


Update
After getting a DOM node, we can update it.
You can directly modify the text of the node. There are two methods:
One is to modify the innerHTML attribute, this The method is very powerful. Not only can you modify the text content of a DOM node, you can also directly modify the subtree inside the DOM node through HTML fragments:
// 获取<p id="p-id">...</p> var p = document.getElementById('p-id'); // 设置文本为abc: p.innerHTML = 'ABC'; // <p id="p-id">ABC</p> // 设置HTML: p.innerHTML = 'ABC <span style="color:red">RED</span> XYZ'; // <p>...</p>的内部结构已修改
Use## When #innerHTML, please pay attention to whether you need to write HTML. If the written string is obtained through the network, pay attention to character encoding to avoid XSS attacks. The second is to modify the
innerText or textContent attribute, so that the string can be automatically HTML-encoded to ensure that it cannot be set. Any HTML tag:
// 获取<p id="p-id">...</p> var p = document.getElementById('p-id'); // 设置文本: p.innerText = '<script>alert("Hi")</script>'; // HTML被自动编码,无法设置一个<script>节点: // <p id="p-id"><script>alert("Hi")</script></p>The difference between the two is that when reading attributes,
innerText does not return hidden elements of text, while textContent returns all text. Also note that IEtextContent. Modifying CSS is also a frequently required operation. The
style attribute of the DOM node corresponds to all CSS and can be obtained or set directly. Because CSS allows names like font-size, but it is not a valid property name in JavaScript, it needs to be rewritten in JavaScript as camel case naming fontSize:
// 获取<p id="p-id">...</p> var p = document.getElementById('p-id'); // 设置CSS: p.style.color = '#ff0000'; p.style.fontSize = '20px'; p.style.paddingTop = '2em';
insert
If this DOM node is empty, for example,
, then use innerHTML = 'child' can modify the content of the DOM node, which is equivalent to "inserting" a new DOM node. If the DOM node is not empty, you cannot do this, because
innerHTML will directly replace all the original child nodes. There are two ways to insert new nodes. One is to use
appendChild to add a child node to the last child node of the parent node. For example:
