


Regarding the need for "traversing the properties and values of objects in js". The reason is to make a js plug-in that partially refreshes the table content.
Problem: Failed to obtain the attribute value of the object by traversing the attribute name array
The initial error code is as follows:
for(var i=0;i<dataList.length;i++) { var dataLine="<tr>"; for(var j=0;j<filedList.length;j++){ dataLine+="<td>"+dataList[i].filedList[j]+"</td>"; } dataLine+="</tr>"; $("#"+tableName).append(dataLine); }
First of all, dataList contains an array of objects; filedList contains an array of attribute field names of objects. At first, I thought like this, traverse the dataList, and get an object every time, then nest a for loop, traverse the filedList, get one of its attribute values each time, and then piece it together into a table.
For example: dataList[0] is an Emp object, and Emp has attributes such as id and name. Normally we can get the id value of the current Emp object through dataList[0].id. But if you traverse the attribute field array, you cannot use dataList[0].filedList[0] in this way. This does not mean that the value is not obtained in filedList[0], because I have already obtained the id value of 1 through alert(filedList[0]). So why does the acquisition fail? Because it is looking for a property called filedList[0] in the Emp object! Of course there is no such attribute in the Emp object, so the acquisition fails as it should. So how do we obtain the attribute value of the object?
Solution: Use "enhanced for loop" to traverse
The correct code is as follows:
for(var i=0;i<dataList.length;i++) { var dataLine="<tr>"; for(var filedName in dataList[i]){ dataLine+="<td>"+dataList[i][filedName]+"</td>"; } dataLine+="</tr>"; $("#"+tableName).append(dataLine); }
