PHP operation to implement a multi-functional shopping website
1. Pages to be implemented:
Index.aspx:浏览商品页面,显示商品列表,用户可以点击“加入购物车“。 ViewCart.aspx:查看购物车页面,显示已购买的商品信息,可以点击“删除“和“提交添加订单购买”商品 ViewAccount.aspx:查看个人账户余额 Login.aspx:登录页面
2. Implementation functions:
1. Display product list
2. Implement the purchase function, and dynamically display the quantity of goods in the shopping cart and the total price of the goods when purchasing
3. After clicking to view the shopping cart, the purchased goods will be displayed. Pay attention to the "Purchase Quantity" column. If you click to buy a product multiple times, its "Purchase Quantity" will continue to increase.
4. Delete the purchased items in the shopping cart.
If the "Purchase Quantity" of a product is 1, click "Delete" to delete the product directly from the shopping cart;
If the "Purchase Quantity" of a product is greater than 1, click "Delete" once When , reduce its purchase quantity by 1. When the purchase quantity of the product reaches 1, and then click Delete, the product will be deleted
5. After viewing the shopping cart, you can also click "Browse Products" to continue purchasing. And display the quantity of goods purchased and the total price above.
6. After "View Shopping Cart", you can submit the order.
But when submitting an order, the following functions must be completed:
(a) Check whether the user is logged in. If not logged in, go to the Login.aspx page
(b) Check the user account Whether the balance is sufficient for this purchase
(c) Check whether the inventory quantity is sufficient for this purchase
(d) If the above conditions are met, then
i. From Deduct the total price of this purchase from the user account
ii. Deduct the purchase quantity of each product from the product inventory
iii. Add this purchase to the order table and order content table Purchased product information
7. Click View Account to view the user’s account balance
The operation code is as follows:
1. First, make a login page: loginpage.php
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title></title> <script src="bootstrap/js/jquery-1.11.2.min.js"></script> <script src="bootstrap/js/bootstrap.min.js"></script> <link href="bootstrap/css/bootstrap.min.css" rel="stylesheet" type="text/css"/> </head> <style> .title{ margin-left: 750px; margin-top: 150px; } .quanju{ margin-left: 650px; margin-top: -460px; } .name,.pwd{ max-width: 120px; } .yangshi1{ margin-top: 200px; } .header{ width: 100%; height: 80px; background: #e0e0e0; } .ps{ margin-left: 100px; margin-top: -100px; } </style> <body> <form class="form-horizontal" role="form" action="dengluchuli.php" method="post"> <p class="header"> <img src="/static/imghwm/default1.png" data-src="img/logo.png" class="lazy" style="max-width:90%" style="max-width:90%" style="margin-top: 10px; margin-left: 100px;" / alt="PHP operation to implement a multi-functional shopping website" > <p style="height: 50px; width: 300px; color: green;float: right; font-size: 50px; margin-right: 350px">果 蔬 网</p> </p> <h3 id="用户登录">用户登录</h3> <img class="ps lazy" src="/static/imghwm/default1.png" data-src="./img/果蔬专场.jpg" style="max-width:90%" style="max-width:90%" / alt="PHP operation to implement a multi-functional shopping website" > <p class="quanju"> <p class="form-group yangshi1"> <label for="firstname" class="col-sm-2 control-label">用户名:</label> <p class="col-sm-10"> <input type="text" class="form-control name" name="uid" placeholder="请输入用户名"> </p> </p> <p class="form-group yangshi2"> <label for="lastname" class="col-sm-2 control-label">密码:</label> <p class="col-sm-10"> <input