


Mixin is an idea that uses partially implemented interfaces to achieve code reuse. It can be used to solve the problem of multiple inheritance and can be used to extend functions. The following article mainly introduces you to the relevant information about adding mixin extensions to WeChat mini programs. Friends in need can refer to it. Let’s take a look together.
Introduction to Mixin
Mixin (weaving) mode is not one of GOF’s “Design Patterns” summary, but it is used in various Languages and frameworks will find some application of this pattern (or idea). Simply put, Mixin is an interface with full or partial implementation, and its main function is better code reuse.
The concept of Mixin is supported in React and Vue. It provides convenience for us to abstract business logic and code reuse. However, the native mini program framework does not directly support Mixin. Let’s first look at a very practical requirement:
Add a running environment class to all mini program pages to facilitate some style hacks. Specifically, when the mini program is run in different operating environments (Developer Tools|iOS|Android), the platform value is the corresponding operating environment value ("ios|android|devtools")
<view class="{{platform}}"> <!--页面模板--> </view>
Reviewing the use of mixin in vue
The problems mentioned at the beginning of the article are very suitable to be solved using Mixin. We converted this requirement into a Vue problem: add a platform style class to each routing page (although this may not be practical). The implementation idea is to add a data: platform
to each routing component. The code is implemented as follows:
// mixins/platform.js const getPlatform = () => { // 具体实现略,这里mock返回'ios' return 'ios'; }; export default { data() { return { platform: getPlatform() } } }
// 在路由组件中使用 // views/index.vue import platform from 'mixins/platform'; export default { mixins: [platform], // ... }
// 在路由组件中使用 // views/detail.vue import platform from 'mixins/platform'; export default { mixins: [platform], // ... }
In this way, in the index and detail routing pages The viewModel has the platform value, which can be used directly in the template.
mixin classification in vue
data mixin
- ##normal method mixin
- lifecycle method mixin
export default { data () { return { platform: 'ios' } }, methods: { sayHello () { console.log(`hello!`) } }, created () { console.log(`lifecycle3`) } }
Mixin merging and execution strategies in vue
If there are duplications between mixins, these mixins will have specific merging and execution strategies. As shown below:How to make the applet support mixin
In front, we reviewed vue Knowledge about mixins. Now we need to make the mini program also support mixin and realize the same mixin function in vue.Implementation ideas
Let’s first take a look at the official mini program page registration method:Page({ data: { text: "This is page data." }, onLoad: function(options) { // Do some initialize when page load. }, onReady: function() { // Do something when page ready. }, onShow: function() { // Do something when page show. }, onHide: function() { // Do something when page hide. }, onUnload: function() { // Do something when page close. }, customData: { hi: 'MINA' } })If we Adding mixin configuration, the above official registration method will become:
Page({ mixins: [platform], data: { text: "This is page data." }, onLoad: function(options) { // Do some initialize when page load. }, onReady: function() { // Do something when page ready. }, onShow: function() { // Do something when page show. }, onHide: function() { // Do something when page hide. }, onUnload: function() { // Do something when page close. }, customData: { hi: 'MINA' } })
There are two points here that we should pay special attention to:
Page(configObj)
- Configure the data, method, lifecycle, etc. of the applet page through configObj
- onLoad method - page main entrance
Page(). In fact,
Page(configObj) is an ordinary function call. We add an intermediate method:
Page(createPage(configObj))In the createPage method, we can preprocess The mixin in configObj merges the configuration into configObj in the correct way, and finally hands it to
Page() . This is the idea of implementing mixins.
