This article mainly shares with you the relevant content about blocking and waking up in Java multi-threading. Through this article, you can roughly understand the methods of entering the thread blocking state and executable state. Friends who need it can learn about it.
Java thread blocking and waking up
1. sleep() method:
sleep (...milliseconds), specifies the time in milliseconds, so that the thread enters the thread blocking state within this time, during which the CPU time slice is not obtained. When the time passes, the thread re-enters the executable state. (Suspending the thread, the lock will not be released)
//测试sleep()方法 class Thread7 implements Runnable{ @Override public void run() { for(int i=0;i<50;i++){ System.out.println(Thread.currentThread().getName()+"num="+i); try { Thread.sleep(500); } catch (InterruptedException e) { e.printStackTrace(); } } } } class Thread8 implements Runnable{ @Override public void run() { for(int i=0;i<1000;i++){ System.out.println(Thread.currentThread().getName()+"num="+i); } } } public static void main(String[] args) { /* * 测试线程阻塞 */ //测试sleep()方法 Thread7 t7=new Thread7(); Thread8 t8=new Thread8(); Thread t81=new Thread(t8, "饺子"); Thread t71=new Thread(t7, "包子"); Thread t72=new Thread(t7, "面包"); t71.start(); t81.start(); t72.start(); }
2.suspend() and resume() methods:.
To suspend and wake up threads, suspend() puts the thread into a blocking state. Only when the corresponding resume() is called, the thread will enter the executable state. (Not recommended, deadlock is prone to occur)
//测试suspend()和resume()方法 class Thread9 implements Runnable{ @Override public void run() { for(long i=0;i<500000000;i++){ System.out.println(Thread.currentThread().getName()+" num= "+i); } } } public static void main(String[] args) { //测试suspend和resume Thread9 t9=new Thread9(); Thread t91=new Thread(t9,"包子"); t91.start(); try { Thread.sleep(2000); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } t91.suspend(); try { Thread.sleep(2000); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } t91.resume(); }
(When the console prints output, it will pause for 2 seconds and then continue printing.)
3. yield() method:
will cause the thread to give up the current CPU time slice, but the thread is still executable at this time status, the CPU time slice can be allocated again at any time. The yield() method can only give threads of the same priority a chance to execute. The effect of calling yield() is equivalent to the scheduler deeming that the thread has executed enough time to move to another thread. (Pause the currently executing thread and execute other threads, and the time to give up is unknown)
//测试yield()方法 class Thread10 implements Runnable{ @Override public void run() { for(int i=0;i<100;i++){ System.out.println(Thread.currentThread().getName()+" num= "+i); if(i==33){ Thread.yield(); } } } } public static void main(String[] args) { //测试yield Thread10 t10 =new Thread10(); Thread t101=new Thread(t10, "包子"); Thread t102=new Thread(t10, "面包"); t101.start(); t102.start(); } /* 运行结果为: …… 包子 num= 24 包子 num= 25 包子 num= 26 包子 num= 27 包子 num= 28 包子 num= 29 包子 num= 30 包子 num= 31 包子 num= 32 包子 num= 33 面包 num= 0 面包 num= 1 面包 num= 2 面包 num= 3 …… 面包 num= 30 面包 num= 31 面包 num= 32 面包 num= 33 包子 num= 34 包子 num= 35 包子 num= 36 包子 num= 37 包子 num= 38 …… */
(As you can see, when the number is 33, all occur Alternate.)
