Descriptions:
Given a sorted array, remove the duplicates in place such that each element appears only once and return the new length.
Do not allocate extra space for another array, you must do this in place with constant memory.For example,
Given input array nums =
[1,1,2]
,Your function should return length =
2
, with the first two elements of nums being1
and2
respectively. It doesn't 't matter what you leave beyond the new length.
I have always had problems with what I wrote... I used a HashSet collection and have not studied this type. The output result of [1,1,2] is always [1,1]
(Write it down in a notebook to study HashSet)
<code class="sourceCode java"><span class="kw">import java.util.HashSet;</span> <span class="kw">import java.util.Set;</span> <span class="kw">public</span> <span class="kw">class</span> Solution { <span class="kw">public</span> <span class="dt">static</span> <span class="dt">int</span> <span class="fu">removeDuplicates</span>(<span class="dt">int</span>[] nums) { Set<Integer> tempSet = <span class="kw">new</span> HashSet<>(); <span class="kw">for</span>(<span class="dt">int</span> i = <span class="dv">0</span>; i < nums.<span class="fu">length</span>; i++) { Integer wrap = Integer.<span class="fu">valueOf</span>(nums[i]); tempSet.<span class="fu">add</span>(wrap); } <span class="kw">return</span> tempSet.<span class="fu">size</span>(); } }</code>
The following are excellent answers
Solutions:
<code class="sourceCode java"><span class="kw">public</span> <span class="kw">class</span> Solution { <span class="kw">public</span> <span class="dt">static</span> <span class="dt">int</span> <span class="fu">removeDuplicates</span>(<span class="dt">int</span>[] nums) { <span class="dt">int</span> j = <span class="dv">0</span>; <span class="kw">for</span>(<span class="dt">int</span> i = <span class="dv">0</span>; i < nums.<span class="fu">length</span>; i++) { <span class="kw">if</span>(nums[i] != nums[j]) { nums[++j] = nums[i]; } } <span class="kw">return</span> ++j; } }</code>
There are two points to note:
- Because there may be multiple duplicates, equality cannot be used as the judgment condition
- Pay attention to the difference between
j++
and++j
. The usage here is very clever and necessary!
The above is the detailed content of LeetCode & Q26-Remove Duplicates from Sorted Array-Easy. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
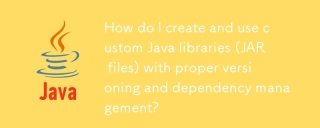
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
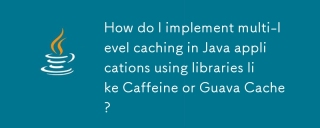
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
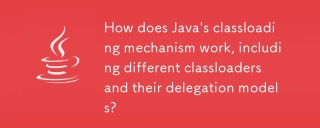
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor
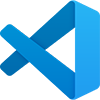
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Zend Studio 13.0.1
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software