This article mainly introduces the implementation of JS paging (synchronous and asynchronous). Friends in need can refer to the following
Paging technology is divided into back-end paging and front-end paging.
Front-end paging
Taking out all the data at once and then paging it through js has its drawbacks: Assume there is a product table dbgoods, which stores 999.99 million pieces of data. Execute the query statement select *from dbgoods where 1=1
Receive the query structure using List<goods>list </goods>
, and the server will pass such a huge amount of data to the front end, It will cause large download volume (traffic is money), high server pressure, etc.
Back-end paging
Back-end paging only queries one page of values per request, taking mysql as an example (starting with the first query, querying 8 items)
select * from dbgoods order by id limit 0,8;
Backend synchronization paging
Principle: There needs to be a Bean to record paging information,
public class PageBean{ private long total; //总记录数 private List<T> list; //结果集 private int pageNum; // 第几页 private int pageSize; // 每页记录数 private int pages; // 总页数 private int size; // 当前页的数量 <= pageSize,该属性来自ArrayList的size属性
When we load for the first time, the paging data of the first page is loaded:
It is worth noting that
In the past, I wrote the sql statement like this to get the total number of items:
select *from dbgoods ; //用Lits<goods> lists去存储 得到的数据,如果数据有几万条, //为了得到一个数字,去开辟这么大的空间,实属浪费 PageBean page=new PageBean(); page.setTotal=lists.size();
In fact, the correct way to open it is:
select count(*) from dbgoods where 1=1 ; //查询语句返回的是一个表的总记录数,int型, //where 1==1是为了查询搜索,做sql语句拼接
Synchronous implementation of asynchronous implementation, passing the currentpage parameters from the jsp interface to the servlet, the servlet obtains the parameters through the request request, and then passes the data to the jsp interface after querying the dao layer database.
The interface effect seen by the browser is: jsp--->servlet----->jsp (jump, poor user experience)
If there is a search box, When performing search paging, click the search button. When the query data is transferred to the jsp interface, the jsp is already a brand new page, and the text box content in the search box has disappeared. The solution is to change the value of the text box when clicking search. Also put it in the request request, send it to the servlet together, and then pass it to the new jsp through the servlet (super cumbersome)
Two solutions:
