This article mainly introduces whether Go has parameter passing by reference (compared to C++). Friends who need it can refer to
Three parameter passing methods in C++
Value passing:
The most common method of passing parameters. The formal parameters of the function are copies of the actual parameters. Changing the formal parameters in the function will not affect to formal parameters outside the function. Generally, value passing is used when modifying parameters within a function without affecting the caller.
Pointer passing
The formal parameter is a pointer pointing to the address of the actual parameter. As the name suggests, when the content pointed to by the formal parameter is manipulated in the function, the actual parameter itself will be modified. .
Passing by reference
In C++, a reference is an alias for a variable. It is actually the same thing and has the same address in memory. In other words, wherever a reference is manipulated, the referenced variable is quite directly manipulated.
Look at the demo below:
#include <iostream> //值传递 void func1(int a) { std::cout << "值传递,变量地址:" << &a << ", 变量值:" << a << std::endl; a ++ ; } //指针传递 void func2 (int* a) { std::cout << "指针传递,变量地址:" << a << ", 变量值:" << *a << std::endl; *a = *a + 1; } //引用传递 void func3 (int& a) { std::cout << "指针传递,变量地址:" << &a << ", 变量值:" << a << std::endl; a ++; } int main() { int a = 5; std::cout << "变量实际地址:" << &a << ", 变量值:" << a << std::endl; func1(a); std::cout << "值传递操作后,变量值:" << a << std::endl; std::cout << "变量实际地址:" << &a << ", 变量值:" << a << std::endl; func2(&a); std::cout << "指针传递操作后,变量值:" << a << std::endl; std::cout << "变量实际地址:" << &a << ", 变量值:" << a << std::endl; func3(a); std::cout << "引用传递操作后,变量值:" << a << std::endl; return 0; }
The output results are as follows:
Actual address of variable: 0x28feac, variable value: 5
value Transfer, variable address: 0x28fe90, variable value: 5
After the value transfer operation, variable value: 5
Actual variable address: 0x28feac, variable value: 5
Pointer transfer, variable address: 0x28feac, variable value: 5
After the pointer transfer operation, the variable value: 6
The actual address of the variable: 0x28feac, the variable value: 6
The pointer transfer operation, the variable address: 0x28feac, the variable value: 6
After the reference transfer operation, the variable Value: 7
Parameter passing in Go
The above introduces the three parameter passing methods of C++, value passing and pointer Passing is easy to understand, so does Go also have these parameter passing methods? This has caused controversy, but compared to the concept of reference passing in C++, we can say that Go has no reference passing method. Why do I say this, because Go does not have the concept of variable references. But Go has reference types, which will be explained later.
Let’s first look at an example of passing value and pointer in Go:
package main import ( "fmt" ) func main() { a := 1 fmt.Println( "变量实际地址:", &a, "变量值:", a) func1 (a) fmt.Println( "值传递操作后,变量值:", a) fmt.Println( "变量实际地址:", &a, "变量值:", a) func2(&a) fmt.Println( "指针传递操作后,变量值:", a) } //值传递 func func1 (a int) { a++ fmt.Println( "值传递,变量地址:", &a, "变量值:", a) } //指针传递 func func2 (a *int) { *a = *a + 1 fmt.Println( "指针传递,变量地址:", a, "变量值:", *a) }
The output result is as follows:
The actual address of the variable: 0xc04203c1d0 variable Value: 1
Value transfer, variable address: 0xc04203c210 Variable value: 2
After value transfer operation, variable value: 1
Actual variable address: 0xc04203c1d0 Variable value: 1
Pointer transfer, variable address: 0xc04203c1d0 Variable value: 2
After the pointer transfer operation, variable value: 2
It can be seen that the value transfer and pointer transfer of Go's basic types are no different from C++, but it does not have the concept of variable reference. So how do you understand Go's reference types?
Go’s reference types
In Go, reference types include slices, dictionaries, channels, etc. Take slicing as an example. Is passing a slice a reference?