type="text" class="form-control pwd" name="pwd" placeholder="请输入密码"> </p> </p> <p class="form-group"> <p class="col-sm-offset-2 col-sm-10"> <p class="checkbox"> <label> <input type="checkbox"> 保存密码 </label> <label> <input type="checkbox"> 下次自动登录 </label> </p> </p> </p> <p class="form-group"> <p class="col-sm-offset-2 col-sm-10"> <button type="submit" class="btn btn-warning" value="登录" onclick="return login()" > 登录 </button> </p> </p> </p> </form> </body> <script> function login(){ var uid = document.getElementsByTagName("input")[0].value; if(uid==""){ alert("请输入用户名!"); return false; } var pwd = document.getElementsByTagName("input")[1].value; if(pwd==""){ alert("请输入密码!"); return false; } } </script> </html>
The effect is as shown in the figure:
2. Make a login processing page: dengluchuli.php
<?php session_start(); $uid = $_POST["uid"]; $pwd = $_POST["pwd"]; require_once "./DBDA.class.php"; $db = new DBDA(); $sql = "select * from login where username='{$uid}'"; $arr = $db->query($sql,0); if($arr[0][2]==$pwd && !empty($pwd)){ $_SESSION["uid"]=$uid; header("location:shopping_list.php"); }else{ echo "登陆失败!"; }
In this way, you can contact the database. This is the login account and password of the database. Verify the account and password, and then jump to the homepage: shopping_list.php
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title></title> <script src="bootstrap/js/jquery-1.11.2.min.js"></script> <script src="bootstrap/js/bootstrap.min.js"></script> <link href="bootstrap/css/bootstrap.min.css" rel="stylesheet" type="text/css"/> </head> <body> <h2 id="水果列表">水果列表</h2> <?php session_start();
//1. Find out how many products are in the shopping cart and the total price
$uid = $_SESSION["uid"]; if(empty($_SESSION["uid"])){ header("location:loginpage.php"); exit; } require_once "./DBDA.class.php"; $db = new DBDA();
//If there are products in the shopping cart, take out the value
if(!empty($_SESSION["gwd"])){ $arr = $_SESSION["gwd"]; $sum = 0; $numbers = count($arr); foreach($arr as $k=>$v){ //$v[0];//水果名称 //$v[1];//购买数量 $sql = "select * from fruit where ids='{$v[0]}'"; $attr = $db->query($sql,0); $dj = $attr[0][2]; //单价 $sum = $sum+$dj*$v[1]; //总价=单价*数量 } } echo @"<p style='margin-left: 250px'>购物车中商品总数为{$numbers}个,商品总价为:{$sum}元</p>"; ?> <a href="loginpage.php" style="float: right; margin-top: -25px; margin-right: 330px; color: blueviolet; font-size: 20px;"> 登录 </a> <table class="table table-bordered" style="max-width: 800px; margin-left: 250px;"> <thead> <tr> <th>代号</th> <th>名称</th> <th>价格</th> <th>产地</th> <th>库存</th> <th>操作</th> </tr> </thead> <tbody> <?php $sql = "select * from fruit"; $arr = $db->query($sql,0); foreach($arr as $v){ echo "<tr> <td>{$v[0]}</td> <td>{$v[1]}</td> <td>{$v[2]}</td> <td>{$v[3]}</td> <td>{$v[4]}</td> <td><a href='shoppingchuli.php?ids={$v[0]}'>加入购物车</a></td> </tr>"; } ?> </tbody> </table> <a href="add_list.php" style="margin-left: 250px;">查看购物车</a> </body> </html>4. Then do the processing page of the home page: shoppingchuli.php
<?php session_start(); //取到传过来的主键值,并且添加到购物车的SESSION里面 $ids = $_GET["ids"]; //如果是第一次添加购物车,造一个二维数组存到SESSION里面 //如果不是第一次添加,有两种情况 //1.如果该商品购物车里面不存在,造一个一维数组扔到二维里面 //2.