There are three commonly used methods to traverse arrays in PHP:
1. Use the for statement to loop through the array;
2 , use the foreach statement to traverse the array;
3. Use list(), each() and while loop in combination to traverse the array.
The most efficient of these three methods is to use the foreach statement to traverse the array. The foreach structure has been introduced since PHP4. It is a statement specially designed for traversing arrays in PHP. It is recommended that everyone use it. Let’s first introduce these methods respectively.
1. Use the for statement to loop through an array
It is worth noting that using the for statement to loop through an array requires that the array traversed must be an index array. There are not only associative arrays but also index arrays in PHP, so the for statement is rarely used in PHP to loop through arrays.
The example code is as follows:
<?php header("Content-Type: text/html; charset=utf-8"); $arr = array('https://www.php.cn','php中文网','PHP教程'); $num = count($arr); for($i=0;$i<$num;++$i){ echo $arr[$i].'<br />'; } ?>
Note: In the above example code, we first calculate the number of elements in the array $arr, and then use it in the for statement. This is very efficient. Because if it is for($i=0;$i
The output result of the above code is:
https://www.php.cn php中文网 PHP教程
2. Use the foreach statement to traverse the array
There are two ways to use the foreach statement to loop through the array There are two ways, and the one we use most is still the first way. The introduction is as follows:
The first way:
foreach(array_expression as $value){ //循环体 }
Example code:
<?php header("Content-Type: text/html; charset=utf-8"); $arr = array('https://www.php.cn','php中文网','PHP教程'); foreach($arr as $value){ echo $value.'<br />'; } ?>
In each loop, the value of the current element is assigned to the variable $value, and the array The internal pointer moves back one step. Therefore, the next element of the array will be obtained in the next loop, and the loop will not stop until the end of the array, ending the traversal of the array.
Second method:
foreach(array_expression as $key=>$value){ //循环体 }
Instance code:
<?php header("Content-Type: text/html; charset=utf-8"); $arr = array('https://www.php.cn','php中文网','PHP教程'); foreach($arr as $k=>$v){ echo $k."=>".$v."<br />"; } ?>
Output:
0=>https://www.php.cn 1=>php中文网 2=>PHP教程
3. Combined use list(), each() and while loops traverse the array
each() function needs to pass an array as a parameter, returns the key/value pair of the current element in the array, and moves the array pointer backward to the position of the next element.
list() function, this is not a real function, it is a language structure of PHP. list() assigns values to a set of variables in one step.
Example code:
<?php header("Content-Type: text/html; charset=utf-8"); //定义循环的数组 $arr = array('website'=>'https://www.php.cn','webname'=>'php中文网'); while(list($k,$v) = each($arr)){ echo $k.'=>'.$v.'<br />'; } ?>
Output:
website=>https://www.php.cn webname=>php中文网
For more related knowledge, please visit PHP Chinese website! !
The above is the detailed content of How to traverse an array in php?. For more information, please follow other related articles on the PHP Chinese website!