(1) Make two interfaces the same, one is used for paging when all data is displayed. When the query is clicked, another page is used after getting the data (the click event on the next page is to perform the search) ) to display
(2) Use session: When you click on the search query, record the search conditions in the session. When you click on the next page, judge the value of the session. If it is empty, execute all data On the next page, if it is not empty, the value of the session is taken out and used as the query condition. The next page executes the query statement with the search conditions. Trouble: Session destruction is difficult to control and prone to bugs
In short, using synchronization to implement paging will cause all kinds of unhappiness
Ajax asynchronous paging
//jsp界面一个函数,传递查询页码,绘制表格 function InitTable(currentpage) { $.ajax({ type:"get", url:"CustomServlet?type=search¤tpage="+currentpage, async:true, dataType:"json", success:function(data) { DrawTable(data); //绘制表格 } }); } function DrawTable(data) //根据传递过来的json绘制表格 { //给总页数赋值 $("#custom_all").text(data.pagelist.total); //给当前页赋值 $("#custom_currunt_page").text(data.pagelist.pageNum); //给总页数赋值 $("#custom_all_page").text(data.pagelist.pages); var _th="<th><input id='cb_all' type='checkbox'></th>" +"<th>ID</th>" +"<th>客户名称</th>" +"<th>公司名称</th>" +"<th>联系人</th>" +"<th>性别</th>" +"<th>联系电话</th>" +"<th>手机</th>" +"<th>QQ</th>" +"<th>电子邮箱</th>" +"<th>通讯地址</th>" +"<th>创建时间</th>"; document.getElementsByTagName("tbody")[0].innerHTML=_th; for(var i=0;i<data.pagelist.list.length;i++) { var customerCreatetime= format(data.pagelist.list[i].customerCreatetime, 'yyyy-MM-dd'); var _tr=document.createElement('tr'); msg="<td><input type='checkbox'></td><td>"+data.pagelist.list[i].customerId+"</td><td>"+data.pagelist.list[i].customerName+"</td><td>"+data.pagelist.list[i].customerCompanyname+"</td><td>"+data.pagelist.list[i].customerContactname+"</td><td>"+data.pagelist.list[i].customerSex+"</td><td>"+data.pagelist.list[i].customerTelephone+"</td><td>"+data.pagelist.list[i].customerPhone+"</td><td>"+data.pagelist.list[i].customerQq+"</td><td>"+data.pagelist.list[i].customerEmail+"</td><td>"+data.pagelist.list[i].customerAddress+"</td><td>"+customerCreatetime+"</td>" _tr.innerHTML=msg; document.getElementsByTagName("tbody")[0].appendChild(_tr); } }
When loading for the first time, the default call is
//初始化表格 InitTable(1);
It is worth noting that the key point is:
When we search, we define a global variable mydata with a scope of page
var mydata="";
Let’s look at the event code of clicking the search button