4.wait() and notify() methods:
//测试wait()和notify()方法 //用生产者和消费者模式模拟这一过程 /*消费者 */ class Consumer implements Runnable { private Vector obj; public Consumer(Vector v) { this.obj = v; } public void run() { synchronized (obj) { while (true) { try { if (obj.size() == 0) { obj.wait(); } System.out.println("消费者:我要买面包。"); System.out.println("面包数: " + obj.size()); obj.clear(); obj.notify(); } catch (Exception e) { e.printStackTrace(); } } } } } /* 生产者 */ class Producter implements Runnable { private Vector obj; public Producter(Vector v) { this.obj = v; } public void run() { synchronized (obj) { while (true) { try { if (obj.size() != 0) { obj.wait(); } obj.add(new String("面包")); obj.notify(); System.out.println("生产者:面包做好了。"); Thread.sleep(500); } catch (Exception e) { e.printStackTrace(); } } } } } public static void main(String[] args) { //测试wait()和notify() Vector obj = new Vector(); Thread consumer = new Thread(new Consumer(obj)); Thread producter = new Thread(new Producter(obj)); consumer.start(); producter.start(); }
5.join() method
//测试join class Thread11 implements Runnable{ @Override public void run() { System.out.println("Start Progress."); try { for(int i=0;i<5;i++){ System.out.println("Thread11线程 : "+i); Thread.sleep(1000); } } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } System.out.println("End Progress."); } } public static void main(String[] args) { //测试join Thread11 t11=new Thread11(); Thread t111=new