Following the first two study notes, this article mainly introduces the layout manager and the dialog box.
1. Layout Manager
Let’s take a small example to introduce the topic. Just add two buttons at will. What kind of effect will it produce? Let’s see the execution results.
import java.awt.Button;import java.awt.Frame;public class Test25 {public static void main(String[] args) { Frame f = new Frame("布局管理器"); f.setSize(300, 400); f.add(new Button("按钮A")); f.add(new Button("按钮B")); f.setVisible(true); } }
Execution result:
From running the program, only the second button B is seen.
The reason is that each component has a specific position and size in the container. It is difficult to determine its position when arranging components in the container. To simplify user control, Java adopts a layout manager for management. Different layout managers can be specified for containers.
1.BorderLayout (border layout, default mode) divides the container into five areas: southeast, northwest, middle and middle
Frame f=new Frame("布局管理器"); f.add(new Button("按钮A"),BorderLayout.NORTH); f.add(new Button("按钮B")); f.add(new Button("按钮C"),"East"); f.setBounds(20,20,300,300); f.setVisible(true);
2. FlowLayout From left to right, from top to bottom Next, if we add parameters such as "North" and "East" to the FlowLayout layout, they will be ignored
Frame f=new Frame("布局管理器"); f.setLayout(new FlowLayout() ); f.add(new Button("按钮A"),BorderLayout.NORTH); f.add(new Button("按钮B")); f.add(new Button("按钮B"));
3. GridLayout grid, from left to right, from From top to bottom, this layout always ignores the optimal size of the component, and the width of all cells is the same
4.CardLayout Tab Layout
5.GridBagLayout The king of layout, powerful function, Complex to use,
6.BoxLayOut is a new layout manager in Swing that allows multiple components to be placed vertically or horizontally, and multiple Panels of this layout manager can be nested to achieve something similar
7. The function of GridBagLayout, but it is much simpler than using GridBagLayOut
More complex code examples (the purpose is a grid layout with one column and three rows on the left, a tab layout on the right, and a button on the left Pressing will trigger the corresponding change on the right)
import java.awt.BorderLayout;import java.awt.Button;import java.awt.CardLayout;import java.awt.Color;import java.awt.Frame;import java.awt.GridLayout;import java.awt.Panel;import java.awt.event.ActionEvent;import java.awt.event.ActionListener;import java.awt.event.WindowAdapter;import java.awt.event.WindowEvent;//布局的例子class LayoutTest {public LayoutTest() { init(); } CardLayout cl = new CardLayout(); // 卡片布局Panel plCenter = new Panel(); // 面板,容器private void init() { Frame f = new Frame(); Panel plWest = new Panel(); plWest.setBackground(Color.pink); plCenter.setBackground(Color.DARK_GRAY); f.add(plWest, BorderLayout.WEST); // 往左边放 f.add(plCenter); plWest.setLayout(new GridLayout(3, 1)); // 网格布局,三行,一列Button btnPrev = new Button("前一个"); Button btnNext = new Button("后一个"); Button btnThree = new Button("第三个"); plWest.add(btnPrev); plWest.add(btnNext); plWest.add(btnThree); plCenter.setLayout(cl); // 中间的主面板,设为卡片布局plCenter.add(new Button("A"), "1AAA"); plCenter.add(new Button("B"), "2AAA"); plCenter.add(new Button("C"), "3AAA"); plCenter.add(new Button("D"), "4AAA"); plCenter.add(new Button("E"), "5AAA"); plCenter.add(new Button("F"), "6AAA");// 用于按钮事件处理的内部类class MyActionListener implements ActionListener {public void actionPerformed(ActionEvent e) {if (e.getActionCommand().equals("前一个")) { cl.previous(plCenter); } else if (e.getActionCommand().equals("后一个")) { cl.next(plCenter); } else if (e.getActionCommand().equals("第三个")) { cl.show(plCenter, "5AAA"); // 指定显示哪一个 } } } MyActionListener listener = new MyActionListener(); btnPrev.addActionListener(listener); btnNext.addActionListener(listener); btnThree.addActionListener(listener); f.setSize(300, 300); f.setVisible(true); f.addWindowListener(new WindowAdapter() {public void windowClosing(WindowEvent e) { System.exit(0); } }); } }class Test26 {public static void main(String[] args) {new LayoutTest(); } }
Note: Cancel the layout manager (code example is as follows)
You can use absolute To specify the position and size of the component using coordinates, at this time, we need to call Container.setLayout(null), and then call the Container.setBounds() method.
import java.awt.Button;import java.awt.Frame;public class Test27 {// 例子,自定义按钮的位置public static void main(String[] args) { Frame f = new Frame(); f.setSize(500, 400); f.setLayout(null); // 取消布局管理器Button btn = new Button("这是按钮"); btn.setBounds(50, 50, 80, 40); f.add(btn); f.setVisible(true); } }
2. Dialog box (Dialog)
Dialog boxes are often seen when we use computers every day, so we won’t go into details here. Go directly to the code example and become familiar with the application.
