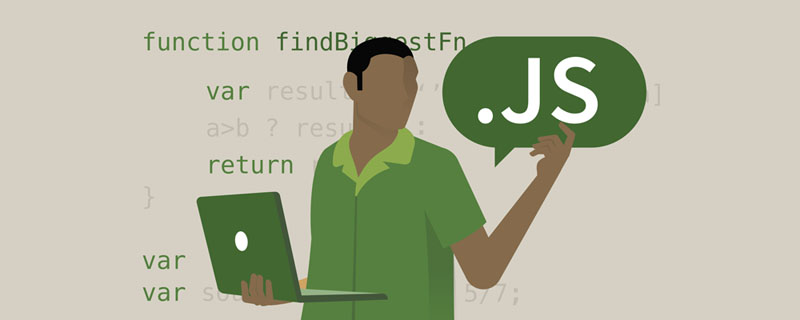
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
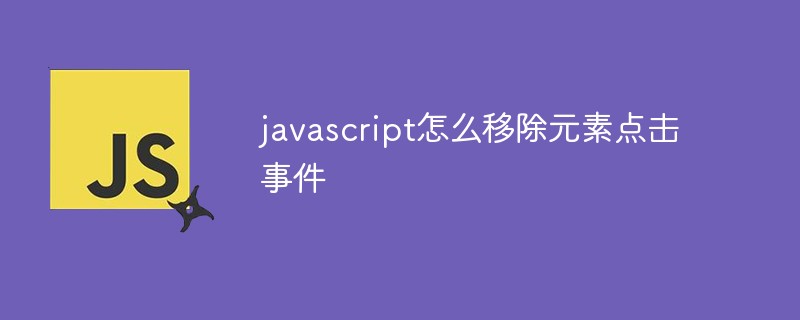
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
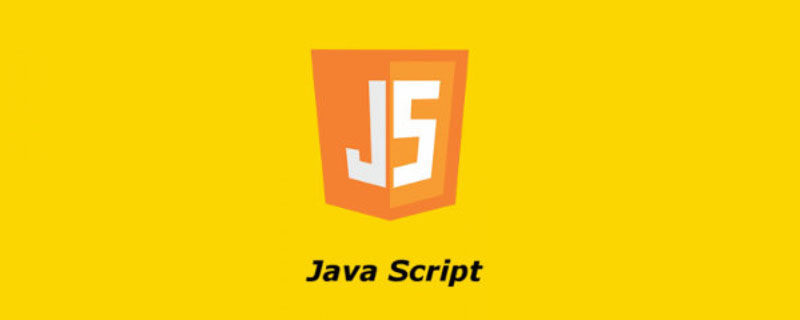
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
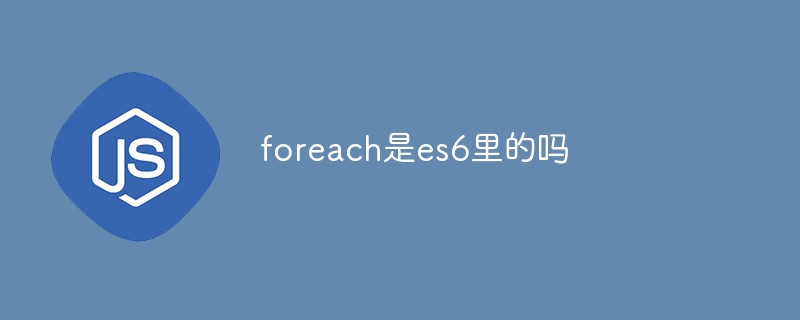
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Linux new version
SublimeText3 Linux latest version

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
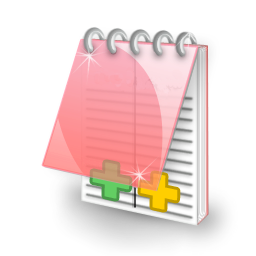
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