Thread(t11); t111.start(); try { t111.join(); } catch (InterruptedException e) { // TODO Auto-generated catch block e.printStackTrace(); } System.out.println("hi,I'm Main线程"); } /* 运行结果为: Start Progress. Thread11线程 : 0 Thread11线程 : 1 Thread11线程 : 2 Thread11线程 : 3 Thread11线程 : 4 End Progress. hi,I'm Main线程 */
Summary
The above is the detailed content of Sample code for multi-thread blocking and wake-up in Java. For more information, please follow other related articles on the PHP Chinese website!
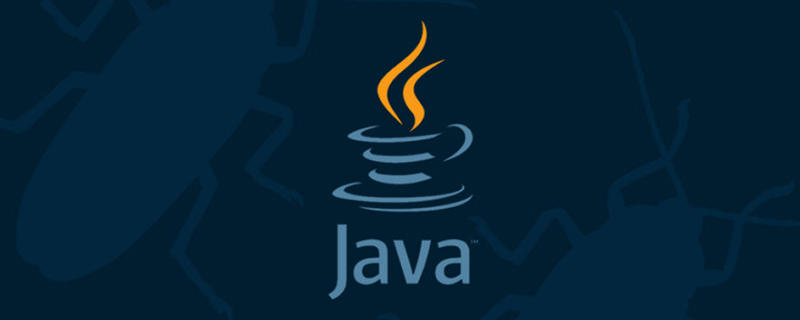
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
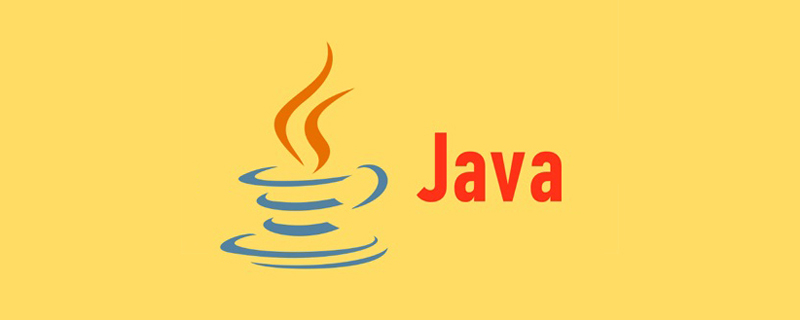
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
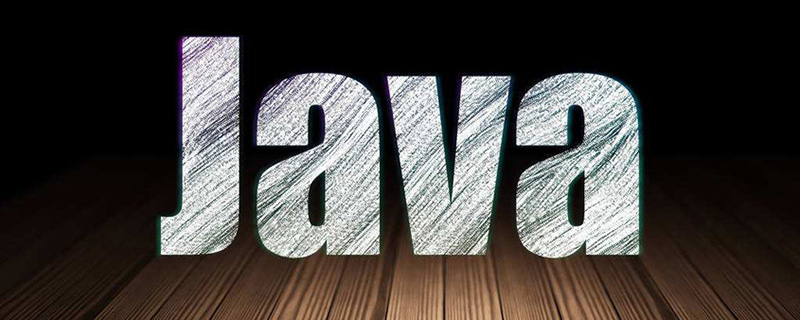
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
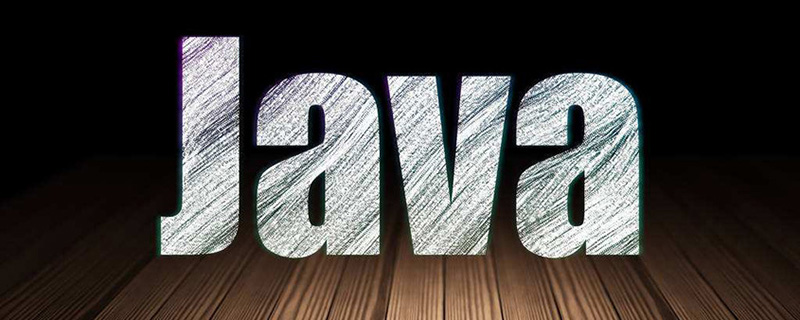
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
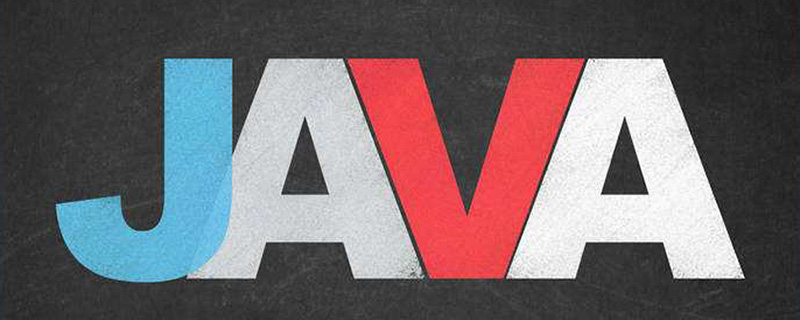
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
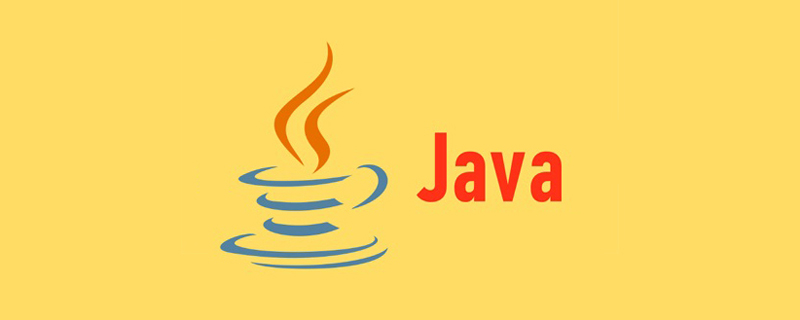
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
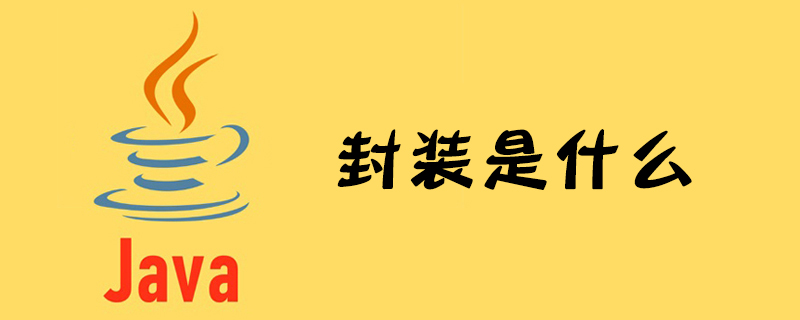
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
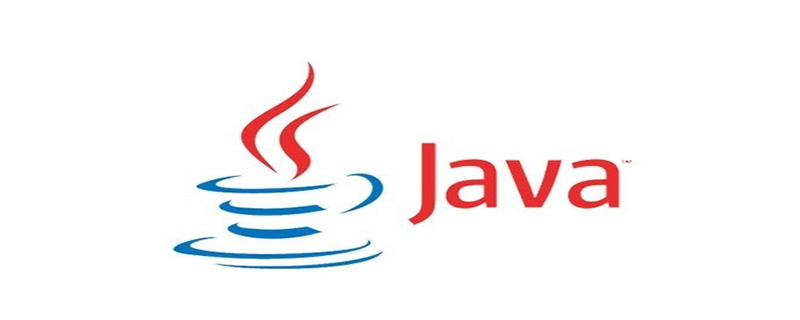
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
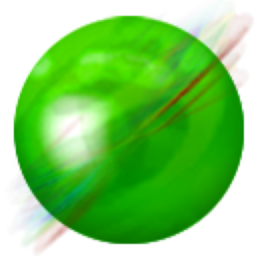
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
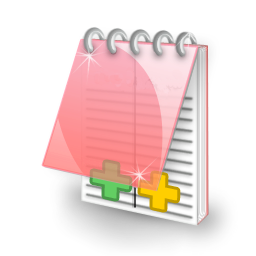
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
