Detailed explanation of examples of React practical projects (3)
React has nearly 70,000 stars on Github and is currently the most popular front-end framework. I have been learning React for a while, and now I am starting to practice it with React+Redux!
Last time we talked about using Redux for state management. This time we use Redux-saga to manage Redux application asynchronous operations
React Practice Project (1)
React Practice Project (2)
React Practice Project (3)
- First, let’s take a look at the logged-in Reducer
export const auth = (state = initialState, action = {}) => { switch (action.type) { case LOGIN_USER: return state.merge({ 'user': action.data, 'error': null, 'token': null, }); case LOGIN_USER_SUCCESS: return state.merge({ 'token': action.data, 'error': null }); case LOGIN_USER_FAILURE: return state.merge({ 'token': null, 'error': action.data }); default: return state } };
Sagas to monitor the action initiated, and then decide What to do based on this action: whether to initiate an asynchronous call (such as an Ajax request), initiate other actions to the Store, or even call other Sagas.
Specifically, this login function will issue a LOGIN_USER
action when we click on the login pop-up window. Sagas monitors the LOGIN_USER
action and initiates an Ajax request to the background. , decide to initiate LOGIN_USER_SUCCESS
action or LOGIN_USER_FAILURE
action
based on the result
Next, let’s implement this process
Create Saga Middleware connects to Redux store
Add redux-saga
dependency in package.json
"redux-saga": "^ 0.15.4"
Modify
src/redux/store/store.js/** * Created by Yuicon on 2017/6/27. */ import {createStore, applyMiddleware } from 'redux'; import createSagaMiddleware from 'redux-saga' import reducer from '../reducer/reducer'; import rootSaga from '../sagas/sagas'; const sagaMiddleware = createSagaMiddleware(); const store = createStore( reducer, applyMiddleware(sagaMiddleware) ); sagaMiddleware.run(rootSaga); export default store;
Redux-saga uses Generator function to implement
Listening action
Create src/redux/sagas/sagas.js
/** * Created by Yuicon on 2017/6/28. */ import { takeLatest } from 'redux-saga/effects'; import {registerUserAsync, loginUserAsync} from './users'; import {REGISTER_USER, LOGIN_USER} from '../action/users'; export default function* rootSaga() { yield [ takeLatest(REGISTER_USER, registerUserAsync), takeLatest(LOGIN_USER, loginUserAsync) ]; }
We can see that two actions are monitored in rootSaga: login and register.
- In the above example, takeLatest only allows one loginUserAsync task to be executed. And this task is the last one to be started. If there is already a task being executed before, the previous task will be automatically canceled.
If we allow multiple loginUserAsync instances to start at the same time. At a certain moment, we can start a new loginUserAsync task, even though one or more previous loginUserAsync tasks have not yet completed. We can use the takeEvery helper function.
Initiate an Ajax request
Get the data on Store state
-
selectors.js
/** * Created by Yuicon on 2017/6/28. */ export const getAuth = state => state.auth;
api
-
api.js
/** * Created by Yuicon on 2017/7/4. * */ /** * 这是我自己的后台服务器,用 Java 实现 * 项目地址: * 文档:http://139.224.135.86:8080/swagger-ui.html#/ */ const getURL = (url) => `http://139.224.135.86:8080/${url}`; export const login = (user) => { return fetch(getURL("auth/login"), { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(user) }).then(response => response.json()) .then(json => { return json; }) .catch(ex => console.log('parsing failed', ex)); };
Create src/redux/sagas/users. js
/** * Created by Yuicon on 2017/6/30. */ import {select, put, call} from 'redux-saga/effects'; import {getAuth, getUsers} from './selectors'; import {loginSuccessAction, loginFailureAction, registerSuccessAction, registerFailureAction} from '../action/users'; import {login, register} from './api'; import 'whatwg-fetch'; export function* loginUserAsync() { // 获取Store state 上的数据 const auth = yield select(getAuth); const user = auth.get('user'); // 发起 ajax 请求 const json = yield call(login.bind(this, user), 'login'); if (json.success) { localStorage.setItem('token', json.data); // 发起 loginSuccessAction yield put(loginSuccessAction(json.data)); } else { // 发起 loginFailureAction yield put(loginFailureAction(json.error)); } }
select(selector, ...args)Used to obtain data on Store state
put(action) Initiate an action to the Storecall(fn, ...args)- Call the fn function with args as the parameter. If the result is a Promise, the middleware will pause until the Promise is resolved. After resolution, the Generator will continue to be executed. Or until the Promise is rejected, in which case an error will be thrown in the Generator.
