


Detailed explanation of js cross-domain principle and two solutions_javascript skills
1. What is cross-domain
We often use ajax to request data from other servers on the page. At this time, cross-domain problems will occur on the client.
The cross-domain problem is caused by the same-origin policy in the JavaScript language security restrictions.
Simply put, the same-origin policy means that a script can only read the properties of windows and documents from the same source. The same source here refers to the combination of host name, protocol and port number.
For example:
2. Implementation principle
In the HTML DOM, the Script tag can access data on the server across domains. Therefore, the src attribute of the script can be specified as a cross-domain URL to achieve cross-domain access.
For example:
This access method is not possible. But the following method is possible.
There is a requirement for the returned data, that is: the data returned by the server cannot be simply {"Name":"zhangsan"}
If this json string is returned, we have no way to reference this string. Therefore, the returned value must be var json={"Name":"zhangsan"}, or json({"Name":"zhangsan"})
In order to prevent the program from reporting errors, we must also create a json function.
3. Solution
Option 1
Server side:
protected void Page_Load(object sender, EventArgs e) { string result = "callback({\"name\":\"zhangsan\",\"date\":\"2012-12-03\"})"; Response.Clear(); Response.Write(result); Response.End(); }
Client:
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title></title> <script type="text/javascript"> var result = null; window.onload = function () { var script = document.createElement("script"); script.type = "text/javascript"; script.src = "http://192.168.0.101/ExampleBusinessApplication.Web/web2.aspx"; var head = document.getElementsByTagName("head")[0]; head.insertBefore(script, head.firstChild); }; function callback(data) { result = data; } function b_click() { alert(result.name); } </script> </head> <body> <input type="button" value="click me!" onclick="b_click();" /> </body> </html>
Option 2: Complete through jquery
Through jquery’s jsonp method. Using this method has requirements for the server side.
The server side is as follows:
protected void Page_Load(object sender, EventArgs e) { string callback = Request.QueryString["jsoncallback"]; string result = callback + "({\"name\":\"zhangsan\",\"date\":\"2012-12-03\"})"; Response.Clear(); Response.Write(result); Response.End(); }
Client:
$.ajax({ async: false, url: "http://192.168.0.5/Web/web1.aspx", type: "GET", dataType: 'jsonp', //jsonp的值自定义,如果使用jsoncallback,那么服务器端,要返回一个jsoncallback的值对应的对象. jsonp: 'jsoncallback', //要传递的参数,没有传参时,也一定要写上 data: null, timeout: 5000, //返回Json类型 contentType: "application/json;utf-8", //服务器段返回的对象包含name,data属性. success: function (result) { alert(result.date); }, error: function (jqXHR, textStatus, errorThrown) { alert(textStatus); } });
Actually, when we execute this js, js sends such a request to the server:
http://192.168.0.5/Web/web1.aspx?jsoncallback=jsonp1354505244726&_=1354505244742
The server also returned the following object accordingly:
jsonp1354506338864({"name":"zhangsan","date":"2012-12-03"})
At this point, the requirements for cross-domain sample data are realized.
The above is an introduction to the js cross-domain principle and two solutions. I hope it will be helpful to everyone in learning cross-domain knowledge points. You can also combine it with other related articles for study and research.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
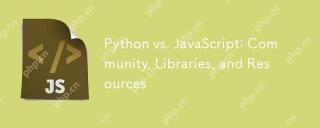
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.
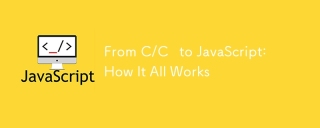
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
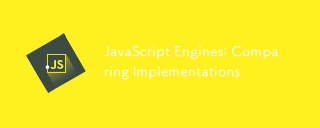
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
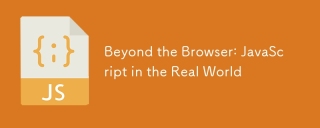
JavaScript's applications in the real world include server-side programming, mobile application development and Internet of Things control: 1. Server-side programming is realized through Node.js, suitable for high concurrent request processing. 2. Mobile application development is carried out through ReactNative and supports cross-platform deployment. 3. Used for IoT device control through Johnny-Five library, suitable for hardware interaction.
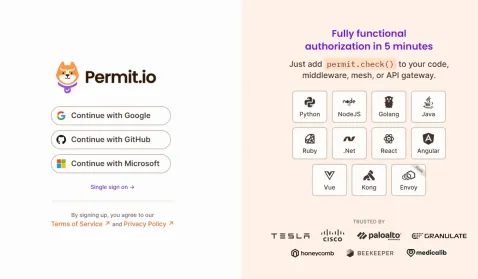
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
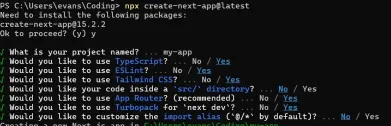
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
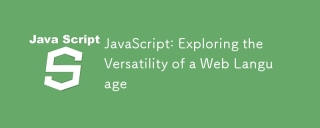
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
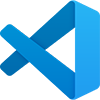
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.