Dialog dlg; Label lblMsg; TextField txtDir; TextArea txtFileList; = Frame("目录查看器"30, 30, 400, 400= TextField(30); btn = Button("显示文件"= TextArea(20, 35); = Dialog(f, "提示信息"); lblMsg = Label("输入的目录不正确"200, 200, 250, 100 0 ""= txtDir.getText(); File file = (file.isDirectory() &&=+ "\r\n"); dlg.setVisible(
The above is the detailed content of Introducing examples of layout managers and dialog boxes. For more information, please follow other related articles on the PHP Chinese website!
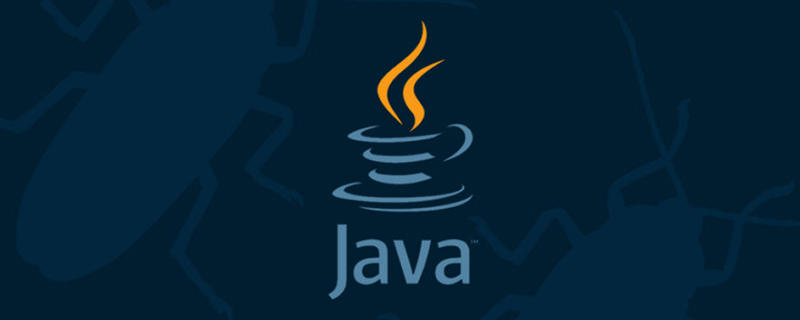
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
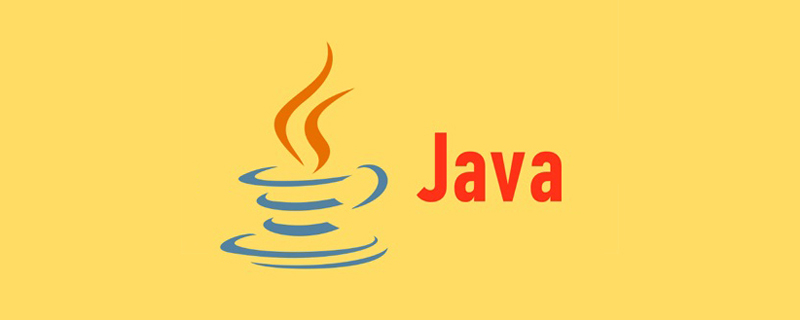
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
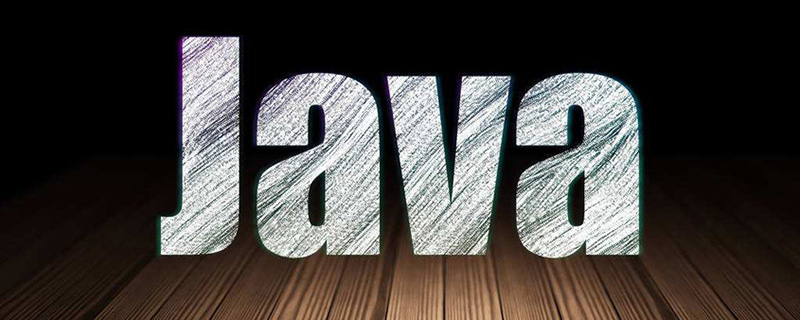
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