Specific implementation
The specific code implementation will not be described in detail. You can see the following code. For more detailed code implementation, more extensions, and tests, please refer to github/** * 为每个页面提供mixin,page invoke桥接 */ const isArray = v => Array.isArray(v); const isFunction = v => typeof v === 'function'; const noop = function () {}; // 借鉴redux https://github.com/reactjs/redux function compose(...funcs) { if (funcs.length === 0) { return arg => arg; } if (funcs.length === 1) { return funcs[0]; } const last = funcs[funcs.length - 1]; const rest = funcs.slice(0, -1); return (...args) => rest.reduceRight((composed, f) => f(composed), last(...args)); } // 页面堆栈 const pagesStack = getApp().$pagesStack; const PAGE_EVENT = ['onLoad', 'onReady', 'onShow', 'onHide', 'onUnload', 'onPullDownRefresh', 'onReachBottom', 'onShareAppMessage']; const APP_EVENT = ['onLaunch', 'onShow', 'onHide', 'onError']; const onLoad = function (opts) { // 把pageModel放入页面堆栈 pagesStack.addPage(this); this.$invoke = (pagePath, methodName, ...args) => { pagesStack.invoke(pagePath, methodName, ...args); }; this.onBeforeLoad(opts); this.onNativeLoad(opts); this.onAfterLoad(opts); }; const getMixinData = mixins => { let ret = {}; mixins.forEach(mixin => { let { data={} } = mixin; Object.keys(data).forEach(key => { ret[key] = data[key]; }); }); return ret; }; const getMixinMethods = mixins => { let ret = {}; mixins.forEach(mixin => { let { methods={} } = mixin; // 提取methods Object.keys(methods).forEach(key => { if (isFunction(methods[key])) { // mixin中的onLoad方法会被丢弃 if (key === 'onLoad') return; ret[key] = methods[key]; } }); // 提取lifecycle PAGE_EVENT.forEach(key => { if (isFunction(mixin[key]) && key !== 'onLoad') { if (ret[key]) { // 多个mixin有相同lifecycle时,将方法转为数组存储 ret[key] = ret[key].concat(mixin[key]); } else { ret[key] = [mixin[key]]; } } }) }); return ret; }; /** * 重复冲突处理借鉴vue: * data, methods会合并,组件自身具有最高优先级,其次mixins中后配置的mixin优先级较高 * lifecycle不会合并。先顺序执行mixins中的lifecycle,再执行组件自身的lifecycle */ const mixData = (minxinData, nativeData) => { Object.keys(minxinData).forEach(key => { // page中定义的data不会被覆盖 if (nativeData[key] === undefined) { nativeData[key] = minxinData[key]; } }); return nativeData; }; const mixMethods = (mixinMethods, pageConf) => { Object.keys(mixinMethods).forEach(key => { // lifecycle方法 if (PAGE_EVENT.includes(key)) { let methodsList = mixinMethods[key]; if (isFunction(pageConf[key])) { methodsList.push(pageConf[key]); } pageConf[key] = (function () { return function (...args) { compose(...methodsList.reverse().map(f => f.bind(this)))(...args); }; })(); } // 普通方法 else { if (pageConf[key] == null) { pageConf[key] = mixinMethods[key]; } } }); return pageConf; }; export default pageConf => { let { mixins = [], onBeforeLoad = noop, onAfterLoad = noop } = pageConf; let onNativeLoad = pageConf.onLoad || noop; let nativeData = pageConf.data || {}; let minxinData = getMixinData(mixins); let mixinMethods = getMixinMethods(mixins); Object.assign(pageConf, { data: mixData(minxinData, nativeData), onLoad, onBeforeLoad, onAfterLoad, onNativeLoad, }); pageConf = mixMethods(mixinMethods, pageConf); return pageConf; };
Summary
1 , This article mainly talks about how to add mixin support to small programs. The implementation idea is: preprocessing configObjPage(createPage(configObj))2. When dealing with mixin duplication, be consistent with vue: data, methods will be merged , the component itself has the highest priority, and the mixin configured later in the mixins has a higher priority.
Summarize
The above is the detailed content of Detailed explanation on adding mixin extensions in WeChat applet development. For more information, please follow other related articles on the PHP Chinese website!