<!-- HTML结构 --> <p id="js">JavaScript</p> <p id="list"> <p id="java">Java</p> <p id="python">Python</p> <p id="scheme">Scheme</p> </p>Add
JavaScript :
##
var js = document.getElementById('js'), list = document.getElementById('list'); list.appendChild(js);
Now, HTML The structure becomes like this:
<!-- HTML结构 --> <p id="list"> <p id="java">Java</p> <p id="python">Python</p> <p id="scheme">Scheme</p> <p id="js">JavaScript</p> </p>
Because the js node we inserted already exists in the current document tree, this node will first be deleted from its original location. Then insert it into the new location. More often we will create a new node from scratch and then insert it into the specified position:
var list = document.getElementById('list'), haskell = document.createElement('p'); haskell.id = 'haskell'; haskell.innerText = 'Haskell'; list.appendChild(haskell);
In this way we dynamically add a new node Node:
<!-- HTML结构 --> <p id="list"> <p id="java">Java</p> <p id="python">Python</p> <p id="scheme">Scheme</p> <p id="haskell">Haskell</p> </p>
Dynamicly create a node and then add it to the DOM tree, which can achieve many functions. For example, the following code dynamically creates a
var d = document.createElement('style'); d.setAttribute('type', 'text/css'); d.innerHTML = 'p { color: red }'; document.getElementsByTagName('head')[0].appendChild(d);
You can execute the above code in the Chrome console and observe the page style. Variety. insertBefore
What if we want to insert the child node into the specified position? You can use
parentElement.insertBefore(newElement, referenceElement);, child nodes will be inserted before referenceElement. Still taking the above HTML as an example, assuming that we want to insert Haskell before Python:
<!-- HTML结构 --> <p id="list"> <p id="java">Java</p> <p id="python">Python</p> <p id="scheme">Scheme</p> </p>
can be written like this:
var list = document.getElementById('list'), ref = document.getElementById('python'), haskell = document.createElement('p'); haskell.id = 'haskell'; haskell.innerText = 'Haskell'; list.insertBefore(haskell, ref);
The new HTML structure is as follows:
<!-- HTML结构 --> <p id="list"> <p id="java">Java</p> <p id="haskell">Haskell</p> <p id="python">Python</p> <p id="scheme">Scheme</p> </p>