如果该商品在购物车存在,让数量加1 if(empty($_SESSION["gwd"])){ //如果是第一次添加购物车,造一个二维数组存到SESSION里面 $arr = array( array($ids,1)); $_SESSION["gwd"]=$arr; }else{ $arr=$_SESSION["gwd"]; if(deep_in_array($ids,$arr)){ //如果该商品在购物车存在,让数量加1 foreach($arr as $k=>$v){ if($v[0]==$ids){ $arr[$k][1]++; } } $_SESSION["gwd"]=$arr; }else{ //如果该商品购物车里面不存在,造一个一维数组扔到二维里面 $arr=$_SESSION["gwd"]; $attr=array($ids,1); $arr[]=$attr; $_SESSION["gwd"]=$arr; } } header("location:shopping_list.php"); function deep_in_array($value, $array) { foreach($array as $item) { if(!is_array($item)) { if ($item == $value) { return true; } else { continue; } } if(in_array($value, $item)) { return true; } else if(deep_in_array($value, $item)) { return true; } } return false; }The effect is as shown in the figure:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title></title> <script src="bootstrap/js/jquery-1.11.2.min.js"></script> <script src="bootstrap/js/bootstrap.min.js"></script> <link href="bootstrap/css/bootstrap.min.css" rel="stylesheet" type="text/css"/> </head> <?php session_start(); $uid = $_SESSION["uid"]; if(empty($_SESSION["uid"])){ header("location:loginpage.php"); exit; } ?> <body> <h2 id="购物车清单">购物车清单</h2> <table class="table table-bordered" style="max-width: 800px; margin-left: 250px;"> <thead> <tr> <th>代号</th> <th>名称</th> <th>价格</th> <th>产地</th> <th>购买数量</th> <th>操作</th> </tr> </thead> <tbody> <?php require_once "./DBDA.class.php"; $db = new DBDA(); if(!empty($_SESSION["gwd"])){ $arr = $_SESSION["gwd"]; $sum = 0; $numbers = count($arr); foreach($arr as $k=>$v){ //$v[0];$v[1]; $sql = "select * from fruit where ids='{$v[0]}'"; $a = $db->query($sql,0); //var_dump($v[1]); echo "<tr> <td>{$v[0]}</td> <td>{$a[0][1]}</td> <td>{$a[0][2]}</td> <td>{$a[0][3]}</td> <td>{$v[1]}</td> <td><a href='goodsdel.php?zj={$k}'>删除</a></td> </tr>"; $dj = $a[0][2]; $sum = $sum+$dj*$v[1]; } } //echo "<p style='margin-left: 250px;'>购物车中商品总数为{$numbers}个,商品总价为:{$sum}元</p>"; ?> </tbody> </table> <a href="submit_order.php?ids={$v[0]}" style="margin-left: 250px;">提交订单</a> </body> </html>The effect is as shown:
<?phpsession_start(); $zj = $_GET["zj"]; //如果该水果数量大于1,减1//如果该水果数量等于1 移除$arr = $_SESSION["gwd"]; if($arr[$zj][1]>1) { $arr[$zj][1]=$arr[$zj][1]-1; } else { unset($arr[$zj]); //清除数组 $arr=array_values($arr); //重新索引数组 } $_SESSION["gwd"] = $arr; header("location:add_list.php"); 7..然后做提交页面 :tijiao.php <?phpsession_start(); $ids = $_GET["ids"]; //查看余额$uid = $_SESSION["uid"]; require_once "./DBDA.class.php"; $db = new DBDA(); $sql = "select account from login where username=' { $uid } '"; $arr = $db->query($sql,0); $aye = $arr[0][0]; //余额//var_dump($aye); if(!empty($_SESSION["gwd"])) { $arr = $_SESSION["gwd"]; $sum = 0; //$numbers = count($arr); foreach($arr as $v) { $sql = "select * from fruit where ids=' { $v[0] } '"; $price = $db->query($sql,0); $dj = $price[0][2]; $sum = $sum+$dj*$v[1]; } }else { echo "您还未购买商品!"; //header("shopping_list.php"); exit; } //判断余额是否满足购买if($aye>=$sum) { //判断库存 foreach($arr as $v) { $skc = "select name,numbers from fruit where ids=' { $v[0] } '"; $akc = $db->query($sql,0); var_dump($akc); $kc = $akc[0][4]; //库存 //var_dump($kc); if($kc<$v[1]) { echo "库存不足!"; exit; } } //提交订单 //账户扣除余额 $skye = "update login set account=account- { $sum } where username=' { $uid } '"; $zhye = $db->query($skye); //扣除库存 foreach($arr as $v) { $skckc = "update fruit set numbers=numbers- { $v[1] } where ids=' { $v[0] } '"; $sykc = $db->query($skckc); } //添加订单 $ddh = date("Y-m-d H:i:s"); $time = time(); $stjd = "insert into orders values(' { $time } ',' { $uid } ',' { $ddh } ')"; $wcdh = $db->query($stjd); //添加订单详情 foreach($arr as $v) { $ddxq = "insert into orderdetails values('',' { $ddh } ',' { $v[0] } ',' { $v[1] } ')"; $axq = $db->query($ddxq); } }else { echo "余额不足,请充值!"; exit; } header("location:shopping_list.php");The user account balance has been reduced:
The above is the detailed content of PHP operation to implement a multi-functional shopping website. For more information, please follow other related articles on the PHP Chinese website!