For example:
package main import ( "fmt" ) func main() { m1 := make([]string, 1) m1[0] = "test" fmt.Println("调用 func1 前 m1 值:", m1) func1(m1) fmt.Println("调用 func1 后 m1 值:", m1) } func func1 (a []string) { a[0] = "val1" fmt.Println("func1中:", a) }
The output result is as follows:
The value of m1 before calling func1: [test]
## In #func1: [val1]The value of m1 after calling func1: [val1]The modification to the slice in the function affects the value of the actual parameter. Does this mean that this is pass-by-reference? In fact, not really. To answer this question, we must first find out whether the calling function slice m1 has changed. First we need to understand the nature of slicing. A slice is a description of an array fragment. It contains a pointer to the array, the length of the fragment. In other words, what we print above is not the slice itself, but the array pointed to by the slice. For another example, verify whether the slice has changed.package main import ( "fmt" ) func main() { m1 := make([]string, 1) m1[0] = "test" fmt.Println("调用 func1 前 m1 值:", m1, cap(m1)) func1(m1) fmt.Println("调用 func1 后 m1 值:", m1, cap(m1)) } func func1 (a []string) { a = append(a, "val1") fmt.Println("func1中:", a, cap(a)) }The output results are as follows: The value of m1 before calling func1: [test] 1In func1: [test val1] 2 The value of m1 after calling func1: [test] 1This result shows that the slices have not changed before and after the call. The so-called "change" in the previous example is actually that the elements of the array pointed to by the pointer to the array in the slice have changed. This sentence may be a bit awkward, but it is actually true. Proving once again that parameter passing of reference types is not pass-by-reference. I want to have a thorough understanding. A slice is a description of an array fragment. It contains the pointer to the array and the length of the fragment. If you are interested, you can read this article: http://www.jb51.net/kf/201604/499045.html. Learn about the memory model of slicing.
Summary
The summary is very simple, and language also needs to see through the phenomenon to see the essence. There is also the conclusion of this article that needs to be remembered: There is no pass-by-reference in Go.The above is the detailed content of Go language comparison C++ reference parameter passing. For more information, please follow other related articles on the PHP Chinese website!
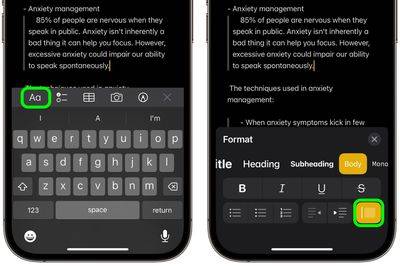
在iOS17和macOSSonoma中,Apple为AppleNotes添加了新的格式选项,包括块引号和新的Monostyle样式。以下是使用它们的方法。借助AppleNotes中的其他格式选项,您现在可以在笔记中添加块引用。块引用格式可以轻松地使用文本左侧的引用栏直观地偏移部分的写作。只需点击/单击“Aa”格式按钮,然后在键入之前或当您在要转换为块引用的行上时选择块引用选项。该选项适用于所有文本类型、样式选项和列表,包括清单。在同一“格式”菜单中,您可以找到新的“单样式”选项。这是对先前“等宽
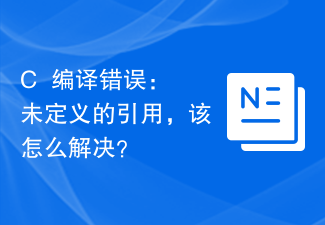
C++是一门广受欢迎的编程语言,但是在使用过程中,经常会出现“未定义的引用”这个编译错误,给程序的开发带来了诸多麻烦。本篇文章将从出错原因和解决方法两个方面,探讨“未定义的引用”错误的解决方法。一、出错原因C++编译器在编译一个源文件时,会将它分为两个阶段:编译阶段和链接阶段。编译阶段将源文件中的源码转换为汇编代码,而链接阶段将不同的源文件合并为一个可执行文
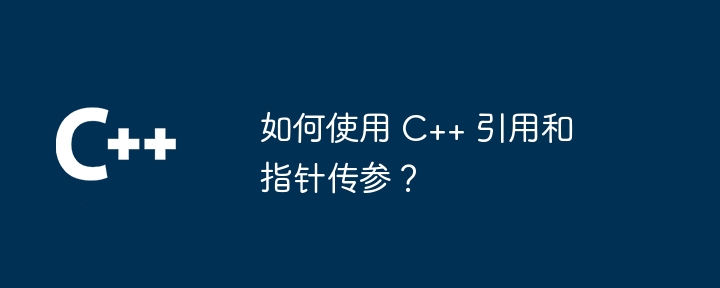
C++中引用和指针都是传递函数参数的方法,但有区别。引用是变量的别名,修改引用会修改原始变量,而指针存储变量的地址,修改指针值不会修改原始变量。在选择使用引用还是指针时,需要考虑是否需要修改原始变量、是否需要传递空值和性能考虑等因素。
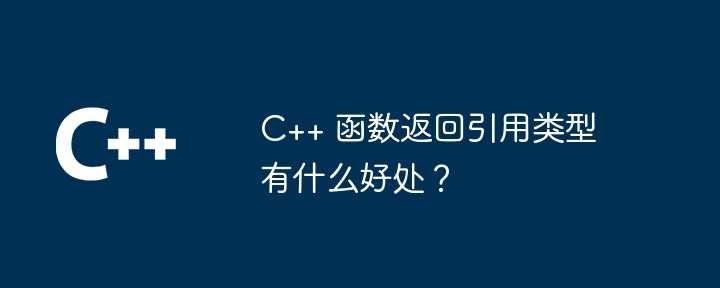
C++中的函数返回引用类型的好处包括:性能提升:引用传递避免了对象复制,从而节省了内存和时间。直接修改:调用方可以直接修改返回的引用对象,而无需重新赋值。代码简洁:引用传递简化了代码,无需额外的赋值操作。
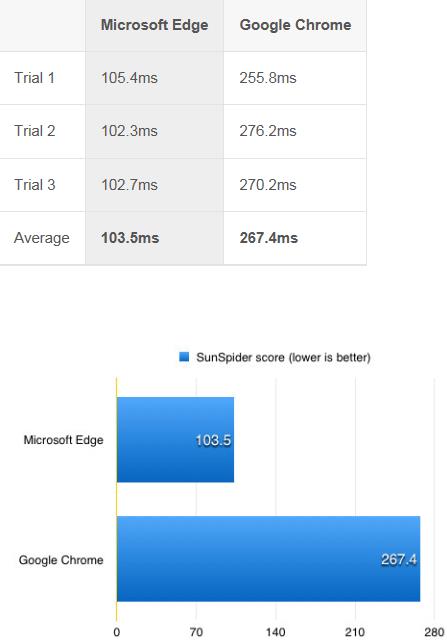
谷歌浏览器一直都是很多小伙伴的首选浏览器,但是微软更新了新版的edge浏览器也很吸引人,那么这两款浏览器到底谁比较好呢?下面就一起来看看详情介绍吧。edge浏览器chrome哪个好:答:新版的edge浏览器更好一点。在使用JavaScript测试后,edge浏览器以平均值103.5击败了谷歌Chrome,这说明它能够更好地处理开发者遇到的性能问题。edge浏览器和chrome浏览器的优点:edge浏览器:1、速度更快edge浏览器已经比之前的版本快了很多,根据CNet测试,edge浏览器要比Fi
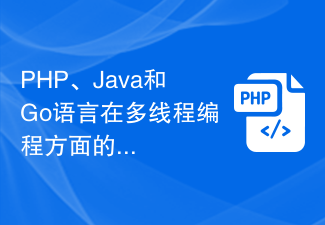
PHP、Java和Go语言在多线程编程方面的对比在现代软件开发中,多线程编程已经成为一种常见的需求。随着互联网规模的不断扩大和多核处理器的广泛应用,开发者们迫切需要一种高效且易于使用的方式来处理并发任务。在这篇文章中,我们将对比三种流行的编程语言:PHP、Java和Go,在多线程编程方面的优劣势。PHP是一门常用于网页开发的脚本语言,具有简单易学、开发快速的
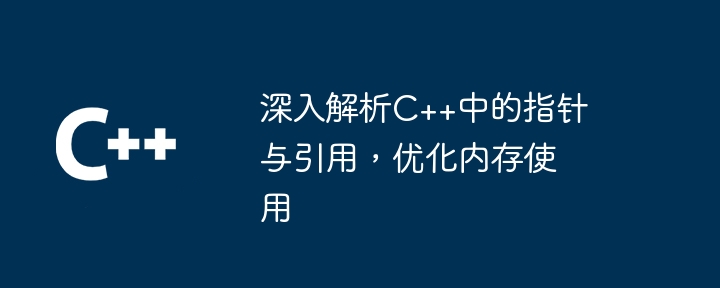
通过使用指针和引用,可以优化C++中的内存使用:指针:存储其他变量地址,可指向不同变量,节约内存,但可能产生野指针。引用:别名为另一个变量,始终指向同一个变量,不会产生野指针,适用于函数参数。通过避免不必要的复制、减少内存分配和节省空间,优化内存使用可以提升代码效率和性能。
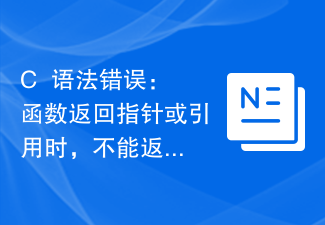
C++是一种面向对象的编程语言,它的灵活性和强大性通常为程序员提供了很大的帮助。然而,也正是因为其灵活性,编程时难以避免各种小错误。其中一个很常见的错误就是函数返回指针或引用时,不能返回局部变量或临时对象。那么该如何处理这个问题呢?本文将详细介绍相关的内容。问题的原因在C++语言中,局部变量和临时对象是在函数运行期间动态分配的。当函数结束时,这些局部变量和临


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
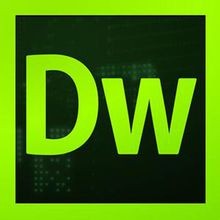
Dreamweaver CS6
Visual web development tools
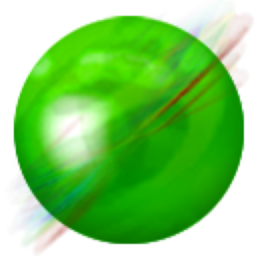
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
