//domain
package com.crazy.goods.tools;/** * 0755-351512 * @author Administrator * */public class Phone {private String qno;private String number;public String getQno() {return qno; }public void setQno(String qno) {this.qno = qno; }public String getNumber() {return number; }public void setNumber(String number) {this.number = number; } }
//Message Converter To implement an abstract class AbstractHttpMessageConverter
package com.crazy.goods.tools;import java.io.BufferedReader;import java.io.IOException;import java.io.InputStream;import java.io.InputStreamReader;import org.springframework.http.HttpInputMessage;import org.springframework.http.HttpOutputMessage;import org.springframework.http.converter.AbstractHttpMessageConverter;import org.springframework.http.converter.HttpMessageNotReadableException;import org.springframework.http.converter.HttpMessageNotWritableException;public class MyMessageConvertor extends AbstractHttpMessageConverter<phone> {/** * 将请求头数据转换成Phone */ @Overrideprotected Phone readInternal(Class extends Phone> arg0, HttpInputMessage msg) throws IOException, HttpMessageNotReadableException {//参数必须使用post提交必须在body中InputStream is=msg.getBody(); BufferedReader br=new BufferedReader(new InputStreamReader(is)); String param=br.readLine(); String phone=param.split("=")[1]; Phone phoneObj=new Phone(); phoneObj.setQno(phone.split("-")[0]); phoneObj.setNumber(phone.split("-")[1]);return phoneObj; }/** * 当前的转换器支持转换的类 */@Overrideprotected boolean supports(Class> arg0) {if(arg0==Phone.class){return true; }return false; }/** * 用于将返回的对象转换成字符串显示在网页 */@Overrideprotected void writeInternal(Phone phone, HttpOutputMessage arg1)throws IOException, HttpMessageNotWritableException { String p=phone.getQno()+"-"+phone.getNumber(); arg1.getBody().write(p.getBytes("UTF-8")); } }</phone>
//springmvc.xml To configure bean: message converter, only the post submission method will be intercepted by the converter
<?xml version="1.0" encoding="UTF-8"?> <beans> <!--springmvc只能扫描控制层 --> <component-scan> <exclude-filter></exclude-filter> <exclude-filter></exclude-filter> </component-scan> <!--消息转换器 必须使用post提交 --> <annotation-driven> <message-converters> <bean> <property> <list> <value>text/html;charset=UTF-8</value> <value>application/x-www-form-urlencoded</value> </list> </property> </bean> </message-converters> </annotation-driven> </beans>
servlet test
<br>
package com.crazy.goods.servlet;
<br>
import java.io.IOException;
<br>
import javax.servlet.ServletException;<br>import javax.servlet.annotation.WebServlet;<br>import javax.servlet.http. HttpServlet;<br>import javax.servlet.http.HttpServletRequest;<br>import javax.servlet.http.HttpServletResponse;
<br>
import org.springframework.stereotype.Controller;<br>import org.springframework. web.bind.annotation.PathVariable;<br>import org.springframework.web.bind.annotation.RequestBody;<br>import org.springframework.web.bind.annotation.RequestMapping;<br>import org.springframework.web. bind.annotation.RequestMethod;<br>import org.springframework.web.bind.annotation.ResponseBody;
<br>
import com.crazy.goods.tools.Phone;
<br>
/** <br> * @author Administrator<br> * Creation time: July 1, 2017 3:11:27 pm<br>*/<br>@Controller<br>public class ReservePageServelt {
<br>
// /**<br>// * forward: forward <br>// * redirect: redirect <br>// * @param req<br>// * @param resp<br>// * @return<br>// * @throws ServletException<br>// * @throws IOException<br>//*/<br>// @RequestMapping(value="/add",method ={RequestMethod.GET})<br>// public String doGet(HttpServletRequest req, HttpServletResponse resp/*,@PathVariable("testid") String testid*/) throws ServletException, IOException {<br>// req.getRequestDispatcher( "/reversegood.jsp").forward(req, resp);<br>// return "/reversegood.jsp";<br>// resp.getWriter().print(testid);<br>// } <br><br><br> //Message converter idea, <br><br> //The principle is to convert the request body or request header data into the parameters of the action method, and at the same time convert the content of the method's return value For the response header <br> //When the url path is accessed, the @RequestBody annotation is used. This annotation indicates that this class is to be processed by the message converter. The message converter will be read in the springmvcxml file, and then the supports method will be entered. <br> //Determine whether this class is supported by the specified converter. If it is supported, call the readInternal method, cut it, and then pass the value to the object. After processing is completed into the object, writeInternal will be called to convert into a response header<br> @RequestMapping(value="/add")<br> @ResponseBody<br> public Phone messageConvertor( @RequestBody Phone phone,HttpServletResponse response) {<br> System.out.println(phone.getQno()+phone. getNumber());<br> return phone;<br><br> }<br><br>}
<br>
Summary: The principle of the message converter is, Customize to convert the request body data into formal parameters (objects), and then convert the method's return value content into response headers
Steps:
When the url path is accessed, you will see the use The @RequestBody annotation indicates that this class is to be processed by the message converter. The message converter will be read in the springmvcxml file, and then the supports method <br> will be entered to determine whether this class is supported by the specified converter. If so, Call the readInternal method, cut, and then pass the value to the object.
After processing is completed into an object, writeInternal will be called to convert into a response header
The above is the detailed content of Example code of spring message converter. For more information, please follow other related articles on the PHP Chinese website!

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
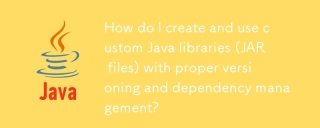
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
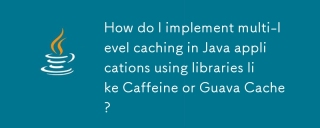
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra

The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
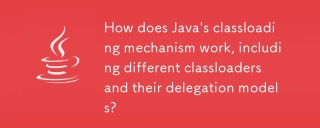
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
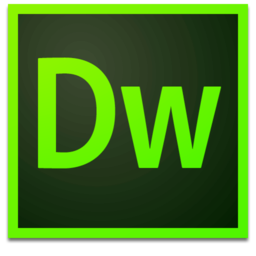
Dreamweaver Mac version
Visual web development tools
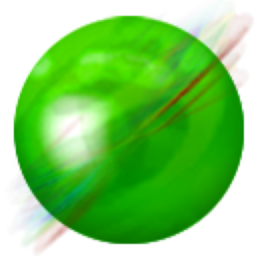
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment