JavaScriptHow is the rebound animation effect achieved? Let me introduce it to you in detail through the code.
The code is as follows:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <style> #box{ width:200px; height:200px; position: absolute; top:0; left:200px; background:lightblue; } .btn{ position:absolute; top:200px; left:100px; height:50px; } .btn input{ display:inline-block; margin-left:50px; outline: none; width:100px; height:50px; border:1px solid green; cursor:pointer; } </style> </head> <body> <p id='box'></p> <p class='btn'> <input type="button" value='向左' id='btnLeft'> <input type="button" value='向右' id='btnRight'> </p> <script> var oBox = document.getElementById("box"); var minLeft = 0; var maxLeft = utils.win('clientWidth')-oBox.offsetWidth; var step = 5; var timer = null; function move(target){ //target:告诉我要运动的目标位置 window.clearTimeout(timer); var curLeft = utils.css(oBox,"left"); if(curLeft<target){//向右走 if(curLeft+step>target){//边界 utils.css(oBox,"left",target); return; } curLeft+=step; utils.css(oBox,"left",curLeft) }else if(curLeft>target){//向左走 if(curLeft-step<target){//边界 utils.css(oBox,"left",target); return; } curLeft-=step; utils.css(oBox,"left",curLeft) }else{//不需要运动 return; } // timer = window.setTimeout(move,10)//这里有一个问题,点击按钮第一次target的值是有的,但是第二次通过setTimeout执行的时候没有给target进行传值。是undefined timer = window.setTimeout(function(){ move(target); },10)//这样使用匿名函数包裹一下,就解决了上面的问题,但是这样写性能不好,因为每一次到达时间的时候,都需要执行一次匿名函数(形成一个私有的作用域),在匿名函数中再执行move,但是move中需要用到的数据值在第一次执行的move方法中,需要把匿名函数形成的这个私有的作用域作为跳板找到之前的,这样就导致了匿名函数形成的这个私有的作用域不能销毁 } document.getElementById('btnLeft').onclick = function(){ move(minLeft) } document.getElementById('btnRight').onclick = function(){ move(maxLeft) } </script> </body> </html>
In order to solve the above problem of poor performance, the following is an optimized code: it uses a function wrapper, so that there is only a private function created by the move function The domain is not destroyed. When _move is executed, move will naturally be destroyed.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document</title> <style> #box{ width:200px; height:200px; position: absolute; top:0; left:200px; background:lightblue; } .btn{ position:absolute; top:200px; left:100px; height:50px; } .btn input{ display:inline-block; margin-left:50px; outline: none; width:100px; height:50px; border:1px solid green; cursor:pointer; } </style> </head> <body> <p id='box'></p> <p class='btn'> <input type="button" value='向左' id='btnLeft'> <input type="button" value='向右' id='btnRight'> </p> <script> var oBox = document.getElementById("box"); var minLeft = 0; var maxLeft = utils.win('clientWidth')-oBox.offsetWidth; var step = 5; var timer = null; function move(target){ //target:告诉我要运动的目标位置 _move(); function _move(){ window.clearTimeout(timer); var curLeft = utils.css(oBox,"left"); if(curLeft<target){//向右走 if(curLeft+step>target){//边界 utils.css(oBox,"left",target); return; } curLeft+=step; utils.css(oBox,"left",curLeft) }else if(curLeft>target){//向左走 if(curLeft-step<target){//边界 utils.css(oBox,"left",target); return; } curLeft-=step; utils.css(oBox,"left",curLeft) }else{//不需要运动 return; } timer = window.setTimeout(_move,10); } } document.getElementById('btnLeft').onclick = function(){ move(minLeft) } document.getElementById('btnRight').onclick = function(){ move(maxLeft) } </script> </body> </html>
Note: In order to allow the current element to run only one animation at the same time (first clear the timer of the previous animation when the next animation starts): ensure that the current element has all The variable that the animation receives the return value of the timer needs to be shared. There are two ways: 1. Global reception (for example, the above code var timer = null) 2. Add custom attributes to the element (as shown in the figure below)
Summary: From the above, we can draw four rules for animation optimization:
1. Boundary judgment and step size
2. Clear useless timers
3. When the outer function needs to pass parameters, you can nest a layer of functions inside to avoid the accumulation of scopes.
4. Store the return value of the timer in the custom attribute of the element to prevent global variable conflicts and multiple animation executions at the same time
The above is the detailed content of How is the JavaScript rebound animation effect implemented?. For more information, please follow other related articles on the PHP Chinese website!
