When working on website projects, scripts are often used to generate sitemaps, which is convenient for crawlers and beneficial to SEO. So how to use Python to generate sitemap? Let’s study it below.
Install lxml
First you need pip install lxml to install the lxml library.
If you encounter the following error on ubuntu:
#include "libxml/xmlversion.h" compilation terminated. error: command 'x86_64-linux-gnu-gcc' failed with exit status 1 ---------------------------------------- Cleaning up... Removing temporary dir /tmp/pip_build_root... Command /usr/bin/python -c "import setuptools, tokenize;__file__='/tmp/pip_build_root/lxml/setup.py';exec(compile(getattr(tokenize, 'open', open)(__file__).read().replace('\r\n', '\n'), __file__, 'exec'))" install --record /tmp/pip-O4cIn6-record/install-record.txt --single-version-externally-managed --compile failed with error code 1 in /tmp/pip_build_root/lxml Exception information: Traceback (most recent call last): File "/usr/lib/python2.7/dist-packages/pip/basecommand.py", line 122, in main status = self.run(options, args) File "/usr/lib/python2.7/dist-packages/pip/commands/install.py", line 283, in run requirement_set.install(install_options, global_options, root=options.root_path) File "/usr/lib/python2.7/dist-packages/pip/req.py", line 1435, in install requirement.install(install_options, global_options, *args, **kwargs) File "/usr/lib/python2.7/dist-packages/pip/req.py", line 706, in install cwd=self.source_dir, filter_stdout=self._filter_install, show_stdout=False) File "/usr/lib/python2.7/dist-packages/pip/util.py", line 697, in call_subprocess % (command_desc, proc.returncode, cwd)) InstallationError: Command /usr/bin/python -c "import setuptools, tokenize;__file__='/tmp/pip_build_root/lxml/setup.py';exec(compile(getattr(tokenize, 'open', open)(__file__).read().replace('\r\n', '\n'), __file__, 'exec'))" install --record /tmp/pip-O4cIn6-record/install-record.txt --single-version-externally-managed --compile failed with error code 1 in /tmp/pip_build_root/lxml
Please install the following dependencies:
sudo apt-get install libxml2-dev libxslt1-dev
Python code
The following is to generate sitemap and sitemapindex indexes code, you can pass in the required parameters as required, or add fields:
#!/usr/bin/env python # -*- coding:utf-8 -*- import io import re from lxml import etree def generate_xml(filename, url_list): """Generate a new xml file use url_list""" root = etree.Element('urlset', xmlns="http://www.sitemaps.org/schemas/sitemap/0.9") for each in url_list: url = etree.Element('url') loc = etree.Element('loc') loc.text = each url.append(loc) root.append(url) header = u'<?xml version="1.0" encoding="UTF-8"?>\n' s = etree.tostring(root, encoding='utf-8', pretty_print=True) with io.open(filename, 'w', encoding='utf-8') as f: f.write(unicode(header+s)) def update_xml(filename, url_list): """Add new url_list to origin xml file.""" f = open(filename, 'r') lines = [i.strip() for i in f.readlines()] f.close() old_url_list = [] for each_line in lines: d = re.findall('<loc>(http:\/\/.+)<\/loc>', each_line) old_url_list += d url_list += old_url_list generate_xml(filename, url_list) def generatr_xml_index(filename, sitemap_list, lastmod_list): """Generate sitemap index xml file.""" root = etree.Element('sitemapindex', xmlns="http://www.sitemaps.org/schemas/sitemap/0.9") for each_sitemap, each_lastmod in zip(sitemap_list, lastmod_list): sitemap = etree.Element('sitemap') loc = etree.Element('loc') loc.text = each_sitemap lastmod = etree.Element('lastmod') lastmod.text = each_lastmod sitemap.append(loc) sitemap.append(lastmod) root.append(sitemap) header = u'<?xml version="1.0" encoding="UTF-8"?>\n' s = etree.tostring(root, encoding='utf-8', pretty_print=True) with io.open(filename, 'w', encoding='utf-8') as f: f.write(unicode(header+s)) if __name__ == '__main__': urls = ['http://www.baidu.com'] * 10 mods = ['2004-10-01T18:23:17+00:00'] * 10 generatr_xml_index('index.xml', urls, mods)
Effect
The generated effect should be in this format:
sitemap format:
<?xml version="1.0" encoding="UTF-8"?> <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <url> <loc>http://www.example.com/foo.html</loc> </url> </urlset> sitemapindex格式: <?xml version="1.0" encoding="UTF-8"?> <sitemapindex xmlns="http://www.sitemaps.org/schemas/sitemap/0.9"> <sitemap> <loc>http://www.example.com/sitemap1.xml.gz</loc> <lastmod>2004-10-01T18:23:17+00:00</lastmod> </sitemap> <sitemap> <loc>http://www.example.com/sitemap2.xml.gz</loc> <lastmod>2005-01-01</lastmod> </sitemap> </sitemapindex>
Lastmod time format problem
The format uses the ISO 8601 standard. If it is a linux/unix system, you can use the following function to obtain it
def get_lastmod_time(filename): time_stamp = os.path.getmtime(filename) t = time.localtime(time_stamp) # return time.strftime('%Y-%m-%dT%H:%M:%S+08:00', t) return time.strftime('%Y-%m-%dT%H:%M:%SZ', t)
Optimization
Generally speaking, using lxml is inefficient and takes up a lot of memory. You can create it directly using the write method of the file.
def generate_xml(filename, url_list): with gzip.open(filename,"w") as f: f.write("""<?xml version="1.0" encoding="utf-8"?> <urlset xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">\n""") for i in url_list: f.write("""<url><loc>%s</loc></url>\n"""%i) f.write("""</urlset>""") def append_xml(filename, url_list): with gzip.open(filename, 'r') as f: for each_line in f: d = re.findall('<loc>(http:\/\/.+)<\/loc>', each_line) url_list.extend(d) generate_xml(filename, set(url_list)) def modify_time(filename): time_stamp = os.path.getmtime(filename) t = time.localtime(time_stamp) return time.strftime('%Y-%m-%dT%H:%M:%S:%SZ', t) def new_xml(filename, url_list): generate_xml(filename, url_list) root = dirname(filename) with open(join(dirname(root), "sitemap.xml"),"w") as f: f.write('<?xml version="1.0" encoding="utf-8"?>\n<sitemapindex xmlns="http://www.sitemaps.org/schemas/sitemap/0.9">\n') for i in glob.glob(join(root,"*.xml.gz")): lastmod = modify_time(i) i = i[len(CONFIG.SITEMAP_PATH):] f.write("<sitemap>\n<loc>http:/%s</loc>\n"%i) f.write("<lastmod>%s</lastmod>\n</sitemap>\n"%lastmod) f.write('</sitemapindex>')
The above is the detailed content of Detailed introduction to using Python to generate sitemap. For more information, please follow other related articles on the PHP Chinese website!
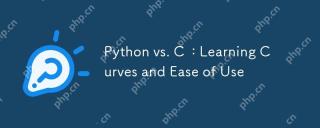
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.

Python and C have significant differences in memory management and control. 1. Python uses automatic memory management, based on reference counting and garbage collection, simplifying the work of programmers. 2.C requires manual management of memory, providing more control but increasing complexity and error risk. Which language to choose should be based on project requirements and team technology stack.
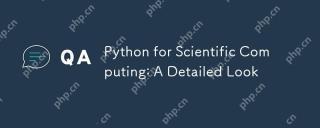
Python's applications in scientific computing include data analysis, machine learning, numerical simulation and visualization. 1.Numpy provides efficient multi-dimensional arrays and mathematical functions. 2. SciPy extends Numpy functionality and provides optimization and linear algebra tools. 3. Pandas is used for data processing and analysis. 4.Matplotlib is used to generate various graphs and visual results.
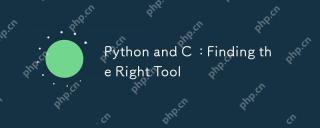
Whether to choose Python or C depends on project requirements: 1) Python is suitable for rapid development, data science, and scripting because of its concise syntax and rich libraries; 2) C is suitable for scenarios that require high performance and underlying control, such as system programming and game development, because of its compilation and manual memory management.
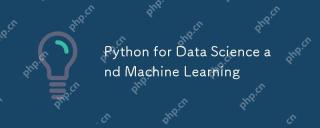
Python is widely used in data science and machine learning, mainly relying on its simplicity and a powerful library ecosystem. 1) Pandas is used for data processing and analysis, 2) Numpy provides efficient numerical calculations, and 3) Scikit-learn is used for machine learning model construction and optimization, these libraries make Python an ideal tool for data science and machine learning.
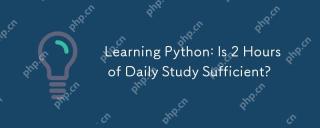
Is it enough to learn Python for two hours a day? It depends on your goals and learning methods. 1) Develop a clear learning plan, 2) Select appropriate learning resources and methods, 3) Practice and review and consolidate hands-on practice and review and consolidate, and you can gradually master the basic knowledge and advanced functions of Python during this period.
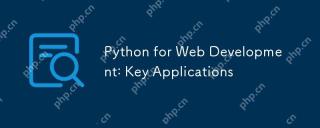
Key applications of Python in web development include the use of Django and Flask frameworks, API development, data analysis and visualization, machine learning and AI, and performance optimization. 1. Django and Flask framework: Django is suitable for rapid development of complex applications, and Flask is suitable for small or highly customized projects. 2. API development: Use Flask or DjangoRESTFramework to build RESTfulAPI. 3. Data analysis and visualization: Use Python to process data and display it through the web interface. 4. Machine Learning and AI: Python is used to build intelligent web applications. 5. Performance optimization: optimized through asynchronous programming, caching and code
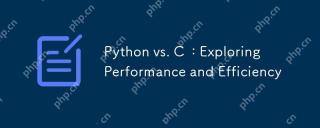
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
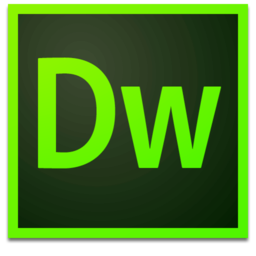
Dreamweaver Mac version
Visual web development tools
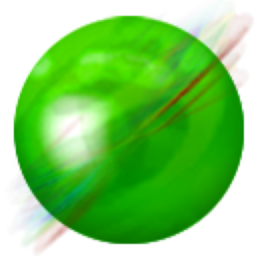
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
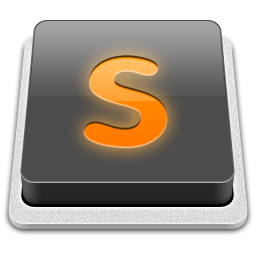
SublimeText3 Mac version
God-level code editing software (SublimeText3)