Solution: Since dataList[i] is an object, so I can get the attribute name of this object every time, and then get the attribute value of this attribute through dataList[i][filedName], that is, object [attribute name].
function displayProp(obj){ var names=""; for(var name in obj){ names+=name+": "+obj[name]+", "; } alert(names); }
The above is the detailed content of Detailed code explanation of how javascript traverses the attribute values of an object. For more information, please follow other related articles on the PHP Chinese website!
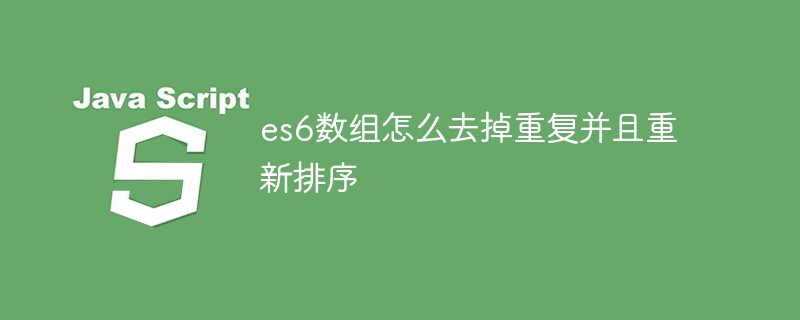
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
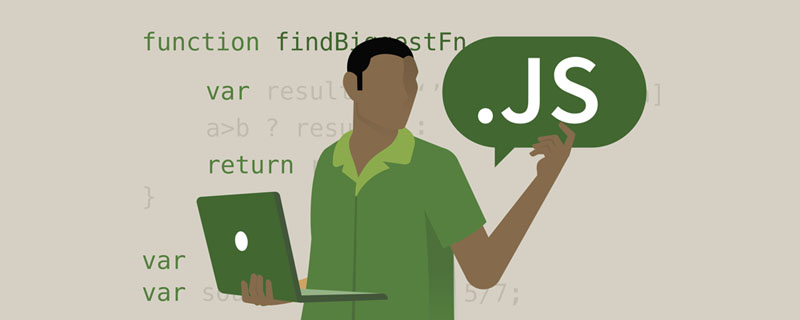
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
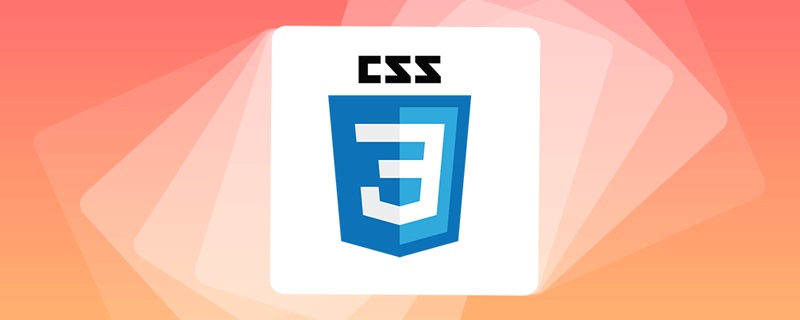
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
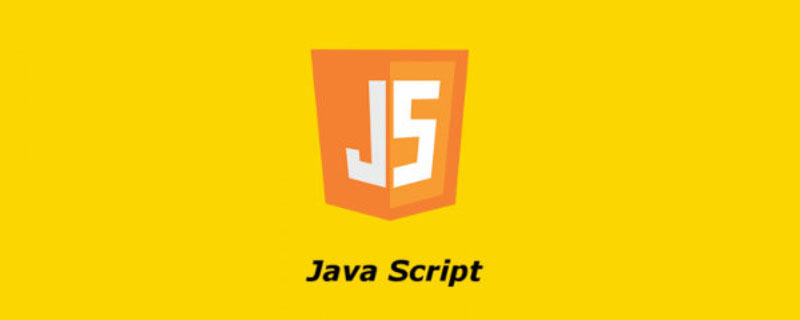
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
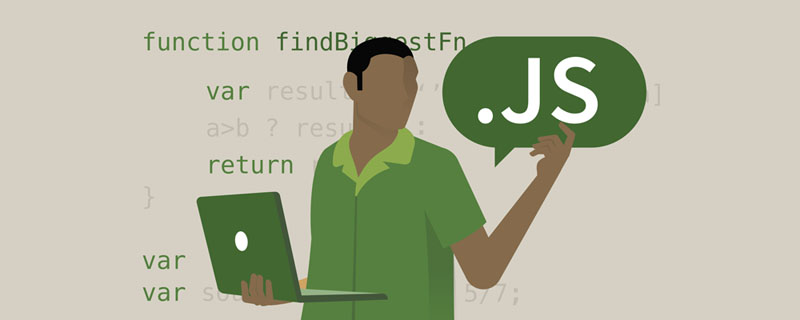
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
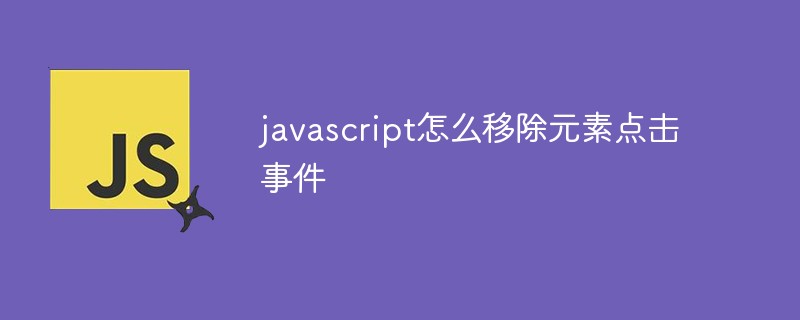
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
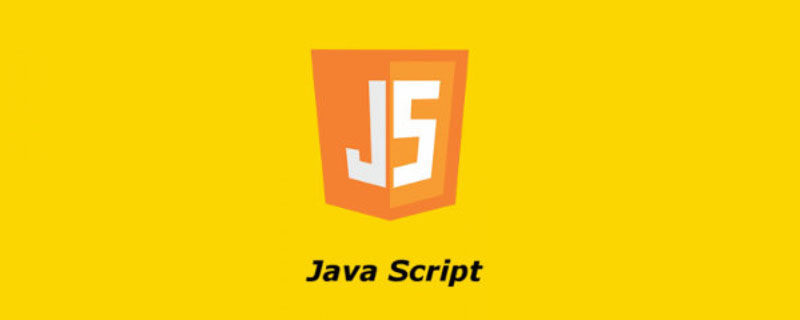
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
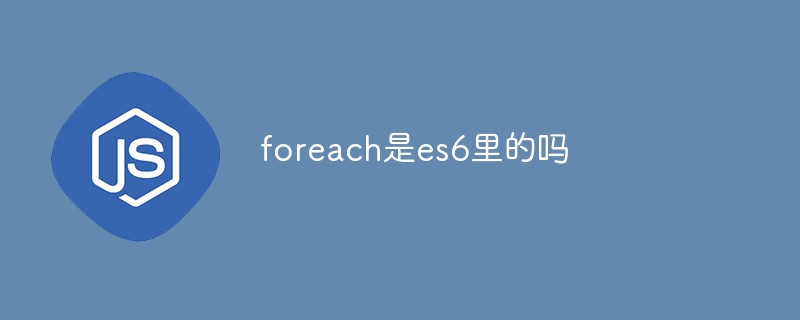
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
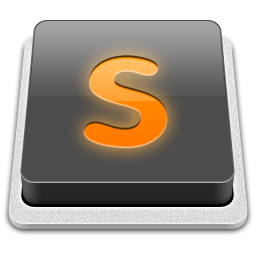
SublimeText3 Mac version
God-level code editing software (SublimeText3)

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
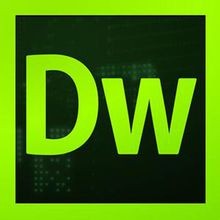
Dreamweaver CS6
Visual web development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
