The
re module brings full regular expression functionality to the Python language. The
compile function generates a regular expression object based on a pattern string and optional flag arguments. This object has a series of methods for regular expression matching and replacement.
re.match(pattern, string, flags=0) # 匹配成功返回一个匹配的对象,否则返回none
1 import re2 print(re.match('www', 'www.runoob.com').span()) # 在起始位置匹配 (0, 3)3 print(re.match('com', 'www.runoob.com')) # 不在起始位置匹配 None
1 import re 2 line = "cats are smarter than dogs" 3 matchObj = re.match(r'(.*) are (.*?) .*', line, re.M|re.I) 4 # matchObj = re.match(r'(.*) are (.*) .*', line, re.M|re.I) # 结果也一样 5 6 if matchObj: 7 print("matchObj.group()", matchObj.group()) # cats are smarter than dogs 8 print("matchObj.group(1)", matchObj.group(1)) # cats 9 print("matchObj.group(2)", matchObj.group(2)) # smarter10 else:11 print("No match!!")
1 import re 2 line = "cats are smarter than dogs" 3 matchObj = re.match(r'(.*) are (.*?) (.*) .*', line, re.M|re.I) 4 # matchObj = re.match(r'(.*) are (.*) (.*) .*', line, re.M|re.I) #这个结果也一样 5 6 if matchObj: 7 print("matchObj.group()", matchObj.group()) # cats are smarter than dogs 8 print("matchObj.group(1)", matchObj.group(1)) # cats 9 print("matchObj.group(2)", matchObj.group(2)) # smarter10 print("matchObj.group(3)", matchObj.group(3)) # than11 else:12 print("No match!!")
Use the group(num) or groups() matching object function to obtain the matching expression.
groups(): Returns a tuple containing all group strings, from 1 to the group number contained in .
re.search Scans the entire string and returns the first successful match.
print(re.search('www', 'www.runoob.com').span()) # 在起始位置匹配 (0, 3)print(re.search('com', 'www.runoob.com').span()) # 不在起始位置匹配 (11, 14)
1 import re2 line = "Cats are smarter than dogs";3 searchObj = re.search(r'(.*) are (.*?) .*', line, re.M | re.I)4 if searchObj:5 print("searchObj.group() : ", searchObj.group()) # Cats are smarter than dogs 6 print("searchObj.group(1) : ", searchObj.group(1)) # Cats7 print("searchObj.group(2) : ", searchObj.group(2)) # smarter8 else:9 print("Nothing found!!")
1 line = "Cats are smarter than dogs" 2 matchObj = re.match(r'dogs', line, re.M | re.I) 3 if matchObj: 4 print("match --> matchObj.group() : ", matchObj.group()) 5 else: 6 print("No match!!") 7 8 matchObj = re.search(r'dogs', line, re.M | re.I) 9 if matchObj:10 print("search --> matchObj.group() : ", matchObj.group())11 else:12 print("No match!!")13 '''14 No match!!15 search --> matchObj.group() : dogs16 '''
re.match only matches the beginning of the string. If the beginning of the string does not match the regular expression, the match fails and the function returns None; while re.search matches the entire string until a match is found.
1 re.search(pattern, string, flags=0)2 re.sub(pattern, repl, string, count=0, flags=0)
re module provides re.sub for replacing matches in strings.
Parameters:
#pattern: Pattern string in regular expression.
repl: The string to be replaced can also be a function.
string : The original string to be searched and replaced.
count: The maximum number of substitutions after pattern matching. The default value is 0, which means replacing all matches.