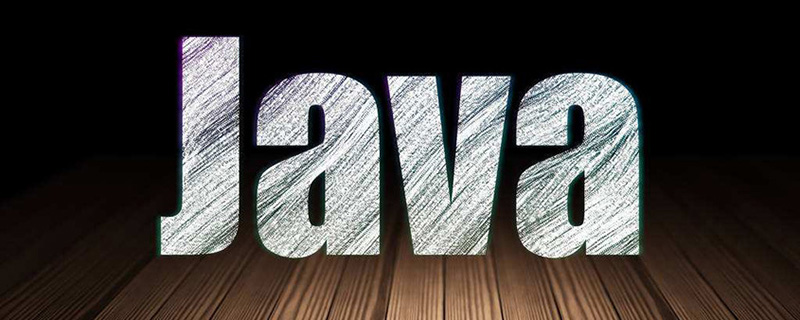
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
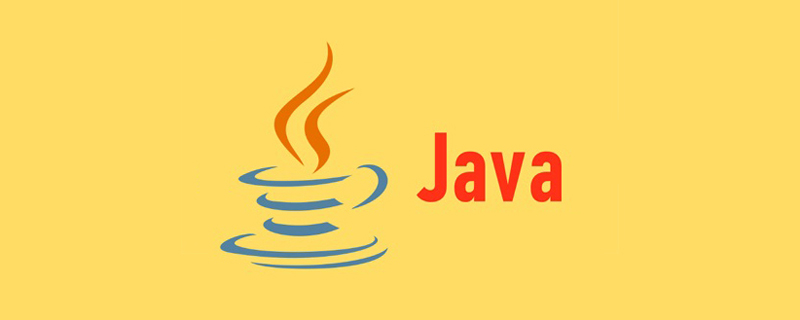
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
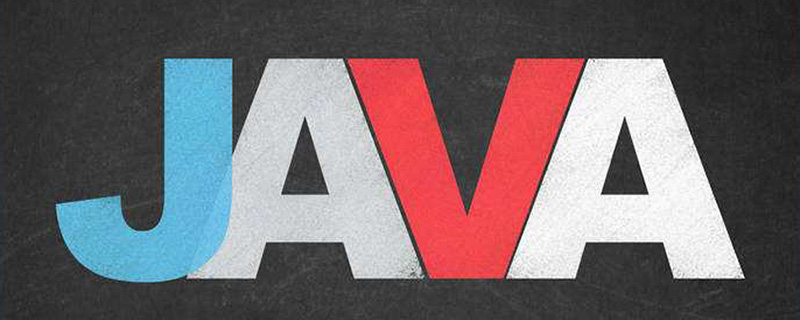
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
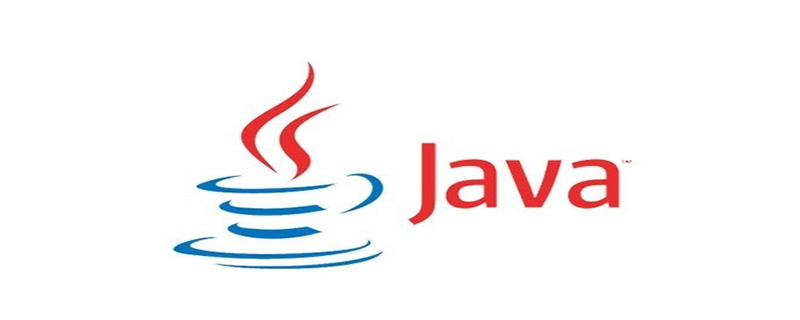
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于平衡二叉树(AVL树)的相关知识,AVL树本质上是带了平衡功能的二叉查找树,下面一起来看一下,希望对大家有帮助。
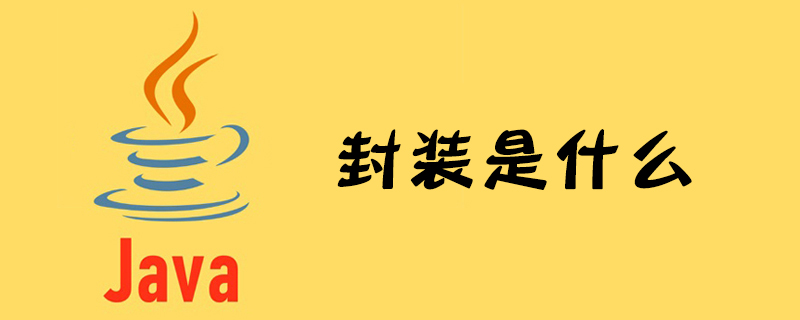
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Notepad++7.3.1
Easy-to-use and free code editor
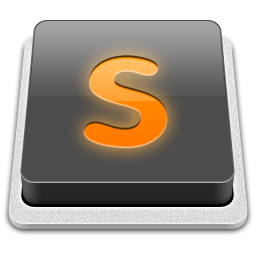
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Atom editor mac version download
The most popular open source editor