btns.eq(1).click( //搜索按钮点击事件 function() { //custom_dialog_form是搜索的form表单,将其搜索条件序列化后赋值给一个全局变量 mydata=$("#custom_dialog_form").serialize(); $.ajax({ type:"post", url:"CustomServlet?type=search¤tpage=1", async:true, dataType:"json", data:mydata, //传递数据 success:function(data) { DrawTable(data); $("#custom_dialog").css("display","none"); } }); } );
Solve the problem that the next page in the case of synchronized search accesses the next page of all data:
function InitTable(currentpage) //无搜索条件下的查询,传递一个页码 { $.ajax({ type:"get", url:"CustomServlet?type=search¤tpage="+currentpage, async:true, dataType:"json", success:function(data) { DrawTable(data); } }); } function InitTableSearch(currentpage)//有搜索添加的查询,传递页码 { $.ajax({ type:"post", url:"CustomServlet?type=search¤tpage="+currentpage, async:true, dataType:"json", data:mydata, success:function(data) { DrawTable(data); $("#custom_dialog").css("display","none"); } }); } //下一页 $("#custom_btn_next").click( function () { var currentpage=$("#custom_currunt_page").text(); //获取页面的当前页的值 var pages=$("#custom_all_page").text(); //获取总页数 currentpage++; if(currentpage<=pages) { if(mydata=="") //判断全局变量mydata是否为空,空表示没有进行搜索查询 { InitTable(currentpage); } else { InitTableSearch(currentpage); //进行条件搜索 } } });
Because it is Asynchronous refresh, so the global variable mydata has a value. If you manually refresh the page and reload it, mydata will be initialized to empty, and the unconditional search statement will be executed by default. Cleverly solves the problem of searching and displaying all the next pages. The same goes for the previous page, home page and last page.
The above is the detailed content of JavaScript implements paging function. For more information, please follow other related articles on the PHP Chinese website!
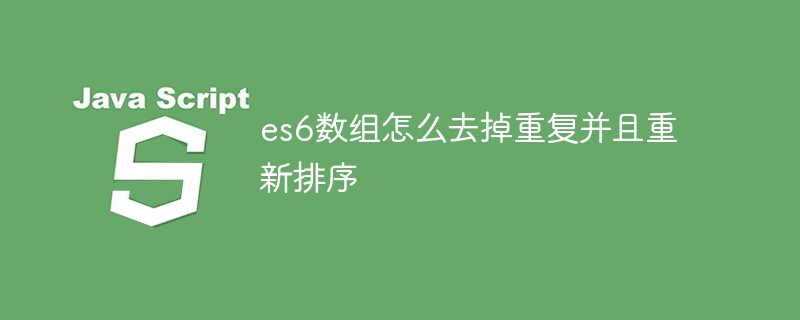
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
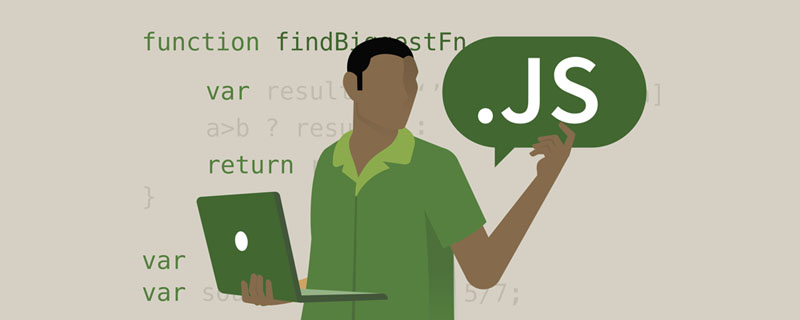
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
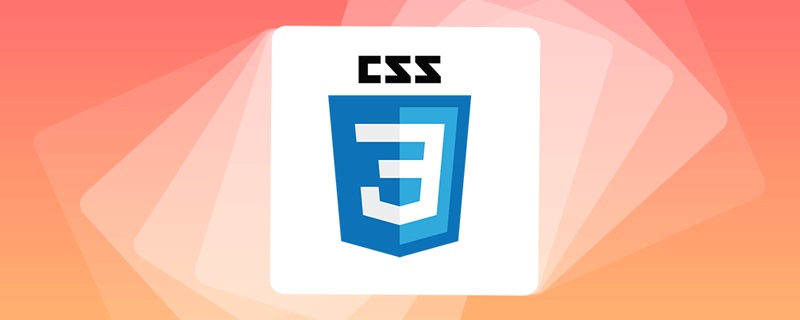
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
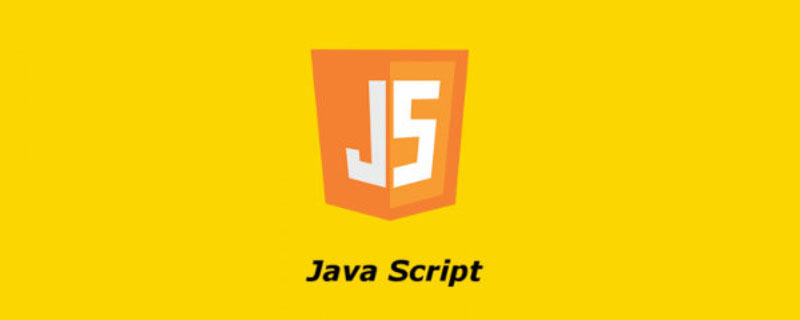
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
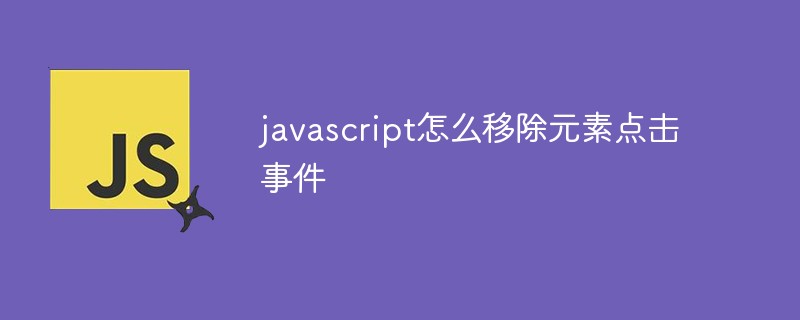
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
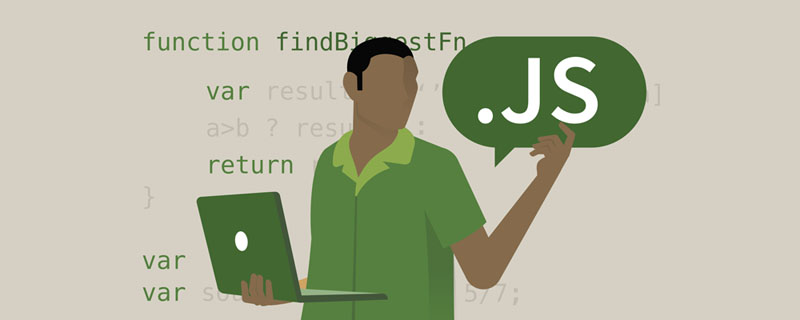
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
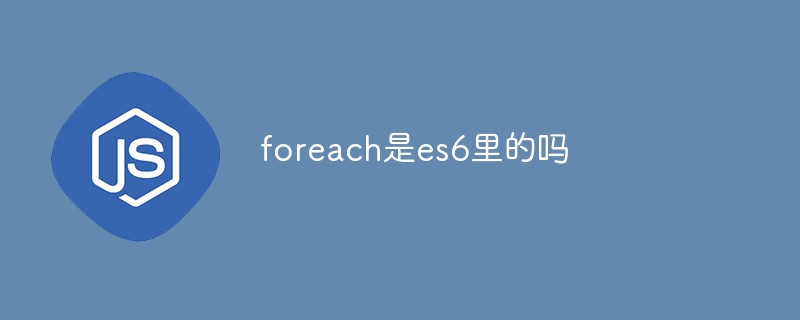
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。
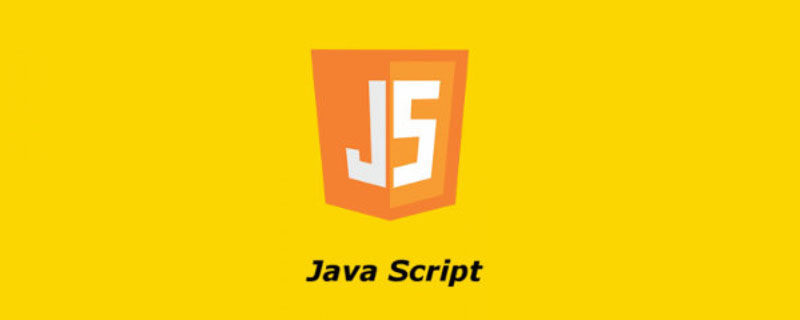
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
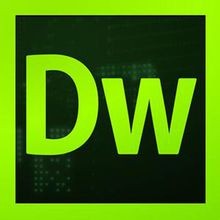
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
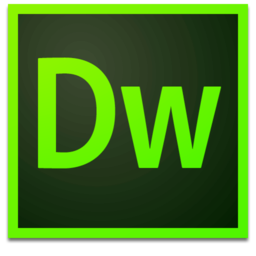
Dreamweaver Mac version
Visual web development tools