Premise: The difference between primitive data types and object types when assigning values
JavaScript data types are divided into primitive data types and object types. The way they are stored in memory is different, resulting in differences in their assignment. Let’s take a chestnut respectively
var x = 1; var y = x; //y获得了和x同样的值 y = 2; console.log(x); // 1 var m = [1,2]; //m存放的是指向[1,2]这个数组对象的引用地址 var n = m; //n也获得 [1,2]数组对象的引用地址 n[0] = 3; console.log(m); //[3,2]
From the chestnut above, we can see that when the original data type is assigned, the actual data value is given. After the assignment, the two values are the same and will not affect each other. ; The object type gives the reference address of the original data, so the old and new data will affect each other, because it is essentially the same data object , such as the array in the above list
What is a shallow copy?
As the name suggests, shallow copy is a superficial copy method; when the attribute value is an object type, only the reference to the object data is copied, resulting in the old and new data not being completely separated. will also affect each other. Take another example...
//测试数据 var array1 = ['a',1,true,{name:'lei',age:18}]; //concat() slice() 实现浅拷贝 var array2 = array1.concat() //修改拷贝后的数据 array2[0] = 'b'; //array1[0]是原始数据类型 所以是直接赋值的 array2[3].name = 'zhang'; //array1[3]是对象数据类型 所以拷贝的是对象的引用,其实还是和原数组使用同一对象 console.log(array1); // ['a',1,true,{name:'zhang',age:18}]
In the chestnut, array2 is a shallow copy object of array1. The array elements are of primitive data types and will not affect each other (array1[0] ), but array1[3] is an object type and will still affect each other.
How to implement shallow copy
array.concat() or array.slice() in Shangli is a special implementation of shallow copy of array Way.
How to implement it yourself? Wouldn’t it be enough to traverse each attribute of the object/array and then assign it to a new object? The following implementation
//实现浅拷贝 function shallowCopy( target ){ if(typeof target !== 'object') return ; //判断目标类型,来创建返回值 var newObj = target instanceof Array ? [] : {}; for(var item in target){ //只复制元素自身的属性,不复制原型链上的 if(target.hasOwnProperty(item)){ newObj[item] = target[item] } } return newObj } //测试 var test = [1,'a',{name:'lei',age:18}]; var copy = shallowCopy(test); console.log(copy[2].name); //lei copy[2].name = 'zhang'; console.log(test[2].name); //zhang 原数据也被修改
deep copy and its implementation
From shallow copy The explanation is basically understandable. Deep copy is a 'complete' copy. After copying, the old and new data are completely separated. They no longer share the attribute values of the object type and will not affect each other.
Implementation method:
Tricky method JSON.parse(JSON.stringify(Obj))
var test = [1,'a',{name:'lei',age:18}]; var copy1 = JSON.parse(JSON.stringify(test)); //特殊方式 console.log(copy1); copy1[2].name = 'zhang' console.log(test); //[1,'a',{name:'lei',age:18}] 未受到影响
Note: This method cannot deep copy objects whose attribute values are functions. You can try it yourself
2. Implement deep copy
Having implemented shallow copy, think about it It should be that when assigning the object type attribute value, the result is not complete separation, so we need to modify the way of copying the object type attribute value, and call a deep copy on it again, thus realizing the deep copy, as follows:
//实现深拷贝 function deepCopy( target ){ if(typeof target !== 'object') return ; //判断目标类型,来创建返回值 var newObj = target instanceof Array ? [] : {}; for(var item in target){ //只复制元素自身的属性,不复制原型链上的 if(target.hasOwnProperty(item)){ newObj[item] = typeof target[item] == 'object' ? deepCopy(target[item]) : target[item] //判断属性值类型 } } return newObj } //测试 var test = [1,'a',{name:'lei',age:18}]; var copy2 = deepCopy(test); copy2[2].name = 'zhang' console.log(test); ////[1,'a',{name:'lei',age:18}] 未受到影响
Summary
Be sure to understand the reasons for shallow copy: when copying object type data, the reference address is copied, and the same data object is used; so the way to implement deep copy is to copy the object type attribute value Perform deep copy recursively to avoid direct assignment.
The above is the detailed content of How to implement deep and shallow copies of arrays and objects. For more information, please follow other related articles on the PHP Chinese website!
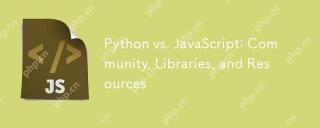
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
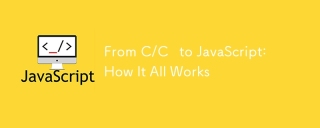
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
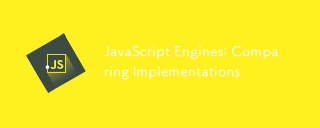
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
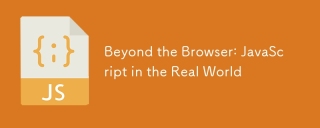
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
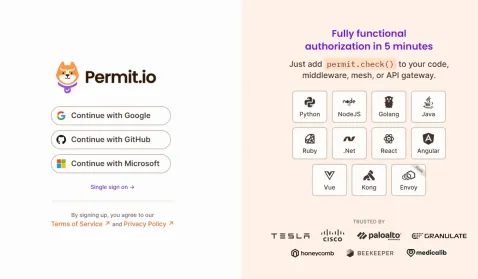
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
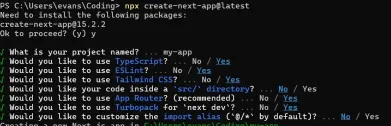
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
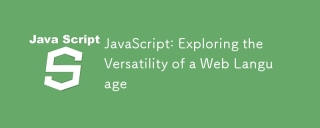
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
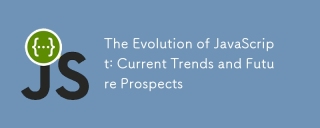
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
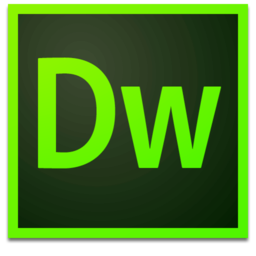
Dreamweaver Mac version
Visual web development tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.