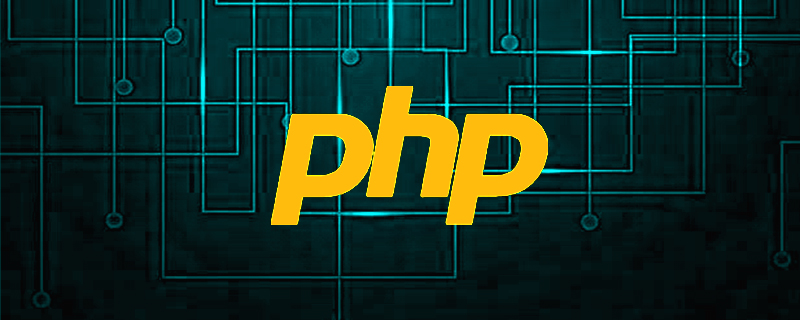
php把负数转为正整数的方法:1、使用abs()函数将负数转为正数,使用intval()函数对正数取整,转为正整数,语法“intval(abs($number))”;2、利用“~”位运算符将负数取反加一,语法“~$number + 1”。
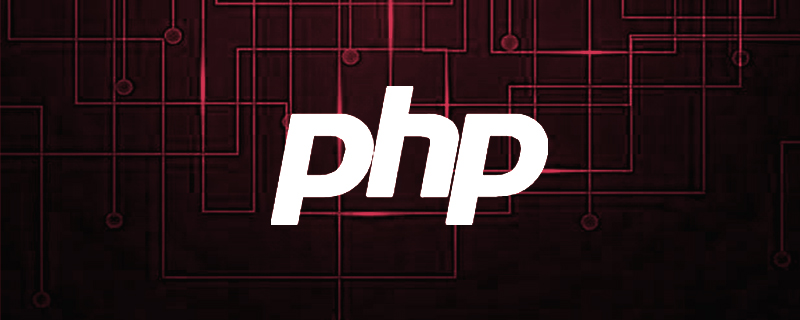
实现方法:1、使用“sleep(延迟秒数)”语句,可延迟执行函数若干秒;2、使用“time_nanosleep(延迟秒数,延迟纳秒数)”语句,可延迟执行函数若干秒和纳秒;3、使用“time_sleep_until(time()+7)”语句。
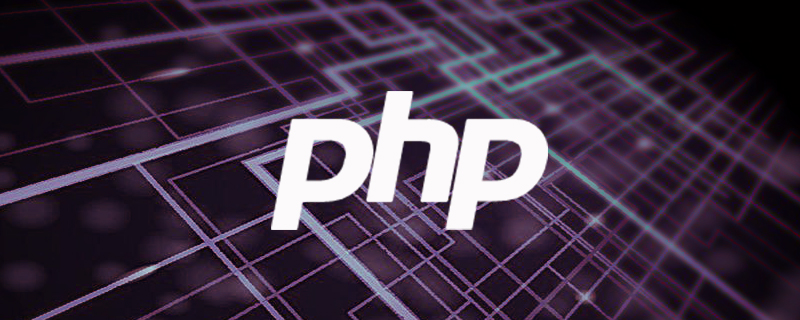
php字符串有下标。在PHP中,下标不仅可以应用于数组和对象,还可应用于字符串,利用字符串的下标和中括号“[]”可以访问指定索引位置的字符,并对该字符进行读写,语法“字符串名[下标值]”;字符串的下标值(索引值)只能是整数类型,起始值为0。
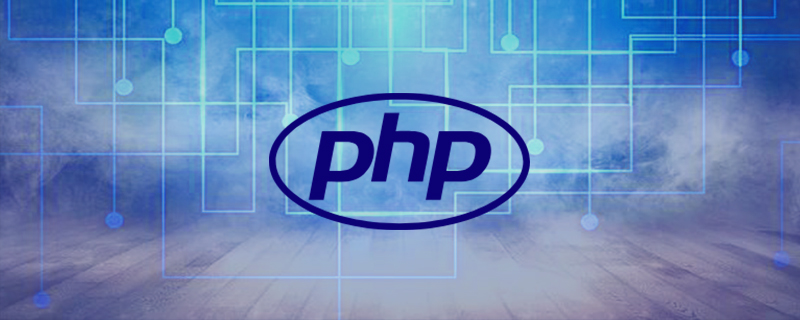
php除以100保留两位小数的方法:1、利用“/”运算符进行除法运算,语法“数值 / 100”;2、使用“number_format(除法结果, 2)”或“sprintf("%.2f",除法结果)”语句进行四舍五入的处理值,并保留两位小数。