1 phone = "2004-959-559 # 国外电话号码"2 # 删除字符串中的Python注释3 num = re.sub(r'#.*$', "", phone)4 print("电话号码是:", num) # 电话号码是: 2004-959-559 5 6 # 删除非数字(-)的字符串7 num = re.sub(r'\D', "", phone)8 print("电话号码是:", num) # 电话号码是: 2004959559
repl parameter is a function
1 # 将匹配的数字乘于22 def double(matched):3 value = int(matched.group('value'))4 return str(value * 2)5 6 s = 'A23G4HFD567'7 print(re.sub('(?P<value>\d+)', double, s))</value>
re.I | Make matching case-insensitive | ||||||||||||||
Do locale-aware matching | |||||||||||||||
Multi-line matching, affects ^ and $ | |||||||||||||||
. Matches all characters including newlines. | |||||||||||||||
Parses characters according to the Unicode | character set. This flag affects \w, \W, \b, \B. | ||||||||||||||
by giving you more flexibility in formatting your regular expressions Written to be easier to understand. |
^ | Matches the beginning of the string |
$ | Matches the beginning of the string end. |
. | Matches any character except newline characters. When the re.DOTALL flag is specified, it can match any character including newline characters. |
[...] | is used to represent a group of characters, listed separately: [amk] matches 'a', 'm' or 'k' |
[^...] | Characters not in []: [^abc] matches characters other than a, b, c. |
re* | Matches 0 or more expressions. |
re+ | Matches one or more expressions. |
#re? | Match 0 or 1 fragment defined by the previous regular expression, non-greedy way |
re{ n} | |
re{ n,} | Exactly matches n previous expressions. |
re{ n, m} | Match n to m times the fragment defined by the previous regular expression, greedy way |
a| b | matches a or b |
(re) | G matches the expression in brackets and also represents a group |
(?imx) | Regular expressions contain three optional flags: i, m, or x. Only affects the area in brackets. |
(?-imx) | Regular expression turns off the i, m, or x optional flags. Only affects the area in brackets. |
(?: re) | Similar to (...), but does not represent a group |
(?imx : re) | Use i, m, or x in parentheses optional flag |
(?-imx: re) | in parentheses Do not use i, m, or x optional flags |
(?#...) | Comments. |
Forward positive delimiter. Succeeds if the contained regular expression, represented by ... , successfully matches the current position, otherwise it fails. But once the contained expression has been tried, the matching engine doesn't improve at all; the remainder of the pattern still has to try the right side of the delimiter. | |
Forward negative delimiter. Opposite of positive delimiter; succeeds when the contained expression cannot be matched at the current position of the string | |
Matched independent pattern, omitted Go backtrack. | |
Matches alphanumeric characters and underscores | |
Matches non-alphanumeric characters and underscores Underscore | |
matches any whitespace character, equivalent to [\t\n\r\f]. | |
Matches any non-empty character | |
Matches any number, equivalent to [0-9]. | |
Match any non-number | |
Match the beginning of the string | |
Matches the end of the string. If there is a newline, only the end of the string before the newline is matched. c | |
Matches the end of the string | |
Matches the position where the last match is completed. | |
Matches a word boundary, which refers to the position between a word and a space. For example, 'er\b' matches 'er' in "never" but not in "verb". | |
Matches non-word boundaries. 'er\B' matches 'er' in "verb" but not in "never". | |
matches a newline character. Matches a tab character. Wait | |
matches the content of the nth group. | |
Matches the content of the nth group if it is matched. Otherwise it refers to the expression of the octal character code. |
Matches "Python" or "python" | |||||||||||||||
Match "ruby" or "rube" | |||||||||||||||
Match any letter within the square brackets | |||||||||||||||
Matches any number. Similar to [0123456789] | |||||||||||||||
matches any lowercase letter | |||||||||||||||
Match any uppercase letters | |||||||||||||||
Match any letters and numbers | ##[ ^aeiou] | ||||||||||||||
[^0-9] | |||||||||||||||
. | Matches any single character except "\n". To match any character including '\n', use a pattern like '[.\n]'. |
\d | Matches a numeric character. Equivalent to [0-9]. |
\D | Matches a non-numeric character. Equivalent to [^0-9]. |
\s | Matches any whitespace character, including spaces, tabs, form feeds, etc. Equivalent to [ \f\n\r\t\v]. |
\S | Matches any non-whitespace character. Equivalent to [^ \f\n\r\t\v]. |
\w | Matches any word character including an underscore. Equivalent to '[A-Za-z0-9_]'. |
\W | Matches any non-word character. Equivalent to '[^A-Za-z0-9_]'. |
r indicates that the string is an unescaped raw string, allowing the compiler to ignore backslashes, that is, to ignore escape characters.
(.*)The first matching group, * means matching all characters except newline characters.
