1. What is a list
1.1 As a type of sequence, a list is a collection of ordered elements.
1.2 List is the most commonly used built-in data type in Python. It is enclosed in square brackets [Element 1, Element 2...] and separated by commas. There is no relationship between the elements and can be of any type.
2. List declaration and access
#!/usr/bin/python# -*- coding:utf-8 -*- #变量的声明market = ['Apple','Banana','computer']#打印列表元素print market[0],market[1],market[2],market[-1].title()#For循环打印列表元素for element in market:print element,element.title()
3. Modification, addition and deletion of elements in the list
3.1 Modify list elements, list name + corresponding element Index
#修改索引值为2,即第3个元素值 market[2] = 'Telephone' print market #打印结果:['Apple', 'Banana', 'Telephone']
3.2 Add elements to the list. Python provides the append() and insert() methods. append() means adding elements to the end of the list, and insert() can specify the list. Add elements at the position, such as:
market.append('Orange') print market #得到结果:['Apple', 'Banana', 'computer', 'Orange'] market.insert(1,'Watermelon') print market #得到结果:['Apple', 'Watermelon', 'Banana', 'computer', 'Orange']
3.3 To delete elements from the list, you can use the del statement, pop() and remove() methods, such as:
#删除第1个元素Apple del market[0] print market #pop()方法删除列表末尾元素,可以接着使用它赋给其它的列表,如: pop_market = market.pop() #此时把通过pop()方法弹出的末尾元素赋给新的变量pop_market print type(pop_market) #通过打印pop_market的类型得知,此时类型为String <type> #如果想让弹出的元素赋值给新的列表该怎么办呢,可以先声明列表,然后直接用 append()方法追加,如下: pop_list_p = [] pop_list_p.append(market.pop()) print pop_list_p #或列表的切片,后续会提到 pop_list = market[-1] print market print pop_market print pop_list #remove()方法从列表中删除元素时,也可以接着使用它的值: #使用remove()方法时,只需要制定元素对应的值即可,如:删除元素Watermelon market.remove('Watermelon') print market</type>
4. The organization of the list, the sort() method represents permanent sorting, the sorted() function represents temporary sorting, the reverse() method represents reversing the order of the list elements, len() The function represents the length of the list
market = ['Computer','Banana','Apple'] market.sort()print market #sort()方法永久性改变了列表的元素排列的顺序,结果:['Apple', 'Banana', 'Computer']#sorted()函数临时性改变了列表的元素排列的顺序market = ['Computer','Banana','Apple']print (sorted(market)) #临时性排序['Apple', 'Banana', 'Computer']print market #再次打印还是预先的顺序['Computer', 'Banana', 'Apple']market.reverse()print market #reverse()方法反转列表元素的顺序#确定列表元素长度,也即是列表包含的元素个数,注意在用len()函数统计列表元素时是从1开始的print len(market)
Some common operations on the list:
Use subscript index to access the value in the list, you can also use the form of square brackets Intercept the characters as follows:
list1 = ['physics', 'chemistry', 1997, 2000]
list2 = [1, 2, 3, 4, 5, 6, 7 ]
print("list1[0]: ", list1[0])
print("list2[1: 5]: ", list2[1:5])
Output result
list1[0]: physics
list2[1:5] : [2, 3, 4, 5]
Update list
You can modify or update the data items in the list, or you can use append() method to add list items
list = ['physics', 'chemistry', 1997, 2000]
print("Value available at index 2: ")
print(list[2])
list[2] = 2001
print("New value available at index 2 : ")
print(list[2])
Output result:
Value available at index 2:
1997
New value available at index 2 :
2001
Delete list elements
Use the del statement to delete elements of the list
list1 = ['physics', 'chemistry', 1997, 2000]
print(list1)
del list1[2]
print("After deleting value at index 2 : ")
print(list1)
The above example output result:
['physics', 'chemistry', 1997, 2000]
After deleting value at index 2 :
['physics', 'chemistry', 2000]
Python list script operators
The operators for + and * on lists are similar to strings. The + sign is used for combined lists, and the * sign is used for repeated lists
len([1, 2, 3])
>>>3
[1, 2, 3] + [4, 5, 6]
>>>[1, 2, 3, 4, 5, 6]
['Hi!'] * 4
>>>['Hi!', 'Hi!', 'Hi!', 'Hi !']
