


The difference between function literal and Function() constructor
Although function literal is an anonymous function, the syntax allows you to specify any function name for it. When writing a recursive function, you can call it yourself, using Function( ) constructor does not work.
var f = function fact(x) { if (x < = 1) return 1; else return x*fact(x-1); };
The Function() constructor allows Javascript code to be dynamically created and compiled at runtime. In this way it is similar to the global function eval().
The Function() constructor parses the function body and creates a new function object each time it is executed. Therefore, the efficiency of calling the Function() constructor in a loop or frequently executed function is very low. In contrast, function literals are not recompiled every time they are encountered.
When creating a function using the Function() constructor, it does not follow the typical scope. It always executes it as a top-level function.
var y = "global"; function constructFunction() { var y = "local"; return new Function("return y"); // 无法获取局部变量} alert(constructFunction()()); // 输出 "global" 函数直接量:
As long as it is an expression syntax, the script host considers function to be a direct function. If nothing is added, just starting with function is considered to be a function declaration, and function is written into an expression. , such as the four arithmetic operations, the host will also treat it as a direct quantity, as follows:
var a = 10 + function(){ return 5; }();
(function(){ alert(1); } ) ( ); ( function(){ alert(2); } ( ) ); void function(){ alert(3); }() 0, function(){ alert(4); }(); -function(){ alert(5); }(); +function(){ alert(6); }(); !function(){ alert(7); }(); ~function(){ alert(8); }(); typeof function(){ alert(9); }();
