1.1: Type
There are 8 types of variables. There is no need to memorize them by rote. Deepen your understanding in practical applications
1) 整型 [integer] 数学中的整数 2) 浮点型 [float,double] 数学中的小数 3) 字符串 [string] 一串字符 4) 布尔 [boolean] 真假 5) 数组 [array] 键值对复合数据 6) 对象 [Object] [在后面的面向对象中会学到] 7) NULL 没有值 8) 资源 [resource] “吸管”
What is NULL? The NULL type only marks its type as NULL
Its value field is empty, NULL has no value
$a = 3;//整型 $b = 3.14;//浮点型 $c = null;//null型 $d = 'hello';//字符串 $e = true;//布尔型 echo $D;
Naming convention of variable names:
[a-zA-Z0-9] and underscore (_)
1) Variable names are case-sensitive
2) They cannot start with a number
1.2: Variable detection
echo A non-existent variable will report a notice-level error,
So we need to check whether this variable exists
How to check whether the variable exists?
isset - Check whether the variable is set
Declared variables return true, undeclared variables return false.
Detect whether a variable exists: just check whether the variable name exists in the roster
$b = null; $c = false; $d=0; $e=''; //分别检测上述变量是否存在 if(isset($a)) { echo '变量b存在'; }else{ echo '变量b不存在'; }
For variables with a value of NULL, false is also returned, because null has no value. Undeclared variables certainly do not exist.
1.3: Type detection
Detect a variable and what type PHP stores it as. It is very simple for PHP to get its variable type because it is already stored in the box. Its variable type
gettype — Get the type of variable [ready-made system function]
$a = false; echo gettype($a); $b = "1"; echo gettype($b); $c = 1.11; echo gettype($c); $d = 'hello'; echo gettype($d); $e = null; echo gettype($e);
Judge whether the variable is a certain type
is_float()[is_double] 检测变量是否为浮点型 is_int()[is_integer] 检测变量是否为整型 is_string() 检测变量是否为字符串 is_object() 检测变量是否为对象 is_array() 检测变量是否为数组 is_resource 检测变量是否为资源类型 is_bool 检测变量是否是布尔型 is_null 检测变量是否为 NULL $a = 'hello'; if(is_string($a)) { echo 'a是字符串'; }else{ echo 'a不是字符串'; }
1.4: Debugging Print variables
echo strings, numbers
print_r Print hierarchical data, such as: arrays, objects
var_dump Print the type and value of variables [convenient for debugging code]
$a = 'hello'; $b = array(1,2,"3"); $c = false; $d = null; $e=18; $f = true; //布尔型的true会打印出1,false和null什么都不显示 echo $a,$b,$c,$d,$e,$f,'<hr>'; //print_r 打印层次化的数据,比如数组和对象 print_r($b); print_r($c); print_r($d); print_r($f); //不要用echo和print_r打印布尔型的值,因为会干扰我们 //用var_dump打印布尔和null var_dump($c); var_dump($d);
1.5: Type conversion
In PHP, the type of variables can be converted at any time. It is very flexible. The most common one is the conversion between characters and numbers, or numbers/strings-> Conversion of Boolean values
Conversion from string to number
Intercept from left to right, until an illegal number is encountered, the intercepted part is converted into a number
$a = '12'; $b=$a+3; var_dump($b); $a = '12.5hello'; //$a = '12.5hello99'; $b=$a+3; var_dump($b); $a = 'word12.5hello'; $b=$a+3; var_dump($b);
Conversion of numbers to strings
$a = 123; $b = $a . 'hello'; var_dump($b);
Conversion of numbers/strings/arrays to Boolean values
$b=3; if($b){ echo 'b is true'; }else{ echo 'b is false'; }
if judged It should be a Boolean value. If the number 3 is converted into a Boolean value, then should it be understood as true or false?
The following values are all understood as false as Boolean values
'','0',0,0.0,false,NULL,array(); and other values are treated as Boolean true
if('' == false) { echo '空字符串果然假'; }
empty(var) — Check a Whether the variable is empty
If var is a non-empty or non-zero value, empty() returns FALSE
In other words, "", 0, 0.0, "0", NULL, FALSE, array( ); and objects without any attributes will be considered empty. If var is empty, TRUE will be returned
$arr = array(); if(empty($arr)) { echo '变量为空'; }
1.6: Assignment
There are two ways to assign values:
1 .Assignment by value; (Two people watch the same TV station)
2. Assignment by reference; (Two people watch the same TV)
1. Assignment by value
Variable In fact, the name is not posted on the box, but there is a variable table (like a class roster). The variable value and variable type are placed in the box; the variable name in the variable table points to its corresponding box.
$li = 23; $wang = $li; echo $li, '~', $wang;
Change the value of $li, will the value of $wang change?
$li = 99; echo $li, '~' ,$wang;
This assignment process is to assign the value of $li to $wang
2. Reference assignment
$a = 'tvb'; $b = &$a; //$a,$b共同指向同一个值 echo $a,'~',$b;
Change the value of $a
$a = 'btv'; echo $a,'~',$b;
1.7: Destruction
Why should we destroy variables?