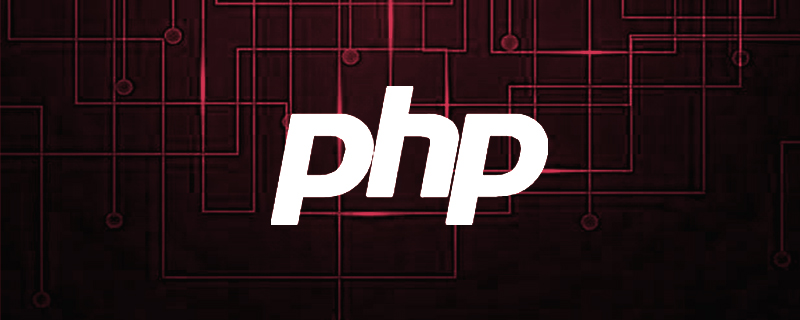
判断方法:1、使用“strtotime("年-月-日")”语句将给定的年月日转换为时间戳格式;2、用“date("z",时间戳)+1”语句计算指定时间戳是一年的第几天。date()返回的天数是从0开始计算的,因此真实天数需要在此基础上加1。
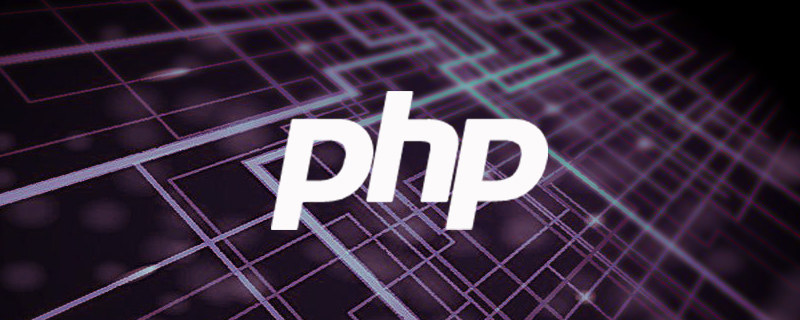
在php中,可以使用substr()函数来读取字符串后几个字符,只需要将该函数的第二个参数设置为负值,第三个参数省略即可;语法为“substr(字符串,-n)”,表示读取从字符串结尾处向前数第n个字符开始,直到字符串结尾的全部字符。
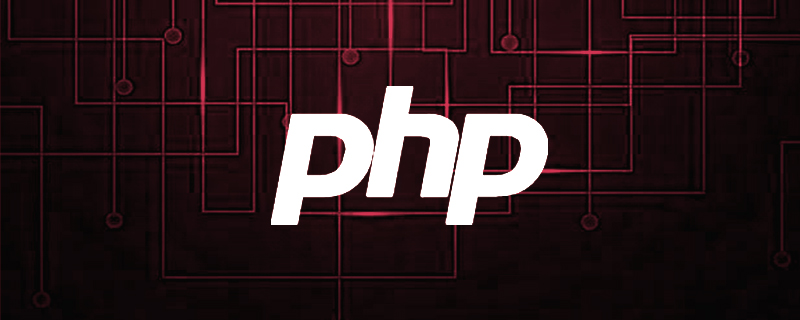
方法:1、用“str_replace(" ","其他字符",$str)”语句,可将nbsp符替换为其他字符;2、用“preg_replace("/(\s|\ \;||\xc2\xa0)/","其他字符",$str)”语句。
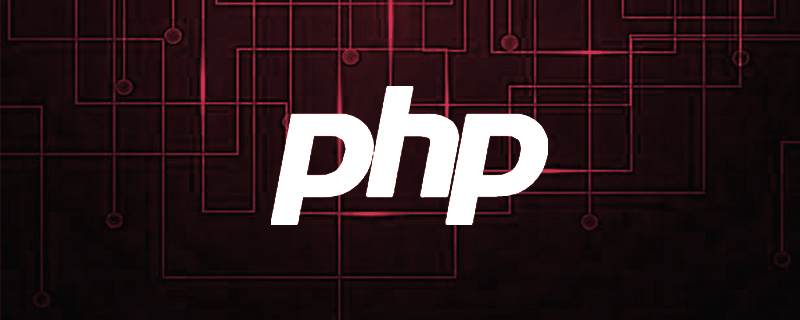
php判断有没有小数点的方法:1、使用“strpos(数字字符串,'.')”语法,如果返回小数点在字符串中第一次出现的位置,则有小数点;2、使用“strrpos(数字字符串,'.')”语句,如果返回小数点在字符串中最后一次出现的位置,则有。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