is visible, use
insertBeforeThe key point is to get a reference to a "reference child node". Many times, it is necessary to loop through all child nodes of a parent node, which can be achieved by iterating the children attribute:
var i, c, list = document.getElementById('list'); for (i = 0; i < list.children.length; i++) { c = list.children[i]; // 拿到第i个子节点 }
delete
删除一个DOM节点就比插入要容易得多。 注意到删除后的节点虽然不在文档树中了,但其实它还在内存中,可以随时再次被添加到别的位置。 例如,对于如下HTML结构: 当我们用如下代码删除子节点时: 浏览器报错:parent.children[1]不是一个有效的节点。原因就在于,当 First 因此,删除多个节点时,要注意children属性时刻都在变化。
要删除一个节点,首先要获得该节点本身以及它的父节点,然后,调用父节点的removeChild把自己删掉:// 拿到待删除节点:
var self = document.getElementById('to-be-removed');
// 拿到父节点:
var parent = self.parentElement;
// 删除:
var removed = parent.removeChild(self);
removed === self; // true
当你遍历一个父节点的子节点并进行删除操作时,要注意,children属性是一个只读属性,并且它在子节点变化时会实时更新。<p id="parent">
<p>First</p>
<p>Second</p>
</p>
var parent = document.getElementById('parent');
parent.removeChild(parent.children[0]);
parent.removeChild(parent.children[1]); // <-- 浏览器报错
The above is the detailed content of JavaScript tutorial: detailed explanation of how to update, insert, and modify dom node instance code. For more information, please follow other related articles on the PHP Chinese website!
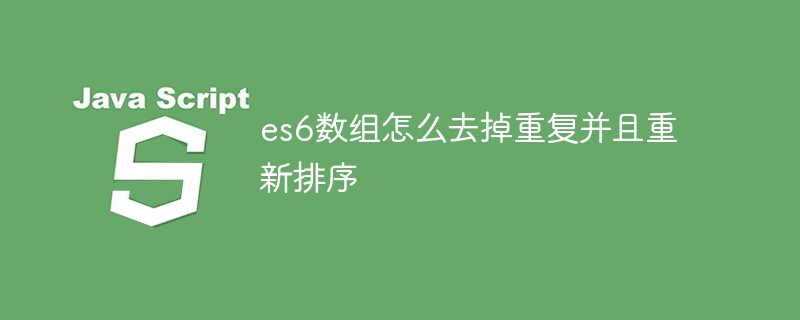
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
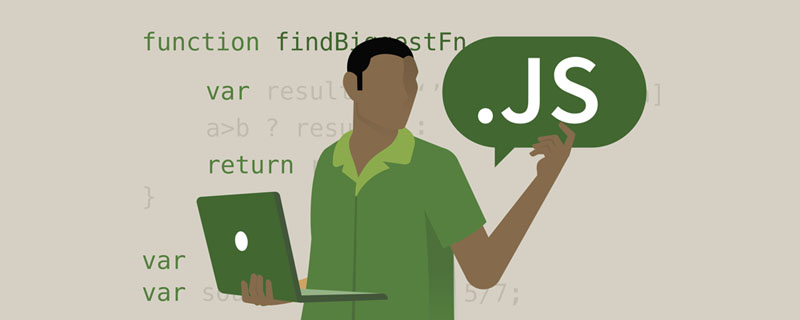
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
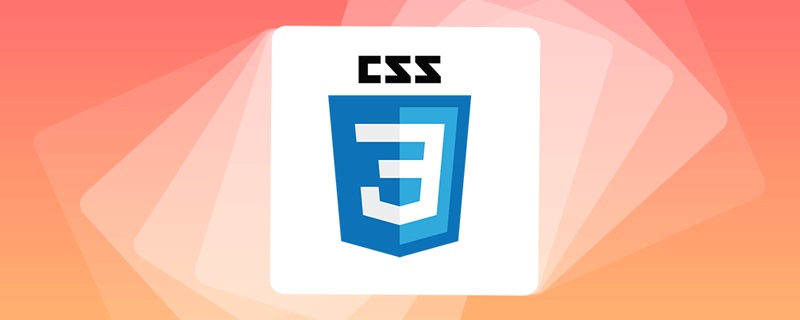
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
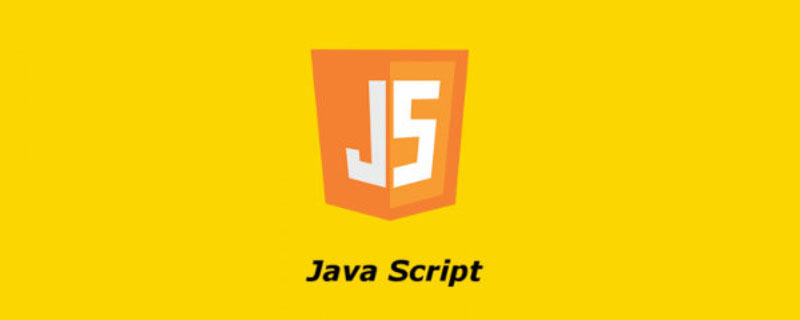
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
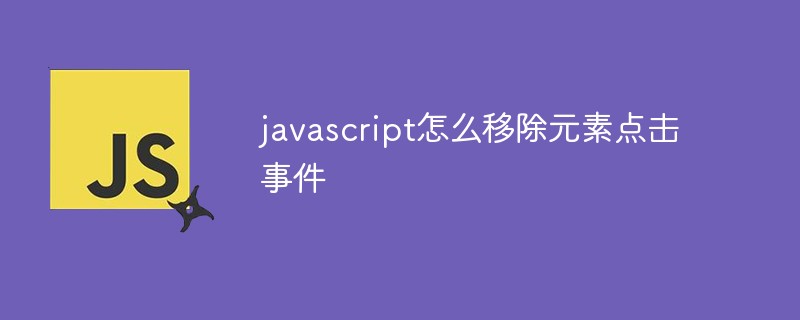
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
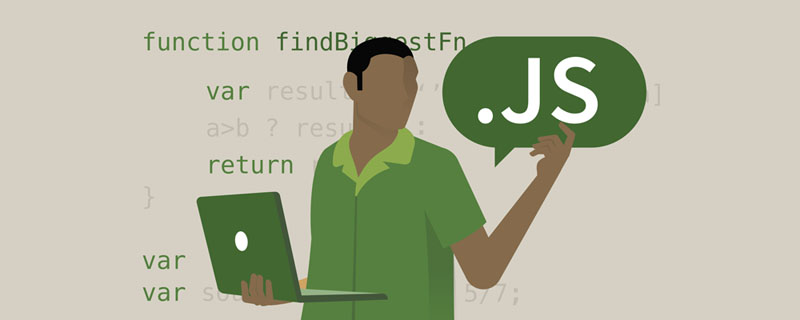
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
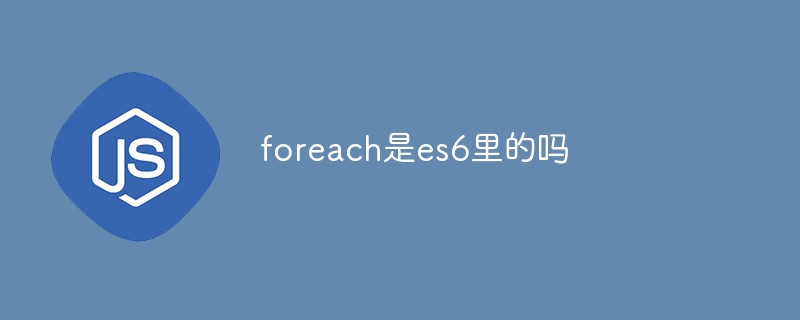
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。
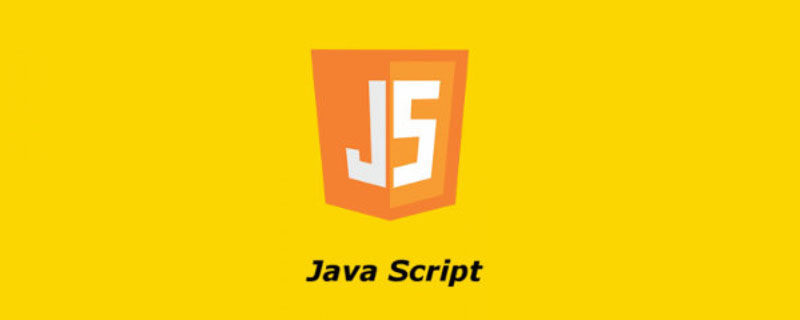
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
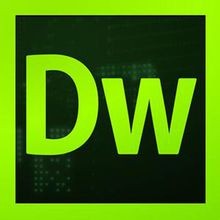
Dreamweaver CS6
Visual web development tools
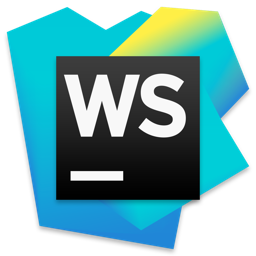
WebStorm Mac version
Useful JavaScript development tools