Redux-saga detailed api documentation
Conclusion
The above is the detailed content of Detailed explanation of examples of React practical projects (3). For more information, please follow other related articles on the PHP Chinese website!
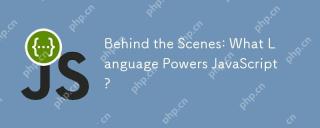
JavaScript runs in browsers and Node.js environments and relies on the JavaScript engine to parse and execute code. 1) Generate abstract syntax tree (AST) in the parsing stage; 2) convert AST into bytecode or machine code in the compilation stage; 3) execute the compiled code in the execution stage.
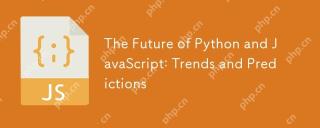
The future trends of Python and JavaScript include: 1. Python will consolidate its position in the fields of scientific computing and AI, 2. JavaScript will promote the development of web technology, 3. Cross-platform development will become a hot topic, and 4. Performance optimization will be the focus. Both will continue to expand application scenarios in their respective fields and make more breakthroughs in performance.
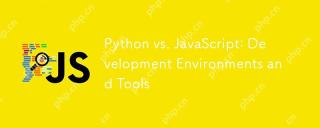
Both Python and JavaScript's choices in development environments are important. 1) Python's development environment includes PyCharm, JupyterNotebook and Anaconda, which are suitable for data science and rapid prototyping. 2) The development environment of JavaScript includes Node.js, VSCode and Webpack, which are suitable for front-end and back-end development. Choosing the right tools according to project needs can improve development efficiency and project success rate.
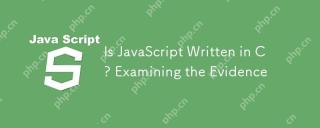
Yes, the engine core of JavaScript is written in C. 1) The C language provides efficient performance and underlying control, which is suitable for the development of JavaScript engine. 2) Taking the V8 engine as an example, its core is written in C, combining the efficiency and object-oriented characteristics of C. 3) The working principle of the JavaScript engine includes parsing, compiling and execution, and the C language plays a key role in these processes.
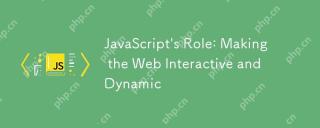
JavaScript is at the heart of modern websites because it enhances the interactivity and dynamicity of web pages. 1) It allows to change content without refreshing the page, 2) manipulate web pages through DOMAPI, 3) support complex interactive effects such as animation and drag-and-drop, 4) optimize performance and best practices to improve user experience.
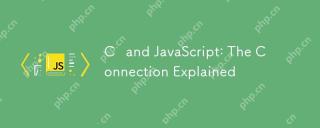
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
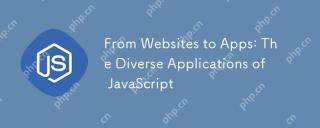
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
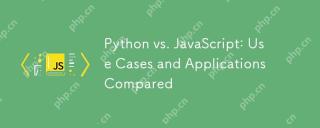
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
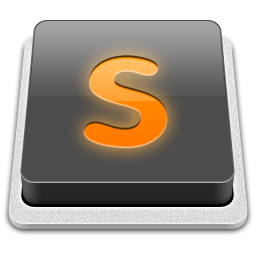
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

SublimeText3 English version
Recommended: Win version, supports code prompts!