There are many ways to define functions in js, and function direct quantity is one of them. For example, var fun = function(){}, if function is not assigned to fun, then it is an anonymous function.
See how the anonymous function is called.
1. Function calls that get return values after execution
//方式一,调用函数,得到返回值。强制运算符使函数调用执行 (function(x,y){ alert(x+y); return x+y; }(3,4)); //方式二,调用函数,得到返回值。强制函数直接量执行再返回一个引用,引用在去调用执行 (function(x,y){ alert(x+y); return x+y; })(3,4);
2. Ignore return values after execution
//方式三,调用函数,忽略返回值 void function(x) { x = x-1; alert(x); }(9);
Well, finally look at the wrong calling method
//错误的调用方式 function(x,y){ alert(x+y); return x+y; }(3,4);
The above is the detailed content of Detailed examples of the difference between javascript function literals and Function() constructors. For more information, please follow other related articles on the PHP Chinese website!
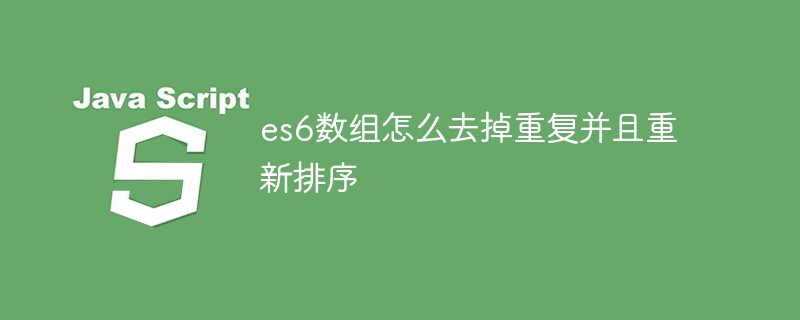
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
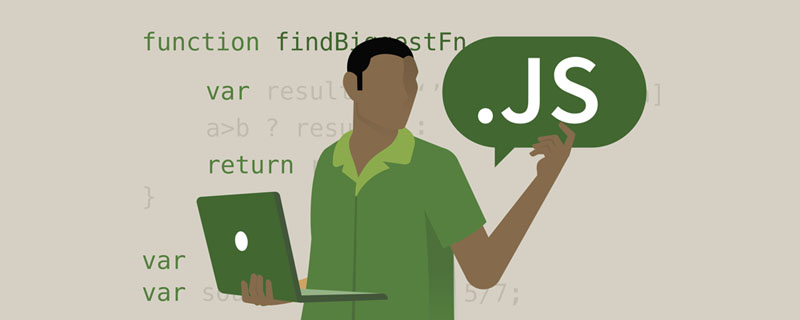
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
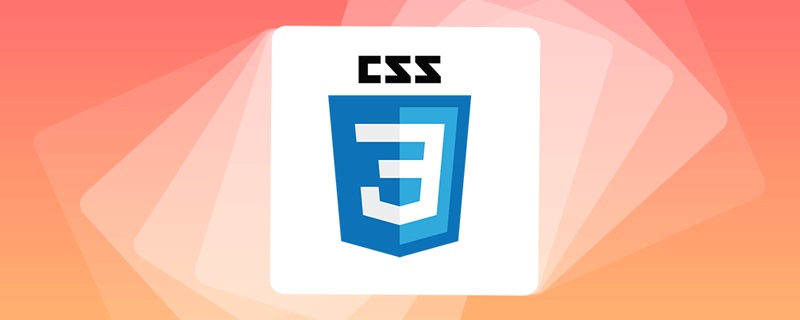
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
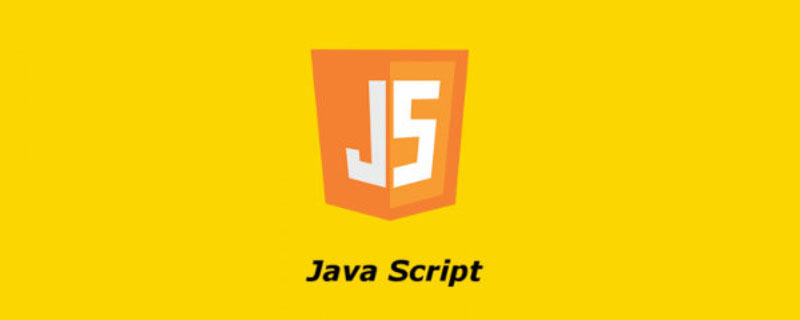
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
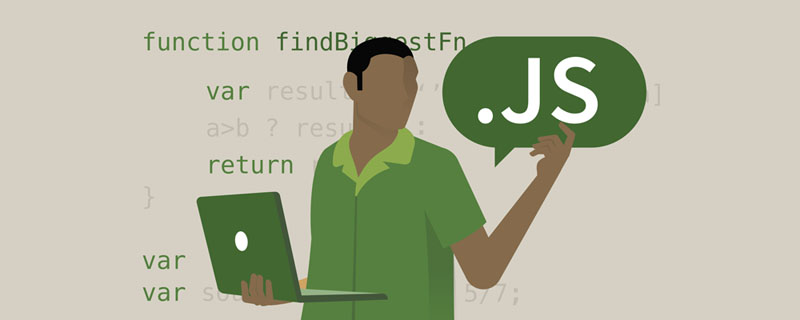
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
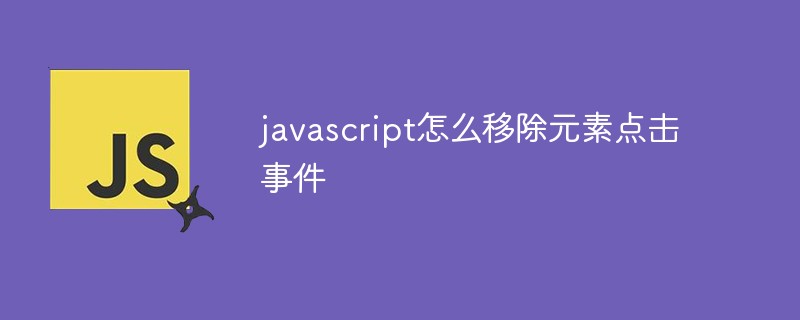
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
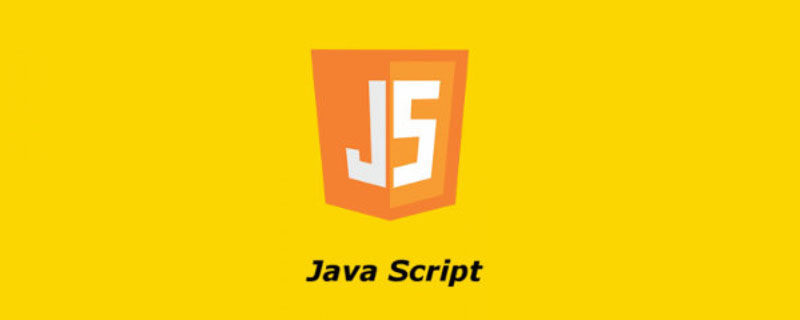
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
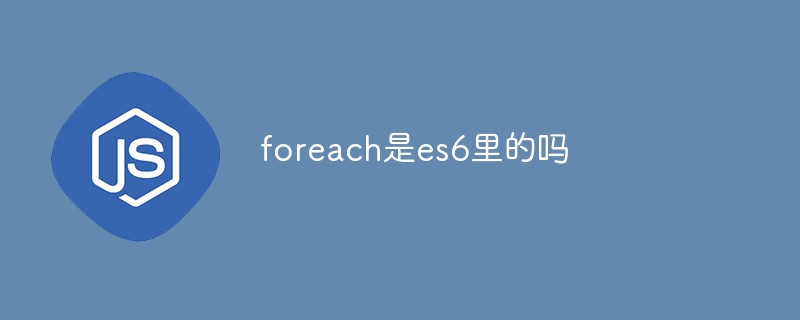
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
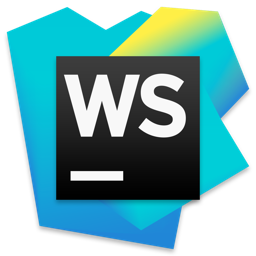
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Zend Studio 13.0.1
Powerful PHP integrated development environment
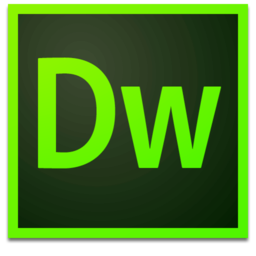
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor