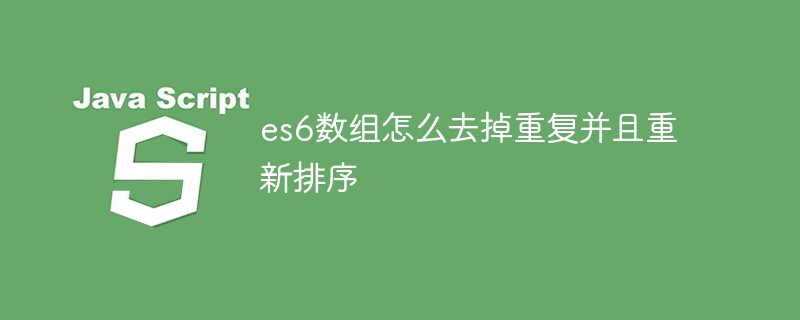
去掉重复并排序的方法:1、使用“Array.from(new Set(arr))”或者“[…new Set(arr)]”语句,去掉数组中的重复元素,返回去重后的新数组;2、利用sort()对去重数组进行排序,语法“去重数组.sort()”。
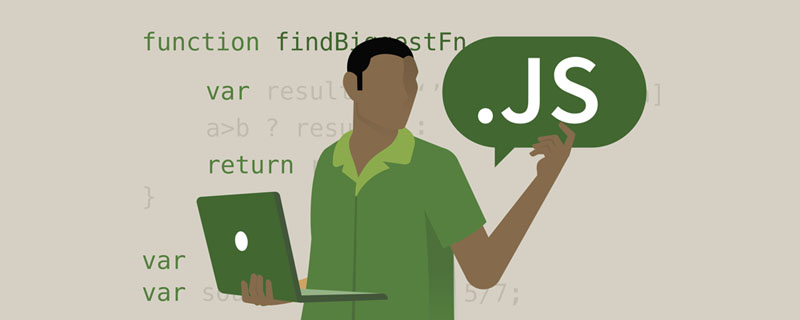
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于Symbol类型、隐藏属性及全局注册表的相关问题,包括了Symbol类型的描述、Symbol不会隐式转字符串等问题,下面一起来看一下,希望对大家有帮助。
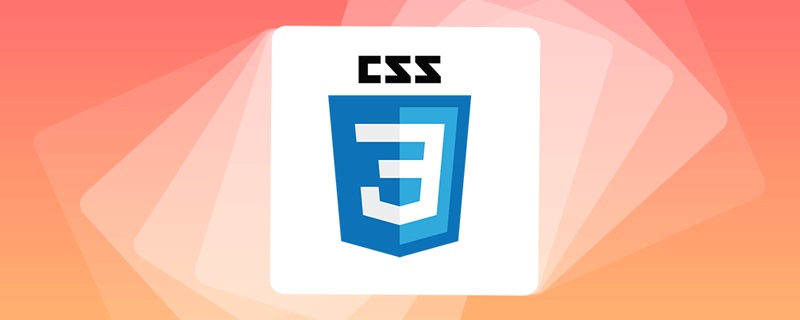
怎么制作文字轮播与图片轮播?大家第一想到的是不是利用js,其实利用纯CSS也能实现文字轮播与图片轮播,下面来看看实现方法,希望对大家有所帮助!