3 in [1, 2, 3]
>>>True
for x in [1, 2, 3]:
print(x)
>>>123
Python list interception
Python's list interception and string operation types are as follows
L = ['spam', 'Spam', 'SPAM!' ]
L[2] #Read the third element in the list
>>>SPAM!
L[-2] #Read the second to last element in the list
>>>Spam
L[1:] #Intercept the list starting from the second element
>>>'Spam', 'SPAM!'
##
The above is the detailed content of Python list List. For more information, please follow other related articles on the PHP Chinese website!
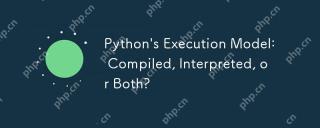
Pythonisbothcompiledandinterpreted.WhenyourunaPythonscript,itisfirstcompiledintobytecode,whichisthenexecutedbythePythonVirtualMachine(PVM).Thishybridapproachallowsforplatform-independentcodebutcanbeslowerthannativemachinecodeexecution.
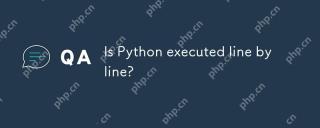
Python is not strictly line-by-line execution, but is optimized and conditional execution based on the interpreter mechanism. The interpreter converts the code to bytecode, executed by the PVM, and may precompile constant expressions or optimize loops. Understanding these mechanisms helps optimize code and improve efficiency.
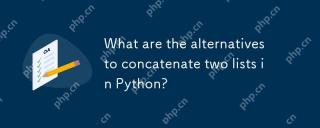
There are many methods to connect two lists in Python: 1. Use operators, which are simple but inefficient in large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use the = operator, which is both efficient and readable; 4. Use itertools.chain function, which is memory efficient but requires additional import; 5. Use list parsing, which is elegant but may be too complex. The selection method should be based on the code context and requirements.
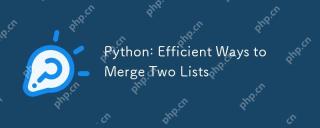
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, merge small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
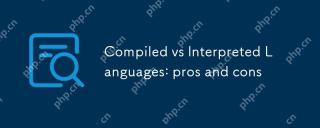
Compiledlanguagesofferspeedandsecurity,whileinterpretedlanguagesprovideeaseofuseandportability.1)CompiledlanguageslikeC arefasterandsecurebuthavelongerdevelopmentcyclesandplatformdependency.2)InterpretedlanguageslikePythonareeasiertouseandmoreportab
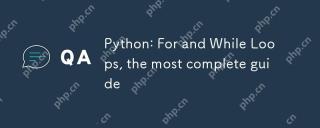
In Python, a for loop is used to traverse iterable objects, and a while loop is used to perform operations repeatedly when the condition is satisfied. 1) For loop example: traverse the list and print the elements. 2) While loop example: guess the number game until you guess it right. Mastering cycle principles and optimization techniques can improve code efficiency and reliability.
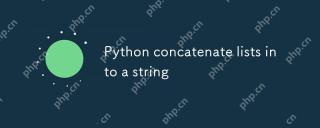
To concatenate a list into a string, using the join() method in Python is the best choice. 1) Use the join() method to concatenate the list elements into a string, such as ''.join(my_list). 2) For a list containing numbers, convert map(str, numbers) into a string before concatenating. 3) You can use generator expressions for complex formatting, such as ','.join(f'({fruit})'forfruitinfruits). 4) When processing mixed data types, use map(str, mixed_list) to ensure that all elements can be converted into strings. 5) For large lists, use ''.join(large_li
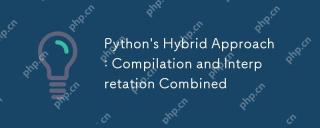
Pythonusesahybridapproach,combiningcompilationtobytecodeandinterpretation.1)Codeiscompiledtoplatform-independentbytecode.2)BytecodeisinterpretedbythePythonVirtualMachine,enhancingefficiencyandportability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Atom editor mac version download
The most popular open source editor
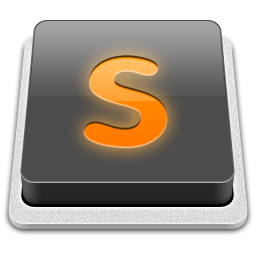
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 Chinese version
Chinese version, very easy to use
