In Java, object-oriented programming has three major characteristics: Encapsulation, Inheritance, Polymorphism .
Let’s talk about encapsulation first.
Encapsulation, as the name suggests, is through Abstract Data Type (i.e. ADT, a data type and the possibility to defined for operations) encapsulates data and data-based operations together, making them independent "entities".
First let’s look at a Person class:
1 public class Person implements Comparable<person> { 2 private String firstname; 3 private String lastname; 4 5 public String getFirstname() { 6 return firstname; 7 } public void setFirstname(String firstname) {10 this.firstname = firstname; } public String getLastname() {14 return lastname;15 }16 17 public void setLastname(String lastname) {18 this.lastname = lastname;19 } hash = 83 * hash + Objects.hashCode(this.firstname);25 ride30 return true;34 }35 //检查参数是否是正确的类型36 if (!(obj on)) {37 return false;38 }39 //将参数转换成正确的类型40 Person person = (Person) obj;41 //对实体域进行匹配42 return Objects.equals(this.firstname, person.firstname)43 && Objects.equals(this.lastname, person.lastname);44 }45 46 @Override47 public int compareTo(Person o) {48 if (this == o) {49 return 0;50 } if (null == o) {52 return 1;53 } //先判断姓氏是否相同55 int comparison = this.firstname.compareTo(o.firstname);56 if (comparison != 0) {57 return comparison;58 }59 //姓氏相同则比较名60 comparison = this.lastname.compareTo(o.lastname);61 return comparison;62 }63 }</person>
For the idea of encapsulation, we need tohide internal details as much as possible and only retain some external operations.
For example, in the Person class, I simply defined two member variables firstname and lastname. In the setter method, we can set some formats for the first and last names, such as the first letter is capitalized and the rest are lowercase for "formatting" ", open the getter to the outside world to obtain the value of the variable.
Now let’s summarize the advantages of encapsulation:
1. Can better control member variables and even the internal structure of the management class;
2. Good encapsulation It can reduce coupling and make the code more robust;
3. External programs can access it through the external interface without paying attention to implementation details.
Let’s talk about inheritance.
Inheritance describes the is-a relationship. It is a way of reusing code. The idea is that when a class is defined and implemented, it can be extended on an existing class. The content of the existing class is used as its own content. At the same time, new content can be added or the original method can be modified to adapt to different needs.
Let’s look at two examples:
1 public class Person { 2 3 private String name; 4 private String sex; 5 private int age; 6 7 Person(String name, String sex, int age) { 8 this.name = name; 9 this.sex = sex;10 this.age = age;11 }12 13 //省略setter和getter方法...14 37 }
1 public class Yang extends Person { 2 3 Yang(String name, String sex, int age) { 4 super(name, sex, age); 5 } 6 7 public String getName() { 8 return super.getName() + " is " + "genius"; 9 } }
1 public static void main(String... argc) {2 // Yang yang = new Yang("yang", "male", 23);3 Person person = new Yang("yang", "male", 23);4 out.print(person.getName());5 }
输出: yang is genius
注意,如果父类没有默认的构造器,子类构造函数中需要指定父类的构造器,否则编译将会失败!
从上面的代码中不得不引出关于继承的三大重要的东西,即构造器,protected关键字以及向上转型。
我们知道,构造器是不能被继承的,只许被调用!需要注意的是,子类是依赖于父类的(这也说明了一个弊端,即继承是一种强耦合关系),子类拥有父类的非private属性和方法(弊端二:破坏了封装),所以父类应先于子类被创建。
所以当父类没有默认构造器时,子类需要指定一个父类的构造器,并且写于子类构造器的第一行!当然父类有默认构造器,子类就无需super了,Java会自动调用。
再说protected关键字。插一句,只有合理使用访问修饰符,程序才能更好的为我们服务!!
对于子类,为了使用父类的方法,我们可以修改它的访问修饰符为protected,而不是一股脑儿的写上public!一劳永逸的做法可能会带来更大的危害!
而对于类的成员变量,保持它的private!