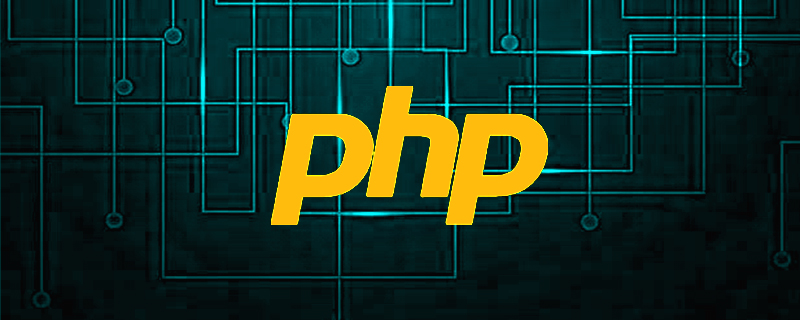
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
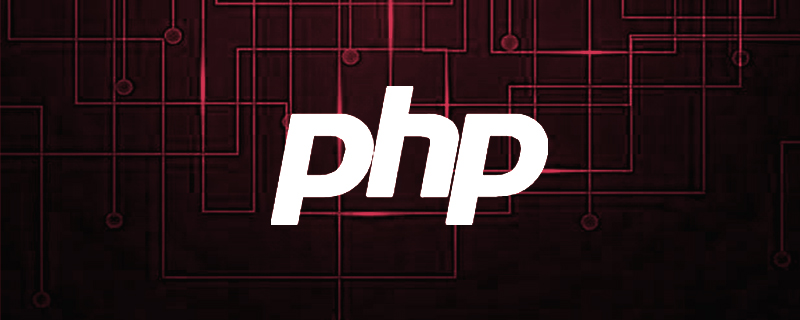
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
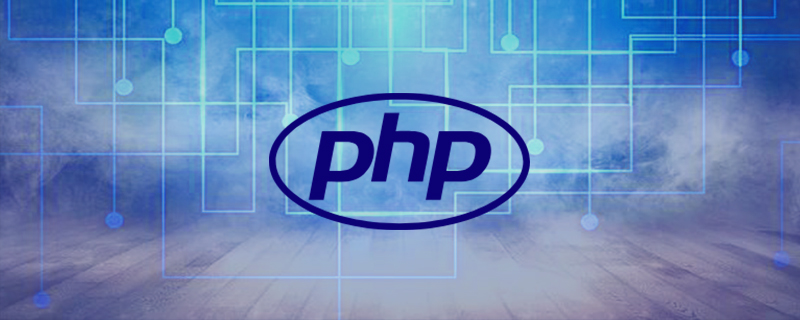
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
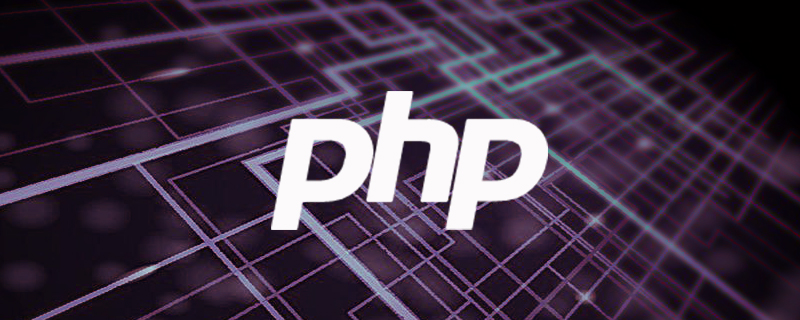
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
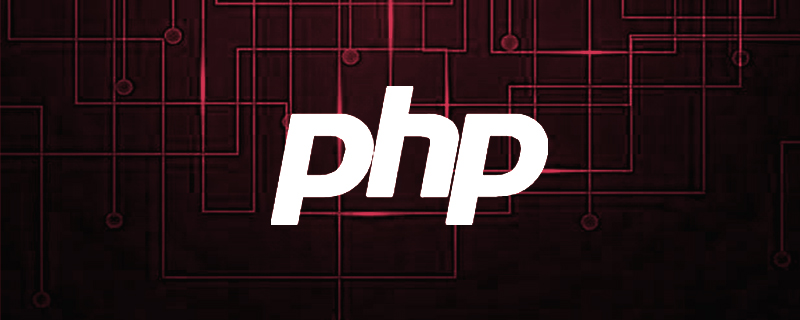
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