(.*?) The second matching group, *? followed by multiple question marks, represents non-greedy mode, that is to say, only the minimum characters that meet the conditions are matched
The last one.* None Surrounded by parentheses, it is not grouped. The matching effect is the same as the first one, but it is not included in the matching result.
The above is the detailed content of How to learn python regular expressions?. For more information, please follow other related articles on the PHP Chinese website!
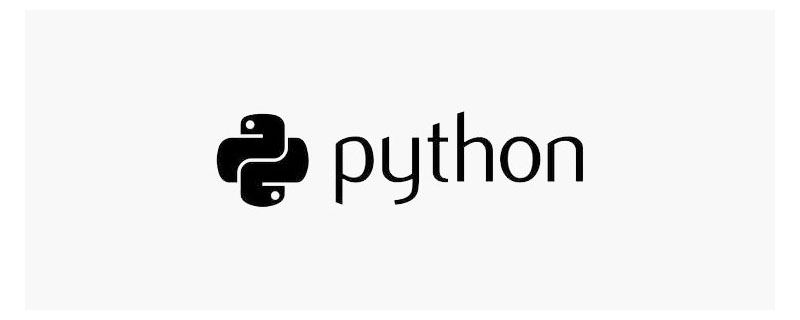
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于Seaborn的相关问题,包括了数据可视化处理的散点图、折线图、条形图等等内容,下面一起来看一下,希望对大家有帮助。
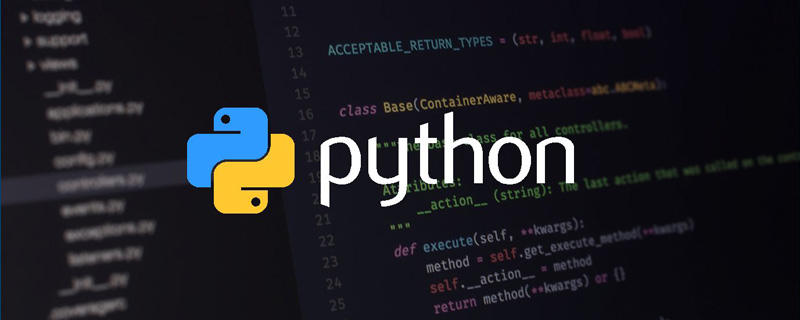
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于进程池与进程锁的相关问题,包括进程池的创建模块,进程池函数等等内容,下面一起来看一下,希望对大家有帮助。
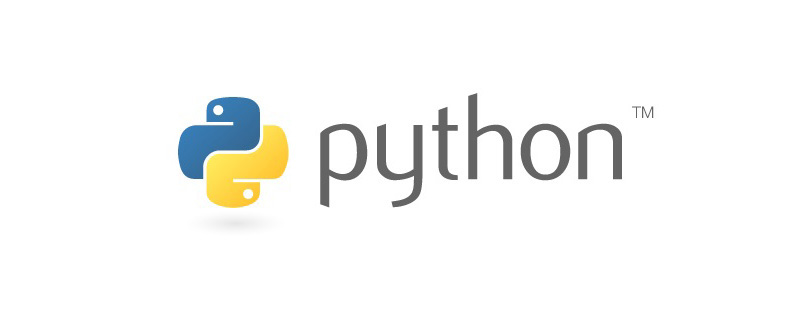
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于简历筛选的相关问题,包括了定义 ReadDoc 类用以读取 word 文件以及定义 search_word 函数用以筛选的相关内容,下面一起来看一下,希望对大家有帮助。