最后是向上转型了,它是一个重要的方面。从上面的的代码中,我写上了Person person = new Yang("yang", "male", 23); 这样结果是将Yang向上转型为Person,带来的影响可能就是属性和方法的丢失,但是它将是安全的。
同时它最大的作用是.....子类能够提供父类更加强大的功能以适用于不同的场合,完成不同的操作。
不太清楚可以看看这两个: List
我们知道ArrayList是数组实现,查找更快;而LinkedList是链表实现,添加元素和删除元素效率更好!
但是向上转型有一个弊端,指向子类的父类引用因为向上转型了,它将只拥有父类的属性和方法,同时子类拥有而父类没有的方法,是无法使用了的!
所以,继承实现了软件的可重用性和可拓展性。但是Java是单继承的,并且继承也有多个弊端(上面有提),其实还有一个弊端是父类一旦改变,子类可能也得进行改变!所以慎用吧。
最后一个特性是多态了。多态性就是不同的子类型可以对同一个请求做出不同的操作。同时多态性分为编译时多态性和运行时多态性,对应着方法重载overload和方法重写override!
对于方法重写,存在在继承中。它作为运行时多态性的表现,首先需要子类继承和实现父类的方法,然后向上转型,父类引用子类对象,对同一件事作出不同的响应。
方法重写时,需要注意的是子类的访问修饰符的访问范围不能小于父类,如父类的一个方法修饰符为protected,那么子类继承时,只能选用public或者protected。除了访问修饰符,其余完全相同于父类!
对于方法重载,出现在同一个类中,它是编译时多态性的表现。定义为:同名的方法如果有不同的参数列表便视为重载。
最后有一道经典的题目作为结尾,我也不知道出自哪....Look and think!
1 public class A { public String show(D obj) { 4 return ("Father and D"); 5 } 6 7 public String show(A obj) { 8 return ("Father and Father"); 9 } } class B extends A {13 14 public String show(B obj) {15 return ("Child and Child"); } public String show(A obj) {19 return ("Child and Father");20 }21 } class C extends B {24 }25 26 class D extends B {27 } class Test {30 public static void main(String[] args) {31 A a1 = new A();32 A a2 = new B();33 B b = new B();34 C c = new C();35 D d = new D();36 37 System.out.println("1--" + a1.show(b));38 System.out.println("2--" + a1.show(c));39 System.out.println("3--" + a1.show(d));40 System.out.println("4--" + a2.show(b));41 System.out.println("5--" + a2.show(c));42 System.out.println("6--" + a2.show(d));43 System.out.println("7--" + b.show(b));44 System.out.println("8--" + b.show(c));45 System.out.println("9--" + b.show(d));46 } }
The above is the detailed content of The three major features of Java-encapsulation, inheritance, and polymorphism. For more information, please follow other related articles on the PHP Chinese website!
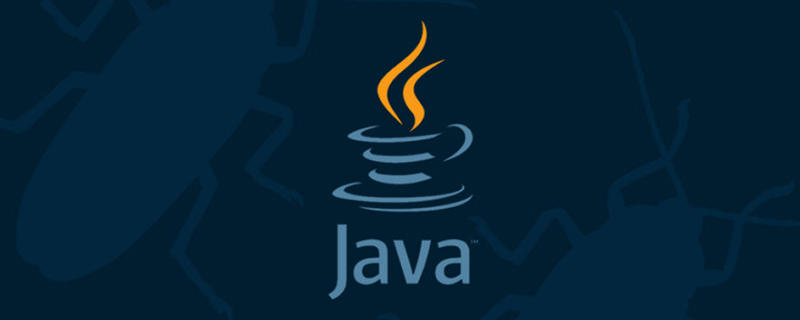
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于结构化数据处理开源库SPL的相关问题,下面就一起来看一下java下理想的结构化数据处理类库,希望对大家有帮助。
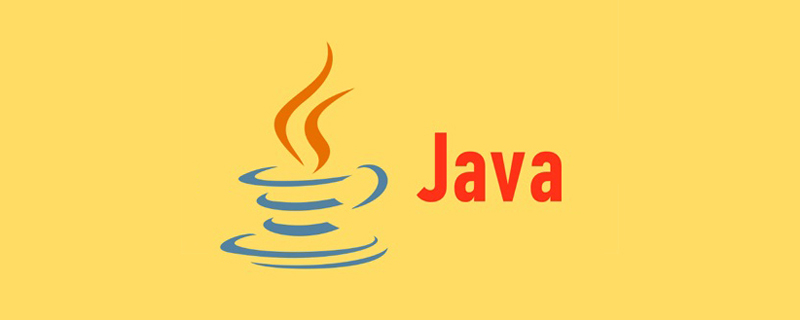
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于PriorityQueue优先级队列的相关知识,Java集合框架中提供了PriorityQueue和PriorityBlockingQueue两种类型的优先级队列,PriorityQueue是线程不安全的,PriorityBlockingQueue是线程安全的,下面一起来看一下,希望对大家有帮助。
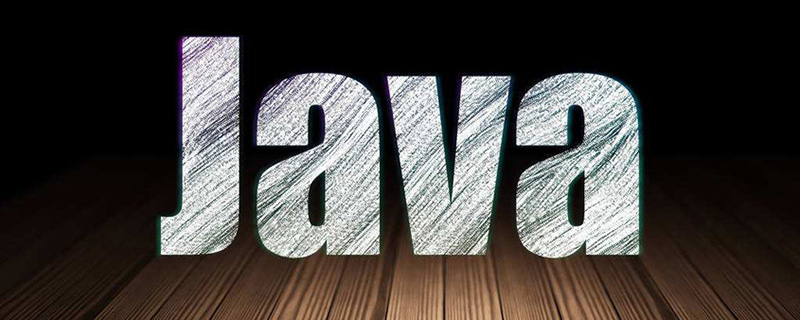
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于java锁的相关问题,包括了独占锁、悲观锁、乐观锁、共享锁等等内容,下面一起来看一下,希望对大家有帮助。
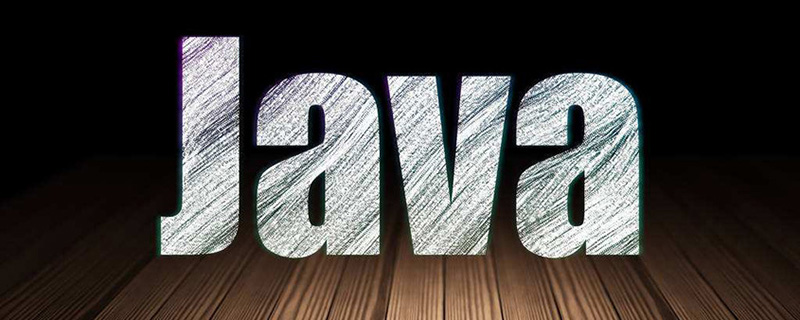
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于多线程的相关问题,包括了线程安装、线程加锁与线程不安全的原因、线程安全的标准类等等内容,希望对大家有帮助。
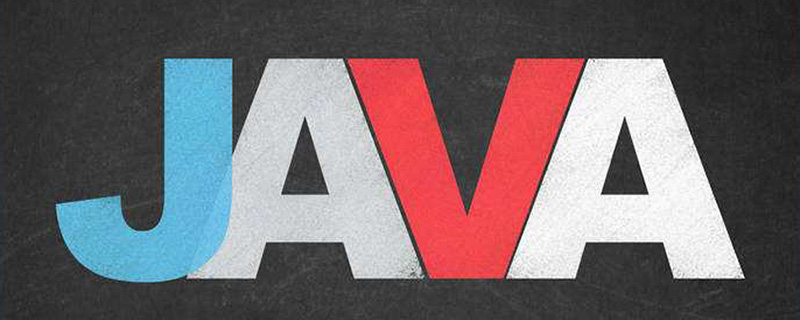
本篇文章给大家带来了关于Java的相关知识,其中主要介绍了关于关键字中this和super的相关问题,以及他们的一些区别,下面一起来看一下,希望对大家有帮助。
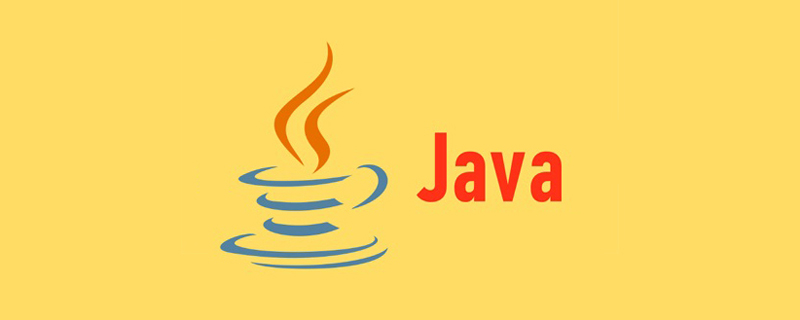
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于枚举的相关问题,包括了枚举的基本操作、集合类对枚举的支持等等内容,下面一起来看一下,希望对大家有帮助。
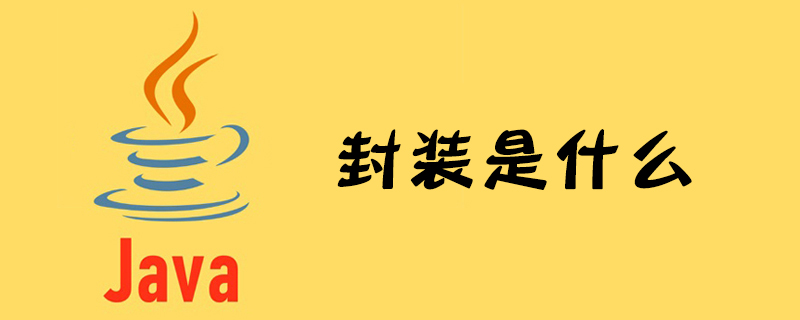
封装是一种信息隐藏技术,是指一种将抽象性函式接口的实现细节部分包装、隐藏起来的方法;封装可以被认为是一个保护屏障,防止指定类的代码和数据被外部类定义的代码随机访问。封装可以通过关键字private,protected和public实现。
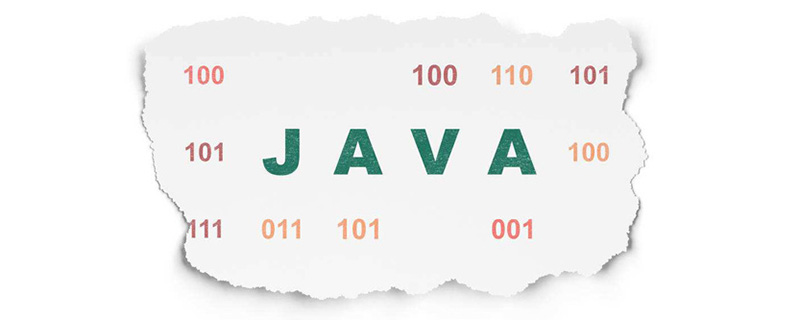
本篇文章给大家带来了关于java的相关知识,其中主要介绍了关于设计模式的相关问题,主要将装饰器模式的相关内容,指在不改变现有对象结构的情况下,动态地给该对象增加一些职责的模式,希望对大家有帮助。


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
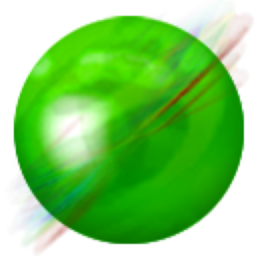
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
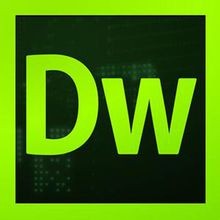
Dreamweaver CS6
Visual web development tools