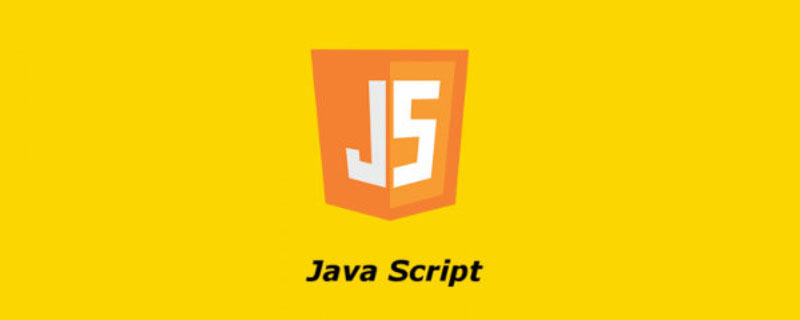
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于对象的构造函数和new操作符,构造函数是所有对象的成员方法中,最早被调用的那个,下面一起来看一下吧,希望对大家有帮助。
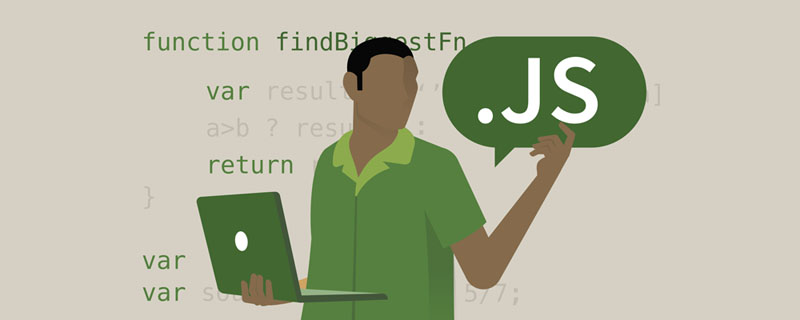
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于面向对象的相关问题,包括了属性描述符、数据描述符、存取描述符等等内容,下面一起来看一下,希望对大家有帮助。
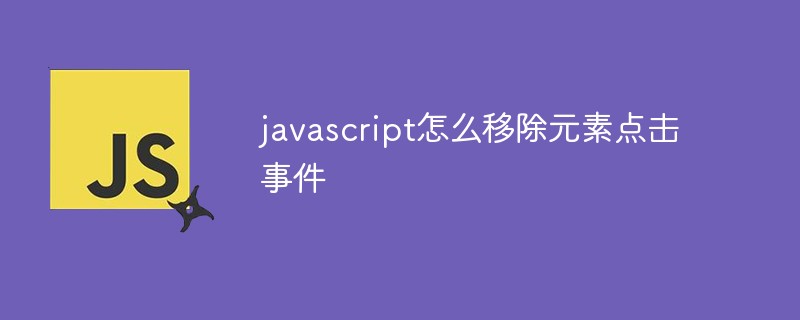
方法:1、利用“点击元素对象.unbind("click");”方法,该方法可以移除被选元素的事件处理程序;2、利用“点击元素对象.off("click");”方法,该方法可以移除通过on()方法添加的事件处理程序。
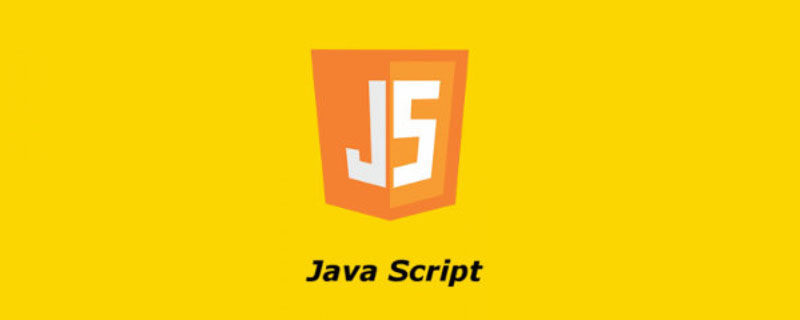
本篇文章给大家带来了关于JavaScript的相关知识,其中主要介绍了关于BOM操作的相关问题,包括了window对象的常见事件、JavaScript执行机制等等相关内容,下面一起来看一下,希望对大家有帮助。
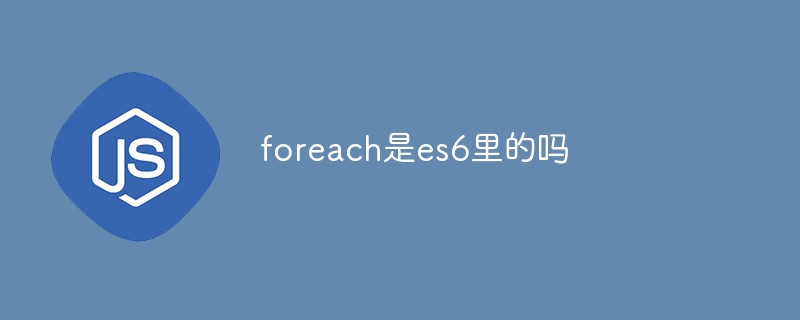
foreach不是es6的方法。foreach是es3中一个遍历数组的方法,可以调用数组的每个元素,并将元素传给回调函数进行处理,语法“array.forEach(function(当前元素,索引,数组){...})”;该方法不处理空数组。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
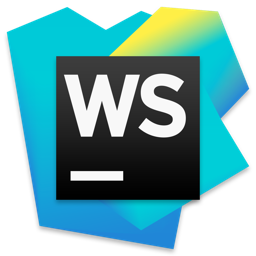
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