Because, sometimes there are larger arrays or larger objects Special GD drawing consumes resources. Unset it and you can release the memory in time
unset (variable name); To destroy the specified variable, first delete the variable name from the variable table (roster), and then find its corresponding The box is also deleted.
$a=99; //unset($a); if(isset($a)) { echo 'a存在'; }else{ echo 'a不存在'; }
Note: Reference assignment, if two variables point to the same box, the box cannot be destroyed when one of the variables is destroyed.
$a=99; $b = &$a; unset($a); echo $a,$b;//报一个notice的错误
Reassign a new value to $a
$a=18; echo $a,$b;
1.8: Dynamic variable name
Dynamic variable name can reflect the very flexibility of PHP
Use the value of the variable to make another variable Name
$laoda = 'liubei'; echo $laoda , '<br >'; $paihang = 'laoda'; echo $paihang , '~' , $$paihang; //排行 $rank = 'paihang'; echo $$$rank;
The above is the detailed content of Introduction to PHP variables. For more information, please follow other related articles on the PHP Chinese website!
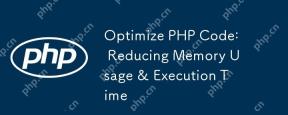
TooptimizePHPcodeforreducedmemoryusageandexecutiontime,followthesesteps:1)Usereferencesinsteadofcopyinglargedatastructurestoreducememoryconsumption.2)LeveragePHP'sbuilt-infunctionslikearray_mapforfasterexecution.3)Implementcachingmechanisms,suchasAPC
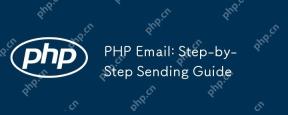
PHPisusedforsendingemailsduetoitsintegrationwithservermailservicesandexternalSMTPproviders,automatingnotificationsandmarketingcampaigns.1)SetupyourPHPenvironmentwithawebserverandPHP,ensuringthemailfunctionisenabled.2)UseabasicscriptwithPHP'smailfunct
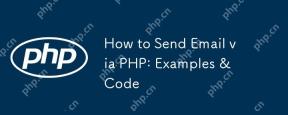
The best way to send emails is to use the PHPMailer library. 1) Using the mail() function is simple but unreliable, which may cause emails to enter spam or cannot be delivered. 2) PHPMailer provides better control and reliability, and supports HTML mail, attachments and SMTP authentication. 3) Make sure SMTP settings are configured correctly and encryption (such as STARTTLS or SSL/TLS) is used to enhance security. 4) For large amounts of emails, consider using a mail queue system to optimize performance.
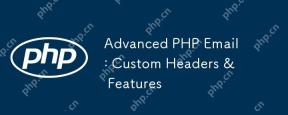
CustomheadersandadvancedfeaturesinPHPemailenhancefunctionalityandreliability.1)Customheadersaddmetadatafortrackingandcategorization.2)HTMLemailsallowformattingandinteractivity.3)AttachmentscanbesentusinglibrarieslikePHPMailer.4)SMTPauthenticationimpr
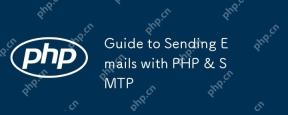
Sending mail using PHP and SMTP can be achieved through the PHPMailer library. 1) Install and configure PHPMailer, 2) Set SMTP server details, 3) Define the email content, 4) Send emails and handle errors. Use this method to ensure the reliability and security of emails.
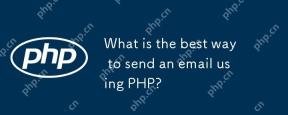
ThebestapproachforsendingemailsinPHPisusingthePHPMailerlibraryduetoitsreliability,featurerichness,andeaseofuse.PHPMailersupportsSMTP,providesdetailederrorhandling,allowssendingHTMLandplaintextemails,supportsattachments,andenhancessecurity.Foroptimalu
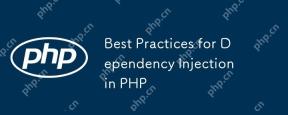
The reason for using Dependency Injection (DI) is that it promotes loose coupling, testability, and maintainability of the code. 1) Use constructor to inject dependencies, 2) Avoid using service locators, 3) Use dependency injection containers to manage dependencies, 4) Improve testability through injecting dependencies, 5) Avoid over-injection dependencies, 6) Consider the impact of DI on performance.
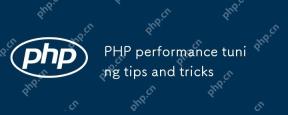
PHPperformancetuningiscrucialbecauseitenhancesspeedandefficiency,whicharevitalforwebapplications.1)CachingwithAPCureducesdatabaseloadandimprovesresponsetimes.2)Optimizingdatabasequeriesbyselectingnecessarycolumnsandusingindexingspeedsupdataretrieval.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
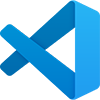
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

SublimeText3 English version
Recommended: Win version, supports code prompts!

Notepad++7.3.1
Easy-to-use and free code editor