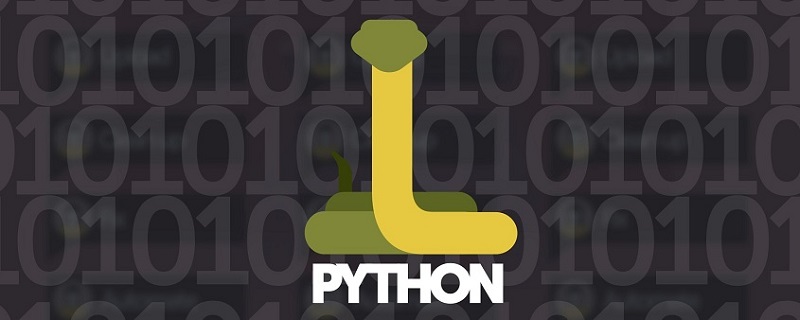
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于数据类型之字符串、数字的相关问题,下面一起来看一下,希望对大家有帮助。
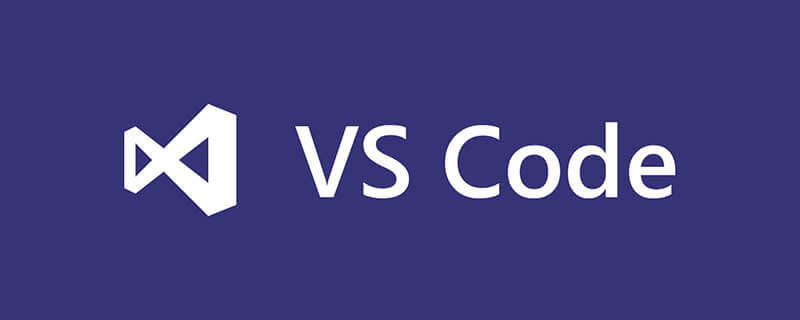
VS Code的确是一款非常热门、有强大用户基础的一款开发工具。本文给大家介绍一下10款高效、好用的插件,能够让原本单薄的VS Code如虎添翼,开发效率顿时提升到一个新的阶段。
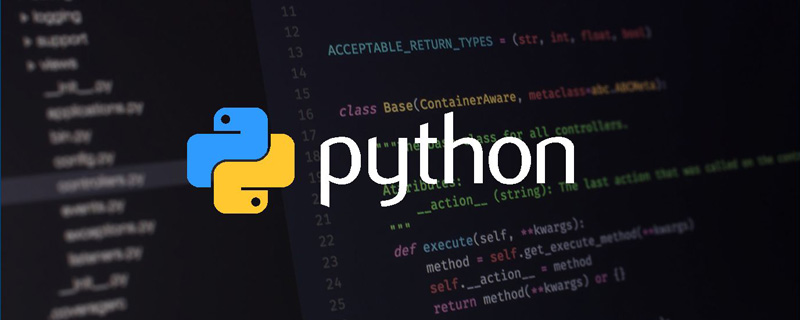
本篇文章给大家带来了关于Python的相关知识,其中主要介绍了关于numpy模块的相关问题,Numpy是Numerical Python extensions的缩写,字面意思是Python数值计算扩展,下面一起来看一下,希望对大家有帮助。
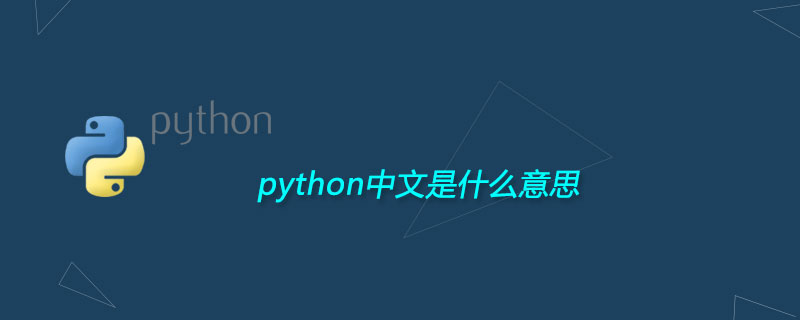
pythn的中文意思是巨蟒、蟒蛇。1989年圣诞节期间,Guido van Rossum在家闲的没事干,为了跟朋友庆祝圣诞节,决定发明一种全新的脚本语言。他很喜欢一个肥皂剧叫Monty Python,所以便把这门语言叫做python。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
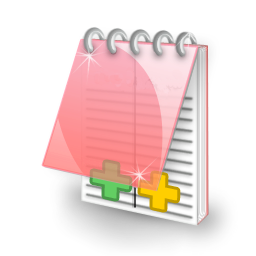
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
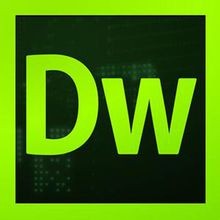
Dreamweaver CS6
Visual web development tools

SublimeText3 Chinese version
Chinese version, very easy to use

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