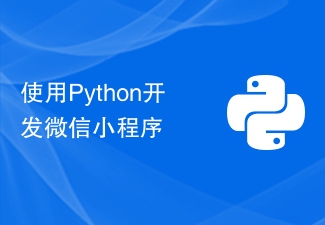
随着移动互联网技术和智能手机的普及,微信成为了人们生活中不可或缺的一个应用。而微信小程序则让人们可以在不需要下载安装应用的情况下,直接使用小程序来解决一些简单的需求。本文将介绍如何使用Python来开发微信小程序。一、准备工作在使用Python开发微信小程序之前,需要安装相关的Python库。这里推荐使用wxpy和itchat这两个库。wxpy是一个微信机器
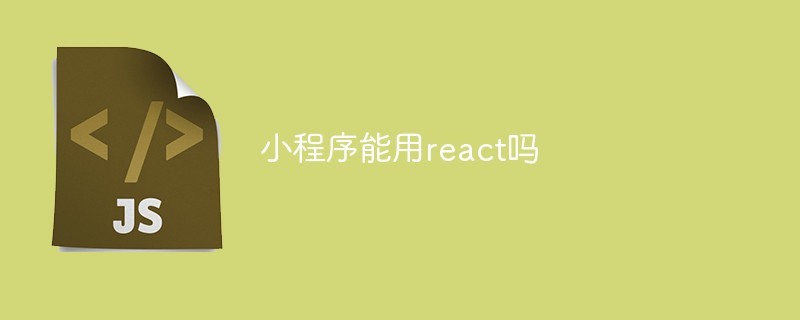
小程序能用react,其使用方法:1、基于“react-reconciler”实现一个渲染器,生成一个DSL;2、创建一个小程序组件,去解析和渲染DSL;3、安装npm,并执行开发者工具中的构建npm;4、在自己的页面中引入包,再利用api即可完成开发。
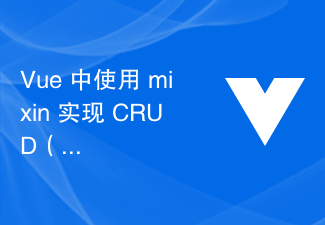
Vue中的mixin是一个非常有用的特性,它可以将一些可重用的代码封装在一个mixin对象中,然后在需要使用这些代码的组件中使用mixin进行引入。这种方法大大提高了代码的可复用性和可维护性,特别是在一些重复的CRUD(增删改查)操作中。本文将介绍如何使用mixin在Vue中实现CRUD操作的技巧。首先,我们需要了解如何创建一个
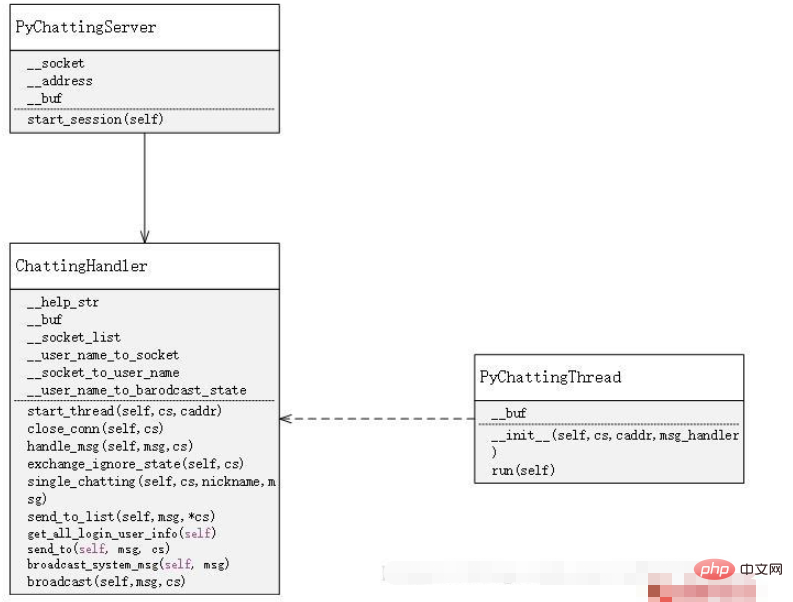
实现思路x01服务端的建立首先,在服务端,使用socket进行消息的接受,每接受一个socket的请求,就开启一个新的线程来管理消息的分发与接受,同时,又存在一个handler来管理所有的线程,从而实现对聊天室的各种功能的处理x02客户端的建立客户端的建立就要比服务端简单多了,客户端的作用只是对消息的发送以及接受,以及按照特定的规则去输入特定的字符从而实现不同的功能的使用,因此,在客户端这里,只需要去使用两个线程,一个是专门用于接受消息,一个是专门用于发送消息的至于为什么不用一个呢,那是因为,只
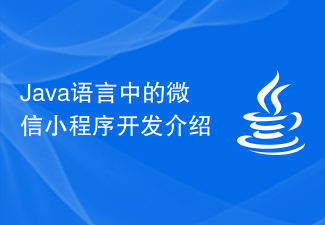