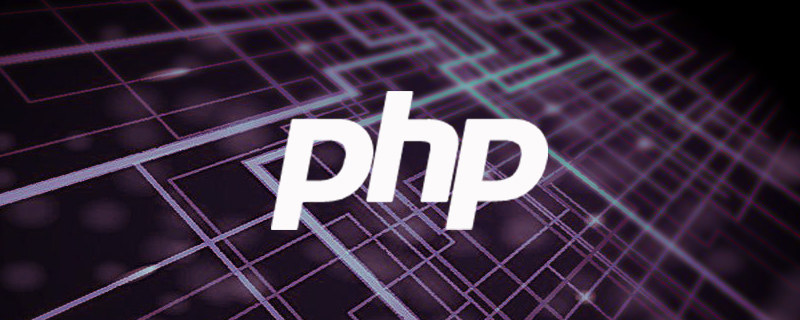
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
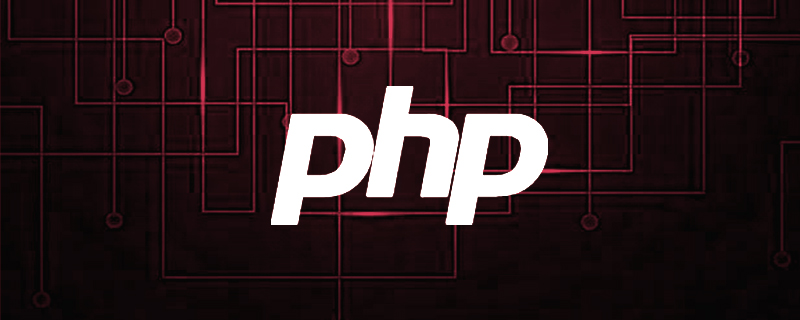
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
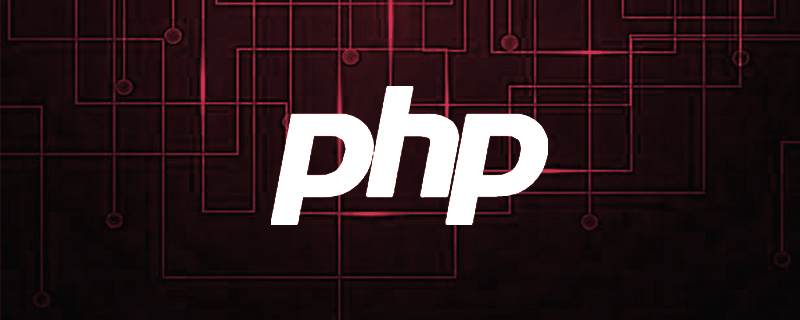
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
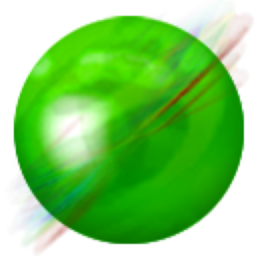
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
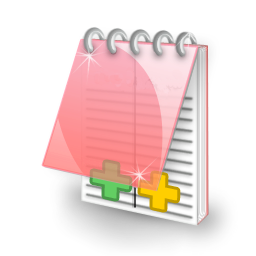
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