微信小程序是一种轻量级的应用程序,可以在微信平台上运行,不需要下载安装,方便快捷。Java语言作为一种广泛应用于企业级应用开发的语言,也可以用于微信小程序的开发。在Java语言中,可以使用SpringBoot框架和第三方工具包来开发微信小程序。下面是一个简单的微信小程序开发过程。创建微信小程序首先,需要在微信公众平台上注册一个小程序。注册成功后,可以获取到
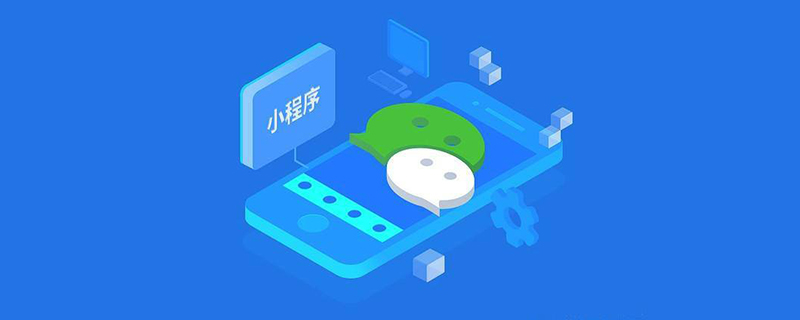
本篇文章给大家带来了关于微信小程序的相关问题,其中主要介绍了如何在小程序中用公众号模板消息,下面一起来看一下,希望对大家有帮助。
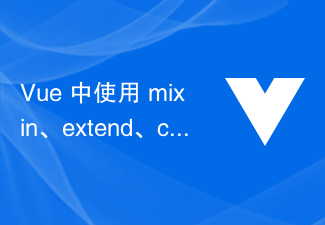
Vue.js是一个流行的前端框架,它提供了许多API用于组件的定制。本文将介绍Vue中mixin、extend、component等API,帮助您掌握组件定制的技巧。mixinmixin是Vue中重用组件代码的一种方式。它允许我们将已经编写的代码复用到不同的组件中,从而减少重复代码的编写。例如,我们可以使用mixin帮助我们在多个组
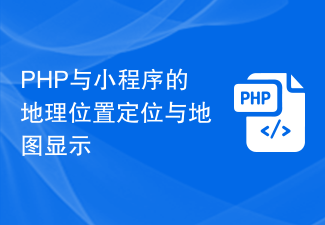
PHP与小程序的地理位置定位与地图显示地理位置定位与地图显示在现代科技中已经成为了必备的功能之一。随着移动设备的普及,人们对于定位和地图显示的需求也越来越高。在开发过程中,PHP和小程序是常见的两种技术选择。本文将为大家介绍PHP与小程序中的地理位置定位与地图显示的实现方法,并附上相应的代码示例。一、PHP中的地理位置定位在PHP中,我们可以使用第三方地理位


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
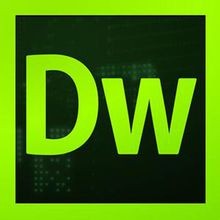
Dreamweaver CS6
Visual web development tools
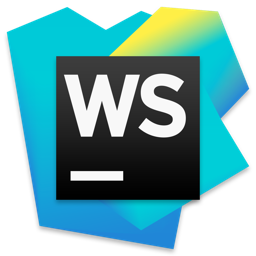
WebStorm Mac version
Useful JavaScript development tools